AndroidJUnitRunner
类是一个 JUnit 测试运行程序,可让您在 Android 设备上运行插桩 JUnit 4 测试,包括使用 Espresso、UI Automator 和 Compose 测试框架的测试。
此测试运行程序负责将测试软件包和被测应用加载到设备上,运行测试并报告测试结果。
此测试运行程序支持几项常见的测试任务,包括以下各项:
编写 JUnit 测试
以下代码段展示了如何编写 JUnit 4 插桩测试以验证 ChangeTextBehavior
类中的 changeText
操作是否正常工作:
Kotlin
@RunWith(AndroidJUnit4::class) @LargeTest // Optional runner annotation class ChangeTextBehaviorTest { val stringToBeTyped = "Espresso" // ActivityTestRule accesses context through the runner @get:Rule val activityRule = ActivityTestRule(MainActivity::class.java) @Test fun changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()) onView(withId(R.id.changeTextBt)).perform(click()) // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))) } }
Java
@RunWith(AndroidJUnit4.class) @LargeTest // Optional runner annotation public class ChangeTextBehaviorTest { private static final String stringToBeTyped = "Espresso"; @Rule public ActivityTestRule<MainActivity>; activityRule = new ActivityTestRule<>;(MainActivity.class); @Test public void changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()); onView(withId(R.id.changeTextBt)).perform(click()); // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))); } }
访问应用的上下文
使用 AndroidJUnitRunner
运行测试时,您可以通过调用静态 ApplicationProvider.getApplicationContext()
方法来访问被测应用的上下文。如果您已在应用中创建了 Application
的自定义子类,则此方法会返回自定义子类的上下文。
如果您是工具实现者,则可以使用 InstrumentationRegistry
类访问低级测试 API。此类包含 Instrumentation
对象、目标应用 Context
对象、测试应用 Context
对象以及传入测试的命令行参数。
过滤测试
在 JUnit 4.x 测试中,您可以使用注解来配置测试运行。此功能可最大限度地减少在测试中添加样板代码和条件代码的需求。除 JUnit 4 支持的标准注解之外,此测试运行程序还支持 Android 专用注解,包括以下各项:
@RequiresDevice
:指定测试应只能在物理设备上运行,而不能在模拟器上运行。@SdkSuppress
:禁止在低于给定级别的 Android API 级别上运行测试。例如,如需禁止在低于 23 的所有 API 级别上运行测试,请使用注解@SDKSuppress(minSdkVersion=23)
。@SmallTest
、@MediumTest
和@LargeTest
:对运行测试应需要多长时间进行分类,从而对测试运行的频率进行分类。您可以使用此注解设置android.testInstrumentationRunnerArguments.size
属性,以过滤要运行的测试:
-Pandroid.testInstrumentationRunnerArguments.size=small
将测试分片
如果您需要并行执行测试,并将其分布在多个服务器上以加快运行速度,则可以将测试拆分为组或分片。此测试运行程序支持将单个测试套件拆成多个分片,以便您可以将属于同一分片的测试作为一组轻松地一起运行。每个分片都由一个索引编号进行标识。运行测试时,使用 -e numShards
选项指定要创建的独立分片数量,并使用 -e shardIndex
选项指定要运行哪个分片。
例如,如需将测试套件拆分为 10 个分片,并且仅运行分组在第二个分片中的测试,请使用以下 adb 命令:
adb shell am instrument -w -e numShards 10 -e shardIndex 2
使用 Android Test Orchestrator
借助 Android Test Orchestrator,您可以在应用自己的 Instrumentation
调用中运行应用的各项测试。使用 AndroidJUnitRunner 1.0 或更高版本时,您可以使用 Android Test Orchestrator。
Android Test Orchestrator 可为您的测试环境提供以下优势:
- 最小共享状态:每个测试都在自己的
Instrumentation
实例中运行。因此,如果您的测试共享应用状态,则每次测试后都会从设备的 CPU 或内存中移除该共享状态的一大部分。如需在每次测试后从设备的 CPU 和内存中移除全部共享状态,请使用clearPackageData
标志。如需查看示例,请参阅从 Gradle 启用部分。 - 崩溃被隔离:即使某个测试崩溃,也只会使自己的
Instrumentation
实例下线。这意味着,套件中的其他测试仍会运行,并提供完整的测试结果。
由于 Android Test Orchestrator 会在每次测试后重启应用,因此这种隔离可能会增加测试执行时间。
Android Studio 和 Firebase 测试实验室都预安装了 Android Test Orchestrator,但您需要在 Android Studio 中启用该功能。
从 Gradle 启用
如需使用 Gradle 命令行工具启用 Android Test Orchestrator,请完成以下步骤:
- 第 1 步:修改 Gradle 文件。将以下语句添加到项目的
build.gradle
文件中:
android {
defaultConfig {
...
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
// The following argument makes the Android Test Orchestrator run its
// "pm clear" command after each test invocation. This command ensures
// that the app's state is completely cleared between tests.
testInstrumentationRunnerArguments clearPackageData: 'true'
}
testOptions {
execution 'ANDROIDX_TEST_ORCHESTRATOR'
}
}
dependencies {
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestUtil 'androidx.test:orchestrator:1.1.0'
}
- 第 2 步:通过执行以下命令运行 Android Test Orchestrator:
./gradlew connectedCheck
从 Android Studio 启用
如需在 Android Studio 中启用 Android Test Orchestrator,请将从 Gradle 启用部分中所示的语句添加到应用的 build.gradle
文件中。
从命令行启用
如需在命令行上使用 Android Test Orchestrator,请在终端窗口中运行以下命令:
DEVICE_API_LEVEL=$(adb shell getprop ro.build.version.sdk)
FORCE_QUERYABLE_OPTION=""
if [[ $DEVICE_API_LEVEL -ge 30 ]]; then
FORCE_QUERYABLE_OPTION="--force-queryable"
fi
# uninstall old versions
adb uninstall androidx.test.services
adb uninstall androidx.test.orchestrator
# Install the test orchestrator.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/orchestrator/1.4.2/orchestrator-1.4.2.apk
# Install test services.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/services/test-services/1.4.2/test-services-1.4.2.apk
# Replace "com.example.test" with the name of the package containing your tests.
# Add "-e clearPackageData true" to clear your app's data in between runs.
adb shell 'CLASSPATH=$(pm path androidx.test.services) app_process / \
androidx.test.services.shellexecutor.ShellMain am instrument -w -e \
targetInstrumentation com.example.test/androidx.test.runner.AndroidJUnitRunner \
androidx.test.orchestrator/.AndroidTestOrchestrator'
如命令语法所示,您先安装 Android Test Orchestrator,然后直接使用它。
adb shell pm list instrumentation
使用不同的工具链
如果您使用其他工具链测试应用,仍可使用 Android Test Orchestrator,只需完成以下步骤即可:
架构
Orchestrator 服务 APK 存储在与测试 APK 和被测应用的 APK 分离的进程中:
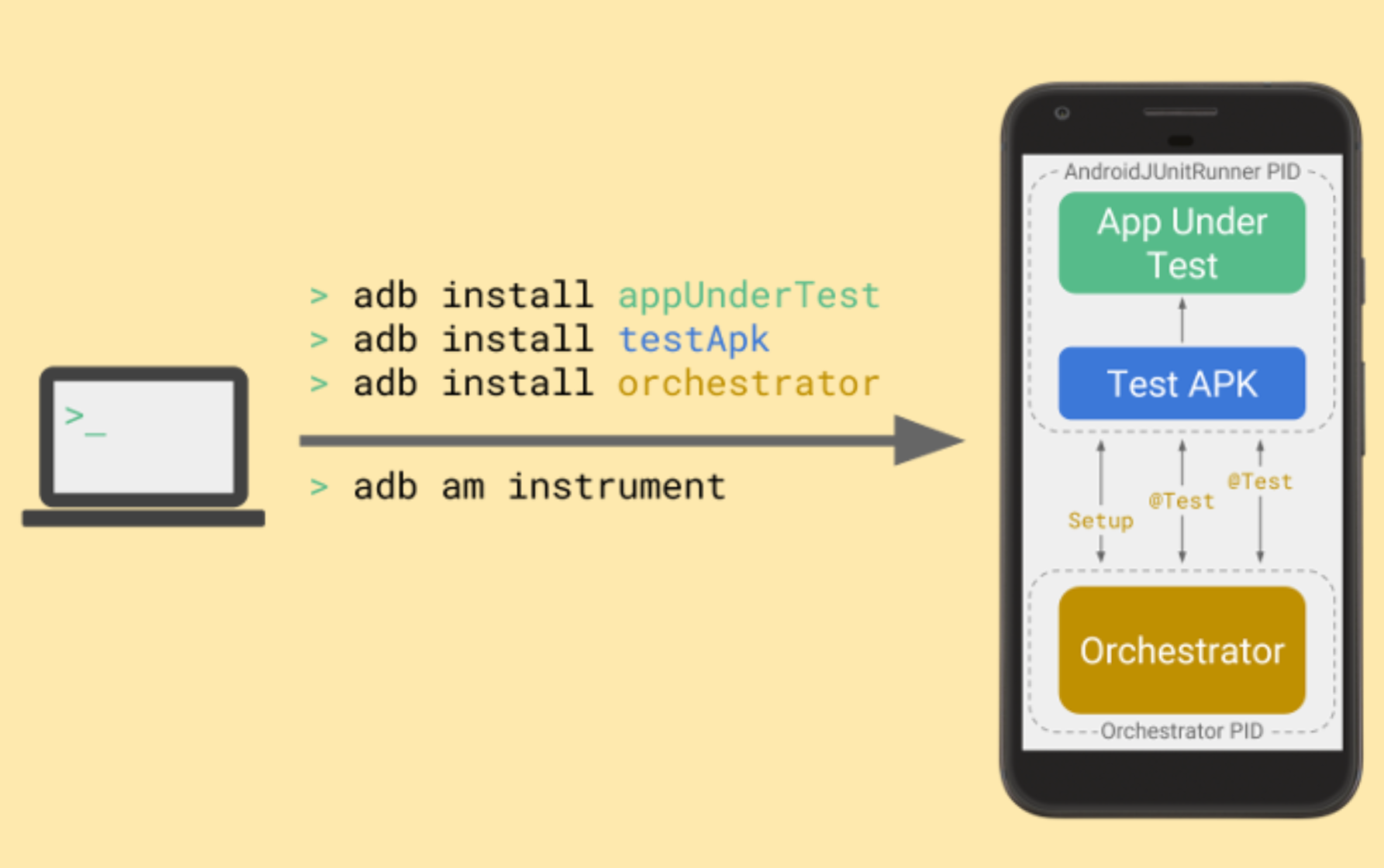
Android Test Orchestrator 会在测试套件运行开始时收集 JUnit 测试,但随后会在每个测试自己的 Instrumentation
实例中单独执行这些测试。
更多信息
如需详细了解如何使用 AndroidJUnitRunner,请参阅 API 参考文档。
其他资源
如需详细了解如何使用 AndroidJUnitRunner
,请参阅以下资源。
示例
- AndroidJunitRunnerSample:展示测试注解、参数化测试和测试套件的创建。