輪轉介面會顯示可捲動的項目清單,這些項目會根據視窗大小動態調整。使用輪轉介面展示相關內容集合。輪轉介面項目著重於視覺效果,但也可以包含可配合項目大小調整的簡短文字。
輪轉介面有四種版面配置,可配合不同用途:
- 多瀏覽:包含不同尺寸的商品。建議用於瀏覽多個項目 (例如相片)。
- Uncontained:包含單一大小的項目,並流過螢幕邊緣。可自訂,在每個項目上方或下方顯示更多文字或其他 UI。
- 主圖片:以大圖片突顯重點,並透過小圖片提供接下來要顯示的內容。建議用於強調你想強調的內容,例如電影或節目的縮圖。
- 全螢幕:一次顯示一個從邊到邊的大型項目,並垂直捲動。建議用於高度大於寬度的內容。
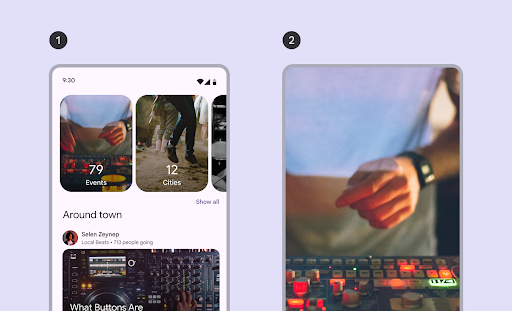
本頁說明如何實作多瀏覽和不含容器的輪轉介面版面配置。如要進一步瞭解版面配置類型,請參閱 Carousel Material 3 規範。
API 介面
如要實作多瀏覽和不含容器的輪轉介面,請使用 HorizontalMultiBrowseCarousel
和 HorizontalUncontainedCarousel
可組合函式。這些可組合項共用下列重要參數:
state
:管理目前項目索引和捲動位置的CarouselState
例項。使用rememberCarouselState { itemCount }
建立此狀態,其中itemCount
是輪轉介面中的項目總數。itemSpacing
:定義輪轉介面中相鄰項目之間的空白空間大小。contentPadding
:在輪轉介面的內容區域周圍套用邊框間距。您可以使用這個屬性,在第一個項目之前或最後一個項目之後新增間距,或是為捲動區域中的項目提供邊界。content
:可接收整數索引的可組合函式。使用這個 lambda 函式,根據輪轉介面中的索引定義每個項目的 UI。
這些可組合項的項目大小指定方式有所不同:
itemWidth
(適用於HorizontalUncontainedCarousel
):指定未包含的輪轉介面中每個項目的確切寬度。preferredItemWidth
(適用於HorizontalMultiBrowseCarousel
):建議多瀏覽輪轉介面中項目的理想寬度,讓元件在空間許可的情況下顯示多個項目。
範例:多瀏覽輪轉介面
這個程式碼片段會實作多瀏覽輪轉介面:
@Composable fun CarouselExample_MultiBrowse() { data class CarouselItem( val id: Int, @DrawableRes val imageResId: Int, val contentDescription: String ) val items = remember { listOf( CarouselItem(0, R.drawable.cupcake, "cupcake"), CarouselItem(1, R.drawable.donut, "donut"), CarouselItem(2, R.drawable.eclair, "eclair"), CarouselItem(3, R.drawable.froyo, "froyo"), CarouselItem(4, R.drawable.gingerbread, "gingerbread"), ) } HorizontalMultiBrowseCarousel( state = rememberCarouselState { items.count() }, modifier = Modifier .fillMaxWidth() .wrapContentHeight() .padding(top = 16.dp, bottom = 16.dp), preferredItemWidth = 186.dp, itemSpacing = 8.dp, contentPadding = PaddingValues(horizontal = 16.dp) ) { i -> val item = items[i] Image( modifier = Modifier .height(205.dp) .maskClip(MaterialTheme.shapes.extraLarge), painter = painterResource(id = item.imageResId), contentDescription = item.contentDescription, contentScale = ContentScale.Crop ) } }
程式碼的重點
- 定義
CarouselItem
資料類別,為輪轉介面中的每個元素建構資料結構。 - 建立並記住
CarouselItem
物件的List
,填入圖片資源和說明。 - 使用
HorizontalMultiBrowseCarousel
可組合函式,這是專門用於在輪轉介面中顯示多個項目。- 輪轉介面的狀態會使用
rememberCarouselState
進行初始化,並提供項目的總數。 - 項目具有
preferredItemWidth
(此處為186.dp
),可建議每個項目的最佳寬度。輪轉介面會使用這個值,判斷一次可在畫面上顯示多少個項目。 itemSpacing
參數會在項目之間加入小間距。HorizontalMultiBrowseCarousel
的結尾 lambda 會逐一處理CarouselItems
。在每次迭代中,它會擷取索引i
的項目,並為該項目算繪Image
可組合項。Modifier.maskClip(MaterialTheme.shapes.extraLarge)
會將預先定義的形狀遮罩套用至每個圖片,讓圖片的邊角變圓。contentDescription
會提供圖片的無障礙說明。
- 輪轉介面的狀態會使用
結果
下圖顯示上述程式碼片段的結果:
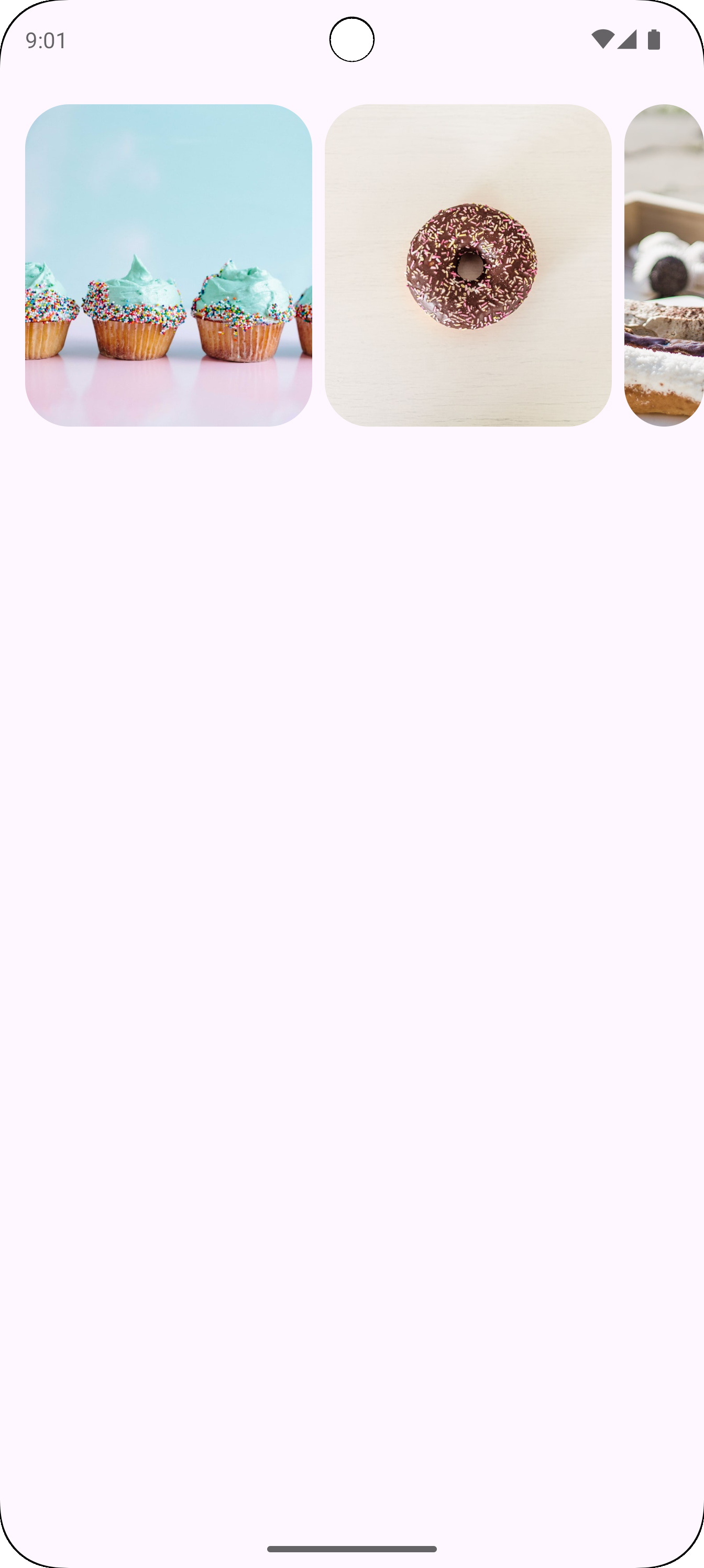
範例:不含容器的輪轉介面
以下程式碼片段實作不含容器的輪轉介面:
@Composable fun CarouselExample() { data class CarouselItem( val id: Int, @DrawableRes val imageResId: Int, val contentDescription: String ) val carouselItems = remember { listOf( CarouselItem(0, R.drawable.cupcake, "cupcake"), CarouselItem(1, R.drawable.donut, "donut"), CarouselItem(2, R.drawable.eclair, "eclair"), CarouselItem(3, R.drawable.froyo, "froyo"), CarouselItem(4, R.drawable.gingerbread, "gingerbread"), ) } HorizontalUncontainedCarousel( state = rememberCarouselState { carouselItems.count() }, modifier = Modifier .fillMaxWidth() .wrapContentHeight() .padding(top = 16.dp, bottom = 16.dp), itemWidth = 186.dp, itemSpacing = 8.dp, contentPadding = PaddingValues(horizontal = 16.dp) ) { i -> val item = carouselItems[i] Image( modifier = Modifier .height(205.dp) .maskClip(MaterialTheme.shapes.extraLarge), painter = painterResource(id = item.imageResId), contentDescription = item.contentDescription, contentScale = ContentScale.Crop ) } }
程式碼的重點
HorizontalUncontainedCarousel
可組合函式會建立輪轉介面版面配置。itemWidth
參數可為輪轉介面中的每個項目設定固定寬度。
結果
下圖顯示上述程式碼片段的結果:
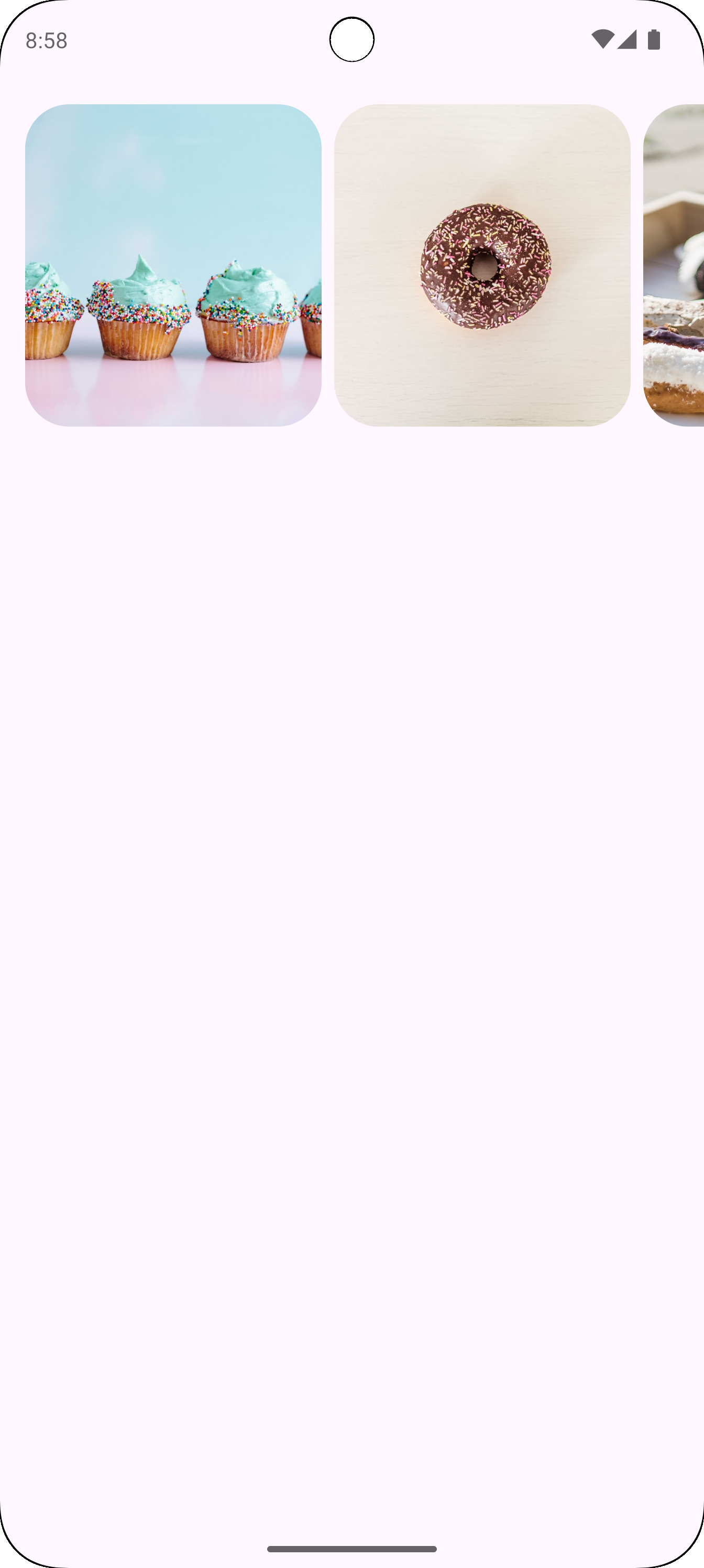