本指南說明如何在 Compose 中建立動態頂端應用程式列,在清單中選取項目時變更選項。您可以根據選取狀態修改頂端應用程式列的標題和動作。
實作動態頂端應用程式列行為
以下程式碼會為頂端應用程式列定義可組合函式,該函式會根據項目選取項目而變更:
@Composable fun AppBarSelectionActions( selectedItems: Set<Int>, modifier: Modifier = Modifier, ) { val hasSelection = selectedItems.isNotEmpty() val topBarText = if (hasSelection) { "Selected ${selectedItems.size} items" } else { "List of items" } TopAppBar( title = { Text(topBarText) }, colors = TopAppBarDefaults.topAppBarColors( containerColor = MaterialTheme.colorScheme.primaryContainer, titleContentColor = MaterialTheme.colorScheme.primary, ), actions = { if (hasSelection) { IconButton(onClick = { /* click action */ }) { Icon( imageVector = Icons.Filled.Share, contentDescription = "Share items" ) } } }, ) }
程式碼的重點
AppBarSelectionActions
會接受所選項目索引的Set
。topBarText
會根據是否選取任何項目而有所變更。- 選取項目後,
TopAppBar
會顯示描述所選項目數量的文字。 - 如果未選取任何項目,
topBarText
就是「項目清單」。
- 選取項目後,
actions
區塊會定義在頂端應用程式列中顯示的動作。如果hasSelection
為 true,文字後方會顯示分享圖示。IconButton
的onClick
lambda 會在點選圖示時處理分享動作。
結果

將可選清單整合至動態頂端應用程式列
以下範例說明如何在動態頂端應用程式列中加入可選取清單:
@Composable private fun AppBarMultiSelectionExample( modifier: Modifier = Modifier, ) { val listItems by remember { mutableStateOf(listOf(1, 2, 3, 4, 5, 6)) } var selectedItems by rememberSaveable { mutableStateOf(setOf<Int>()) } Scaffold( topBar = { AppBarSelectionActions(selectedItems) } ) { innerPadding -> LazyColumn(contentPadding = innerPadding) { itemsIndexed(listItems) { _, index -> val isItemSelected = selectedItems.contains(index) ListItemSelectable( selected = isItemSelected, Modifier .combinedClickable( interactionSource = remember { MutableInteractionSource() }, indication = null, onClick = { /* click action */ }, onLongClick = { if (isItemSelected) selectedItems -= index else selectedItems += index } ) ) } } } }
程式碼的重點
- 頂端列會根據所選清單項目數量更新。
selectedItems
會保留所選項目索引的集合。AppBarMultiSelectionExample
會使用Scaffold
來建構螢幕結構。topBar = { AppBarSelectionActions(selectedItems) }
將AppBarSelectionActions
可組合函式設為頂端應用程式列。AppBarSelectionActions
會收到selectedItems
狀態。
LazyColumn
會以垂直清單顯示項目,只轉譯螢幕上看得到的項目。ListItemSelectable
代表可選取的清單項目。combinedClickable
可同時處理點選和長按項目選取項目。點選會執行動作,而長按項目則會切換選取狀態。
結果
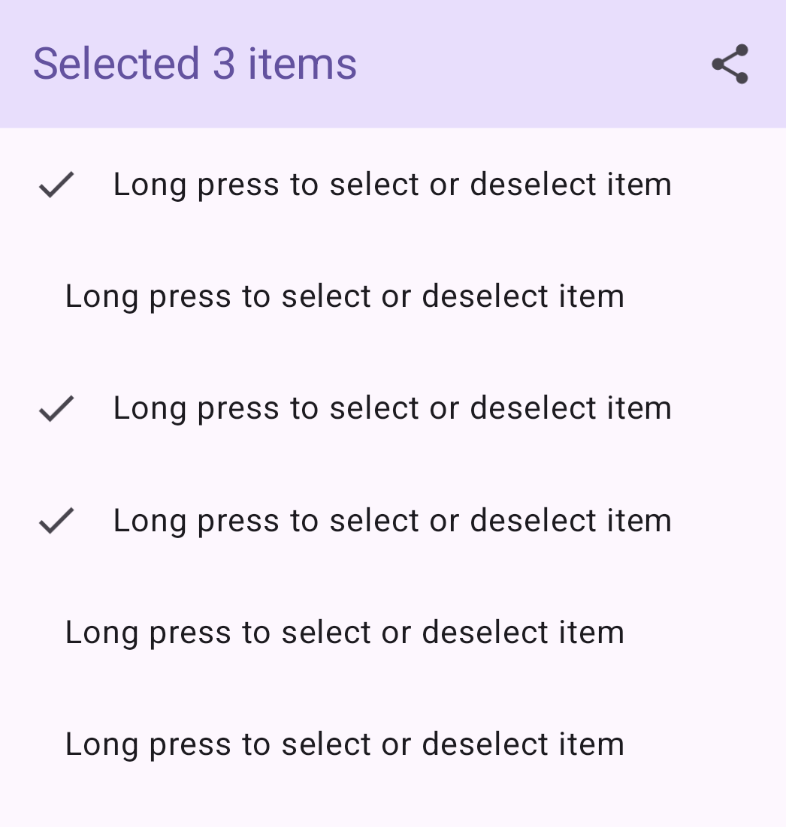