AndroidJUnitRunner
類別是一個 JUnit 測試執行器,
可讓您在 Android 裝置上執行 JUnit 4 檢測設備測試。
包括使用 Espresso、UI Automator 和 Compose 的應用程式
測試架構
測試執行器會負責將測試套件和測試中的應用程式載入 測試裝置、執行測試,以及回報測試結果。
這個測試執行器支援多種常見的測試工作,包括:
編寫 JUnit 測試
下列程式碼片段說明如何編寫檢測的 JUnit 4
測試以驗證 ChangeTextBehavior
中的 changeText
作業
類別正常運作:
Kotlin
@RunWith(AndroidJUnit4::class) // Only needed when mixing JUnit 3 and 4 tests @LargeTest // Optional runner annotation class ChangeTextBehaviorTest { val stringToBeTyped = "Espresso" // ActivityTestRule accesses context through the runner @get:Rule val activityRule = ActivityTestRule(MainActivity::class.java) @Test fun changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()) onView(withId(R.id.changeTextBt)).perform(click()) // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))) } }
Java
@RunWith(AndroidJUnit4.class) // Only needed when mixing JUnit 3 and 4 tests @LargeTest // Optional runner annotation public class ChangeTextBehaviorTest { private static final String stringToBeTyped = "Espresso"; @Rule public ActivityTestRule<MainActivity>; activityRule = new ActivityTestRule<>;(MainActivity.class); @Test public void changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()); onView(withId(R.id.changeTextBt)).perform(click()); // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))); } }
存取應用程式的背景資訊
使用 AndroidJUnitRunner
執行測試時,您可以存取
方法是呼叫
ApplicationProvider.getApplicationContext()
方法。如果您以自訂方式
應用程式中 Application
的子類別,這個方法會傳回自訂欄位
子類別的結構定義
如果您是工具實作,可以使用
InstrumentationRegistry
類別。這個類別包含
Instrumentation
物件,目標應用程式 Context
物件、測試
應用程式 Context
物件,以及傳遞至測試的指令列引數。
篩選測試
在 JUnit 4.x 測試中,您可以使用註解設定測試執行作業。這個 這項功能可讓您省去在 測試。除了 JUnit 4 支援的標準註解外, 執行器也支援 Android 專用的註解,包括 包括:
@RequiresDevice
:指定測試只會在實體電腦上執行 而不是模擬器上@SdkSuppress
:在較低的 Android API 上執行測試 等級 而非指定等級例如,如要在所有 API 級別中隱藏測試,就用這種方式 而不是執行 23 註解,請使用註解@SDKSuppress(minSdkVersion=23)
。@SmallTest
、@MediumTest
和@LargeTest
:分類測試時間長度 以及執行測試的頻率。個人中心 使用這個註解,即可篩選要執行的測試,並將android.testInstrumentationRunnerArguments.size
屬性:
-Pandroid.testInstrumentationRunnerArguments.size=small
資料分割測試
如果您需要平行執行測試,與
為了加快執行速度,您可以將這些伺服器分成多個群組
資料分割。測試執行工具支援將單一測試套件分割成多個
資料分割,因此您可以輕鬆將屬於同一個資料分割的測試
群組。每個資料分割都是由索引編號識別。執行測試時,請使用
-e numShards
選項,用於指定要建立的獨立資料分割數量,以及
-e shardIndex
選項,用於指定要執行的資料分割。
例如,將測試套件分割成 10 個資料分割,然後只執行測試 使用下列 adb 指令,組成第二個資料分割:
adb shell am instrument -w -e numShards 10 -e shardIndex 2
使用 Android Test Orchestrator
Android Test Orchestrator 可讓您在個別應用程式測試中
自己的 Instrumentation
叫用。使用 AndroidJUnitRunner 1.0 版本時
以上版本,即可存取 Android Test Orchestrator。
Android Test Orchestrator 提供下列測試好處 環境:
- 最小共用狀態:每項測試會在各自的
Instrumentation
中執行 執行個體。因此,如果測試共用應用程式狀態,大部分的共用狀態 且每次測試後就會從裝置的 CPU 或記憶體中移除。 分別從裝置的 CPU 和記憶體中移除「所有」共用狀態。 測試,請使用clearPackageData
旗標。請參閱「從 Gradle 啟用」 一節。 - 當機隔離:即使有一次測試當機,也只會終止當機
自己的
Instrumentation
例項。也就是說 套件仍會運作,並提供完整的測試結果。
這種隔離機制會導致測試執行時間可能增加, 每次測試後,Android Test Orchestrator 都會重新啟動應用程式。
Android Studio 和 Firebase Test Lab 皆有 Android Test Orchestrator 預先安裝,但您需要在 Android 裝置上啟用這項功能 Studio。
透過 Gradle 啟用
如要使用 Gradle 指令列工具啟用 Android Test Orchestrator,請前往 步驟如下:
- 步驟 1:修改 Gradle 檔案。將以下陳述式加入
專案的
build.gradle
檔案:
android {
defaultConfig {
...
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
// The following argument makes the Android Test Orchestrator run its
// "pm clear" command after each test invocation. This command ensures
// that the app's state is completely cleared between tests.
testInstrumentationRunnerArguments clearPackageData: 'true'
}
testOptions {
execution 'ANDROIDX_TEST_ORCHESTRATOR'
}
}
dependencies {
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestUtil 'androidx.test:orchestrator:1.1.0'
}
- 步驟 2:執行下列指令,執行 Android Test Orchestrator:
./gradlew connectedCheck
從 Android Studio 啟用
如要在 Android Studio 中啟用 Android Test Orchestrator,請新增顯示的陳述式
在「Enable from Gradle」中前往應用程式的 build.gradle
檔案。
透過指令列啟用
如要透過指令列使用 Android Test Orchestrator,請執行下列指令 在終端機視窗中:
DEVICE_API_LEVEL=$(adb shell getprop ro.build.version.sdk)
FORCE_QUERYABLE_OPTION=""
if [[ $DEVICE_API_LEVEL -ge 30 ]]; then
FORCE_QUERYABLE_OPTION="--force-queryable"
fi
# uninstall old versions
adb uninstall androidx.test.services
adb uninstall androidx.test.orchestrator
# Install the test orchestrator.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/orchestrator/1.4.2/orchestrator-1.4.2.apk
# Install test services.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/services/test-services/1.4.2/test-services-1.4.2.apk
# Replace "com.example.test" with the name of the package containing your tests.
# Add "-e clearPackageData true" to clear your app's data in between runs.
adb shell 'CLASSPATH=$(pm path androidx.test.services) app_process / \
androidx.test.services.shellexecutor.ShellMain am instrument -w -e \
targetInstrumentation com.example.test/androidx.test.runner.AndroidJUnitRunner \
androidx.test.orchestrator/.AndroidTestOrchestrator'
如指令語法所示,您需要安裝 Android Test Orchestrator,然後
adb shell pm list instrumentation
使用不同的工具鍊
如果您使用其他工具鍊測試應用程式,仍可以使用 Android 如要測試 Orchestrator,請完成下列步驟:
建築
Orchestrator 服務 APK 的儲存程序是獨立於 測試 APK 和受測試應用程式的 APK:
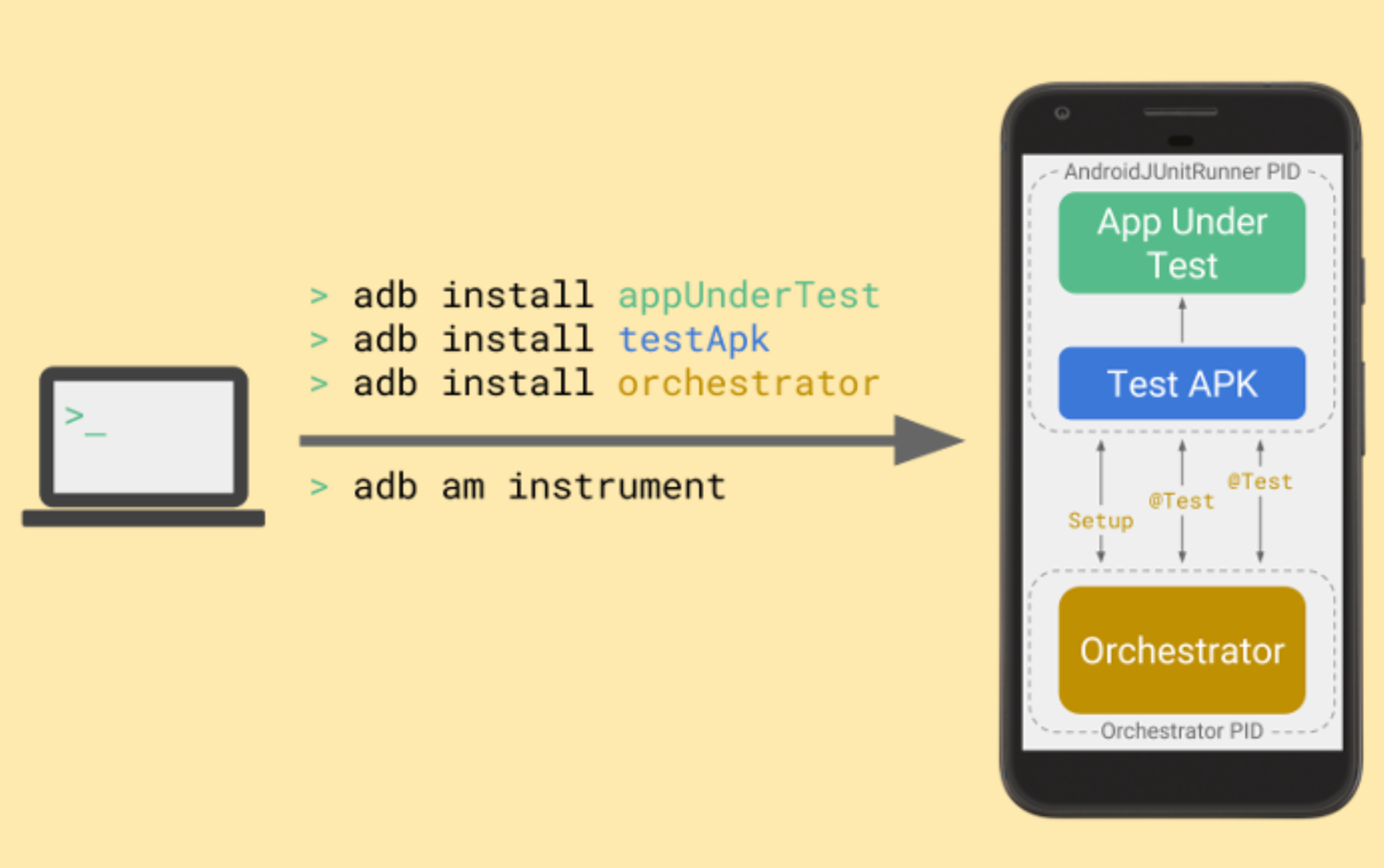
Android Test Orchestrator 會在測試開始時收集 JUnit 測試
然後,它會在各自的執行個體中個別執行每項測試
Instrumentation
。
更多資訊
如要進一步瞭解如何使用 AndroidJUnitRunner,請參閱 API 參考資料。
其他資源
如要進一步瞭解如何使用 AndroidJUnitRunner
,請參閱下列資源
再複習一下,機構節點
是所有 Google Cloud Platform 資源的根節點
範例
- AndroidJunitRunnerSample:展示測試註解 參數化測試及測試套件建立作業。