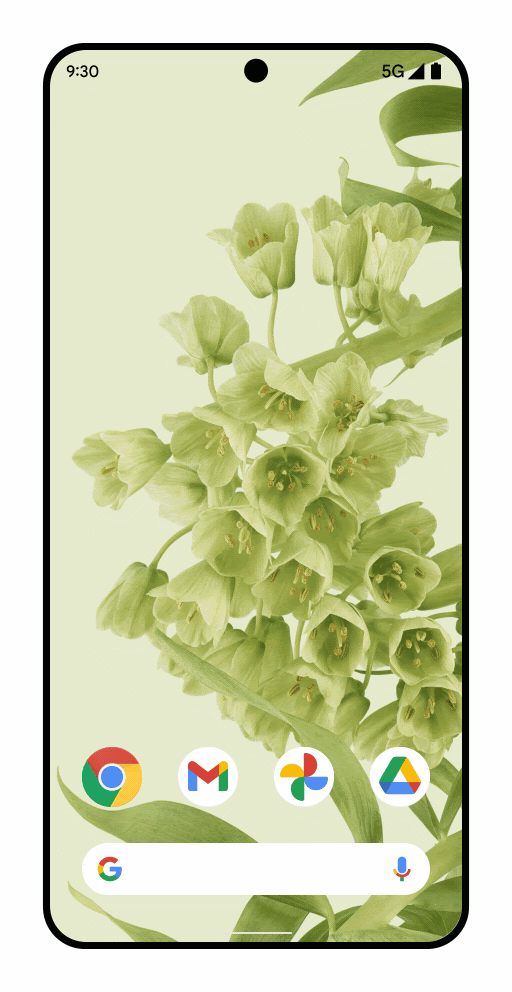
ভবিষ্যদ্বাণীমূলক পিছনে, একটি অঙ্গভঙ্গি নেভিগেশন বৈশিষ্ট্য, ব্যবহারকারীদের পূর্বরূপ দেখতে দেয় যেখানে পিছনের সোয়াইপ তাদের নিয়ে যায়।
উদাহরণস্বরূপ, একটি পিছনের অঙ্গভঙ্গি ব্যবহার করে আপনার অ্যাপের পিছনে হোম স্ক্রিনের একটি অ্যানিমেটেড প্রিভিউ দেখাতে পারে, যেমনটি চিত্র 1-এ উপস্থাপিত হয়েছে৷
অ্যান্ড্রয়েড 15 দিয়ে শুরু করে, ভবিষ্যদ্বাণীমূলক ব্যাক অ্যানিমেশনের জন্য বিকাশকারী বিকল্পটি আর উপলব্ধ নেই। সিস্টেম অ্যানিমেশন যেমন ব্যাক-টু-হোম, ক্রস-টাস্ক, এবং ক্রস-অ্যাক্টিভিটি এখন এমন অ্যাপগুলির জন্য উপস্থিত হয় যেগুলি সম্পূর্ণরূপে বা কোনও কার্যকলাপ স্তরে পূর্বাভাসমূলক ব্যাক জেসচারে বেছে নিয়েছে৷
আপনি এই ব্যাক-টু-হোম অ্যানিমেশনটি পরীক্ষা করতে পারেন (যেমন এই পৃষ্ঠার নিম্নলিখিত বিভাগে বর্ণিত হয়েছে)।
ভবিষ্যদ্বাণীমূলক ব্যাক জেসচার সমর্থন করার জন্য আপনার অ্যাপ আপডেট করতে হবে, ব্যাকওয়ার্ড সামঞ্জস্যপূর্ণ OnBackPressedCallback
AppCompat 1.6.0-alpha05 (AndroidX) বা উচ্চতর API ব্যবহার করে, অথবা নতুন OnBackInvokedCallback
প্ল্যাটফর্ম API ব্যবহার করে। বেশিরভাগ অ্যাপই ব্যাকওয়ার্ড সামঞ্জস্যপূর্ণ AndroidX API ব্যবহার করে।
এই আপডেটটি ব্যাক নেভিগেশনকে সঠিকভাবে ইন্টারসেপ্ট করার জন্য একটি মাইগ্রেশন পাথ প্রদান করে, যার মধ্যে KeyEvent.KEYCODE_BACK
থেকে ব্যাক ইন্টারসেপশন প্রতিস্থাপন করা হয় এবং নতুন সিস্টেম ব্যাক এপিআই-এর সাথে Activity
এবং Dialog
মতো onBackPressed
পদ্ধতি সহ যেকোনো ক্লাস প্রতিস্থাপন করা হয়।
কোডল্যাব এবং Google I/O ভিডিও
এই পৃষ্ঠায় এই ডকুমেন্টেশন ব্যবহার করার পাশাপাশি, আমাদের কোডল্যাব ব্যবহার করে দেখুন । এটি AndroidX কার্যকলাপ API ব্যবহার করে ভবিষ্যদ্বাণীমূলক ব্যাক জেসচার পরিচালনা করে একটি WebView-এর একটি সাধারণ ব্যবহার-কেস বাস্তবায়ন প্রদান করে।
এছাড়াও আপনি আমাদের Google I/O ভিডিও দেখতে পারেন, যা AndroidX এবং প্ল্যাটফর্ম APIs বাস্তবায়নের অতিরিক্ত উদাহরণ কভার করে।
ডিফল্ট ব্যাক নেভিগেশন ব্যবহার করে এমন একটি অ্যাপ আপডেট করুন
এই বৈশিষ্ট্যটিকে সমর্থন করার জন্য আপনার অ্যাপ আপডেট করা সহজ যদি আপনার অ্যাপটি কোনো কাস্টম ব্যাক আচরণ বাস্তবায়ন না করে (অন্য কথায়, এটি সিস্টেমের কাছে হ্যান্ডলিং ছেড়ে দেয়)। এই নির্দেশিকায় বর্ণিত এই বৈশিষ্ট্যটিতে অপ্ট-ইন করুন ৷
যদি আপনার অ্যাপটি ফ্র্যাগমেন্টস বা নেভিগেশন কম্পোনেন্ট ব্যবহার করে, তাহলে AndroidX Activity 1.6.0-alpha05 বা উচ্চতর সংস্করণেও আপগ্রেড করুন।
কাস্টম ব্যাক নেভিগেশন ব্যবহার করে এমন একটি অ্যাপ আপডেট করুন
যদি আপনার অ্যাপটি কাস্টম ব্যাক আচরণ প্রয়োগ করে, তবে এটি AndroidX ব্যবহার করে কিনা এবং এটি কীভাবে ব্যাক নেভিগেশন পরিচালনা করে তার উপর নির্ভর করে বিভিন্ন মাইগ্রেশন পাথ রয়েছে।
আপনার অ্যাপ AndroidX ব্যবহার করে | কীভাবে আপনার অ্যাপ ব্যাক নেভিগেশন পরিচালনা করে | প্রস্তাবিত মাইগ্রেশন পথ (এই পৃষ্ঠার লিঙ্ক) |
হ্যাঁ | AndroidX APIs | একটি বিদ্যমান AndroidX ব্যাক বাস্তবায়ন স্থানান্তর করুন |
অসমর্থিত প্ল্যাটফর্ম API | AndroidX API-এ অসমর্থিত ব্যাক নেভিগেশন API সমন্বিত একটি AndroidX অ্যাপ স্থানান্তর করুন | |
না | অসমর্থিত প্ল্যাটফর্ম API, স্থানান্তর করতে সক্ষম | প্ল্যাটফর্ম API-এ অসমর্থিত ব্যাক নেভিগেশন API ব্যবহার করে এমন একটি অ্যাপ স্থানান্তর করুন |
অসমর্থিত প্ল্যাটফর্ম API, কিন্তু স্থানান্তর করতে অক্ষম | এটি একটি প্রয়োজনীয় বৈশিষ্ট্য না হওয়া পর্যন্ত অপ্ট-ইন স্থগিত করুন |
একটি AndroidX ব্যাক নেভিগেশন বাস্তবায়ন স্থানান্তর করুন
এই ব্যবহারের ক্ষেত্রে সবচেয়ে সাধারণ (এবং সবচেয়ে প্রস্তাবিত)। এটি নতুন বা বিদ্যমান অ্যাপগুলির ক্ষেত্রে প্রযোজ্য যেগুলি OnBackPressedDispatcher
এর সাথে কাস্টম অঙ্গভঙ্গি নেভিগেশন হ্যান্ডলিং প্রয়োগ করে, যেমনটি কাস্টম ব্যাক নেভিগেশন প্রদানে বর্ণিত হয়েছে৷
যদি আপনার অ্যাপ এই বিভাগে ফিট করে, তাহলে ভবিষ্যদ্বাণীমূলক ব্যাক জেসচারের জন্য সমর্থন যোগ করতে এই পদক্ষেপগুলি অনুসরণ করুন:
যে APIগুলি ইতিমধ্যেই
OnBackPressedDispatcher
API ব্যবহার করছে (যেমন ফ্র্যাগমেন্টস এবং নেভিগেশন কম্পোনেন্ট) ভবিষ্যদ্বাণীমূলক ব্যাক জেসচারের সাথে নির্বিঘ্নে কাজ করে তা নিশ্চিত করতে, AndroidX Activity 1.6.0-alpha05- এ আপগ্রেড করুন।// In your build.gradle file: dependencies { // Add this in addition to your other dependencies implementation "androidx.activity:activity:1.6.0-alpha05"
এই পৃষ্ঠায় বর্ণিত ভবিষ্যদ্বাণীমূলক ব্যাক জেসচারে অপ্ট-ইন করুন ৷
AndroidX API-এ অসমর্থিত ব্যাক নেভিগেশন API সমন্বিত একটি AndroidX অ্যাপ স্থানান্তর করুন
যদি আপনার অ্যাপটি AndroidX লাইব্রেরি ব্যবহার করে কিন্তু অসমর্থিত ব্যাক নেভিগেশন APIগুলিকে প্রয়োগ করে বা রেফারেন্স করে, তাহলে নতুন আচরণ সমর্থন করার জন্য আপনাকে AndroidX API ব্যবহার করে মাইগ্রেট করতে হবে।
অসমর্থিত APIগুলিকে AndroidX APIগুলিতে স্থানান্তর করতে:
OnBackPressedCallback
এর একটি বাস্তবায়নের সাথে AndroidX-এরOnBackPressedDispatcher
এ আপনার সিস্টেম ব্যাক হ্যান্ডলিং লজিক মাইগ্রেট করুন। বিস্তারিত নির্দেশনার জন্য, কাস্টম ব্যাক নেভিগেশন প্রদান দেখুন।পিছনের অঙ্গভঙ্গি বাধা দেওয়া বন্ধ করার জন্য প্রস্তুত হলে
OnBackPressedCallback
অক্ষম করুন৷OnBackPressed
বাKeyEvent.KEYCODE_BACK
এর মাধ্যমে ব্যাক ইভেন্টে বাধা দেওয়া বন্ধ করুন।AndroidX Activity 1.6.0-alpha05- এ আপগ্রেড করা নিশ্চিত করুন।
// In your build.gradle file: dependencies { // Add this in addition to your other dependencies implementation "androidx.activity:activity:1.6.0-alpha05"
আপনি যখন সফলভাবে আপনার অ্যাপ স্থানান্তরিত করেছেন, ব্যাক-টু-হোম সিস্টেম অ্যানিমেশন দেখতে ভবিষ্যদ্বাণীমূলক ব্যাক জেসচারে অপ্ট-ইন করুন (এই পৃষ্ঠায় বর্ণিত)।
প্ল্যাটফর্ম API-এ অসমর্থিত ব্যাক নেভিগেশন API ব্যবহার করে এমন একটি অ্যাপ স্থানান্তর করুন
যদি আপনার অ্যাপ AndroidX লাইব্রেরি ব্যবহার করতে না পারে এবং পরিবর্তে অসমর্থিত API ব্যবহার করে কাস্টম ব্যাক নেভিগেশন প্রয়োগ করে বা রেফারেন্স করে, তাহলে আপনাকে অবশ্যই OnBackInvokedCallback
প্ল্যাটফর্ম API-এ স্থানান্তর করতে হবে।
প্ল্যাটফর্ম API-এ অসমর্থিত APIগুলি স্থানান্তর করতে নিম্নলিখিত পদক্ষেপগুলি সম্পূর্ণ করুন:
অ্যান্ড্রয়েড 13 বা উচ্চতর সংস্করণে চলমান ডিভাইসগুলিতে নতুন
OnBackInvokedCallback
API ব্যবহার করুন এবং Android 12 বা তার পরবর্তী সংস্করণে চলমান ডিভাইসগুলিতে অসমর্থিত APIগুলির উপর নির্ভর করুন৷OnBackInvokedCallback
এonBackInvokedDispatcher
এর সাথে আপনার কাস্টম ব্যাক লজিক নিবন্ধন করুন। এটি বর্তমান কার্যকলাপকে সমাপ্ত হতে বাধা দেয় এবং ব্যবহারকারী সিস্টেম ব্যাক নেভিগেশন সম্পূর্ণ করলে আপনার কলব্যাক ব্যাক অ্যাকশনে প্রতিক্রিয়া জানানোর সুযোগ পায়।পিছনের অঙ্গভঙ্গি বাধা দেওয়া বন্ধ করার জন্য প্রস্তুত হলে
OnBackInvokedCallback
এর নিবন্ধনমুক্ত করুন৷ অন্যথায়, ব্যবহারকারীরা একটি সিস্টেম ব্যাক নেভিগেশন ব্যবহার করার সময় অবাঞ্ছিত আচরণ দেখতে পারে—উদাহরণস্বরূপ, ভিউগুলির মধ্যে "আটকে যাওয়া" এবং তাদের আপনার অ্যাপটি ছেড়ে দিতে বাধ্য করা।onBackPressed
থেকে যুক্তিকে কীভাবে স্থানান্তর করা যায় তার একটি উদাহরণ এখানে রয়েছে:কোটলিন
@Override fun onCreate() { if (BuildCompat.isAtLeastT()) { onBackInvokedDispatcher.registerOnBackInvokedCallback( OnBackInvokedDispatcher.PRIORITY_DEFAULT ) { /** * onBackPressed logic goes here. For instance: * Prevents closing the app to go home screen when in the * middle of entering data to a form * or from accidentally leaving a fragment with a WebView in it * * Unregistering the callback to stop intercepting the back gesture: * When the user transitions to the topmost screen (activity, fragment) * in the BackStack, unregister the callback by using * OnBackInvokeDispatcher.unregisterOnBackInvokedCallback * (https://developer.android.com/reference/kotlin/android/window/OnBackInvokedDispatcher#unregisteronbackinvokedcallback) */ } } }
জাভা
@Override void onCreate() { if (BuildCompat.isAtLeastT()) { getOnBackInvokedDispatcher().registerOnBackInvokedCallback( OnBackInvokedDispatcher.PRIORITY_DEFAULT, () -> { /** * onBackPressed logic goes here - For instance: * Prevents closing the app to go home screen when in the * middle of entering data to a form * or from accidentally leaving a fragment with a WebView in it * * Unregistering the callback to stop intercepting the back gesture: * When the user transitions to the topmost screen (activity, fragment) * in the BackStack, unregister the callback by using * OnBackInvokeDispatcher.unregisterOnBackInvokedCallback * (https://developer.android.com/reference/kotlin/android/view/OnBackInvokedDispatcher#unregisteronbackinvokedcallback) */ } ); } }
Android 13 এবং তার পরের জন্য
OnBackPressed
বাKeyEvent.KEYCODE_BACK
এর মাধ্যমে ব্যাক ইভেন্টগুলিকে বাধা দেওয়া বন্ধ করুন৷আপনি যখন আপনার অ্যাপ সফলভাবে স্থানান্তরিত করেছেন, তখন পূর্বাভাসমূলক ব্যাক জেসচারে অপ্ট-ইন করুন (যেমন এই পৃষ্ঠায় বর্ণনা করা হয়েছে) যাতে
OnBackInvokedCallback
কার্যকর হয়৷
আপনি PRIORITY_DEFAULT
বা PRIORITY_OVERLAY
এর সাথে একটি OnBackInvokedCallback
নিবন্ধন করতে পারেন, যা অনুরূপ AndroidX OnBackPressedCallback
এ উপলব্ধ নয়৷ PRIORITY_OVERLAY
এর সাথে একটি কলব্যাক নিবন্ধন করা কিছু ক্ষেত্রে সহায়ক৷
এটি প্রযোজ্য হয় যখন আপনি onKeyPreIme()
থেকে স্থানান্তরিত হন এবং আপনার কলব্যাকে একটি খোলা IME এর পরিবর্তে পিছনের অঙ্গভঙ্গি গ্রহণ করতে হবে৷ IME গুলি খোলার সময় PRIORITY_DEFAULT
দিয়ে কলব্যাক নিবন্ধন করে৷ OnBackInvokedDispatcher
ওপেন IME-এর পরিবর্তে আপনার কলব্যাকে পিছনের অঙ্গভঙ্গি প্রেরণ করে তা নিশ্চিত করতে PRIORITY_OVERLAY
এর সাথে আপনার কলব্যাক নিবন্ধন করুন৷
ভবিষ্যদ্বাণীমূলক ব্যাক জেসচারে অপ্ট-ইন করুন
একবার আপনি আপনার ক্ষেত্রের উপর ভিত্তি করে কীভাবে আপনার অ্যাপ আপডেট করবেন তা নির্ধারণ করলে, পূর্বাভাসমূলক ব্যাক জেসচার সমর্থন করার জন্য অপ্ট-ইন করুন।
অপ্ট-ইন করতে, AndroidManifest.xml
এ, <application>
ট্যাগে, android:enableOnBackInvokedCallback
পতাকাটিকে true
সেট করুন।
<application
...
android:enableOnBackInvokedCallback="true"
... >
...
</application>
যদি আপনি একটি মান প্রদান না করেন, এটি ডিফল্ট হিসাবে false
এবং নিম্নলিখিতগুলি করে:
- ভবিষ্যদ্বাণীমূলক ব্যাক জেসচার সিস্টেম অ্যানিমেশন অক্ষম করে৷
-
OnBackInvokedCallback
উপেক্ষা করে, কিন্তুOnBackPressedCallback
কলগুলি কাজ করতে থাকে৷
একটি কার্যকলাপ স্তরে অপ্ট-ইন করুন
Android 14 দিয়ে শুরু করে, android:enableOnBackInvokedCallback
পতাকা আপনাকে কার্যকলাপ স্তরে ভবিষ্যদ্বাণীমূলক সিস্টেম অ্যানিমেশন বেছে নিতে দেয়। এই আচরণটি বৃহৎ মাল্টি-অ্যাক্টিভিটি অ্যাপগুলিকে ভবিষ্যদ্বাণীমূলক ব্যাক জেসচারে স্থানান্তর করা আরও পরিচালনাযোগ্য করে তোলে। Android 15 এর সাথে, ভবিষ্যদ্বাণীমূলক ব্যাক আর বিকাশকারী বিকল্পের পিছনে নেই। অ্যাপ্লিকেশানগুলি সম্পূর্ণরূপে বা কার্যকলাপ স্তরে ভবিষ্যদ্বাণী করতে বেছে নিতে পারে৷
নিম্নলিখিত কোডটি MainActivity
থেকে ব্যাক-টু-হোম সিস্টেম অ্যানিমেশন সক্ষম করতে enableOnBackInvokedCallback
ব্যবহার করার একটি উদাহরণ দেখায়:
<manifest ...>
<application . . .
android:enableOnBackInvokedCallback="false">
<activity
android:name=".MainActivity"
android:enableOnBackInvokedCallback="true"
...
</activity>
<activity
android:name=".SecondActivity"
android:enableOnBackInvokedCallback="false"
...
</activity>
</application>
</manifest>
পূর্ববর্তী উদাহরণে, ".SecondActivity"
এর জন্য android:enableOnBackInvokedCallback=true
সেট করা ক্রস-অ্যাক্টিভিটি সিস্টেম অ্যানিমেশনকে সক্ষম করে।
android:enableOnBackInvokedCallback
পতাকা ব্যবহার করার সময় নিম্নলিখিত বিবেচনাগুলি মনে রাখবেন:
-
android:enableOnBackInvokedCallback=false
সেট করা হল অ্যাক্টিভিটি লেভেলে বা অ্যাপ লেভেলে ভবিষ্যদ্বাণীমূলক ব্যাক অ্যানিমেশন বন্ধ করে, যেখানে আপনি ট্যাগ সেট করেছেন তার উপর নির্ভর করে, এবং সিস্টেমকেOnBackInvokedCallback
প্ল্যাটফর্ম API-এ কলগুলি উপেক্ষা করার নির্দেশ দেয়৷ যাইহোক,OnBackPressedCallback
এ কলগুলি চলতে থাকে কারণOnBackPressedCallback
ব্যাকওয়ার্ড সামঞ্জস্যপূর্ণ এবংonBackPressed
API-কে কল করে, যা Android 13-এর আগে অসমর্থিত। - অ্যাপ স্তরে
enableOnBackInvokedCallback
পতাকা সেট করা অ্যাপের সমস্ত কার্যকলাপের জন্য ডিফল্ট মান স্থাপন করে। পূর্ববর্তী কোড উদাহরণে দেখানো হিসাবে, কার্যকলাপ স্তরে পতাকা সেট করে আপনি প্রতি কার্যকলাপ ডিফল্ট ওভাররাইড করতে পারেন।
কলব্যাক সেরা অনুশীলন
এখানে সমর্থিত সিস্টেম ব্যাক কলব্যাক ব্যবহার করার জন্য সর্বোত্তম অনুশীলন রয়েছে; BackHandler
(কম্পোজের জন্য), OnBackPressedCallback
, বা OnBackInvokedCallback
।
UI অবস্থা নির্ধারণ করুন যা প্রতিটি কলব্যাককে সক্ষম এবং নিষ্ক্রিয় করে
UI স্টেট হল একটি সম্পত্তি যা UI বর্ণনা করে। আমরা এই উচ্চ-স্তরের পদক্ষেপগুলি অনুসরণ করার পরামর্শ দিই।
UI অবস্থা নির্ধারণ করুন যা প্রতিটি কলব্যাককে সক্ষম এবং নিষ্ক্রিয় করে।
StateFlow
বা কম্পোজ স্টেটের মতো একটি পর্যবেক্ষণযোগ্য ডেটা হোল্ডার টাইপ ব্যবহার করে সেই স্টেটটিকে সংজ্ঞায়িত করুন এবং স্টেট পরিবর্তনের সাথে সাথে কলব্যাক সক্রিয় বা অক্ষম করুন।
যদি আপনার অ্যাপ পূর্বে শর্তসাপেক্ষ বিবৃতিগুলির সাথে ব্যাক লজিক যুক্ত করে থাকে, তাহলে এটি ইঙ্গিত দিতে পারে যে আপনি পিছনের ইভেন্টটি ইতিমধ্যে ঘটে যাওয়ার পরে প্রতিক্রিয়া জানাচ্ছেন। নতুন কলব্যাকের সাথে এই প্যাটার্ন এড়িয়ে চলুন। যদি সম্ভব হয়, কলব্যাকটিকে শর্তসাপেক্ষ বিবৃতির বাইরে নিয়ে যান এবং পরিবর্তে একটি পর্যবেক্ষণযোগ্য ডেটা হোল্ডার টাইপের সাথে কলব্যাকটিকে সংযুক্ত করুন৷
UI লজিকের জন্য সিস্টেম ব্যাক কলব্যাক ব্যবহার করুন
UI যুক্তি নির্দেশ করে কিভাবে UI প্রদর্শন করতে হয়। UI লজিক চালানোর জন্য সিস্টেম ব্যাক কলব্যাক ব্যবহার করুন, যেমন একটি পপ-আপ প্রদর্শন করা বা একটি অ্যানিমেশন চালানো।
যদি আপনার অ্যাপ একটি সিস্টেম ব্যাক কলব্যাক সক্ষম করে, তাহলে ভবিষ্যদ্বাণীমূলক অ্যানিমেশন চলবে না এবং আপনাকে অবশ্যই ব্যাক ইভেন্টটি পরিচালনা করতে হবে। শুধুমাত্র নন-ইউআই লজিক চালানোর জন্য কলব্যাক তৈরি করবেন না।
উদাহরণস্বরূপ, আপনি যদি শুধুমাত্র লগ করার জন্য ইভেন্টগুলিকে ব্যাক করে থাকেন তবে পরিবর্তে কার্যকলাপ বা ফ্র্যাগমেন্ট লাইফসাইকেলের মধ্যে লগ করুন৷
- অ্যাক্টিভিটি-টু-অ্যাক্টিভিটি কেস বা ফ্র্যাগমেন্ট-টু-অ্যাক্টিভিটি ক্ষেত্রে, লগ ইন করুন যদি
onDestroy
মধ্যেisFinishing
অ্যাক্টিভিটি জীবনচক্রের মধ্যেtrue
হয়। - ফ্র্যাগমেন্ট-টু-ফ্র্যাগমেন্ট ক্ষেত্রে, লগ করুন যদি
onDestroy
মধ্যেisRemoving
ফ্র্যাগমেন্টের ভিউ লাইফসাইকেলের মধ্যে সত্য। অথবাFragmentManager.OnBackStackChangedListener
মধ্যেonBackStackChangeStarted
বাonBackStackChangeCommitted
পদ্ধতি ব্যবহার করে লগ করুন।
রচনার ক্ষেত্রে, রচনা গন্তব্যের সাথে যুক্ত একটি ViewModel
onCleared()
কলব্যাকের মধ্যে লগ করুন৷ যখন একটি রচনা গন্তব্য ব্যাক স্ট্যাকের থেকে পপ করা হয় এবং ধ্বংস হয় তখন এটি জানার জন্য এটি সর্বোত্তম সংকেত।
একক দায়িত্ব কলব্যাক তৈরি করুন
আপনি প্রেরণকারীতে একাধিক কলব্যাক যোগ করতে পারেন। কলব্যাকগুলি একটি স্ট্যাকে যোগ করা হয় যেখানে শেষ যোগ করা কলব্যাকটি পরবর্তী ব্যাক জেসচার প্রতি ব্যাক জেসচারে একটি কলব্যাক দিয়ে পরিচালনা করে।
কলব্যাকের সক্রিয় অবস্থা পরিচালনা করা সহজ যদি সেই কলব্যাকের একক দায়িত্ব থাকে। যেমন:
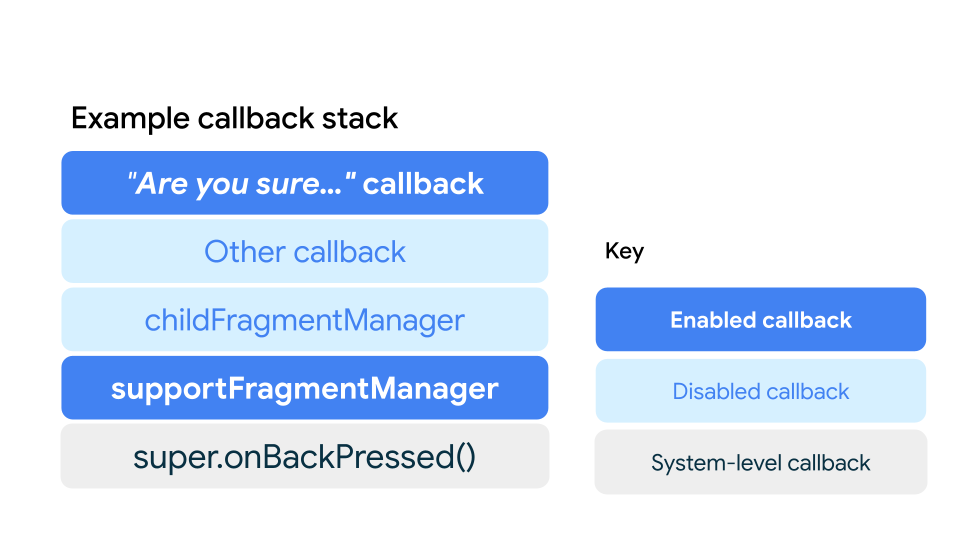
চিত্র 2 দেখায় কিভাবে আপনি স্ট্যাকে একাধিক কলব্যাক রাখতে পারেন, প্রতিটি একটি জিনিসের জন্য দায়ী। একটি কলব্যাক কেবল তখনই চলে যদি স্ট্যাকের উপরে থাকা কলব্যাকগুলি অক্ষম থাকে৷ এই উদাহরণে, "আপনি কি নিশ্চিত..." কলব্যাক সক্ষম হয় যখন ব্যবহারকারী একটি ফর্মে ডেটা প্রবেশ করে, এবং অন্যথায় অক্ষম করা হয়৷ যখন ব্যবহারকারী ফর্ম থেকে প্রস্থান করতে ফিরে সোয়াইপ করে তখন কলব্যাক একটি নিশ্চিতকরণ ডায়ালগ খোলে।
অন্যান্য কলব্যাকে একটি উপাদান উপাদান অন্তর্ভুক্ত থাকতে পারে যা ভবিষ্যদ্বাণীমূলক ব্যাক সমর্থন করে, অগ্রগতি API ব্যবহার করে একটি AndroidX রূপান্তর বা অন্য কাস্টম কলব্যাক।
উপরের কলব্যাকগুলি অক্ষম থাকলে এবং এই FragmentManager
ব্যাক স্ট্যাক খালি না থাকলে একটি childFragmentManager
কলব্যাক চলে, যেখানে childFragmentManager
একটি ফ্র্যাগমেন্টের মধ্যে সংযুক্ত থাকে। এই উদাহরণে, এই অভ্যন্তরীণ কলব্যাক অক্ষম করা হয়েছে৷
একইভাবে, supportFragmentManager
এর অভ্যন্তরীণ কলব্যাক চলে যদি উপরের কলব্যাকগুলি নিষ্ক্রিয় থাকে এবং এর স্ট্যাক খালি না থাকে। নেভিগেশনের জন্য FragmentManager
বা NavigationComponent
ব্যবহার করার সময় এই আচরণটি সামঞ্জস্যপূর্ণ, কারণ NavigationComponent
FragmentManager
উপর নির্ভর করে। এই উদাহরণে, ব্যবহারকারী যদি "আপনি কি নিশ্চিত..." কলব্যাক অক্ষম করে ফর্মে পাঠ্য না লিখলে এই কলব্যাক চলে।
অবশেষে, super.onBackPressed()
হল সিস্টেম-স্তরের কলব্যাক, যেটি আবার চলে যদি উপরের কলব্যাকগুলি নিষ্ক্রিয় থাকে। ব্যাক-টু-হোম, ক্রস-অ্যাক্টিভিটি এবং ক্রস-টাস্কের মতো সিস্টেম অ্যানিমেশনগুলিকে ট্রিগার করার জন্য, supportFragmentManager
এর ব্যাক স্ট্যাক খালি থাকতে হবে যাতে এর অভ্যন্তরীণ কলব্যাক অক্ষম করা হয়।
ভবিষ্যদ্বাণীমূলক ব্যাক জেসচার অ্যানিমেশন পরীক্ষা করুন
আপনি যদি এখনও Android 13 বা Android 14 ব্যবহার করেন, তাহলে আপনি চিত্র 1-এ দেখানো ব্যাক-টু-হোম অ্যানিমেশন পরীক্ষা করতে পারেন।
এই অ্যানিমেশন পরীক্ষা করতে, নিম্নলিখিত পদক্ষেপগুলি সম্পূর্ণ করুন:
আপনার ডিভাইসে, সেটিংস > সিস্টেম > বিকাশকারী বিকল্পগুলিতে যান।
ভবিষ্যদ্বাণীমূলক ব্যাক অ্যানিমেশন নির্বাচন করুন।
আপনার আপডেট করা অ্যাপটি চালু করুন এবং এটিকে কার্যকর দেখতে পিছনের অঙ্গভঙ্গিটি ব্যবহার করুন৷