Trang này cung cấp thông tin tổng quan về API cho doanh nghiệp, các tính năng và thay đổi về hành vi có trong Android 9.
Giao diện người dùng của hồ sơ công việc
Android 9 (API cấp 28) có các thay đổi về giao diện người dùng trong trình chạy mặc định để giúp người dùng tách biệt ứng dụng cá nhân và ứng dụng công việc. Các nhà sản xuất thiết bị hỗ trợ tính năng này có thể trình bày ứng dụng của người dùng trong các thẻ công việc và thẻ cá nhân riêng biệt. Chúng tôi cũng giúp người dùng thiết bị bật và tắt hồ sơ công việc dễ dàng hơn bằng cách đưa nút chuyển vào thẻ công việc của trình chạy.
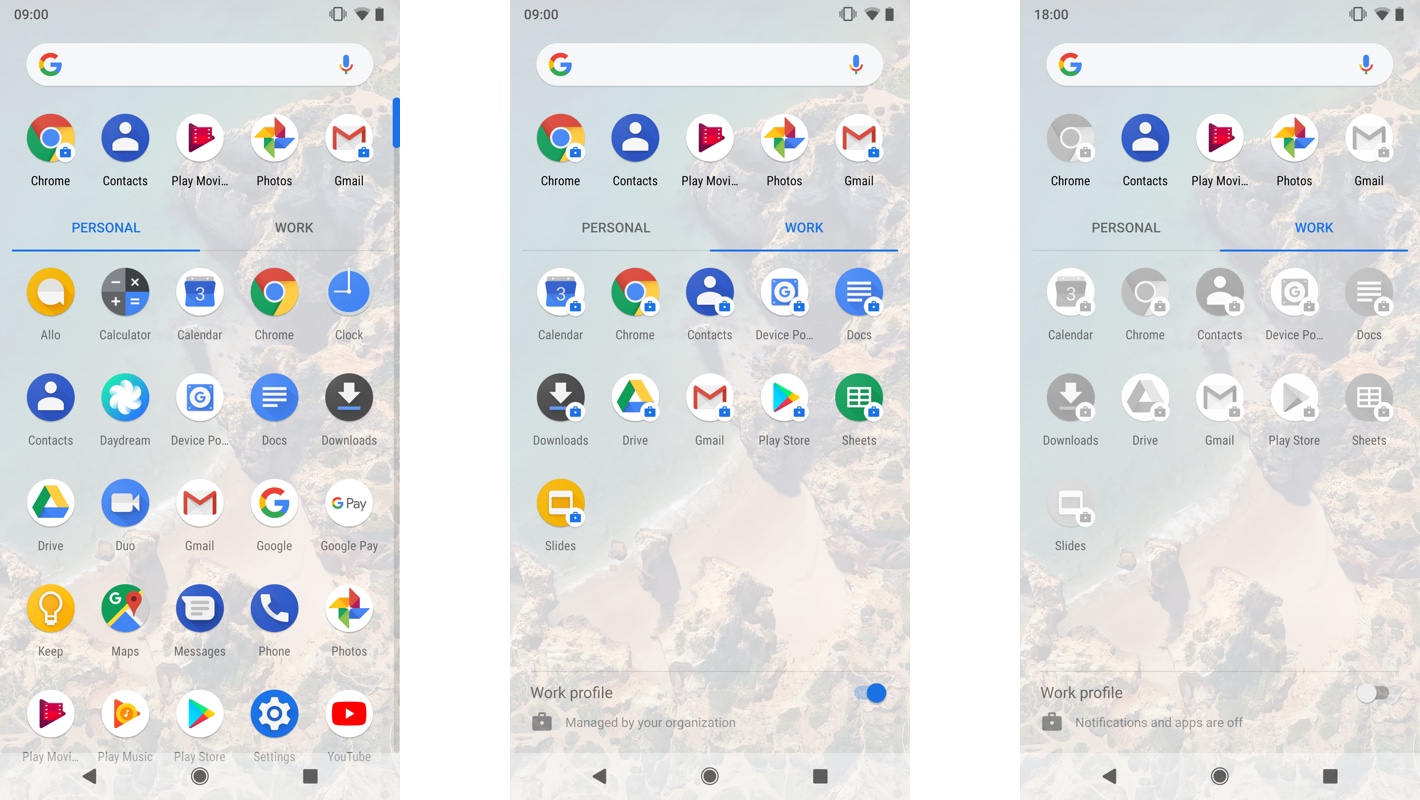
Khi cấp phép hồ sơ công việc và thiết bị được quản lý, Android 9 cung cấp hình minh hoạ động để giúp người dùng thiết bị hiểu được các tính năng này.
Chuyển đổi ứng dụng giữa các hồ sơ
Android 9 bao gồm các API để chạy một thực thể khác của ứng dụng trong hồ sơ khác nhằm giúp người dùng chuyển đổi giữa các tài khoản. Ví dụ: một ứng dụng email có thể cung cấp giao diện người dùng cho phép người dùng chuyển đổi giữa hồ sơ cá nhân và hồ sơ công việc để truy cập vào 2 tài khoản email. Tất cả ứng dụng đều có thể gọi các API này để chạy hoạt động chính của cùng một ứng dụng nếu ứng dụng đó đã được cài đặt trong hồ sơ khác. Để thêm tính năng chuyển đổi tài khoản giữa nhiều hồ sơ vào ứng dụng, hãy làm theo các bước bên dưới để gọi các phương thức của lớp CrossProfileApps
:
- Hãy gọi
getTargetUserProfiles()
để nhận danh sách hồ sơ mà bạn có thể chạy một thực thể khác của ứng dụng. Phương thức này sẽ kiểm tra để đảm bảo rằng ứng dụng đã được cài đặt trong các hồ sơ. - Gọi
getProfileSwitchingIconDrawable()
để nhận biểu tượng mà bạn có thể dùng để đại diện cho một hồ sơ khác. - Hãy gọi
getProfileSwitchingLabel()
để nhận văn bản đã bản địa hoá nhắc người dùng chuyển đổi hồ sơ. - Gọi
startMainActivity()
để chạy một thực thể của ứng dụng trong một hồ sơ khác.
Kiểm tra để đảm bảo hoạt động chính mà bạn muốn chạy được khai báo trong tệp kê khai của ứng dụng, với thao tác theo ý định ACTION_MAIN
và có chứa danh mục ý định CATEGORY_LAUNCHER
.
Bật hoặc tắt hồ sơ công việc theo phương thức lập trình
Trình chạy mặc định (hoặc các ứng dụng có quyền MANAGE_USERS
hoặc MODIFY_QUIET_MODE
) có thể bật hoặc tắt hồ sơ công việc bằng cách gọi UserManager.requestQuietModeEnabled()
. Bạn có thể kiểm tra giá trị trả về để biết người dùng có cần xác nhận thông tin đăng nhập của họ trước khi trạng thái thay đổi hay không. Vì thay đổi có thể không xảy ra ngay lập tức, hãy theo dõi thông báo ACTION_MANAGED_PROFILE_AVAILABLE
hoặc ACTION_MANAGED_PROFILE_UNAVAILABLE
để biết thời điểm cập nhật giao diện người dùng.
Ứng dụng của bạn có thể kiểm tra trạng thái của hồ sơ công việc bằng cách gọi UserManager.isQuietModeEnabled()
.
Khoá bất kỳ ứng dụng nào vào một thiết bị
Kể từ Android 9, chủ sở hữu thiết bị và chủ sở hữu hồ sơ (người dùng phụ) có thể khoá bất kỳ ứng dụng nào khỏi màn hình thiết bị bằng cách đặt ứng dụng đó ở chế độ khoá tác vụ. Trước đây, nhà phát triển ứng dụng phải thêm tính năng hỗ trợ cho chế độ khoá tác vụ trong ứng dụng của họ. Android 9 cũng mở rộng các API tác vụ khoá cho chủ sở hữu hồ sơ của người dùng phụ không liên kết. Hãy làm theo các bước dưới đây để khoá một ứng dụng vào màn hình:
- Gọi
DevicePolicyManager.setLockTaskPackages()
để đưa các ứng dụng vào danh sách cho phép của chế độ khoá tác vụ. - Gọi
ActivityOptions.setLockTaskEnabled()
để chạy một ứng dụng trong danh sách cho phép ở chế độ khoá tác vụ.
Để dừng một ứng dụng ở chế độ khoá tác vụ, hãy xoá ứng dụng đó khỏi danh sách cho phép của chế độ khoá tác vụ bằng cách sử dụng DevicePolicyManager.setLockTaskPackages()
.
Bật các tính năng giao diện người dùng hệ thống
Khi bạn bật chế độ khoá tác vụ, chủ sở hữu thiết bị và chủ sở hữu hồ sơ có thể bật một số tính năng giao diện người dùng hệ thống trên thiết bị bằng cách gọi DevicePolicyManager.setLockTaskFeatures()
và truyền trường bit của các cờ tính năng sau đây:
LOCK_TASK_FEATURE_NONE
LOCK_TASK_FEATURE_SYSTEM_INFO
LOCK_TASK_FEATURE_HOME
- Bạn chỉ có thể sử dụng
LOCK_TASK_FEATURE_NOTIFICATIONS
kết hợp vớiLOCK_TASK_FEATURE_HOME
. LOCK_TASK_FEATURE_KEYGUARD
- Bạn chỉ có thể sử dụng
LOCK_TASK_FEATURE_OVERVIEW
kết hợp vớiLOCK_TASK_FEATURE_HOME
. LOCK_TASK_FEATURE_GLOBAL_ACTIONS
Bạn có thể gọi DevicePolicyManager.getLockTaskFeatures()
để nhận danh sách các tính năng có trên thiết bị khi chế độ khoá tác vụ đang bật. Khi thoát khỏi chế độ khoá tác vụ, thiết bị sẽ trở về trạng thái bắt buộc theo các chính sách thiết bị khác.
Loại bỏ hộp thoại lỗi
Trong một số môi trường, chẳng hạn như minh hoạ bán lẻ hoặc hiển thị thông tin công khai, bạn có thể không muốn hiển thị hộp thoại lỗi cho người dùng. Trình kiểm soát chính sách thiết bị (DPC) có thể ngăn hộp thoại lỗi hệ thống cho các ứng dụng gặp sự cố hoặc không phản hồi bằng cách thêm quy tắc hạn chế người dùng DISALLOW_SYSTEM_ERROR_DIALOGS
. Quy định hạn chế này ảnh hưởng đến tất cả hộp thoại khi chủ sở hữu thiết bị áp dụng, nhưng chỉ các hộp thoại lỗi hiển thị trong người dùng chính hoặc phụ mới bị chặn khi chủ sở hữu hồ sơ áp dụng quy định hạn chế này. Quy định hạn chế này không ảnh hưởng đến hồ sơ công việc.
Trên Android 9, các ứng dụng chạy ở chế độ toàn màn hình sống động sẽ không hiển thị bong bóng lời nhắc khi ở chế độ khoá tác vụ. Bong bóng lời nhắc là một bảng điều khiển mà người dùng nhìn thấy (trong lần khởi chạy đầu tiên) giải thích cách thoát khỏi chế độ hiển thị tối đa.
Hỗ trợ nhiều người dùng trên các thiết bị chuyên dụng
Android 9 ra mắt khái niệm về người dùng tạm thời dành cho các thiết bị chuyên dụng (trước đây gọi là thiết bị cosU). Người dùng tạm thời là người dùng ngắn hạn, dành cho các trường hợp nhiều người dùng dùng chung một thiết bị chuyên dụng. Điều này bao gồm các phiên hoạt động công khai của người dùng trên các thiết bị như kiosk thẻ xác nhận phòng trong thư viện hoặc dịch vụ nhà hàng khách sạn, cũng như các phiên liên tục giữa một nhóm người dùng cố định trên các thiết bị, chẳng hạn như nhân viên làm việc theo ca.
Người dùng tạm thời phải được tạo trong nền. Chúng được tạo dưới dạng người dùng phụ trên một thiết bị và bị xoá (cùng với các ứng dụng và dữ liệu liên kết) khi bị dừng, chuyển đổi hoặc khởi động lại thiết bị. Để tạo người dùng tạm thời, chủ sở hữu thiết bị có thể:
- Đặt cờ
MAKE_USER_EPHEMERAL
khi gọiDevicePolicyManager.createAndManageUser()
. - Gọi
DevicePolicyManager.startUserInBackground()
để khởi động người dùng tạm thời trong nền.
Lưu ý rằng các ứng dụng nhắm đến Android 9 nên nắm bắt UserManager.UserOperationException
khi gọi createAndManageUser()
. Hãy gọi phương thức getUserOperationResult()
của ngoại lệ để tìm hiểu lý do tại sao người dùng không được tạo.
Nhận thông báo sự kiện
DeviceAdminReceiver
nhận thông báo về các sự kiện sau:
onUserStarted()
: Được gọi khi người dùng bắt đầu.onUserSwitched()
: Được gọi khi quá trình chuyển đổi người dùng hoàn tất.onUserStopped()
: Được gọi cùng vớionUserRemoved()
khi người dùng dừng hoặc đăng xuất.
Hiển thị thông báo sự kiện cho người dùng
Chủ sở hữu thiết bị có thể định cấu hình các thông báo hiển thị cho người dùng khi họ bắt đầu và kết thúc phiên hoạt động:
- Sử dụng
DevicePolicyManager.setStartUserSessionMessage()
để đặt thông báo mà người dùng nhìn thấy khi phiên của người dùng bắt đầu. Để truy xuất thông báo, hãy gọiDevicePolicyManager.getStartUserSessionMessage()
. - Sử dụng
DevicePolicyManager.setEndUserSessionMessage()
để đặt thông báo mà người dùng nhìn thấy khi phiên hoạt động của người dùng kết thúc. Để truy xuất thông báo, hãy gọiDevicePolicyManager.getEndUserSessionMessage()
.
Đăng xuất và ngừng người dùng
Chủ sở hữu thiết bị có thể sử dụng DevicePolicyManager.setLogoutEnabled()
để chỉ định xem có bật tính năng đăng xuất cho người dùng phụ hay không. Để kiểm tra xem bạn đã bật tính năng đăng xuất hay chưa, hãy gọi DevicePolicyManager.isLogoutEnabled()
.
Chủ sở hữu hồ sơ của người dùng phụ có thể gọi DevicePolicyManager.logoutUser()
để dừng người dùng phụ và chuyển về người dùng chính.
Chủ sở hữu thiết bị có thể sử dụng DevicePolicyManager.stopUser()
để dừng một người dùng phụ đã chỉ định.
Lưu gói vào bộ nhớ đệm
Để đơn giản hoá việc cấp phép cho người dùng trên thiết bị dùng chung đối với một nhóm người dùng cố định, chẳng hạn như thiết bị dành cho worker chuyển đổi, bạn có thể lưu các gói cần thiết cho phiên nhiều người dùng vào bộ nhớ đệm:
Gọi
DevicePolicyManager.setKeepUninstalledPackages()
để chỉ định danh sách các gói cần giữ lại làm tệp APK. Để truy xuất danh sách các gói này, hãy gọiDevicePolicyManager.getKeepUninstalledPackages()
.Gọi
DevicePolicyManager.installExistingPackage()
để cài đặt một gói đã được giữ lại sau khi xoá quasetKeepUninstalledPackages()
.
Phương thức và hằng số bổ sung
Android 9 cũng bao gồm các phương thức và hằng số sau đây để hỗ trợ thêm cho các phiên hoạt động của người dùng trên các thiết bị dùng chung:
DevicePolicyManager.getSecondaryUsers()
nhận danh sách tất cả người dùng phụ trên thiết bị.DISALLOW_USER_SWITCH
là một chế độ hạn chế người dùng mà bạn có thể bật bằng cách gọiDevicePolicyManager.addUserRestriction()
để chặn việc chuyển đổi người dùng.LEAVE_ALL_SYSTEM_APPS_ENABLED
là một cờ dành choDevicePolicyManager.createAndManageUser()
. Khi bạn đặt chính sách này, các ứng dụng hệ thống sẽ không tắt trong quá trình cấp phép người dùng.UserManager.UserOperationException
được gửi bởiDevicePolicyManager.createAndManageUser()
khi không thể tạo người dùng – trường hợp ngoại lệ sẽ chứa lý do không thành công.
Xoá dữ liệu gói và loại bỏ tài khoản
Chủ sở hữu thiết bị và chủ sở hữu hồ sơ có thể gọi clearApplicationUserData()
để xoá dữ liệu người dùng cho một gói cụ thể. Để xoá một tài khoản khỏi AccountManager
, chủ sở hữu thiết bị và hồ sơ có thể gọi removeAccount()
.
Hạn chế đối với người dùng và tăng cường quyền kiểm soát đối với các chế độ cài đặt
Android 9 đưa ra một bộ hạn chế đối với người dùng đối với DPC, cũng như khả năng định cấu hình APN, thời gian và múi giờ cũng như các chế độ cài đặt hệ thống trên thiết bị.
Định cấu hình APN
Chủ sở hữu thiết bị có thể dùng các phương thức sau trong lớp DevicePolicyManager
để định cấu hình APN trên một thiết bị:
addOverrideApn()
updateOverrideApn()
removeOverrideApn()
getOverrideApns()
setOverrideApnEnabled()
isOverrideApnEnabled()
Định cấu hình thời gian và múi giờ
Chủ sở hữu thiết bị có thể sử dụng các phương thức sau trong lớp DevicePolicyManager
để đặt giờ và múi giờ trên thiết bị:
Thực thi hạn chế người dùng đối với các chế độ cài đặt quan trọng
Android 9 bổ sung các hạn chế đối với người dùng để tắt các tính năng và chế độ cài đặt của hệ thống. Để thêm một quy tắc hạn chế, hãy gọi DevicePolicyManager.addUserRestriction()
với một trong các hằng số UserManager
sau:
DISALLOW_AIRPLANE_MODE
DISALLOW_AMBIENT_DISPLAY
DISALLOW_CONFIG_BRIGHTNESS
DISALLOW_CONFIG_DATE_TIME
DISALLOW_CONFIG_LOCATION
DISALLOW_CONFIG_SCREEN_TIMEOUT
DISALLOW_PRINTING
Nếu DISALLOW_CONFIG_BRIGHTNESS
và DISALLOW_CONFIG_SCREEN_TIMEOUT
được thực thi trên một thiết bị, chủ sở hữu thiết bị vẫn có thể đặt các chế độ cài đặt độ sáng màn hình, chế độ độ sáng màn hình và thời gian chờ màn hình trên thiết bị bằng API DevicePolicyManager.setSystemSetting()
.
Dữ liệu có đo lượng dữ liệu
Chủ sở hữu thiết bị và chủ sở hữu hồ sơ có thể ngăn ứng dụng dùng mạng dữ liệu có đo lượng dữ liệu của thiết bị. Mạng được coi là có đo lượng dữ liệu khi người dùng nhạy cảm với việc sử dụng nhiều dữ liệu do chi phí, giới hạn dữ liệu hoặc vấn đề về pin và hiệu suất. Để ngăn ứng dụng dùng mạng có đo lượng dữ liệu, hãy gọi DevicePolicyManager.setMeteredDataDisabledPackages()
để truyền danh sách tên gói. Để truy xuất các ứng dụng đang bị hạn chế, hãy gọi
DevicePolicyManager.getMeteredDataDisabledPackages()
.
Để tìm hiểu thêm về dữ liệu có đo lượng dữ liệu trong Android, hãy đọc bài viết Tối ưu hoá mức sử dụng dữ liệu mạng.
Di chuyển DPC
Trình kiểm soát chính sách thiết bị (DPC) có thể chuyển quyền sở hữu thiết bị hoặc hồ sơ công việc sang DPC khác. Bạn có thể chuyển quyền sở hữu để di chuyển một số tính năng sang API Quản lý Android, di chuyển thiết bị từ DPC cũ hoặc giúp quản trị viên CNTT di chuyển sang EMM của bạn. Vì chỉ thay đổi quyền sở hữu DPC nên bạn không thể sử dụng tính năng này để thay đổi hình thức quản lý, chẳng hạn như di chuyển từ một thiết bị được quản lý sang hồ sơ công việc hoặc ngược lại.
Bạn có thể sử dụng tài nguyên XML chính sách quản trị thiết bị để cho biết rằng phiên bản DPC này hỗ trợ tính năng di chuyển. DPC mục tiêu cho biết họ có thể nhận quyền sở hữu bằng cách thêm một phần tử có tên là <support-transfer-ownership>
. Ví dụ bên dưới cho thấy cách bạn có thể thực hiện việc này trong tệp XML quản trị thiết bị của DPC:
<device-admin xmlns:android="http://schemas.android.com/apk/res/android">
<support-transfer-ownership />
<uses-policies>
<limit-password />
<watch-login />
<reset-password />
</uses-policies>
</device-admin>
Các DPC muốn di chuyển quyền sở hữu sang ứng dụng DPC mới có thể kiểm tra xem phiên bản của DPC mục tiêu có hỗ trợ di chuyển hay không bằng cách gọi phương thức DeviceAdminInfo
supportsTransferOwnership()
. Trước khi chuyển quyền sở hữu, DPC nguồn có trách nhiệm xác minh DPC mục tiêu bằng cách so sánh chữ ký ứng dụng. Lớp PackageManager
bao gồm các phương thức để làm việc với chữ ký ký mã.
Android duy trì các chính sách người dùng và hệ thống của DPC nguồn thông qua hoạt động chuyển quyền sở hữu — các DPC không cần di chuyển những chính sách này. DPC nguồn có thể truyền dữ liệu tuỳ chỉnh đến DPC đích bằng cách sử dụng các cặp khoá-giá trị trong PersistableBundle
. Sau khi chuyển thành công, DPC mục tiêu có thể truy xuất dữ liệu này bằng cách gọi DevicePolicyManager.getTransferOwnershipBundle()
.
Các bước để chuyển quyền sở hữu của một thiết bị được quản lý hoặc hồ sơ công việc đều giống nhau:
- DPC nguồn sẽ kiểm tra để đảm bảo phiên bản của DPC mục tiêu có hỗ trợ quá trình di chuyển và xác nhận rằng chữ ký ứng dụng của DPC mục tiêu khớp với một giá trị dự kiến.
- DPC nguồn gọi
transferOwnership()
để bắt đầu quá trình chuyển. - Hệ thống đặt DPC mục tiêu làm quản trị viên đang hoạt động và đặt DPC đó làm chủ sở hữu của thiết bị được quản lý hoặc hồ sơ công việc.
- DPC mục tiêu nhận được lệnh gọi lại
onTransferOwnershipComplete()
và có thể tự định cấu hình bằng cách sử dụng các giá trị từ đối sốbundle
. - Nếu xảy ra sự cố trong quá trình chuyển, hệ thống sẽ chuyển quyền sở hữu về DPC nguồn. Nếu DPC nguồn của bạn cần xác nhận rằng quá trình chuyển quyền sở hữu đã thành công, hãy gọi
isAdminActive()
để kiểm tra nhằm đảm bảo rằng DPC nguồn không còn là quản trị viên đang hoạt động.
Tất cả ứng dụng chạy trong hồ sơ công việc đều nhận được thông báo ACTION_PROFILE_OWNER_CHANGED
khi chủ sở hữu hồ sơ thay đổi. Các ứng dụng chạy trên một thiết bị được quản lý sẽ nhận được thông báo ACTION_DEVICE_OWNER_CHANGED
khi chủ sở hữu thiết bị thay đổi.
Hồ sơ công việc trên các thiết bị được quản lý đầy đủ
Quá trình chuyển hai thực thể của DPC đang chạy với tư cách chủ sở hữu thiết bị và chủ sở hữu hồ sơ sẽ diễn ra trong hai giai đoạn. Khi hồ sơ cá nhân và hồ sơ công việc được liên kết, hãy hoàn tất quá trình chuyển theo thứ tự sau:
- Trước tiên, hãy chuyển quyền sở hữu hồ sơ công việc.
- Hãy chờ lệnh gọi lại
DeviceAdminReceiver
onTransferAffiliatedProfileOwnershipComplete()
để xác nhận rằng hồ sơ công việc đã được chuyển sang DPC đích. - Cuối cùng, hãy chuyển quyền sở hữu thiết bị được quản lý sang DPC đích.
Hoãn cập nhật qua mạng không dây (OTA)
Chủ sở hữu thiết bị có thể trì hoãn các bản cập nhật hệ thống OTA cho thiết bị trong tối đa 90 ngày để đóng băng phiên bản hệ điều hành đang chạy trên các thiết bị này trong các khoảng thời gian quan trọng (chẳng hạn như ngày lễ). Hệ thống sẽ thực thi một vùng đệm bắt buộc 60 ngày sau khoảng thời gian đóng băng đã xác định để ngăn việc đóng băng thiết bị vô thời hạn.
Trong thời gian khoá:
- Thiết bị không nhận được thông báo về các bản cập nhật OTA đang chờ xử lý.
- Thiết bị không cài đặt bản cập nhật hệ điều hành qua mạng không dây (OTA).
- Người dùng thiết bị không thể tự kiểm tra các bản cập nhật OTA trong phần Cài đặt.
Để đặt một khoảng thời gian đóng băng, hãy gọi SystemUpdatePolicy.setFreezePeriods()
. Vì khoảng thời gian đóng băng lặp lại hằng năm, nên ngày bắt đầu và ngày kết thúc của khoảng thời gian này được biểu thị bằng số nguyên đếm số ngày kể từ đầu năm. Ngày bắt đầu phải bắt đầu ít nhất 60 ngày sau khi kết thúc bất kỳ khoảng thời gian đóng băng nào trước đó. Chủ sở hữu thiết bị có thể gọi SystemUpdatePolicy.getFreezePeriods()
để lấy danh sách các khoảng thời gian đóng băng đã đặt trước đó cho đối tượng của chính sách cập nhật hệ thống.
DevicePolicyManager.getSystemUpdatePolicy()
đã được cập nhật để trả về bất kỳ khoảng thời gian đóng băng nào do chủ sở hữu thiết bị đặt.
Hạn chế chia sẻ trong hồ sơ công việc
Chủ sở hữu hồ sơ có thể ngăn người dùng chia sẻ dữ liệu cá nhân vào hồ sơ công việc trên thiết bị bằng cách thêm giới hạn đối với người dùng DISALLOW_SHARE_INTO_MANAGED_PROFILE
.
Quy định hạn chế này ngăn chặn việc xử lý và chia sẻ ý định sau đây:
- Ứng dụng hồ sơ cá nhân chia sẻ dữ liệu và tệp với ứng dụng hồ sơ công việc.
- Các ứng dụng hồ sơ công việc chọn các mục từ hồ sơ cá nhân (ví dụ: hình ảnh hoặc tệp).
Sau khi đặt quy tắc hạn chế này, DPC của bạn vẫn có thể cho phép các ý định Hoạt động trên nhiều hồ sơ bằng cách gọi addCrossProfileIntentFilter()
.
Khoá và chứng chỉ máy được bảo mật phần cứng
Android 9 bổ sung các API để giúp bạn thao tác với các khoá và chứng chỉ mà bạn có thể kết hợp để xác định thiết bị một cách an toàn. DPC chạy ở chế độ chủ sở hữu hồ sơ hoặc chủ sở hữu thiết bị hoặc trình cài đặt chứng chỉ được uỷ quyền có thể hoàn tất các tác vụ sau:
- Tạo khoá và chứng chỉ trong phần cứng bảo mật (chẳng hạn như môi trường thực thi đáng tin cậy (TEE) hoặc Secure Element (SE)) của thiết bị Android. Các khoá đã tạo không bao giờ rời khỏi phần cứng bảo mật và có thể được sử dụng từ KeyChain của Android. Gọi
DevicePolicyManager.generateKeyPair()
cung cấp thuật toán (xemKeyPairGenerator
) và mọi mã phần cứng mà bạn muốn chứng thực, chẳng hạn như số sê-ri hoặc IMEI. Để tìm hiểu thêm về những thay đổi về phần cứng bảo mật, hãy xem nội dung Các tính năng nâng cao bảo mật của Android 9. - Liên kết chứng chỉ với khoá hiện có do thiết bị tạo. Gọi
DevicePolicyManager.setKeyPairCertificate()
cung cấp bí danh của khoá hiện có và chuỗi chứng chỉ – bắt đầu bằng chứng chỉ leaf và bao gồm chuỗi tin cậy theo thứ tự. - Hãy xác nhận rằng phần cứng bảo mật đã bảo vệ khoá trước khi sử dụng. Để kiểm tra xem cơ chế nào bảo vệ khoá, hãy làm theo các bước trong phần Chứng thực khoá.
- Chủ sở hữu thiết bị và trình cài đặt chứng chỉ được uỷ quyền có thể nhận được câu lệnh đã ký về mã phần cứng của thiết bị theo phiên bản hệ thống Android. Gọi
DevicePolicyManager.generateKeyPair()
truyền một hoặc nhiềuID_TYPE_BASE_INFO
,ID_TYPE_SERIAL
,ID_TYPE_IMEI
hoặcID_TYPE_MEID
trong đối sốidAttestationFlags
. Chứng chỉ được trả về bao gồm mã nhận dạng phần cứng trong bản ghi chứng thực. Nếu bạn không muốn thêm mã nhận dạng phần cứng, hãy chuyển0
. Chủ sở hữu hồ sơ chỉ có thể nhận thông tin của nhà sản xuất (bằng cách truyềnID_TYPE_BASE_INFO
). Để kiểm tra xem thiết bị có thể chứng thực mã nhận dạng hay không, hãy gọiisDeviceIdAttestationSupported()
. - Ngăn người dùng thiết bị sử dụng khoá doanh nghiệp sai mục đích (trong các tác vụ không dành cho doanh nghiệp) bằng cách đặt các chứng chỉ khoá ở chế độ không thể chọn được. Hệ thống không bao gồm các chứng chỉ không thể chọn trong bảng điều khiển bộ chọn. Trong phương thức gọi lại
DeviceAdminReceiver.onChoosePrivateKeyAlias()
, hãy trả về bí danh cho khoá doanh nghiệp để hệ thống tự động chọn chứng chỉ thay cho người dùng. Để bỏ chọn một khoá, hãy gọi các phương thứcDevicePolicyManager
sau:setKeyPairCertificate()
và truyềnfalse
cho đối sốisUserSelectable
.installKeyPair (ComponentName, PrivateKey, Certificate[], String, int)
và loại bỏINSTALLKEY_SET_USER_SELECTABLE
khỏi đối sốflags
.
Bằng cách kết hợp các API này, doanh nghiệp có thể xác định thiết bị và xác nhận tính toàn vẹn của các thiết bị một cách an toàn trước khi cấp quyền truy cập:
- Thiết bị Android tạo một khoá riêng tư mới trong phần cứng bảo mật. Vì khoá riêng tư không bao giờ bị rời khỏi phần cứng bảo mật nên khoá này vẫn là khoá bí mật.
- Thiết bị sử dụng khoá để tạo và gửi một yêu cầu ký chứng chỉ (CSR) đến máy chủ. CSR bao gồm bản ghi chứng thực chứa mã thiết bị.
- Máy chủ xác thực chuỗi chứng chỉ (bắt nguồn từ một chứng chỉ của Google) và trích xuất siêu dữ liệu thiết bị từ bản ghi chứng thực.
- Máy chủ xác nhận rằng phần cứng bảo mật bảo vệ khoá riêng tư và mã thiết bị khớp với bản ghi của doanh nghiệp. Máy chủ cũng có thể kiểm tra để đảm bảo rằng hệ thống và phiên bản bản vá Android đáp ứng mọi yêu cầu.
- Máy chủ tạo chứng chỉ từ CSR và gửi chứng chỉ đó đến thiết bị.
- Thiết bị ghép nối chứng chỉ với khoá riêng tư (vẫn ở trong phần cứng bảo mật) để cho phép các ứng dụng kết nối với các dịch vụ dành cho doanh nghiệp.
Các thay đổi, tính năng và API bảo mật khác
Mã nhận dạng cho nhật ký bảo mật và nhật ký mạng
Android 9 đưa các mã nhận dạng vào nhật ký hoạt động mạng và bảo mật. Mã nhận dạng dạng số tăng đơn điệu cho mỗi sự kiện, giúp quản trị viên CNTT dễ dàng phát hiện khoảng trống trong nhật ký. Vì nhật ký bảo mật và nhật ký mạng là các bộ sưu tập riêng biệt, nên hệ thống sẽ duy trì các giá trị mã nhận dạng riêng biệt.
Gọi SecurityEvent.getId()
, DnsEvent.getId()
hoặc ConnectEvent.getId()
để nhận giá trị mã nhận dạng. Hệ thống sẽ đặt lại mã nhận dạng này bất cứ khi nào DPC bật tính năng ghi nhật ký hoặc khi thiết bị khởi động lại.
Nhật ký bảo mật được tìm nạp bằng cách gọi DevicePolicyManager.retrievePreRebootSecurityLogs()
không chứa các mã nhận dạng này.
Ghi nhật ký bảo mật
Tính năng ghi nhật ký bảo mật sẽ chỉ định mỗi SecurityEvent
một cấp độ nhật ký. Để lấy cấp độ nhật ký, hãy gọi getLogLevel()
. Phương thức này trả về một giá trị cấp độ nhật ký có thể là một trong các giá trị: LEVEL_INFO
, LEVEL_WARNING
hoặc LEVEL_ERROR
.
Android 9 ghi lại các sự kiện trong bảng dưới đây vào nhật ký bảo mật. Để kiểm tra thẻ của một sự kiện, hãy gọi getTag()
. Để truy xuất dữ liệu sự kiện, hãy gọi getData()
.
Thẻ | Nội dung mô tả sự kiện |
---|---|
TAG_CERT_AUTHORITY_INSTALLED |
Lần thử cài đặt chứng chỉ gốc mới vào bộ nhớ thông tin xác thực của hệ thống. |
TAG_CERT_AUTHORITY_REMOVED |
Lần thử xoá chứng chỉ gốc khỏi bộ nhớ thông tin xác thực của hệ thống. |
TAG_CERT_VALIDATION_FAILURE |
Chứng chỉ Wi-Fi không vượt qua được quy trình kiểm tra xác thực trong quá trình kết nối. |
TAG_CRYPTO_SELF_TEST_COMPLETED |
Hệ thống đã hoàn tất quy trình tự kiểm tra mật mã. |
TAG_KEYGUARD_DISABLED_FEATURES_SET |
Ứng dụng quản trị đã tắt các tính năng của màn hình khoá của hồ sơ công việc hoặc thiết bị. |
TAG_KEY_DESTRUCTION |
Thao tác xoá khoá mã hoá. |
TAG_KEY_GENERATED |
Lần thử tạo khoá mã hoá mới. |
TAG_KEY_IMPORT |
Lần thử nhập khoá mã hoá mới. |
TAG_KEY_INTEGRITY_VIOLATION |
Android đã phát hiện thấy khoá mã hoá hoặc xác thực bị hỏng. |
TAG_LOGGING_STARTED |
Đã bắt đầu ghi nhật ký bảo mật. |
TAG_LOGGING_STOPPED |
Tính năng ghi nhật ký bảo mật đã dừng ghi. |
TAG_LOG_BUFFER_SIZE_CRITICAL |
Bộ đệm nhật ký bảo mật đã đạt 90% dung lượng. |
TAG_MAX_PASSWORD_ATTEMPTS_SET |
Một ứng dụng quản trị đã đặt số lần nhập sai mật khẩu được phép. |
TAG_MAX_SCREEN_LOCK_TIMEOUT_SET |
Một ứng dụng quản trị đã đặt thời gian chờ khoá màn hình tối đa. |
TAG_MEDIA_MOUNT |
Thiết bị đã gắn phương tiện lưu trữ di động. |
TAG_MEDIA_UNMOUNT |
Thiết bị đã tháo phương tiện lưu trữ di động. |
TAG_OS_SHUTDOWN |
Hệ thống Android đã tắt. |
TAG_OS_STARTUP |
Hệ thống Android đã khởi động. |
TAG_PASSWORD_COMPLEXITY_SET |
Một ứng dụng quản trị đặt ra các yêu cầu về độ phức tạp của mật khẩu. |
TAG_PASSWORD_EXPIRATION_SET |
Một ứng dụng quản trị đặt khoảng thời gian hết hạn mật khẩu. |
TAG_PASSWORD_HISTORY_LENGTH_SET |
Một ứng dụng quản trị đặt độ dài nhật ký mật khẩu để ngăn người dùng sử dụng lại mật khẩu cũ. |
TAG_REMOTE_LOCK |
Ứng dụng quản trị đã khoá thiết bị hoặc hồ sơ công việc. |
TAG_USER_RESTRICTION_ADDED |
Một ứng dụng quản trị đã đặt giới hạn cho người dùng. |
TAG_USER_RESTRICTION_REMOVED |
Một ứng dụng quản trị đã loại bỏ chế độ hạn chế đối với người dùng. |
TAG_WIPE_FAILURE |
Không xóa được thiết bị hoặc hồ sơ công việc. |
Thử thách trên màn hình khoá hồ sơ công việc
Kể từ Android 9, chủ sở hữu hồ sơ có thể yêu cầu người dùng đặt một thử thách màn hình khoá riêng cho hồ sơ công việc của họ bằng cách sử dụng giới hạn người dùng DISALLOW_UNIFIED_PASSWORD
. Để kiểm tra xem người dùng có đặt cùng một thử thách trên màn hình khoá cho thiết bị và hồ sơ công việc của họ hay không, hãy gọi DevicePolicyManager.isUsingUnifiedPassword()
.
Nếu thiết bị có màn hình khoá riêng cho hồ sơ công việc, DevicePolicyManager.setMaximumTimeToLock()
sẽ chỉ đặt thời gian chờ khoá màn hình cho hồ sơ công việc thay vì cho toàn bộ thiết bị.
Quyền truy cập công cụ cho nhà phát triển
Để giúp lưu giữ dữ liệu công việc trong hồ sơ công việc, công cụ Cầu gỡ lỗi Android (adb) không thể truy cập vào các thư mục và tệp trong hồ sơ công việc.
Hỗ trợ nhiều tuỳ chọn sinh trắc học khác
Android 9 bổ sung quyền kiểm soát chi tiết đối với việc xác thực phần cứng sinh trắc học trên màn hình khoá của hồ sơ công việc. Gọi phương thức DevicePolicyManager.setKeyguardDisabledFeatures()
hiện có bằng KEYGUARD_DISABLE_FACE
và KEYGUARD_DISABLE_IRIS
.
Để tắt tất cả các phương thức xác thực bằng sinh trắc học do thiết bị cung cấp, hãy thêm KEYGUARD_DISABLE_BIOMETRICS
.
Ngừng sử dụng chính sách quản trị thiết bị
Android 9 đánh dấu các chính sách liệt kê dưới đây là không dùng nữa đối với DPC thông qua quản trị viên thiết bị. Các chính sách này sẽ tiếp tục hoạt động trong Android 9 như trước đây. Kể từ bản phát hành Android 10, các chính sách tương tự sẽ gửi một SecurityException khi quản trị viên thiết bị gọi.
USES_POLICY_DISABLE_CAMERA
USES_POLICY_DISABLE_KEYGUARD_FEATURES
USES_POLICY_EXPIRE_PASSWORD
USES_POLICY_LIMIT_PASSWORD
Một số ứng dụng sử dụng tính năng quản trị thiết bị để quản trị thiết bị của người dùng thông thường. Ví dụ: khoá và xoá sạch thiết bị thất lạc. Các chính sách sau đây sẽ tiếp tục có sẵn để cho phép việc này:
Để biết thêm thông tin về những thay đổi này, hãy đọc bài viết Ngừng sử dụng quản trị viên thiết bị.
Đơn giản hoá quy trình đăng ký bằng mã QR
Thư viện mã QR tích hợp sẵn
Android 9 đi kèm với thư viện QR để đơn giản hoá việc cấp phép thiết bị bằng mã QR. Quản trị viên CNTT không còn phải nhập thông tin chi tiết về Wi-Fi theo cách thủ công để thiết lập thiết bị. Thay vào đó, với Android 9, bạn có thể đưa các thông tin chi tiết về Wi-Fi này vào mã QR. Khi quản trị viên CNTT quét mã QR bằng thiết bị thuộc sở hữu của công ty, thiết bị sẽ tự động kết nối với Wi-Fi và chuyển sang quy trình cấp phép mà không cần thêm bất kỳ thao tác đầu vào thủ công nào.
Phương thức cấp phép bằng mã QR hỗ trợ các tiện ích bổ sung cấp phép sau đây để chỉ định thông tin chi tiết về Wi-Fi:
EXTRA_PROVISIONING_WIFI_HIDDEN
EXTRA_PROVISIONING_WIFI_PAC_URL
EXTRA_PROVISIONING_WIFI_PASSWORD
EXTRA_PROVISIONING_WIFI_PROXY_BYPASS
EXTRA_PROVISIONING_WIFI_PROXY_HOST
EXTRA_PROVISIONING_WIFI_PROXY_PORT
EXTRA_PROVISIONING_WIFI_SECURITY_TYPE
EXTRA_PROVISIONING_WIFI_SSID
Đặt ngày và múi giờ bằng cách sử dụng tiện ích bổ sung cấp phép
Phương thức cấp phép bằng mã QR hỗ trợ các ứng dụng khác để đặt thời gian và múi giờ trên thiết bị:
Tuỳ chọn xoá dữ liệu
Quản trị viên thiết bị có thể hiển thị thông báo dành riêng cho người dùng khi xoá hồ sơ công việc hoặc người dùng phụ. Thông báo này giúp người dùng thiết bị hiểu rằng quản trị viên CNTT của họ đã xoá hồ sơ công việc hoặc người dùng phụ. Gọi wipeData(int, CharSequence)
và cung cấp một thông báo giải thích ngắn. Khi được người dùng chính hoặc chủ sở hữu thiết bị gọi, hệ thống sẽ không hiển thị thông báo và bắt đầu đặt lại thiết bị về trạng thái ban đầu.
Để xoá dữ liệu gói thuê bao khỏi SIM eUICC đã nhúng, hãy gọi wipeData()
và đưa WIPE_EUICC
vào đối số flags
.
Phương thức dành cho chủ sở hữu trang doanh nghiệp liên kết
Các phương thức sau có sẵn cho chủ sở hữu hồ sơ được liên kết:
DevicePolicyManager.setKeyguardDisabled()
DevicePolicyManager.setStatusBarDisabled()
PackageInstaller.createSession()