透過 Compose,您可以建立以多邊形製成的形狀。舉例來說,您可以建立以下類型的形狀:
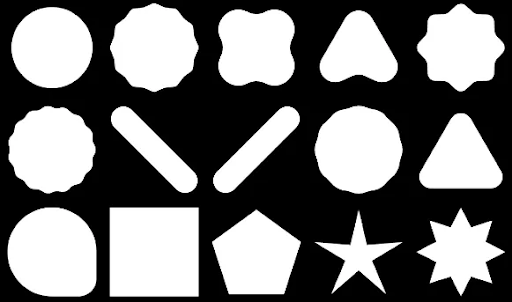
如要在 Compose 中建立自訂圓角多邊形,請將 graphics-shapes
依附元件新增至 app/build.gradle
:
implementation "androidx.graphics:graphics-shapes:1.0.0-alpha05"
這個程式庫可讓您建立由多邊形組成的形狀。雖然多邊形形狀只有直邊和銳利的邊角,但這些形狀允許選用的圓角。可讓您輕鬆地在兩種形狀之間不斷變換。任意形狀之間難以構思,而且通常在設計期間遭遇問題。但這個程式庫透過在相似多邊形結構類似的形狀之間進行變換,來簡化作業。
建立多邊形
下列程式碼片段會建立基本的多邊形形狀,在繪製區域中心有 6 個路徑點:
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 6, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2 ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Blue) } } .fillMaxSize() )
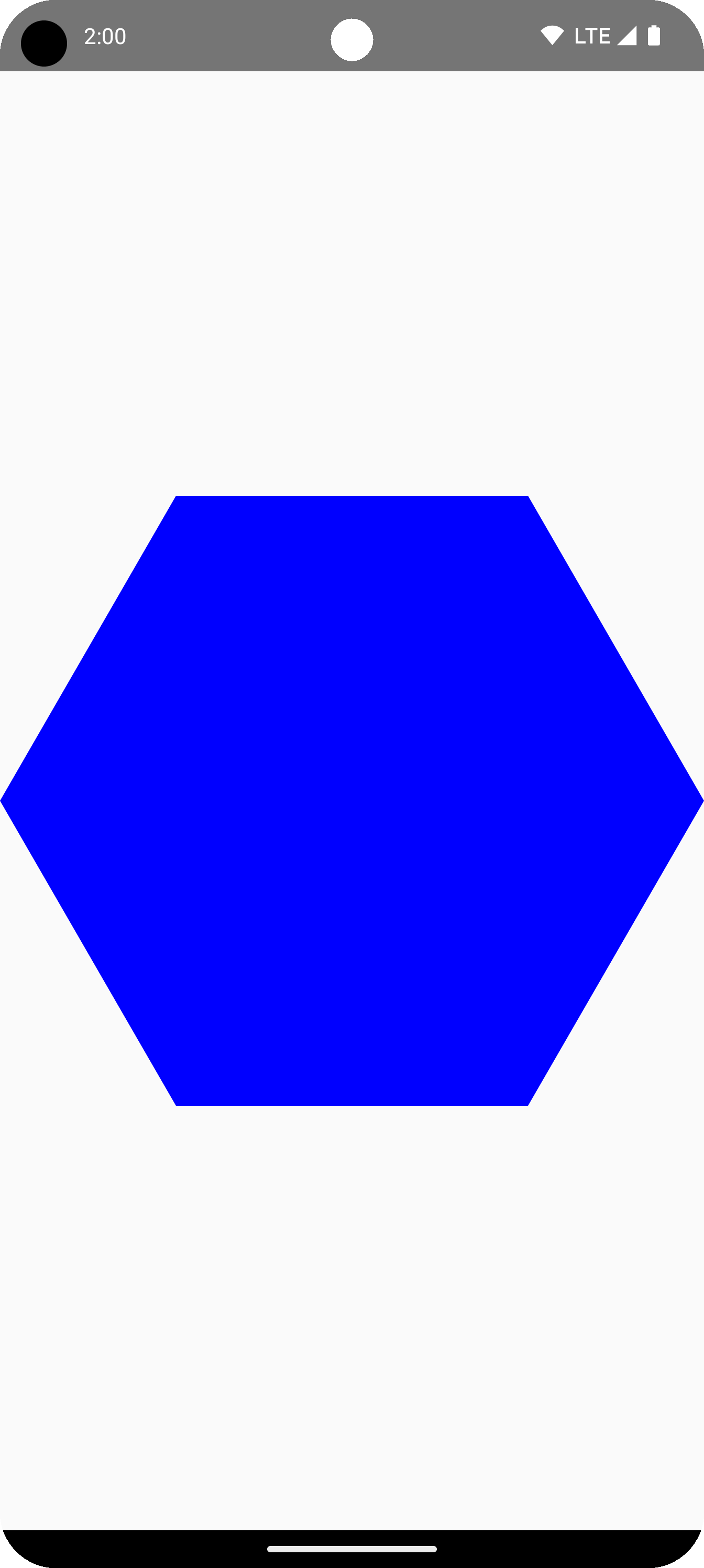
在這個範例中,程式庫會建立 RoundedPolygon
,其中包含代表要求的形狀的幾何圖形。如要在 Compose 應用程式中繪製該形狀,您必須從其取得 Path
物件,讓形狀變成一種格式,Compose 知道如何繪圖。
將多邊形的邊角設為圓角
如要將多邊形的邊角設為圓角,請使用 CornerRounding
參數。這會採用兩個參數:radius
和 smoothing
。每個圓角由 1 至 3 立方體曲線組成,其中心擁有圓形弧形,雙邊 (「傾斜」) 曲線會從形狀邊緣轉換成中心曲線。
Radius
radius
是用於將頂點四捨五入的圓形半徑。
例如,以下圓角三角形的製作方式如下:
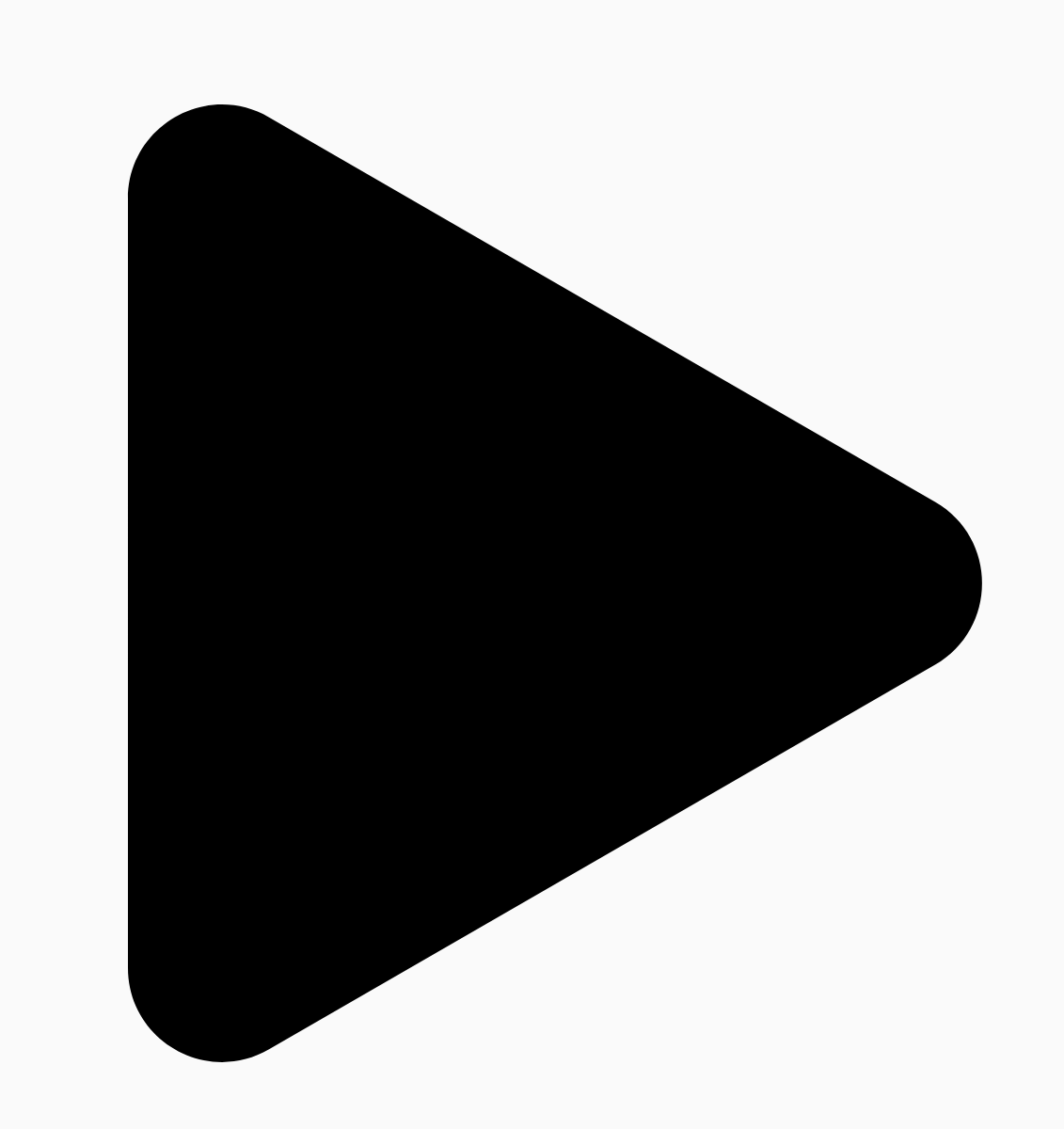
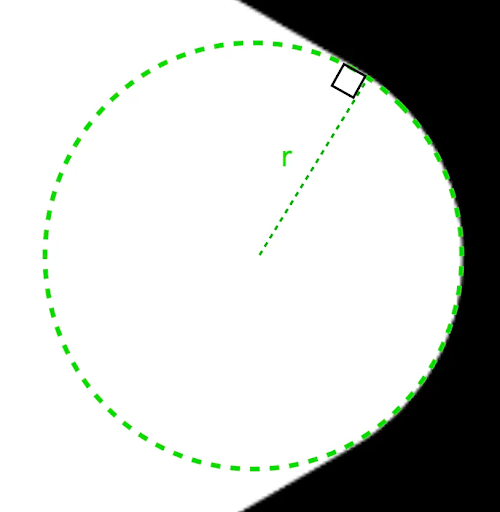
r
會決定圓角的圓形圓角大小。平滑程度
平滑是影響因素,可決定從角落的圓角捨入部分到邊緣所需的時間。平滑係數為 0 (不流暢,CornerRounding
的預設值) 會產生純圓角。非零平滑係數 (上限為 1.0 上限) 會導致邊角四捨五入為三個獨立的曲線。
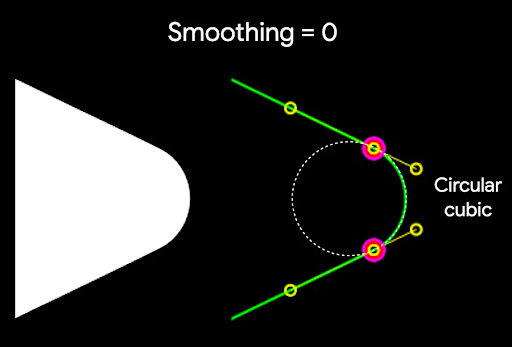
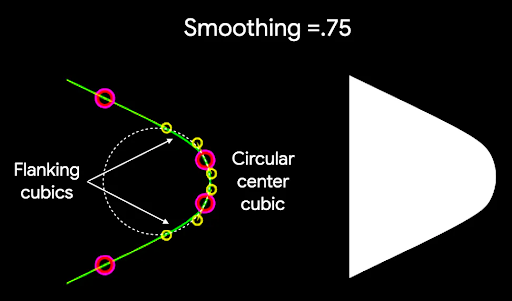
例如,以下程式碼片段展示了將平滑設為 0 與 1 設定之間的細微差異:
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Black) } } .size(100.dp) )
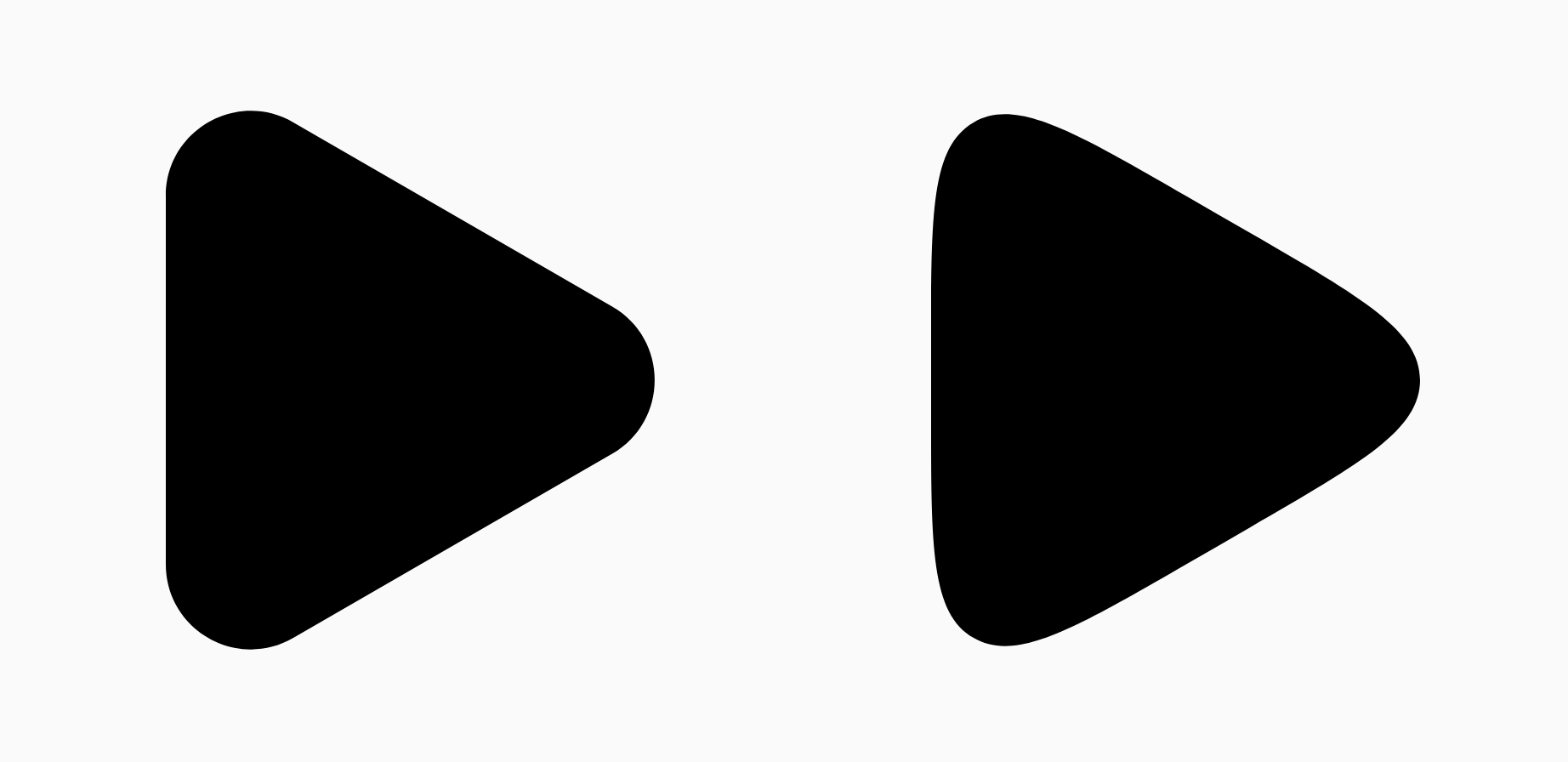
大小和位置
根據預設,建立形狀時,會以 1
為中心點 (0, 0
) 的半徑。這個半徑代表形狀的多邊形中心點與外部頂點之間的距離。請注意,將邊角四捨五入會導致形狀較小,因為圓角與圓角相距接近中心點。如要調整多邊形大小,請調整 radius
值。如要調整位置,請變更多邊形的 centerX
或 centerY
。或者,您也可以使用標準 DrawScope
轉換函式 (例如 DrawScope#translate()
) 轉換物件來變更物件的大小、位置和旋轉方式。
變形圖案
Morph
物件是一種新形狀,代表兩個多邊形形狀之間的動畫。如要在兩個形狀之間變換,請建立兩個 RoundedPolygons
和 Morph
物件,納入這兩個形狀。如要計算起始和結束形狀之間的形狀,請提供介於 0 至 1 之間的 progress
值,以便判斷形狀在起始 (0) 和結束 (1) 形狀之間:
Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = 0.5f).asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
在上述範例中,進度正介於兩個形狀 (圓角三角形和正方形) 之間,產生以下結果:
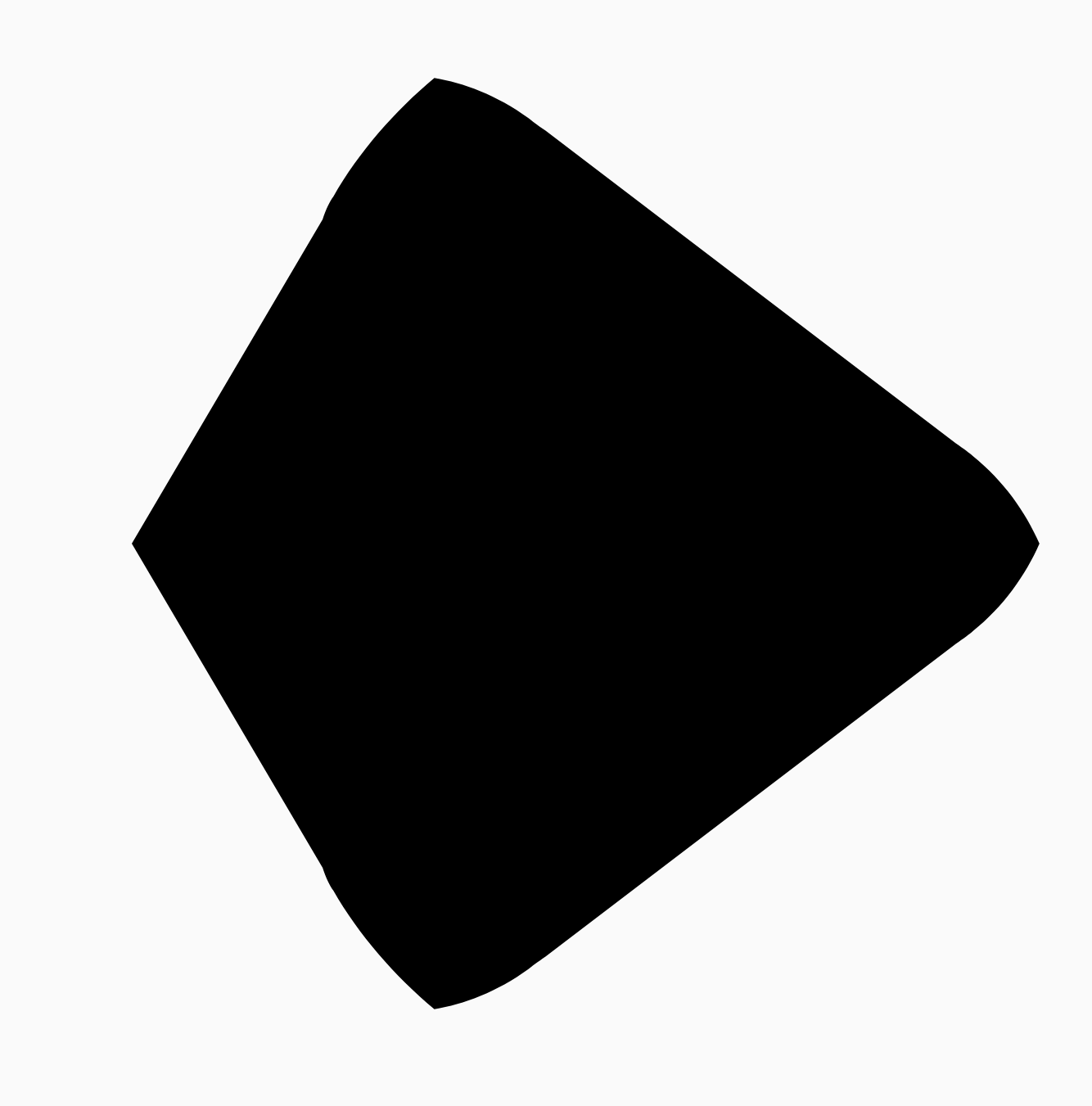
在大多數情況下,系統會在動畫中完成變形,而不只是在靜態算繪中。如要在兩者之間建立動畫,您可以使用標準 Compose 中的動畫 API 隨時變更進度值。舉例來說,您可以在這兩種形狀之間無限次建立變形動畫,如下所示:
val infiniteAnimation = rememberInfiniteTransition(label = "infinite animation") val morphProgress = infiniteAnimation.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(500), repeatMode = RepeatMode.Reverse ), label = "morph" ) Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = morphProgress.value) .asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
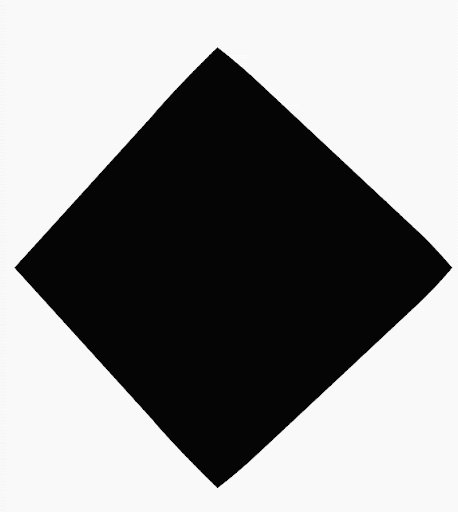
使用多邊形做為片段
在 Compose 中使用 clip
修飾符來變更可組合項的算繪方式,並利用在裁剪區域周圍繪製的陰影,是常見的做法:
fun RoundedPolygon.getBounds() = calculateBounds().let { Rect(it[0], it[1], it[2], it[3]) } class RoundedPolygonShape( private val polygon: RoundedPolygon, private var matrix: Matrix = Matrix() ) : Shape { private var path = Path() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { path.rewind() path = polygon.toPath().asComposePath() matrix.reset() val bounds = polygon.getBounds() val maxDimension = max(bounds.width, bounds.height) matrix.scale(size.width / maxDimension, size.height / maxDimension) matrix.translate(-bounds.left, -bounds.top) path.transform(matrix) return Outline.Generic(path) } }
接著,您可以使用多邊形做為剪輯片段,如以下程式碼片段所示:
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier .clip(clip) .background(MaterialTheme.colorScheme.secondary) .size(200.dp) ) { Text( "Hello Compose", color = MaterialTheme.colorScheme.onSecondary, modifier = Modifier.align(Alignment.Center) ) }
這會產生以下結果:
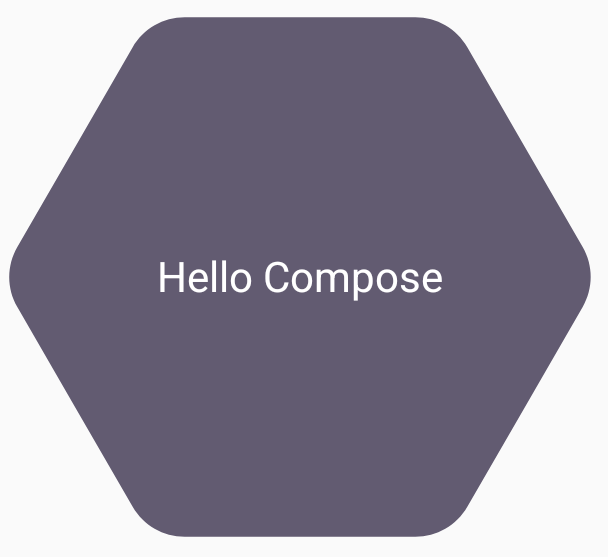
這可能與之前算繪的內容不同,但允許在 Compose 中使用其他功能。舉例來說,這項技巧可用來裁剪圖片,並在裁剪區域周圍套用陰影:
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .graphicsLayer { this.shadowElevation = 6.dp.toPx() this.shape = clip this.clip = true this.ambientShadowColor = Color.Black this.spotShadowColor = Color.Black } .size(200.dp) ) }
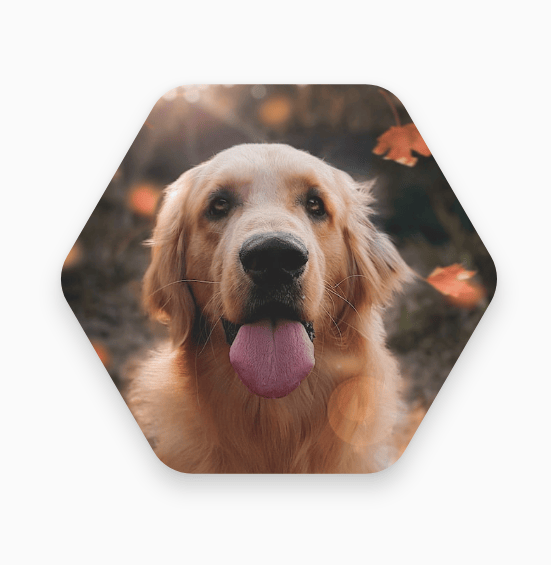
點擊上的跳轉按鈕
您可以使用 graphics-shape
程式庫建立按鈕,按下後兩個形狀之間會出現變形。首先,請建立可擴充 Shape
的 MorphPolygonShape
,將其縮放並轉譯成適當大小。請注意傳遞進度,以便讓形狀產生動畫效果:
class MorphPolygonShape( private val morph: Morph, private val percentage: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } }
如要使用這個變形形狀,請建立兩個多邊形:shapeA
和 shapeB
。請建立並記住 Morph
。接著,使用 interactionSource
做為動畫後方的驅動力,將變形套用至按鈕做為片段輪廓:
val shapeA = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 6, rounding = CornerRounding(0.1f) ) } val morph = remember { Morph(shapeA, shapeB) } val interactionSource = remember { MutableInteractionSource() } val isPressed by interactionSource.collectIsPressedAsState() val animatedProgress = animateFloatAsState( targetValue = if (isPressed) 1f else 0f, label = "progress", animationSpec = spring(dampingRatio = 0.4f, stiffness = Spring.StiffnessMedium) ) Box( modifier = Modifier .size(200.dp) .padding(8.dp) .clip(MorphPolygonShape(morph, animatedProgress.value)) .background(Color(0xFF80DEEA)) .size(200.dp) .clickable(interactionSource = interactionSource, indication = null) { } ) { Text("Hello", modifier = Modifier.align(Alignment.Center)) }
輕觸方塊時就會產生以下動畫:
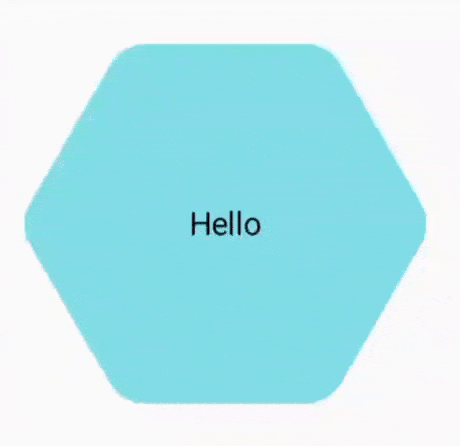
呈現無限變形的動畫
如要持續為變形形狀加上動畫效果,請使用 rememberInfiniteTransition
。下方的個人資料相片範例會任意變更形狀 (及旋轉)。這個方法會對上述 MorphPolygonShape
進行微幅調整:
class CustomRotatingMorphShape( private val morph: Morph, private val percentage: Float, private val rotation: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) matrix.rotateZ(rotation) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } } @Preview @Composable private fun RotatingScallopedProfilePic() { val shapeA = remember { RoundedPolygon( 12, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 12, rounding = CornerRounding(0.2f) ) } val morph = remember { Morph(shapeA, shapeB) } val infiniteTransition = rememberInfiniteTransition("infinite outline movement") val animatedProgress = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(2000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) val animatedRotation = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 360f, animationSpec = infiniteRepeatable( tween(6000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .clip( CustomRotatingMorphShape( morph, animatedProgress.value, animatedRotation.value ) ) .size(200.dp) ) } }
此程式碼提供以下有趣的結果:
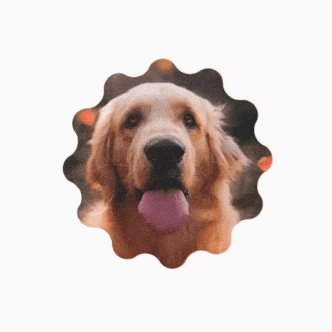
自訂多邊形
如果從一般多邊形建立的形狀未涵蓋您的用途,您可以使用端點清單建立更多自訂形狀。舉例來說,您可能想建立像這樣的心形形狀:
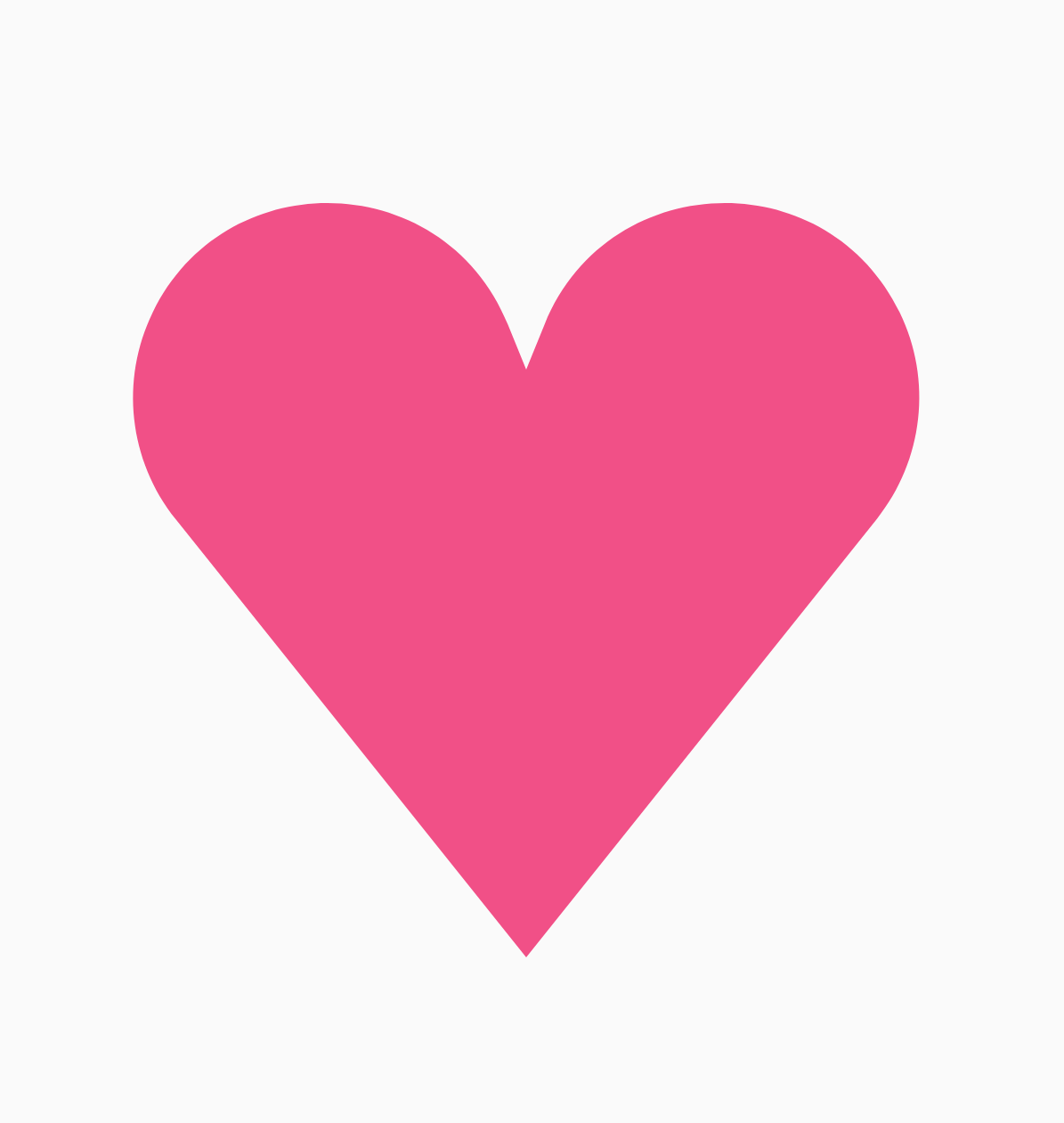
您可以使用接受 x, y 座標的浮點陣列 RoundedPolygon
超載,指定此形狀的個別頂點。
為了分割心形多邊形,請注意,指定點的極座標系統比使用笛卡兒 (x,y) 座標系統更加容易 (其中 0°
從右側開始,然後以順時針方向繼續,270°
為 12 點鐘位置):
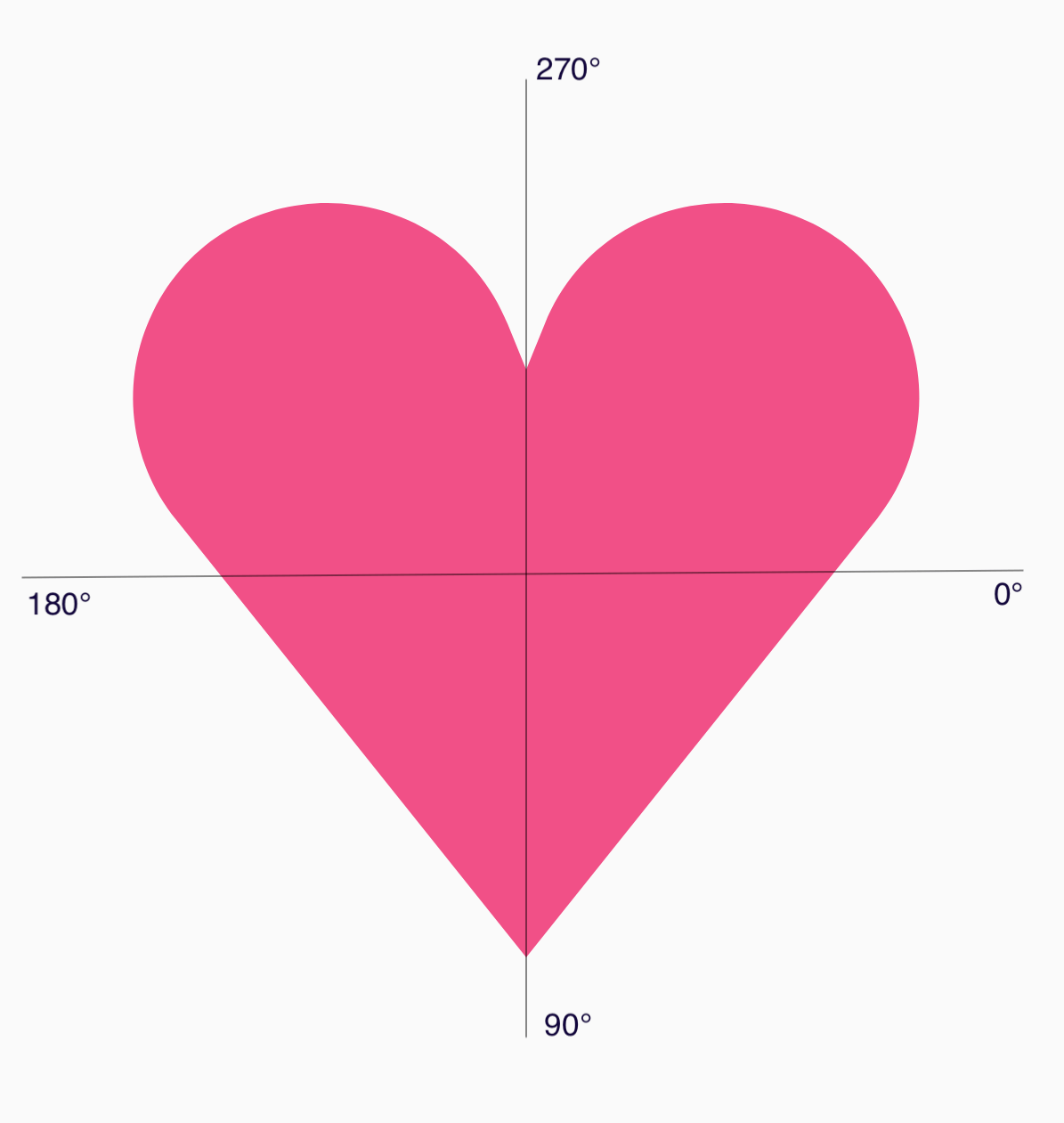
您現在可以透過指定每個點的中心角度 (Θ) 和半徑,以更簡單的方式定義形狀:
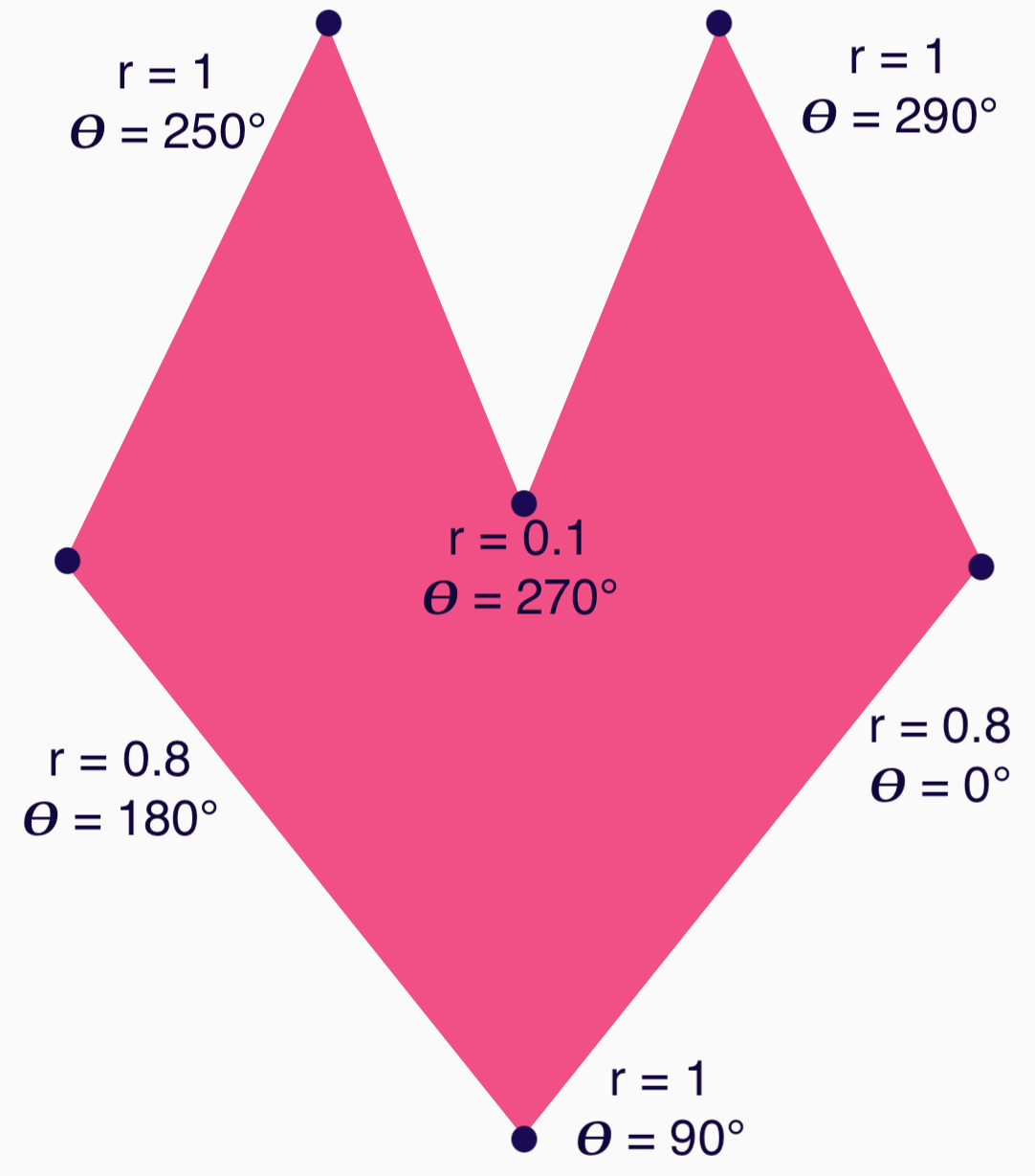
您現在可以建立端點,並傳遞至 RoundedPolygon
函式:
val vertices = remember { val radius = 1f val radiusSides = 0.8f val innerRadius = .1f floatArrayOf( radialToCartesian(radiusSides, 0f.toRadians()).x, radialToCartesian(radiusSides, 0f.toRadians()).y, radialToCartesian(radius, 90f.toRadians()).x, radialToCartesian(radius, 90f.toRadians()).y, radialToCartesian(radiusSides, 180f.toRadians()).x, radialToCartesian(radiusSides, 180f.toRadians()).y, radialToCartesian(radius, 250f.toRadians()).x, radialToCartesian(radius, 250f.toRadians()).y, radialToCartesian(innerRadius, 270f.toRadians()).x, radialToCartesian(innerRadius, 270f.toRadians()).y, radialToCartesian(radius, 290f.toRadians()).x, radialToCartesian(radius, 290f.toRadians()).y, ) }
您必須使用這個 radialToCartesian
函式,將頂點轉譯為笛卡兒座標:
internal fun Float.toRadians() = this * PI.toFloat() / 180f internal val PointZero = PointF(0f, 0f) internal fun radialToCartesian( radius: Float, angleRadians: Float, center: PointF = PointZero ) = directionVectorPointF(angleRadians) * radius + center internal fun directionVectorPointF(angleRadians: Float) = PointF(cos(angleRadians), sin(angleRadians))
上述程式碼能提供心臟的原始頂點,但是您需要將特定邊角四捨五入,才能取得選定的心形形狀。90°
和 270°
的邊角沒有四捨五入,但其他邊角則需要四捨五入。如要為個別邊角自訂四捨五入,請使用 perVertexRounding
參數:
val rounding = remember { val roundingNormal = 0.6f val roundingNone = 0f listOf( CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), ) } val polygon = remember(vertices, rounding) { RoundedPolygon( vertices = vertices, perVertexRounding = rounding ) } Box( modifier = Modifier .drawWithCache { val roundedPolygonPath = polygon.toPath().asComposePath() onDrawBehind { scale(size.width * 0.5f, size.width * 0.5f) { translate(size.width * 0.5f, size.height * 0.5f) { drawPath(roundedPolygonPath, color = Color(0xFFF15087)) } } } } .size(400.dp) )
這會造成粉紅色的心:
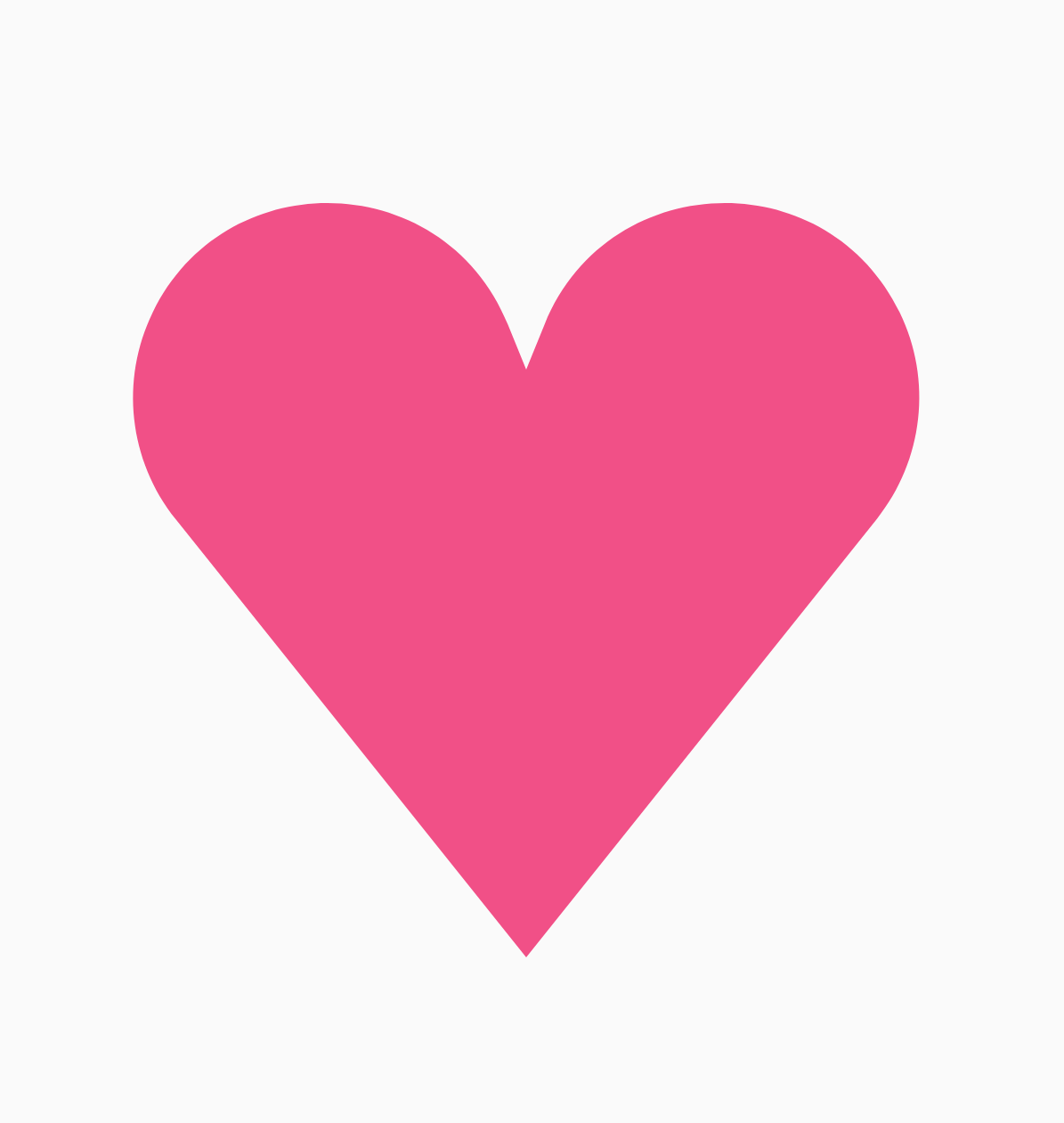
如果上述形狀皆不適用於您的用途,建議您使用 Path
類別來繪製自訂形狀,或是從磁碟載入 ImageVector
檔案。graphics-shapes
程式庫不適用於任何形狀,但專門用於簡化圓角多邊形和變形動畫的建立作業。
其他資源
如需詳細資訊和範例,請參閱下列資源: