When creating Wear OS apps for China, you need to account for handsets without Google Play services pre-installed. This page contains common changes that developers might need to adopt for the Chinese market.
Use the correct version of Google Play services
Google Play services version 10.2.0 provides worldwide support for the Fused Location Provider API and the Data Layer API. You must use this version of Google Play services if you use these APIs to ensure support of a wider array of Wear OS devices in China. In other cases this dependency is optional.
Note: Although Google Play services contains
APIs for Wear OS apps, Wear OS apps for China should continue to
use APIs related to GoogleApiClient
; see
Access the Wearable API.
Fused Location Provider API
If you use the Fused Location Provider API, include the following dependency in
the build.gradle
file of your Wear OS module:
Groovy
dependencies { ... implementation 'com.google.android.gms:play-services-location:10.2.0' }
Kotlin
dependencies { ... implementation("com.google.android.gms:play-services-location:10.2.0") }
Data Layer API
If your app uses the Data Layer API, you need to add the following line to the
build.gradle
file of your Wear OS module. The line requires use of the 10.2.0 version of
the client library.
Groovy
dependencies { ... implementation 'com.google.android.gms:play-services-wearable:10.2.0' ... }
Kotlin
dependencies { ... implementation("com.google.android.gms:play-services-wearable:10.2.0") ... }
Add the following line to the build.gradle
file of
your mobile module. Replace the Google Play services dependency with a reference to the
10.2.0 version.
Groovy
dependencies { ... implementation 'com.google.android.gms:play-services-wearable:10.2.0' }
Kotlin
dependencies { ... implementation("com.google.android.gms:play-services-wearable:10.2.0") }
Authentication
Before implementing authentication, review your use cases to see whether authentication is actually needed. For example, for an app delivering the weather forecast, there likely is no need for sign-in and thus for authentication.
If you do require authentication, we recommend using the AndroidX Oauth library. This requires using the Authorization Code Grant with PKCE flow. You can also use one of the other methods described in Authentication on wearables. Use of the Wearable Support Library is not recommended.
For more information, see the Wear OS OAuth Sample on GitHub.
Bridged notifications
Bridged notifications aren't supported in China. Phone notifications are bridged to Wear OS only if the Wear OS device is connected to the phone using Bluetooth.
Location and mapping coordinates compatibility
Use the
FusedLocationProvider
(FLP) to detect the user's location in China, as you would
for the rest of the world. This ensures that your app takes into account the best information
regardless of the watch hardware and the phone platform that the watch is paired to.
Using the FLP also adds battery optimization that is built into the Wear OS platform.
When integrating FusedLocationProvider
with third-party map SDKs,
take into account the coordinates compatibility among providers.
FusedLocationProvider
reports the location according to the
WGS84 standard.
Be sure to convert coordinate systems, as appropriate.
Google Fit support
Google Fit's accumulated-step counter, move minutes, and heart points are supported in China, with up to seven days of history. You can access this without providing a user credential.
Voice action support
The Wear OS platform provides several voice intents that are based on user actions such as _"Show heart rate"_ or _"Set an alarm"_. This lets users say what they want to do and lets the system figure out the best activity to start.
When users speak a voice action, your app can filter for the intent that is fired to start an
activity. To start a service in the background, show an activity as a visual cue and start the
service in the activity. Make sure to call
finish()
to get rid of the visual cue.
Here is a list of the voice intents supported by the Wear OS platform:
Category | Example | Intent spec |
Car hailing | 打车去三里屯 | Action
Extra
The extra is optional. |
Set alarm | 设置一个明早七点的闹钟 | Action
Extras
These extras are optional. Provide either, both, or neither of these extras. |
Set timer | 设置一个三分钟的倒计时 | Action
Extras
|
Start stopwatch | 开始计时 | Action
|
Start or stop a bike ride | 开始骑车 | Action
Mime type
Extras
|
Start or stop a run | 开始跑步 | Action
Mime type
Extras
|
Start or stop a workout | 开始锻炼 | Action
Mime type
Extras
|
Show heart rate | 查看心率 | Action
Mime type
|
Show step count | 查看步数 | Action
Mime type
|
Navigation | 导航去三里屯 | Action
Data geo:latitude,longitude?q=融科资讯中心 |
Voice Assistant can also use existing Android common intents to trigger certain behaviors where applicable.
Emulator support
You can use the China version of the Wear OS emulator image to test your apps. This is supported by Android Studio 3.0 and higher.
To test your apps on the China version of the emulator, follow these steps:
- Install the Android Emulator.
- Download the Wear OS for China images from the SDK manager. Use the version for Wear OS 3.5 (API level 30).
- Choose the Wear OS for China image when creating an AVD profile.
- Run the Wear OS for China emulator for development.
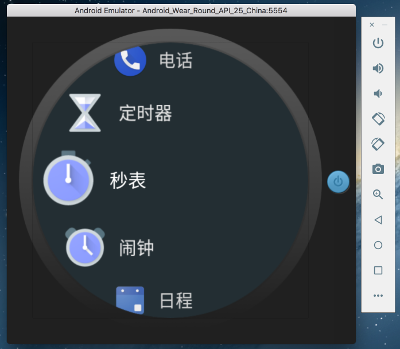
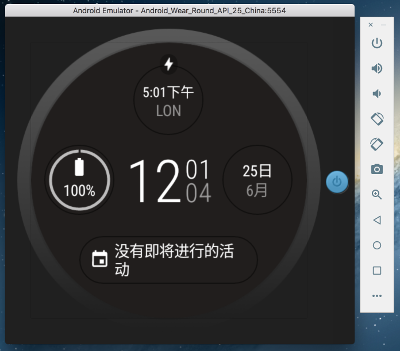
Figure 1. Examples of the China version of the Wear OS emulator.
This version of the Wear OS emulator comes with several pre-installed apps:
- Ambient mode
- Contacts
- Google Handwriting Input
- Google Play services
- Health Services for Wear OS
- Hotword recognition for LE devices
- Pinyin
- Play Store (adapted for devices in China)
- Pocketwatch
- TalkBack
- Watchfaces (both analog and digital versions)
- Wear Core Services
Initiate an app-specific Bluetooth and Wi-Fi channel
Wear OS automatically routes network requests. In most cases, there's no requirement for the app to open an app-specific Bluetooth and Wi-Fi channel.
If an app does request an app-specific Bluetooth and Wi-Fi channel in China, the request
silently fails. Instead, a dialog displays
asking the user for confirmation. If the user confirms, the channel opens. This happens every
time, not just on first use. BluetoothAdapter.enable()
or
WifiManager.setEnabled(true)
is
called.
Note: For an app targeting
Android 10 (API level 29) or higher to call
WifiManager.setEnabled()
, it must be a system app or a
device policy controller (DPC).
Permission review mode
In China, Wear OS for China devices run in Permission Review Mode, which
imposes some limits on how to use apps with a targetApiLevel
lower than 23. Review the following limits:
- Even though permissions are granted at installation time, when an app with a
targetApiLevel
lower than 23 starts for the first time, a dialog appears asking the user to confirm permissions for this app. - Components in the app, such as broadcast receivers, services, and activities don't respond to corresponding events before the app is used for the first time.
As a result, we recommend you use targetApiLevel
23 or higher
and adopt the
app permissions
best practices.
Use other Google Play services APIs
If your app uses Google Play services APIs other than the Wearable API, then your app needs to check whether these APIs are available to use during runtime and respond appropriately. There are two ways to check the availability of Google Play service APIs:
- Use a separate
GoogleApiClient
instance for connecting to other APIs. This interface contains callbacks to alert your app to the success or failure of the connection. In the case of failed connection, theConnectionResult
showsAPI_UNAVAILABLE
. To learn how to handle connection failures, see Access Google APIs. - Use the
addApiIfAvailable()
method ofGoogleApiClient.Builder
to connect to the required APIs. After theonConnected()
callback fires, use thehasConnectedApi()
method to ensure that each of the requested APIs is connected correctly.
Distribute apps in China
To effectively reach users of Wear OS for China, you can distribute through third-party Wear OS app stores such as the following:
- Galaxy Store for Samsung devices
- Xiaomi Store for Xiaomi devices
- Mobvoi for all other devices