S'appuyant sur les API Device Discovery et Secure Connection, L'API Sessions fournit une abstraction puissante pour créer des applications multi-appareils fluides expériences. Une session représente une expérience utilisateur d'application qui peut être transférées et partagées entre les appareils.
L'API Sessions est également basée sur la notion de personnel et de communauté. expériences représentées par les cas d'utilisation de transfert et de partage de session respectivement. Le schéma suivant illustre les sessions dans les grandes lignes:
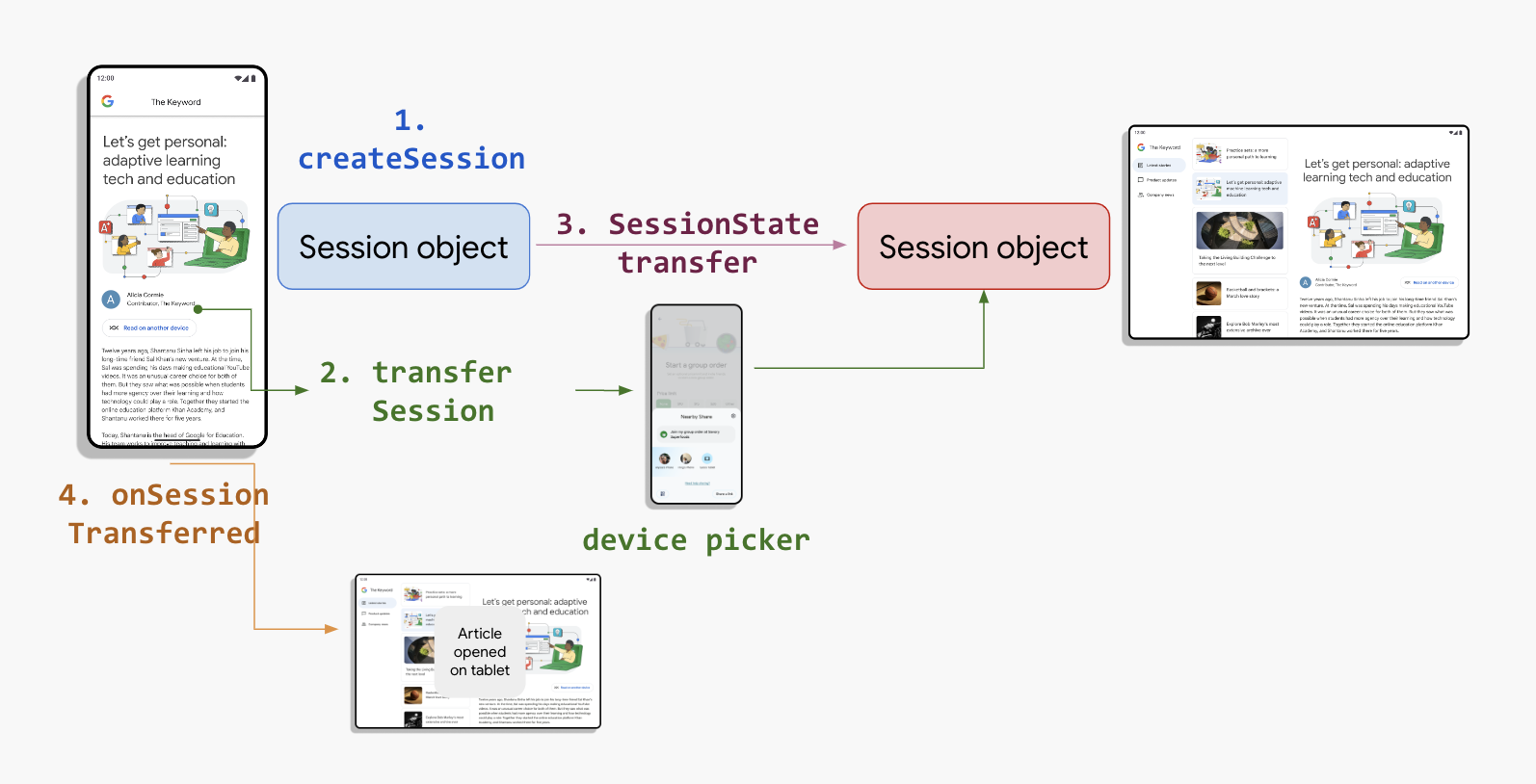
Créer et transférer une session
L'API Sessions fait la différence entre l'appareil d'origine et l'appareil destinataire. L'appareil d'origine crée la session et recherche un un appareil capable de gérer la session. Utilisateur de l'appareil d'origine sélectionne un appareil dans la liste fournie par la boîte de dialogue du système. Une fois que l’utilisateur sélectionne l'appareil destinataire, la session d'origine est transférée et supprimée de l'appareil d'origine.
Pour transférer la session, vous devez d'abord la créer à l'aide des éléments suivants : paramètres:
- Une balise de session d'application (un identifiant qui vous permet de différencier entre plusieurs sessions dans votre application.
Lancez ensuite le transfert à l'aide des paramètres suivants:
- Un
DeviceFilter
pour filtrer les appareils capables de gérer la session - Objet de rappel implémentant
OriginatingSessionStateCallback
Sur l'appareil d'origine, créez une session en utilisant l'exemple ci-dessous:
Kotlin
private val HELLO_WORLD_TRANSFER_ACTION = "hello_world_transfer" private lateinit var originatingSession: OriginatingSession private lateinit var sessions: Sessions override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) sessions = Sessions.create(context = this) } suspend fun transferSession() { val sessionId = sessions.createSession( ApplicationSessionTag("hello_world_transfer"), ) originatingSession = sessions.transferSession( sessionId, StartComponentRequest.Builder() .setAction(HELLO_WORLD_TRANSFER_ACTION) .setReason("Transfer reason here") .build(), emptyList(), HelloWorldTransferSessionStateCallback() ) }
Java
private static final String HELLO_WORLD_TRANSFER_ACTION = "hello_world_transfer"; private OriginatingSession originatingSession; private Sessions sessions; @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); sessions = Sessions.create(/* context= */ this); } public void transferSession() { SessionId sessionId = sessions.createSession(new ApplicationSessionTag("hello_world_transfer")); ListenableFuture<OriginatingSession> originatingSessionFuture = sessions.transferSessionFuture( sessionId, new StartComponentRequest.Builder() .setAction(HELLO_WORLD_TRANSFER_ACTION) .setReason("Transfer reason here") .build(), Collections.emptyList(), new HelloWorldTransferSessionStateCallback()); Futures.addCallback( originatingSessionFuture, new FutureCallback<>() { @Override public void onSuccess(OriginatingSession result) { // Do nothing, handled in HelloWorldTransferSessionStateCallback originatingSession = result; } @Override public void onFailure(Throwable t) { Log.d(TAG, "onFailure called for transferSessionFuture", t); } }, mainExecutor); }
Ensuite, définissez le rappel de session sur l'appareil d'origine:
Kotlin
private inner class HelloWorldTransferSessionStateCallback : OriginatingSessionStateCallback { override fun onConnected(sessionId: SessionId) { val startupRemoteConnection = originatingSession.getStartupRemoteConnection() lifecycleScope.launchWhenResumed { startupRemoteConnection.send("hello, world".toByteArray(UTF_8)) startupRemoteConnection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { val ok = payload.contentEquals("ok".toByteArray(UTF_8)) Log.d(TAG, "Session transfer initialized. ok=$ok") } } ) } } override fun onSessionTransferred(sessionId: SessionId) { Log.d(TAG, "Transfer done.") } override fun onTransferFailure(sessionId: SessionId, exception: SessionException) { // Handle error } }
Java
private class HelloWorldTransferSessionStateCallback implements OriginatingSessionStateCallback { @Override public void onConnected(SessionId sessionId) { SessionRemoteConnection startupRemoteConnection = originatingSession.getStartupRemoteConnection(); Futures.addCallback( startupRemoteConnection.sendFuture("hello, world".getBytes()), new FutureCallback<>() { @Override public void onSuccess(Void result) { Log.d(TAG, "Successfully sent initialization message"); } @Override public void onFailure(Throwable t) { Log.d(TAG, "Failed to send initialization message", t); } }, mainExecutor); } @Override public void onSessionTransferred(SessionId sessionId) { Log.d(TAG, "Transfer done."); } @Override public void onTransferFailure(SessionId sessionId, SessionException exception) { // Handle error } }
Une fois le transfert de session lancé, l'appareil destinataire reçoit un rappel
dans la méthode onNewIntent(intent: Intent)
. Les données d'intent contiennent tout
nécessaires au transfert de la session.
Pour terminer le transfert sur l'appareil destinataire:
Kotlin
private lateinit var sessions: Sessions override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) sessions = Sessions.create(context = this) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) lifecycleScope.launchWhenResumed { val receivingSession = sessions.getReceivingSession(intent, HelloWorldReceivingSessionStateCallback()) // Get info from receiving device and init. val startupRemoteConnection = receivingSession.getStartupRemoteConnection() startupRemoteConnection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { lifecycleScope.launchWhenResumed { val transferInfo = String(payload) startupRemoteConnection.send("ok".toByteArray(UTF_8)) // Complete transfer. Log.d(TAG, "Transfer info: " + transferInfo) receivingSession.onComplete() } } } ) } } private inner class HelloWorldReceivingSessionStateCallback : ReceivingSessionStateCallback { override fun onTransferFailure(sessionId: SessionId, exception: SessionException) { // Handle error } }
Java
private Sessions sessions; @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); sessions = Sessions.create(/* context= */ this); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); ListenableFuture<ReceivingSession> receivingSessionFuture = sessions.getReceivingSessionFuture(intent, new HelloWorldReceivingSessionStateCallback()); ListenableFuture<Void> registerReceiverFuture = Futures.transform( receivingSessionFuture, receivingSession -> { SessionRemoteConnection startupRemoteConnection = receivingSession.getStartupRemoteConnection(); SessionConnectionReceiver receiver = (participant, payload) -> { Log.d( TAG, "Successfully received initialization message of size: " + payload.length); applicationInitialization(receivingSession, payload); }; startupRemoteConnection.registerReceiver(receiver); return null; }, mainExecutor); Futures.addCallback( registerReceiverFuture, new FutureCallback<Void>() { @Override public void onSuccess(Void unused) { Log.d(TAG, "Connection receiver registerd successfully"); } @Override public void onFailure(Throwable t) { Log.w(TAG, "Failed to register connection receiver", t); } }, mainExecutor); } private void applicationInitialization(ReceivingSession receivingSession, byte[] initMessage) { ListenableFuture<SessionId> disconnectFuture = Futures.transform( receivingSession.onCompleteFuture(), sessionId -> { Log.d(TAG, "Succeeded to complete receive transfer for: " + sessionId); return sessionId; }, mainExecutor); Futures.addCallback( disconnectFuture, new FutureCallback<SessionId>() { @Override public void onSuccess(SessionId result) { Log.d(TAG, "Succeeded to remove the old session: " + result); } @Override public void onFailure(Throwable t) { Log.d(TAG, "Failed to remove the old session, which is now orphaned", t); } }, mainExecutor); } private static class HelloWorldReceivingSessionStateCallback implements ReceivingSessionStateCallback { @Override public void onTransferFailure(SessionId sessionId, SessionException exception) { // Handle error } }
L'appareil destinataire peut maintenant poursuivre l'expérience utilisateur.
Partager une session
En partageant une session, vous pouvez inviter d'autres personnes autour de vous à participer à un groupe par exemple:
- Partagez une position sur une carte en tant que passager directement avec la voiture de votre ami.
- Partagez votre itinéraire à vélo du dimanche avec les autres personnes avec lesquelles vous faites du vélo.
- Récupérez des articles pour une commande groupée de repas sans passer votre téléphone.
- Votez de groupe pour la prochaine série TV à regarder ensemble.
Lorsqu'un utilisateur choisit de partager une session avec un autre appareil, l'appareil d'origine recherche et présente les appareils capables de rejoindre la session et l'utilisateur sélectionne le ou les appareils récepteurs. L'application invite l'utilisateur appareil récepteur pour rejoindre la session à partir de l'appareil d'origine. A se voit attribuer une session secondaire pour interagir avec la session sur le appareil d'origine. Les applications permettent également d'ajouter des participants session partagée en cours.
Le processus de partage d'une session est
semblable au processus de transfert d'une session,
Au lieu d'appeler transferSession
, appelez shareSession
. L'autre
les différences concernent les méthodes
de rappel d'état de session.
Kotlin
// Originating side. private val HELLO_WORLD_SHARE_ACTION = "hello_world_share" private var activePrimarySession: PrimarySession? = null private lateinit var sessions: Sessions override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) sessions = Sessions.create(context = this) } suspend fun shareSession() { val sessionId = sessions.createSession(ApplicationSessionTag("hello_world_share")) activePrimarySession = sessions.shareSession( sessionId, StartComponentRequest.Builder() .setAction(HELLO_WORLD_SHARE_ACTION) .setReason("Share reason here") .build(), emptyList(), HelloWorldShareSessionStateCallback(), ) } private inner class HelloWorldShareSessionStateCallback : PrimarySessionStateCallback { override fun onShareInitiated(sessionId: SessionId, numPotentialParticipants: Int) { // Custom logic here for when n devices can potentially join. // e.g. if there were 0, cancel/error if desired, // if non-0 maybe spin until numPotentialParticipants join etc. } override fun onParticipantJoined(sessionId: SessionId, participant: SessionParticipant) { // Custom join logic here lifecycleScope.launchWhenResumed { // Example logic: send only to the participant who just joined. val connection = checkNotNull(activePrimarySession).getSecondaryRemoteConnectionForParticipant(participant) connection.send("Initing hello, world.".toByteArray(UTF_8)) connection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { val ok = payload.contentEquals("ok".toByteArray(UTF_8)) Log.d(TAG, "Session share initialized. ok=$ok") // Example logic: broadcast to all participants, including the one // that just joined. lifecycleScope.launchWhenResumed { checkNotNull(activePrimarySession) .broadcastToSecondaries("hello, all.".toByteArray(UTF_8)) } } } ) } } override fun onParticipantDeparted(sessionId: SessionId, participant: SessionParticipant) { // Custom leave logic here. } override fun onPrimarySessionCleanup(sessionId: SessionId) { // Custom cleanup logic here. activePrimarySession = null } override fun onShareFailureWithParticipant( sessionId: SessionId, exception: SessionException, participant: SessionParticipant ) { // Handle error } }
Java
// Originating side private static final String HELLO_WORLD_SHARE_ACTION = "hello_world_share"; @Nullable private PrimarySession activePrimarySession = null; private Sessions sessions; @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); sessions = Sessions.create(/* context= */ this); } private void shareSession() { SessionId sessionId = sessions.createSession(new ApplicationSessionTag("hello_world_share")); ListenableFuture<PrimarySession> shareSessionFuture = sessions.shareSessionFuture( sessionId, new StartComponentRequest.Builder() .setAction(HELLO_WORLD_SHARE_ACTION) .setReason("Share reason here") .build(), Collections.emptyList(), new HelloWorldShareSessionStateCallback()); Futures.addCallback( shareSessionFuture, new FutureCallback<PrimarySession>() { @Override public void onSuccess(PrimarySession primarySession) { activePrimarySession = primarySession; } @Override public void onFailure(Throwable t) { Log.d(TAG, "Failed to share session", t); } }, mainExecutor); } private class HelloWorldShareSessionStateCallback implements PrimarySessionStateCallback { @Override public void onShareInitiated(SessionId sessionId, int numPotentialParticipants) { // Custom logic here for when n devices can potentially join. // e.g. if there were 0, cancel/error if desired, // if non-0 maybe spin until numPotentialParticipants join etc. } @Override public void onParticipantJoined(SessionId sessionId, SessionParticipant participant) { PrimarySession joinedSession = activePrimarySession; if (joinedSession == null) { return; } SessionRemoteConnection connection = joinedSession.getSecondaryRemoteConnectionForParticipant(participant); Futures.addCallback( connection.sendFuture("Initiating hello, world.".getBytes()), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // Send successful. } @Override public void onFailure(Throwable t) { // Failed to send. } }, mainExecutor); connection.registerReceiver( new SessionConnectionReceiver() { @Override public void onMessageReceived(SessionParticipant participant, byte[] payload) { boolean ok = new String(payload, UTF_8).equals("ok"); Log.d(TAG, "Session share initialized. ok=" + ok); // Example logic: broadcast to all participants, including the one // that just joined. Futures.addCallback( joinedSession.broadcastToSecondariesFuture("hello, all.".getBytes()), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // Broadcast successful. } @Override public void onFailure(Throwable t) { // Failed to broadcast hello world. } }, mainExecutor); } }); } @Override public void onParticipantDeparted(SessionId sessionId, SessionParticipant participant) { // Custom leave logic here. } @Override public void onPrimarySessionCleanup(SessionId sessionId) { // Custom cleanup logic here. activePrimarySession = null; } @Override public void onShareFailureWithParticipant( SessionId sessionId, SessionException exception, SessionParticipant participant) { // Custom error handling logic here. } }
Côté réception:
Kotlin
// Receiving side. override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) lifecycleScope.launchWhenResumed { val secondarySession = sessions.getSecondarySession(intent, HelloWorldSecondaryShareSessionStateCallback()) val remoteConnection = secondarySession.getDefaultRemoteConnection() remoteConnection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { Log.d(TAG, "Payload received: ${String(payload)}") } } ) } } private inner class HelloWorldSecondaryShareSessionStateCallback : SecondarySessionStateCallback { override fun onSecondarySessionCleanup(sessionId: SessionId) { // Custom cleanup logic here. } }
Java
// Receiving side. @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); sessions = Sessions.create(this); ListenableFuture<SecondarySession> secondarySessionFuture = sessions.getSecondarySessionFuture( intent, new HelloWorldSecondaryShareSessionStateCallback()); Futures.addCallback( secondarySessionFuture, new FutureCallback<SecondarySession>() { @Override public void onSuccess(SecondarySession secondarySession) { SessionRemoteConnection remoteConnection = secondarySession.getDefaultRemoteConnection(); remoteConnection.registerReceiver( new SessionConnectionReceiver() { @Override public void onMessageReceived(SessionParticipant participant, byte[] payload) { Log.d(TAG, "Payload received: " + new String(payload, UTF_8)); } }); } @Override public void onFailure(Throwable t) { // Handle error. } }, mainExecutor); } private static class HelloWorldSecondaryShareSessionStateCallback implements SecondarySessionStateCallback { @Override public void onSecondarySessionCleanup(SessionId sessionId) { // Custom cleanup logic here. } }