A Floating Action Button (FAB) is a high-emphasis button that lets the user perform a primary action in an application. It promotes a single, focused action that is the most common pathway a user might take and is typically found anchored to the bottom right of the screen.
Consider these three use cases where you might use a FAB:
- Create new item: In a note-taking app, a FAB might be used to quickly create a new note.
- Add new contact: In a chat app, a FAB could open an interface that lets the user add someone to a conversation.
- Center location: In a map interface, a FAB could center the map on the user's current location.
In Material Design, there are four types of FAB:
- FAB: A floating action button of ordinary size.
- Small FAB: A smaller floating action button.
- Large FAB: A larger floating action button.
- Extended FAB: A floating action button that contains more than just an icon.
API surface
Although there are several composables you can use to create floating action buttons consistent with Material Design, their parameters don't differ greatly. Among the key parameters you should keep in mind are the following:
onClick
: The function called when the user presses the button.containerColor
: The color of the button.contentColor
: The color of the icon.
Floating action button
To create a general floating action button, use the basic
FloatingActionButton
composable. The following example demonstrates a
basic implementation of a FAB:
@Composable fun Example(onClick: () -> Unit) { FloatingActionButton( onClick = { onClick() }, ) { Icon(Icons.Filled.Add, "Floating action button.") } }
This implementation appears as follows:
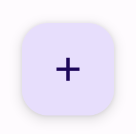
Small button
To create a small floating action button, use the
SmallFloatingActionButton
composable. The following example demonstrates
how to do so, with the addition of custom colors.
@Composable fun SmallExample(onClick: () -> Unit) { SmallFloatingActionButton( onClick = { onClick() }, containerColor = MaterialTheme.colorScheme.secondaryContainer, contentColor = MaterialTheme.colorScheme.secondary ) { Icon(Icons.Filled.Add, "Small floating action button.") } }
This implementation appears as follows:
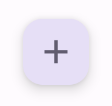
Large button
To create a large floating action button, use the
LargeFloatingActionButton
composable. This composable is not
significantly different from the other examples aside from the fact that it
results in a bigger button.
The following is a straightforward implementation of a large FAB.
@Composable fun LargeExample(onClick: () -> Unit) { LargeFloatingActionButton( onClick = { onClick() }, shape = CircleShape, ) { Icon(Icons.Filled.Add, "Large floating action button") } }
This implementation appears as follows:
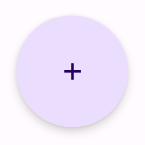
Extended button
You can create more complex floating action buttons with the
ExtendedFloatingActionButton
composable. The key difference between it
and FloatingActionButton
is that it has dedicated icon
and text
parameters. They let you create a button with more complex content that scales
to fit its content appropriately.
The following snippet demonstrates how to implement
ExtendedFloatingActionButton
, with example values passed for icon
and
text
.
@Composable fun ExtendedExample(onClick: () -> Unit) { ExtendedFloatingActionButton( onClick = { onClick() }, icon = { Icon(Icons.Filled.Edit, "Extended floating action button.") }, text = { Text(text = "Extended FAB") }, ) }
This implementation appears as follows:
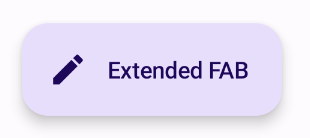