AbsoluteSizeSpan
public
class
AbsoluteSizeSpan
extends MetricAffectingSpan
implements
ParcelableSpan
java.lang.Object | |||
↳ | android.text.style.CharacterStyle | ||
↳ | android.text.style.MetricAffectingSpan | ||
↳ | android.text.style.AbsoluteSizeSpan |
A span that changes the size of the text it's attached to.
For example, the size of the text can be changed to 55dp like this:
SpannableString string = new SpannableString("Text with absolute size span");
string.setSpan(new AbsoluteSizeSpan(55, true), 10, 23, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
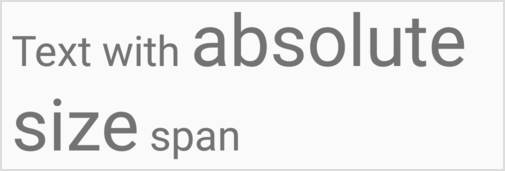
Summary
Inherited constants |
---|
Public constructors | |
---|---|
AbsoluteSizeSpan(int size)
Set the text size to |
|
AbsoluteSizeSpan(int size, boolean dip)
Set the text size to |
|
AbsoluteSizeSpan(Parcel src)
Creates an |
Public methods | |
---|---|
int
|
describeContents()
Describe the kinds of special objects contained in this Parcelable instance's marshaled representation. |
boolean
|
getDip()
Returns whether the size is in device-independent pixels or not, depending on the
|
int
|
getSize()
Get the text size. |
int
|
getSpanTypeId()
Return a special type identifier for this span class. |
String
|
toString()
Returns a string representation of the object. |
void
|
updateDrawState(TextPaint ds)
|
void
|
updateMeasureState(TextPaint ds)
Classes that extend MetricAffectingSpan implement this method to update the text formatting in a way that can change the width or height of characters. |
void
|
writeToParcel(Parcel dest, int flags)
Flatten this object in to a Parcel. |
Inherited methods | |
---|---|
Public constructors
AbsoluteSizeSpan
public AbsoluteSizeSpan (int size)
Set the text size to size
physical pixels.
Parameters | |
---|---|
size |
int |
AbsoluteSizeSpan
public AbsoluteSizeSpan (int size, boolean dip)
Set the text size to size
physical pixels, or to size
device-independent pixels if dip
is true.
Parameters | |
---|---|
size |
int |
dip |
boolean |
AbsoluteSizeSpan
public AbsoluteSizeSpan (Parcel src)
Creates an AbsoluteSizeSpan
from a parcel.
Parameters | |
---|---|
src |
Parcel : This value cannot be null . |
Public methods
describeContents
public int describeContents ()
Describe the kinds of special objects contained in this Parcelable
instance's marshaled representation. For example, if the object will
include a file descriptor in the output of writeToParcel(android.os.Parcel, int)
,
the return value of this method must include the
CONTENTS_FILE_DESCRIPTOR
bit.
Returns | |
---|---|
int |
a bitmask indicating the set of special object types marshaled
by this Parcelable object instance.
Value is either 0 or CONTENTS_FILE_DESCRIPTOR |
getDip
public boolean getDip ()
Returns whether the size is in device-independent pixels or not, depending on the
dip
flag passed in AbsoluteSizeSpan(int, boolean)
Returns | |
---|---|
boolean |
true if the size is in device-independent pixels, false
otherwise |
See also:
getSize
public int getSize ()
Get the text size. This is in physical pixels if getDip()
returns false or in
device-independent pixels if getDip()
returns true.
Returns | |
---|---|
int |
the text size, either in physical pixels or device-independent pixels. |
See also:
getSpanTypeId
public int getSpanTypeId ()
Return a special type identifier for this span class.
Returns | |
---|---|
int |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |
updateDrawState
public void updateDrawState (TextPaint ds)
Parameters | |
---|---|
ds |
TextPaint : This value cannot be null . |
updateMeasureState
public void updateMeasureState (TextPaint ds)
Classes that extend MetricAffectingSpan implement this method to update the text formatting in a way that can change the width or height of characters.
Parameters | |
---|---|
ds |
TextPaint : This value cannot be null . |
writeToParcel
public void writeToParcel (Parcel dest, int flags)
Flatten this object in to a Parcel.
Parameters | |
---|---|
dest |
Parcel : This value cannot be null . |
flags |
int : Additional flags about how the object should be written.
May be 0 or Parcelable.PARCELABLE_WRITE_RETURN_VALUE .
Value is either 0 or a combination of Parcelable.PARCELABLE_WRITE_RETURN_VALUE , and android.os.Parcelable.PARCELABLE_ELIDE_DUPLICATES |