This document describes how to migrate existing games from the games v1 SDK to the games v2 SDK. The Play Games plugin for Unity, versions 10 and earlier, uses the games v1 SDK.
Before you begin
- Make sure that you have already set up Play Console and installed the Unity Hub.
Download the Google Play Games plugin for Unity
To benefit from the latest features in the Play Games Services, download and install the latest plugin version. Download it from the gitHub repository.
Remove the old plugin
In the Unity Hub, remove the following folders or files.
Assets/GooglePlayGames Assets/GeneratedLocalRepo/GooglePlayGames Assets/Plugins/Android/GooglePlayGamesManifest.androidlib Assets/Plugins/Android
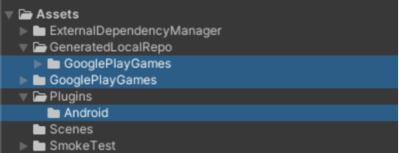
Import the new plugin to your Unity project
To import the plugin to your Unity project, follow these steps:
- Open your game project.
- In the Unity Hub, click Assets > Import Package > Custom Package
to import the downloaded
unitypackage
file into your project's assets. Make sure that your current build platform is set to Android.
In the main menu, click File > Build Settings.
Select Android and click Switch Platform.
There should be a new menu item under Window > Google Play Games. If there isn't, refresh the assets by clicking Assets > Refresh and then try setting the build platform again.
In the Unity Hub, click File > Build Settings > Player Settings > Other Settings.
In the Target API level box, select a version.
In the Scripting backend box, enter
IL2CPP
.In the Target architectures box, select a value.
Note the package name package_name.You can use this information later.
The player settings in your Unity project.
Update automatic sign-in code
Replace the PlayGamesClientConfiguration
initialization class with the
PlayGamesPlatform.Instance.Authenticate()
class.
The initialization and activation of PlayGamesPlatform
is not required.
Calling PlayGamesPlatform.Instance.Authenticate()
fetches the result of
automatic sign-in.
C#
In the Unity Hub, locate the files with
PlayGamesClientConfiguration
class.
using GooglePlayGames;
using GooglePlayGames.BasicApi;
using UnityEngine.SocialPlatforms;
public void Start() {
PlayGamesClientConfiguration config =
new PlayGamesClientConfiguration.Builder()
// Enables saving game progress
.EnableSavedGames()
// Requests the email address of the player be available
// will bring up a prompt for consent
.RequestEmail()
// Requests a server auth code be generated so it can be passed to an
// associated backend server application and exchanged for an OAuth token
.RequestServerAuthCode(false)
// Requests an ID token be generated. This OAuth token can be used to
// identify the player to other services such as Firebase.
.RequestIdToken()
.Build();
PlayGamesPlatform.InitializeInstance(config);
// recommended for debugging:
PlayGamesPlatform.DebugLogEnabled = true;
// Activate the Google Play Games platform
PlayGamesPlatform.Activate();
}
And update it to this:
using GooglePlayGames;
public void Start() {
PlayGamesPlatform.Instance.Authenticate(ProcessAuthentication);
}
internal void ProcessAuthentication(SignInStatus status) {
if (status == SignInStatus.Success) {
// Continue with Play Games Services
} else {
// Disable your integration with Play Games Services or show a login
// button to ask users to sign-in. Clicking it should call
// PlayGamesPlatform.Instance.ManuallyAuthenticate(ProcessAuthentication).
}
}
Choose a social platform
To choose a social platform, see choose a social platform.
Retrieve server authentication codes
To get server side access codes, see retrieve server authentication codes.
Remove sign-out code
Remove the code for sign-out. Play Games Services no longer requires an in-game sign-out button.
Remove the code shown in the following example:
C#
// sign out
PlayGamesPlatform.Instance.SignOut();
Test your game
Ensure your game functions as designed by testing it. The tests you perform depend on your game's features.
The following is a list of common tests to run.
Successful sign-in.
Automatic sign-in works. The user should be signed in to Play Games Services upon launching the game.
The welcome popup is displayed.
Successful log messages are displayed. Run the following command in the terminal:
adb logcat | grep com.google.android.
A successful log message is shown in the following example:
[
$PlaylogGamesSignInAction$SignInPerformerSource@e1cdecc number=1 name=GAMES_SERVICE_BROKER>], returning true for shouldShowWelcomePopup. [CONTEXT service_id=1 ]
Ensure UI component consistency.
Pop ups, leaderboards, and achievements display correctly and consistently on various screen sizes and orientations in the Play Games Services user interface (UI).
Sign-out option is not visible in the Play Games Services UI.
Make sure you can successfully retrieve Player ID, and if applicable, server-side capabilities work as expected.
If the game uses server-side authentication, thoroughly test the
requestServerSideAccess
flow. Ensure the server receives the auth code and can exchange it for an access token. Test both success and failure scenarios for network errors, invalidclient ID
scenarios.
If your game was using any of the following features, test them to ensure that they work the same as before the migration:
- Leaderboards: Submit scores and view leaderboards. Check for the correct ranking and display of player names and scores.
- Achievements: Unlock achievements and verify they are correctly recorded and displayed in the Play Games UI.
- Saved Games: If the game uses saved games, ensure that saving and loading the game progress works flawlessly. This is particularly critical to test across multiple devices and after app updates.
Post migration tasks
Complete the following steps after you have migrated to games v2 SDK.