ほとんどのマルチデバイス エクスペリエンスは、利用可能なデバイスを見つけることから始まります。この一般的なタスクを簡略化するために、Google は Device Discovery API を提供しています。
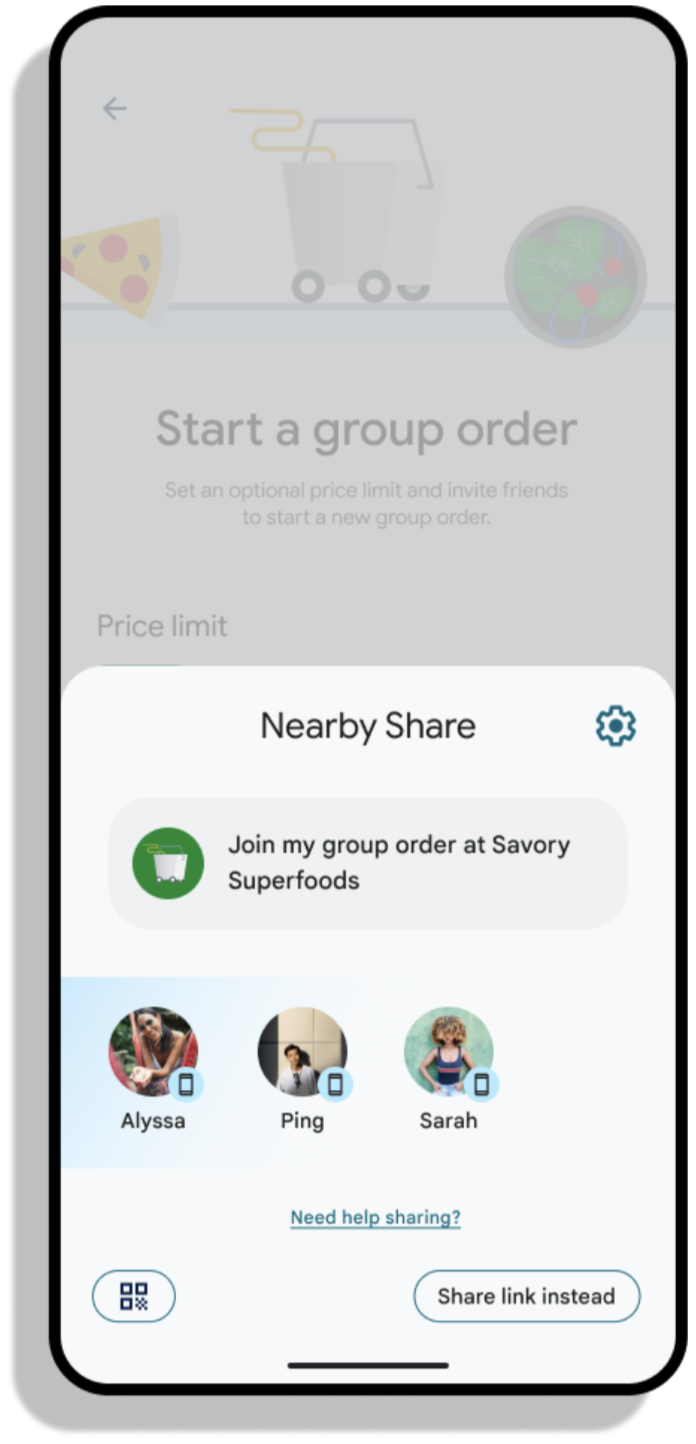
デバイス選択ダイアログの起動
デバイス検出では、システム ダイアログを使用してユーザーが対象デバイスを選択できるようにします。デバイス選択ダイアログを開始するには、まずデバイス検出クライアントを取得して結果レシーバを登録する必要があります。registerForActivityResult
と同様に、このレシーバはアクティビティまたはフラグメントの初期化パスの一部として無条件に登録する必要があります。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices); }
上記のコード スニペットには、未定義の handleDevices
オブジェクトがあります。ユーザーが接続先のデバイスを選択し、SDK が他のデバイスに正常に接続すると、このコールバックは選択された Participants
のリストを受け取ります。
Kotlin
handleDevices = OnDevicePickerResultListener { participants -> participants.forEach { // Use participant info } }
Java
handleDevices = participants -> { for (Participant participant : participants) { // Use participant info } }
デバイス選択ツールを登録したら、devicePickerLauncher
インスタンスを使用して起動します。DevicePickerLauncher.launchDevicePicker
は、デバイス フィルタのリスト(以下のセクションを参照)と startComponentRequest
の 2 つのパラメータを受け取ります。startComponentRequest
は、受信デバイスで開始する必要があるアクティビティと、ユーザーに表示されるリクエストの理由を示すために使用されます。
Kotlin
devicePickerLauncher.launchDevicePicker( listOf(), startComponentRequest { action = "com.example.crossdevice.MAIN" reason = "I want to say hello to you" }, )
Java
devicePickerLauncher.launchDevicePickerFuture( Collections.emptyList(), new StartComponentRequest.Builder() .setAction("com.example.crossdevice.MAIN") .setReason("I want to say hello to you") .build());
接続リクエストを承認する
ユーザーがデバイス選択ツールでデバイスを選択すると、受信デバイスにダイアログが表示され、ユーザーに接続を承認するよう求めることができます。受け入れられると、ターゲット アクティビティが起動されます。これは onCreate
と onNewIntent
で処理できます。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) handleIntent(getIntent()) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) handleIntent(intent) } private fun handleIntent(intent: Intent) { val participant = Discovery.create(this).getParticipantFromIntent(intent) // Accept connection from participant (see below) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); handleIntent(getIntent()); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); handleIntent(intent); } private void handleIntent(Intent intent) { Participant participant = Discovery.create(this).getParticipantFromIntent(intent); // Accept connection from participant (see below) }
デバイス フィルタ
デバイスを検出する際に、対象のユースケースに関連するデバイスのみが表示されるように、デバイスをフィルタするのが一般的です。次に例を示します。
- QR コードをスキャンできるように、カメラを搭載したデバイスのみに絞り込む
- テレビだけに絞って大画面の視聴体験を実現
このデベロッパー プレビューでは、まず、同じユーザーが所有するデバイスに絞り込む機能を導入します。
クラス DeviceFilter
を使用してデバイス フィルタを指定できます。
Kotlin
val deviceFilters = listOf(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY))
Java
List<DeviceFilter> deviceFilters = Arrays.asList(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY));
デバイス フィルタを定義したら、デバイスの検出を開始できます。
Kotlin
devicePickerLauncher.launchDevicePicker(deviceFilters, startComponentRequest)
Java
Futures.addCallback( devicePickerLauncher.launchDevicePickerFuture(deviceFilters, startComponentRequest), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // do nothing, result will be returned to handleDevices callback } @Override public void onFailure(Throwable t) { // handle error } }, mainExecutor);
launchDevicePicker
は、suspend
キーワードを使用する非同期関数です。