共用元素轉換功能可讓您在兩個內容一致的可組合項之間順暢轉換。這些元素通常用於導覽,可讓您在使用者瀏覽不同畫面時,以視覺方式連結這些畫面。
舉例來說,在下方影片中,您可以看到點心圖片和標題從清單頁面分享到詳細資料頁面。
Compose 中有幾個高階 API,可協助您建立共用元素:
SharedTransitionLayout
:實作共用元素轉場效果所需的邊界版面配置。並提供SharedTransitionScope
。可組合項必須位於SharedTransitionScope
中,才能使用共用元素修飾符。Modifier.sharedElement()
:修飾符會將應與其他可組合項比對的可組合項標示為SharedTransitionScope
。Modifier.sharedBounds()
:修飾符會向SharedTransitionScope
標示,這個可組合項的邊界應用於轉場作業的容器邊界。與sharedElement()
不同,sharedBounds()
是專為視覺上不同的內容而設計。
在 Compose 中建立共用元素時,重要的概念是如何與疊加和裁剪作業搭配運作。請參閱「裁剪和覆蓋圖」一節,進一步瞭解這個重要主題。
基本用法
本節將建立以下轉場效果,從較小的「list」項目轉換至較大的詳細項目:
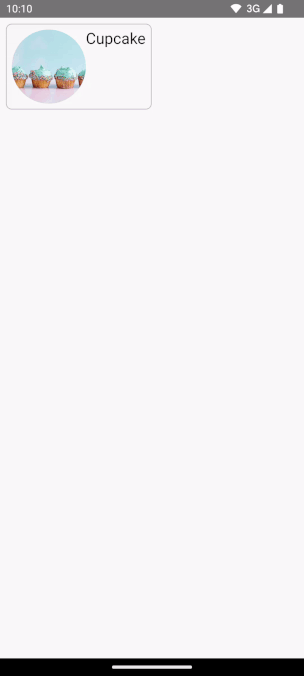
使用 Modifier.sharedElement()
的最佳方式是搭配 AnimatedContent
、AnimatedVisibility
或 NavHost
,這樣系統就能自動管理可組合函式之間的轉換。
起點是現有的基礎 AnimatedContent
,其中包含 MainContent
和 DetailsContent
可組合函式,然後再新增共用元素:
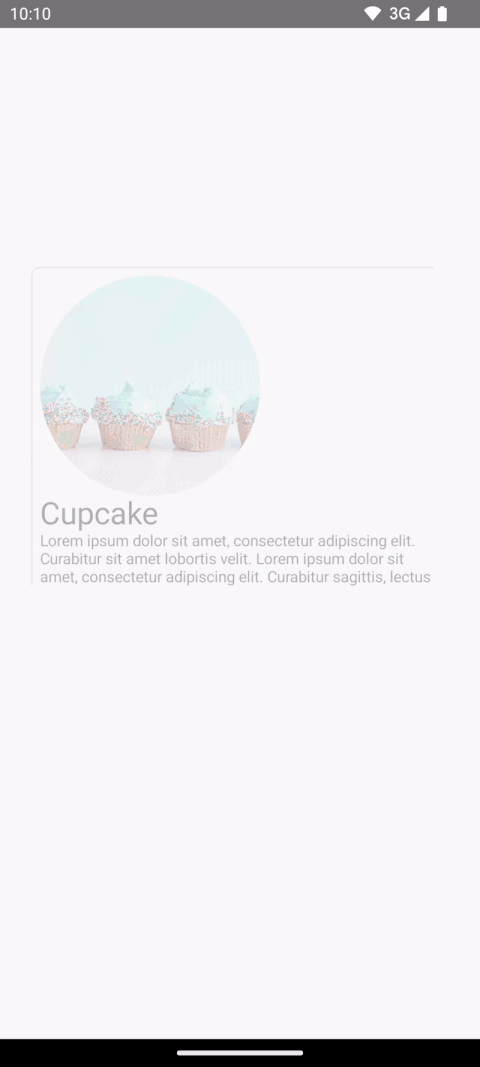
AnimatedContent
。如要讓共用元素在兩個版面配置之間顯示動畫,請將
AnimatedContent
可組合函式與SharedTransitionLayout
包圍。從SharedTransitionLayout
和AnimatedContent
傳遞的範圍會傳遞至MainContent
和DetailsContent
:var showDetails by remember { mutableStateOf(false) } SharedTransitionLayout { AnimatedContent( showDetails, label = "basic_transition" ) { targetState -> if (!targetState) { MainContent( onShowDetails = { showDetails = true }, animatedVisibilityScope = this@AnimatedContent, sharedTransitionScope = this@SharedTransitionLayout ) } else { DetailsContent( onBack = { showDetails = false }, animatedVisibilityScope = this@AnimatedContent, sharedTransitionScope = this@SharedTransitionLayout ) } } }
在兩個相符的可組合函式上,將
Modifier.sharedElement()
新增至可組合函式修飾符鏈結。建立SharedContentState
物件,並使用rememberSharedContentState()
記住該物件。SharedContentState
物件會儲存不重複的鍵,用來決定要共用的元素。提供專屬鍵來識別內容,並使用rememberSharedContentState()
來記住項目。AnimatedContentScope
會傳遞至輔助鍵,用於協調動畫。@Composable private fun MainContent( onShowDetails: () -> Unit, modifier: Modifier = Modifier, sharedTransitionScope: SharedTransitionScope, animatedVisibilityScope: AnimatedVisibilityScope ) { Row( // ... ) { with(sharedTransitionScope) { Image( painter = painterResource(id = R.drawable.cupcake), contentDescription = "Cupcake", modifier = Modifier .sharedElement( rememberSharedContentState(key = "image"), animatedVisibilityScope = animatedVisibilityScope ) .size(100.dp) .clip(CircleShape), contentScale = ContentScale.Crop ) // ... } } } @Composable private fun DetailsContent( modifier: Modifier = Modifier, onBack: () -> Unit, sharedTransitionScope: SharedTransitionScope, animatedVisibilityScope: AnimatedVisibilityScope ) { Column( // ... ) { with(sharedTransitionScope) { Image( painter = painterResource(id = R.drawable.cupcake), contentDescription = "Cupcake", modifier = Modifier .sharedElement( rememberSharedContentState(key = "image"), animatedVisibilityScope = animatedVisibilityScope ) .size(200.dp) .clip(CircleShape), contentScale = ContentScale.Crop ) // ... } } }
如要取得共用元素是否相符的資訊,請將 rememberSharedContentState()
擷取至變數,然後查詢 isMatchFound
。
這會產生以下自動動畫:
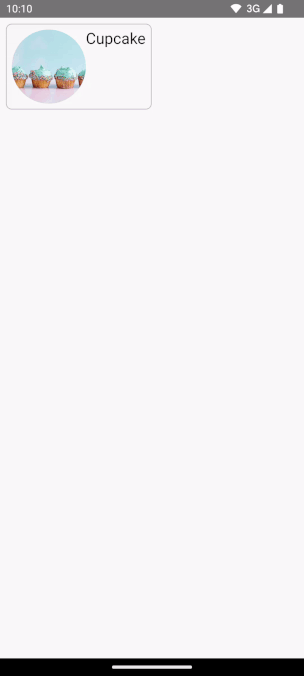
您可能會注意到,整個容器的背景顏色和大小仍使用預設的 AnimatedContent
設定。
共用邊界與共用元素
Modifier.sharedBounds()
與 Modifier.sharedElement()
類似。不過,修飾符有以下差異:
sharedBounds()
適用於視覺上不同的內容,但在狀態之間應共用相同的區域,而sharedElement()
則預期內容會相同。- 使用
sharedBounds()
時,在兩個狀態之間轉換期間,進入和離開畫面的內容會顯示在畫面上,但使用sharedElement()
時,只有目標內容會在轉換邊界中顯示。Modifier.sharedBounds()
有enter
和exit
參數,可用來指定內容的轉場方式,類似於AnimatedContent
的運作方式。 sharedBounds()
最常見的用途是容器轉換模式,而sharedElement()
的範例用途則是主畫面轉場。- 使用
Text
可組合函式時,建議使用sharedBounds()
支援字型變更,例如在斜體和粗體之間轉換,或變更顏色。
在前述範例中,如果在兩種不同情況下將 Modifier.sharedBounds()
新增至 Row
和 Column
,就能共用兩者的邊界並執行轉場動畫,讓兩者之間的邊界彼此擴大:
@Composable private fun MainContent( onShowDetails: () -> Unit, modifier: Modifier = Modifier, sharedTransitionScope: SharedTransitionScope, animatedVisibilityScope: AnimatedVisibilityScope ) { with(sharedTransitionScope) { Row( modifier = Modifier .padding(8.dp) .sharedBounds( rememberSharedContentState(key = "bounds"), animatedVisibilityScope = animatedVisibilityScope, enter = fadeIn(), exit = fadeOut(), resizeMode = SharedTransitionScope.ResizeMode.ScaleToBounds() ) // ... ) { // ... } } } @Composable private fun DetailsContent( modifier: Modifier = Modifier, onBack: () -> Unit, sharedTransitionScope: SharedTransitionScope, animatedVisibilityScope: AnimatedVisibilityScope ) { with(sharedTransitionScope) { Column( modifier = Modifier .padding(top = 200.dp, start = 16.dp, end = 16.dp) .sharedBounds( rememberSharedContentState(key = "bounds"), animatedVisibilityScope = animatedVisibilityScope, enter = fadeIn(), exit = fadeOut(), resizeMode = SharedTransitionScope.ResizeMode.ScaleToBounds() ) // ... ) { // ... } } }
瞭解範圍
如要使用 Modifier.sharedElement()
,可組合函式必須位於 SharedTransitionScope
中。SharedTransitionLayout
可組合函式會提供 SharedTransitionScope
。請務必將其放在 UI 階層中含有要共用元素的同一頂層位置。
一般來說,可組合項也應放置在 AnimatedVisibilityScope
中。除非您手動管理可見度,否則這項功能通常會透過使用 AnimatedContent
在可組合函式之間切換,或直接使用 AnimatedVisibility
,或是透過 NavHost
可組合函式提供。如要使用多個範圍,請在 CompositionLocal
中儲存所需範圍,使用 Kotlin 中的 context 接收器,或將範圍做為參數傳遞至函式。
如果您有需要追蹤的多個範圍,或深層巢狀階層,請使用 CompositionLocals
。CompositionLocal
可讓您選擇要儲存及使用的確切範圍。另一方面,如果您使用內容接收器,階層中的其他版面配置可能會意外覆寫所提供的範圍。舉例來說,如果您有多個巢狀 AnimatedContent
,範圍可能會遭到覆寫。
val LocalNavAnimatedVisibilityScope = compositionLocalOf<AnimatedVisibilityScope?> { null } val LocalSharedTransitionScope = compositionLocalOf<SharedTransitionScope?> { null } @Composable private fun SharedElementScope_CompositionLocal() { // An example of how to use composition locals to pass around the shared transition scope, far down your UI tree. // ... SharedTransitionLayout { CompositionLocalProvider( LocalSharedTransitionScope provides this ) { // This could also be your top-level NavHost as this provides an AnimatedContentScope AnimatedContent(state, label = "Top level AnimatedContent") { targetState -> CompositionLocalProvider(LocalNavAnimatedVisibilityScope provides this) { // Now we can access the scopes in any nested composables as follows: val sharedTransitionScope = LocalSharedTransitionScope.current ?: throw IllegalStateException("No SharedElementScope found") val animatedVisibilityScope = LocalNavAnimatedVisibilityScope.current ?: throw IllegalStateException("No AnimatedVisibility found") } // ... } } } }
或者,如果階層並未深層巢狀,您可以將範圍做為參數傳遞:
@Composable fun MainContent( animatedVisibilityScope: AnimatedVisibilityScope, sharedTransitionScope: SharedTransitionScope ) { } @Composable fun Details( animatedVisibilityScope: AnimatedVisibilityScope, sharedTransitionScope: SharedTransitionScope ) { }
與 AnimatedVisibility
共用的元素
先前的範例說明如何使用共用元素與 AnimatedContent
,但共用元素也適用於 AnimatedVisibility
。
舉例來說,在這個延遲格狀範例中,每個元素都會包裝在 AnimatedVisibility
中。點選項目時,內容會顯示視覺效果,從 UI 拉出至對話方塊類似的元件。
var selectedSnack by remember { mutableStateOf<Snack?>(null) } SharedTransitionLayout(modifier = Modifier.fillMaxSize()) { LazyColumn( // ... ) { items(listSnacks) { snack -> AnimatedVisibility( visible = snack != selectedSnack, enter = fadeIn() + scaleIn(), exit = fadeOut() + scaleOut(), modifier = Modifier.animateItem() ) { Box( modifier = Modifier .sharedBounds( sharedContentState = rememberSharedContentState(key = "${snack.name}-bounds"), // Using the scope provided by AnimatedVisibility animatedVisibilityScope = this, clipInOverlayDuringTransition = OverlayClip(shapeForSharedElement) ) .background(Color.White, shapeForSharedElement) .clip(shapeForSharedElement) ) { SnackContents( snack = snack, modifier = Modifier.sharedElement( sharedContentState = rememberSharedContentState(key = snack.name), animatedVisibilityScope = this@AnimatedVisibility ), onClick = { selectedSnack = snack } ) } } } } // Contains matching AnimatedContent with sharedBounds modifiers. SnackEditDetails( snack = selectedSnack, onConfirmClick = { selectedSnack = null } ) }
AnimatedVisibility
共用的元素。修飾符排序
對於 Modifier.sharedElement()
和 Modifier.sharedBounds()
,修飾符鏈結的順序 (與 Compose 的其餘部分一樣) 很重要。影響大小的修飾符放置位置不正確,可能會導致在共用元素比對期間發生視覺跳躍的情況。
舉例來說,如果您在兩個共用元素的不同位置放置邊框修飾符,動畫就會出現視覺差異。
var selectFirst by remember { mutableStateOf(true) } val key = remember { Any() } SharedTransitionLayout( Modifier .fillMaxSize() .padding(10.dp) .clickable { selectFirst = !selectFirst } ) { AnimatedContent(targetState = selectFirst, label = "AnimatedContent") { targetState -> if (targetState) { Box( Modifier .padding(12.dp) .sharedBounds( rememberSharedContentState(key = key), animatedVisibilityScope = this@AnimatedContent ) .border(2.dp, Color.Red) ) { Text( "Hello", fontSize = 20.sp ) } } else { Box( Modifier .offset(180.dp, 180.dp) .sharedBounds( rememberSharedContentState( key = key, ), animatedVisibilityScope = this@AnimatedContent ) .border(2.dp, Color.Red) // This padding is placed after sharedBounds, but it doesn't match the // other shared elements modifier order, resulting in visual jumps .padding(12.dp) ) { Text( "Hello", fontSize = 36.sp ) } } } }
相符的邊界 |
不相符的邊界:請注意,共用元素動畫需要調整為不正確的邊界,因此動畫會出現偏差 |
---|---|
在共用元素修飾符「前」使用的修飾符,會為共用元素修飾符提供限制條件,這些限制條件會用來衍生初始和目標邊界,以及後續的邊界動畫。
在共用元素修飾符之後使用的修飾符會使用先前的限制,測量及計算子項的目標大小。共用元素修飾符會建立一系列動畫約束條件,逐步將子項從初始大小轉換為目標大小。
例外狀況是,如果您在動畫中使用 resizeMode = ScaleToBounds()
,或在可組合項中使用 Modifier.skipToLookaheadSize()
。在這種情況下,Compose 會使用目標限制條件來安排子項,並使用比例因數執行動畫,而非變更版面配置大小。
不重複的鍵
使用複雜的共用元素時,建議您建立非字串的鍵,因為字串可能會出現比對錯誤。每個鍵都必須是唯一值,才能進行比對。舉例來說,Jetsnack 有以下共用元素:
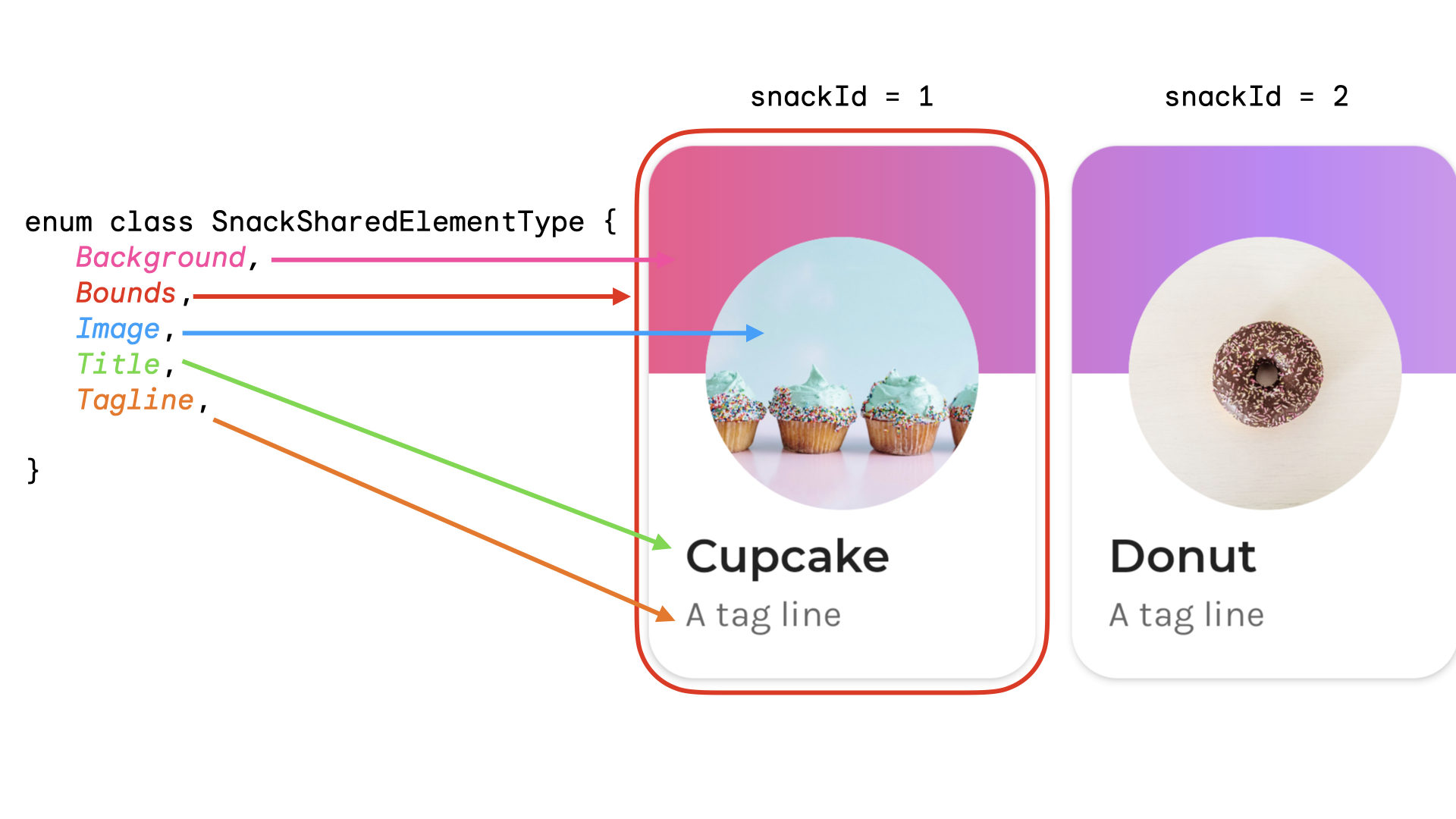
您可以建立列舉來代表共用元素類型。在這個範例中,整個小食資訊卡也可以出現在主畫面的多個不同位置,例如「熱門」和「推薦」區段。您可以建立包含 snackId
、origin
(「熱門」/「建議」) 和共用元素 type
的鍵,以便共用:
data class SnackSharedElementKey( val snackId: Long, val origin: String, val type: SnackSharedElementType ) enum class SnackSharedElementType { Bounds, Image, Title, Tagline, Background } @Composable fun SharedElementUniqueKey() { // ... Box( modifier = Modifier .sharedElement( rememberSharedContentState( key = SnackSharedElementKey( snackId = 1, origin = "latest", type = SnackSharedElementType.Image ) ), animatedVisibilityScope = this@AnimatedVisibility ) ) // ... }
建議使用資料類別做為鍵,因為這類類別會實作 hashCode()
和 isEquals()
。
手動管理共用元素的顯示情形
如果您不使用 AnimatedVisibility
或 AnimatedContent
,可以自行管理共用元素的顯示設定。使用 Modifier.sharedElementWithCallerManagedVisibility()
,並提供您自己的條件,決定項目應顯示或隱藏的時機:
var selectFirst by remember { mutableStateOf(true) } val key = remember { Any() } SharedTransitionLayout( Modifier .fillMaxSize() .padding(10.dp) .clickable { selectFirst = !selectFirst } ) { Box( Modifier .sharedElementWithCallerManagedVisibility( rememberSharedContentState(key = key), !selectFirst ) .background(Color.Red) .size(100.dp) ) { Text(if (!selectFirst) "false" else "true", color = Color.White) } Box( Modifier .offset(180.dp, 180.dp) .sharedElementWithCallerManagedVisibility( rememberSharedContentState( key = key, ), selectFirst ) .alpha(0.5f) .background(Color.Blue) .size(180.dp) ) { Text(if (selectFirst) "false" else "true", color = Color.White) } }
目前限制
這些 API 有一些限制。最值得注意的是:
- 不支援 View 和 Compose 之間的互通性。這包括任何包裝
AndroidView
的可組合函式,例如Dialog
。 - 以下項目不支援自動動畫:
- 共用圖片可組合項:
ContentScale
預設不會顯示動畫。並會對齊設定的結尾ContentScale
。
- 形狀裁剪:系統並未內建支援形狀之間的自動動畫,例如在項目轉換時,從方形轉為圓形的動畫。
- 對於不支援的情況,請使用
Modifier.sharedBounds()
而非sharedElement()
,並在項目上新增Modifier.animateEnterExit()
。
- 共用圖片可組合項: