Mit Wear OS by Google haben Sie mit einer Smartwatch mehrere Möglichkeiten, Daten zu senden und zu synchronisieren. Wir empfehlen, die Daten direkt über das Netzwerk zu senden und zu synchronisieren, da die Anwendung so als eigenständige Anwendung betrachtet werden kann.
Daten direkt über das Netzwerk senden und synchronisieren
Erstellen Sie Wear OS-Apps für die direkte Kommunikation mit dem Netzwerk. Du kannst die gleichen APIs verwenden, die du auch für die mobile Entwicklung nutzt. Beachte dabei aber einige Wear-OS-spezifische Unterschiede.
Daten mit der Wearable Data Layer API senden und synchronisieren
Die Wearable Data Layer API, die Teil der Google Play-Dienste ist, bietet einen optionalen Kommunikationskanal für Apps.
Diese API ist nur auf Wear OS-Smartwatches und gekoppelten Android-Geräten verfügbar. Bei Wear OS-Smartwatches, die mit iOS-Smartphones gekoppelt sind, können Apps andere cloudbasierte APIs abfragen, sofern eine Internetverbindung verfügbar ist.
Für die Wearable Data Layer API gelten die folgenden Abhängigkeiten:
- Die neueste Version der Google Play-Dienste.
- Ein Wear OS-Gerät oder einen Wear OS-Emulator.
Füge die folgende Abhängigkeit in die Datei build.gradle
deines Wear-Moduls ein:
dependencies { ... implementation 'com.google.android.gms:play-services-wearable:18.1.0' }
Wir empfehlen, dass Wearable-Apps Daten direkt von einem Netzwerk oder verbundenen Smartphone senden und synchronisieren. Wenn Sie jedoch direkt zwischen Geräten im RPC-Typ-Format kommunizieren möchten oder keine direkte Verbindung zu einem Datennetzwerk herstellen können, können Sie die Wearable Data Layer API auf folgende Arten verwenden.
- Remote-Funktionen bewerben und abfragen
- Die
CapabilityClient
enthält Informationen dazu, welche Knoten im Wear OS-Netzwerk welche benutzerdefinierten Anwendungsfunktionen unterstützen. Knoten stellen sowohl mobile als auch Wearable-Geräte dar, die mit dem Netzwerk verbunden sind. Eine Capability ist ein Feature, das eine Anwendung entweder beim Build definiert oder während der Laufzeit dynamisch konfiguriert wird. - Beispielsweise könnte in einer Android-App angegeben werden, dass sie die Fernbedienung der Videowiedergabe unterstützt. Wenn die Wearable-Version dieser App installiert ist, kann sie mithilfe von
CapabilityClient
prüfen, ob die mobile Version der App installiert ist und diese Funktion unterstützt. Ist dies der Fall, kann die Wearable-App die Schaltfläche für Wiedergabe/Pause anzeigen, um das Video auf dem anderen Gerät über eine Nachricht zu steuern. - Dies kann mit den unterstützten Wearable-App-Eintragsfunktionen auch in die entgegengesetzte Richtung funktionieren.
- Nachrichten senden
-
Der
MessageClient
kann Nachrichten senden und eignet sich für Remote-Prozeduraufrufe (RPCs), um beispielsweise den Mediaplayer eines Handhelds über das Wearable zu steuern oder über den Handheld einen Intent auf dem Wearable zu starten. Nachrichten eignen sich auch hervorragend für einseitige Anfragen oder für ein Anfrage- oder Antwort-Kommunikationsmodell. - Wenn Handheld und Wearable verbunden sind, stellt das System die Nachricht zur Zustellung in die Warteschlange und gibt einen erfolgreichen Ergebniscode zurück. Wenn die Geräte nicht verbunden sind, wird ein Fehler zurückgegeben. Ein erfolgreicher Ergebniscode bedeutet nicht, dass die Nachricht erfolgreich zugestellt wurde, da die Verbindung der Geräte nach Empfang des Ergebniscodes möglicherweise getrennt wird.
- Daten übertragen
-
Das
ChannelClient
kann Daten von einem Handheld auf ein Wearable-Gerät übertragen. MitChannelClient
haben Sie folgende Möglichkeiten:- Übertragen Sie Datendateien zwischen zwei oder mehr verbundenen Geräten, wenn das Internet nicht verfügbar ist, ohne die automatische Synchronisierung, wenn
Asset
-Objekte verwendet werden, die anDataItem
-Objekte angehängt sind.ChannelClient
spart Speicherplatz überDataClient
. Dabei wird vor der Synchronisierung mit verbundenen Geräten eine Kopie der Assets auf dem lokalen Gerät erstellt. - Senden Sie zuverlässig eine Datei, die zu groß ist, um sie mit
MessageClient
zu senden. - Gestreamte Daten wie Sprachdaten vom Mikrofon übertragen.
- Übertragen Sie Datendateien zwischen zwei oder mehr verbundenen Geräten, wenn das Internet nicht verfügbar ist, ohne die automatische Synchronisierung, wenn
- Daten synchronisieren
- Ein
DataClient
stellt eine API für Komponenten zum Lesen oder Schreiben in einemDataItem
oderAsset
zur Verfügung. - Eine
DataItem
wird auf allen Geräten in einem Wear OS-Netzwerk synchronisiert. Es ist möglich, Datenelemente festzulegen, wenn keine Verbindung zu Knoten besteht. Diese Datenelemente werden synchronisiert, wenn die Knoten online gehen. - Datenelemente sind nur für die Anwendung zugänglich, von der sie erstellt wurden, und sind nur für diese Anwendung auf anderen Knoten zugänglich. Sie sind in der Regel klein. Verwenden Sie
Assets
für die Übertragung von größeren, persistenten Datenobjekten wie Bildern. - Wear OS unterstützt mehrere Wearables, die mit einem Handheld-Gerät verbunden sind. Wenn ein Nutzer beispielsweise eine Notiz auf einem Handheld speichert, wird diese automatisch auf allen Wear OS-Geräten des Nutzers angezeigt. Zur einfacheren Synchronisierung von Daten zwischen Geräten hosten die Google-Server einen Cloud-Knoten im Gerätenetzwerk. Das System synchronisiert Daten mit direkt verbundenen Geräten, dem Cloud-Knoten, und mit Wearable-Geräten, die über WLAN mit dem Cloud-Knoten verbunden sind.
Warnung:Elemente werden auf alle verfügbaren Wear OS-Geräte übertragen, auch auf Geräte, auf denen deine App nicht installiert ist. Wenn Sie große Datenmengen synchronisieren, empfiehlt es sich zu prüfen, ob eine „Empfänger-App“ installiert und online ist, um keine Ressourcenverschwendung sowohl auf Handheld-Geräten als auch auf Wear OS-Geräten zu vermeiden.
- Auf wichtige Datenschichtereignisse warten (für Dienste)
- Wenn Sie
WearableListenerService
erweitern, können Sie wichtige Datenschichtereignisse in einem Dienst im Blick behalten. Das System verwaltet den Lebenszyklus vonWearableListenerService
und erstellt eine Bindung an den Dienst, wenn Datenelemente oder Nachrichten gesendet werden müssen. Wenn keine Arbeit erforderlich ist, hebt das System die Bindung des Dienstes auf. - Auf wichtige Datenschichtereignisse warten (für Aktivitäten im Vordergrund)
-
Wenn Sie
OnDataChangedListener
in eine Aktivität implementieren, können Sie auf wichtige Datenschichtereignisse warten, wenn eine Aktivität im Vordergrund ausgeführt wird. Wenn Sie diese anstelle vonWearableListenerService
verwenden, können Sie nur dann auf Änderungen warten, wenn der Nutzer Ihre Anwendung aktiv verwendet.
Warnung:Da diese APIs für die Kommunikation zwischen Handhelds und Wearables entwickelt wurden, können Sie nur diese APIs verwenden, um die Kommunikation zwischen diesen Geräten einzurichten. Versuchen Sie beispielsweise nicht, Low-Level-Sockets zu öffnen, um einen Kommunikationskanal zu erstellen.
Kundenvergleich
Die folgende Tabelle zeigt die verschiedenen Anforderungen und Anwendungsfälle für jeden Kunden.
Datenclient | Nachrichtenclient | Kanalkunde | |
Datengröße größer als 100 KB | Ja | Nein | Ja |
Kann Nachrichten an derzeit nicht verbundene Knoten senden | Ja | Nein | Nein |
Kommunikationsmuster | Freigegebene netzwerkbasierte Ressource | 1:1-Nachricht weitergegeben (mit Antwort) | 1:1-Streaming |
Konnektivität
Die Datenschicht bietet zwei Kommunikationsoptionen:
- Daten direkt austauschen, wenn eine Bluetooth-Verbindung zwischen der Uhr und einem anderen Gerät besteht.
- Daten über ein verfügbares Netzwerk wie LTE oder WLAN austauschen.
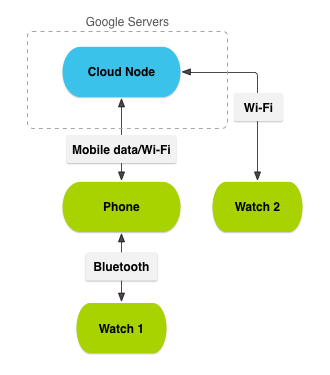
Alle Datenschicht-Clients können Daten entweder über Bluetooth oder Google Cloud Sync austauschen, je nachdem, welche Verbindungen für die Geräte verfügbar sind. Der Cloud-Synchronisierungsdienst ist der Mechanismus von Google für die Kommunikation und den Datenaustausch zwischen Wearables und Smartphones, wenn Bluetooth nicht verfügbar ist.
Sicherheit
Beide Kommunikationsoptionen, Bluetooth und Cloud Sync Service, sind mit Ende-zu-Ende-Verschlüsselung geschützt.
Für die Google Play-Dienste gelten die folgenden Einschränkungen, damit die Kommunikation zwischen Smartphone und Smartwatch sicher ist und zwischen Apps möglich ist.
- Der Paketname muss auf allen Geräten übereinstimmen.
- Die Signatur des Pakets muss auf allen Geräten übereinstimmen.
Bluetooth
Wenn Geräte über Bluetooth verbunden sind, wird diese Verbindung von Data Layer verwendet. Bei Verwendung von Bluetooth gibt es einen einzelnen verschlüsselten Kanal zwischen den Geräten, der die standardmäßige Bluetooth-Verschlüsselung nutzt, die von den Google Play-Diensten verwaltet wird.
Wolke
Wir gehen davon aus, dass Daten, die mit der Datenschicht übertragen werden, irgendwann Server von Google verwenden können. DataClient
, MessageClient
oder ChannelClient
leiten beispielsweise automatisch über Google Cloud weiter, wenn Bluetooth nicht verfügbar ist.
Alle über Google Cloud übertragenen Daten sind mit Ende-zu-Ende-Verschlüsselung geschützt.
Schlüsselgenerierung und -speicherung
Ende-zu-Ende-Schlüssel für die cloudbasierte Kommunikation werden vom Smartphone generiert und direkt mit der Smartwatch ausgetauscht, wenn die beiden Geräte über Bluetooth verbunden sind. Dies geschieht während der Geräteeinrichtung. Server von Google erhalten diese Schlüssel zu keinem Zeitpunkt.
Eine Kommunikation über Google-eigene Server kann erst stattfinden, wenn die End-to-End-Schlüsselgenerierung abgeschlossen ist. Schlüssel werden im privaten Dateispeicher der Google Play-Dienste auf allen gekoppelten Geräten gespeichert.
Gerätesicherung
Schlüssel werden nicht gesichert und verbleiben auf dem Gerät. Wenn neue Schlüssel erforderlich sind (z. B. für ein neues Smartphone), generiert das System neue Schlüssel und teilt sie mit den Geräten, die der Nutzer noch hat.