本頁面將介紹如何使用不同的觸覺 API 建立自訂效果,讓 Android 應用程式不只提供標準的震動波形。
本頁面包含以下範例:
如需其他範例,請參閱「為事件新增觸覺回饋」,並隨時遵循觸覺回饋設計原則。
使用備用方案處理裝置相容性
實作任何自訂效果時,請考量以下事項:
- 特效所需的裝置功能
- 裝置無法播放效果時該怎麼辦
Android haptics API 參考資料詳細說明如何檢查 haptics 相關元件的支援情形,讓應用程式能夠提供一致的整體體驗。
視用途而定,您可能需要停用自訂特效,或根據不同的潛在功能提供其他自訂特效。
請為下列裝置功能的高階類別進行規劃:
如果您使用觸覺原始功能:支援自訂效果所需原始功能的裝置。(詳情請參閱下一節的「基本元素」)。
支援振幅控制的裝置。
支援基本震動功能 (開啟/關閉) 的裝置,也就是缺乏振幅控制功能的裝置。
如果應用程式的觸覺回饋效果選項適用於這些類別,則其觸覺回饋使用者體驗應可在任何個別裝置上保持一致。
使用觸覺回饋原語
Android 提供多種觸覺回饋原始元素,其振幅和頻率各有不同。您可以單獨使用一個原始物件,也可以結合多個原始物件,以獲得豐富的觸覺效果。
- 請使用 50 毫秒或更長的延遲時間,以便在兩個圖元之間產生可辨識的間隔,並盡可能考量圖元持續時間。
- 請使用差異比率為 1.4 以上,這樣才能更清楚看出強度差異。
使用 0.5、0.7 和 1.0 的比例,建立基本元素的低、中和高強度版本。
建立自訂震動模式
震動模式通常用於注意力觸覺回饋,例如通知和鈴聲。Vibrator
服務可播放長時間的震動模式,隨時間變更震動幅度。這類效果稱為波形。
波形效果通常是可感知的,但如果在安靜的環境中播放,突然出現的長時間震動可能會嚇到使用者。過快地提升至目標振幅也可能會產生可聽見的嗡嗡聲。設計波形圖案,以便平滑處理振幅轉換,創造漸增和漸減效果。
震動模式範例
以下各節將提供幾種震動模式範例:
增加曝光量模式
波形會以 VibrationEffect
表示,並包含三個參數:
- Timings:每個波形區段的時間長度陣列,以毫秒為單位。
- 振幅:第一個引數中指定的每個時間長度所需的振動振幅,以 0 到 255 的整數值表示,其中 0 代表振動器「關閉狀態」,255 則是裝置的最大振幅。
- 重複索引:第一個引數中指定陣列的索引,用於開始重複波形圖,如果只播放一次圖案,則為 -1。
以下是脈衝兩次的波形示例,脈衝之間的間隔為 350 毫秒。第一個脈衝是平滑地逐漸增加至最大振幅,第二個脈衝則是快速逐漸增加至最大振幅。停止在結尾的行為是由負重複索引值定義。
Kotlin
val timings: LongArray = longArrayOf(
50, 50, 50, 50, 50, 100, 350, 25, 25, 25, 25, 200)
val amplitudes: IntArray = intArrayOf(
33, 51, 75, 113, 170, 255, 0, 38, 62, 100, 160, 255)
val repeatIndex = -1 // Don't repeat.
vibrator.vibrate(VibrationEffect.createWaveform(
timings, amplitudes, repeatIndex))
Java
long[] timings = new long[] {
50, 50, 50, 50, 50, 100, 350, 25, 25, 25, 25, 200 };
int[] amplitudes = new int[] {
33, 51, 75, 113, 170, 255, 0, 38, 62, 100, 160, 255 };
int repeatIndex = -1; // Don't repeat.
vibrator.vibrate(VibrationEffect.createWaveform(
timings, amplitudes, repeatIndex));
重複圖案
您也可以重複播放波形,直到取消為止。如要建立重複的波形,請設定非負 repeat
參數。播放重複的波形時,震動會持續進行,直到服務中明確取消為止:
Kotlin
void startVibrating() {
val timings: LongArray = longArrayOf(50, 50, 100, 50, 50)
val amplitudes: IntArray = intArrayOf(64, 128, 255, 128, 64)
val repeat = 1 // Repeat from the second entry, index = 1.
VibrationEffect repeatingEffect = VibrationEffect.createWaveform(
timings, amplitudes, repeat)
// repeatingEffect can be used in multiple places.
vibrator.vibrate(repeatingEffect)
}
void stopVibrating() {
vibrator.cancel()
}
Java
void startVibrating() {
long[] timings = new long[] { 50, 50, 100, 50, 50 };
int[] amplitudes = new int[] { 64, 128, 255, 128, 64 };
int repeat = 1; // Repeat from the second entry, index = 1.
VibrationEffect repeatingEffect = VibrationEffect.createWaveform(
timings, amplitudes, repeat);
// repeatingEffect can be used in multiple places.
vibrator.vibrate(repeatingEffect);
}
void stopVibrating() {
vibrator.cancel();
}
這對於需要使用者確認的間歇性事件非常實用。這類事件的例子包括來電和觸發的鬧鐘。
含備用模式的模式
控制震動振幅是硬體相關功能。在沒有這項功能的低階裝置上播放波形時,裝置會以振幅陣列中每個正值項目的最大振幅震動。如果您的應用程式需要支援這類裝置,請使用在該情況下不會產生嗡嗡聲的模式,或是設計可做為備用的簡單開/關模式。
Kotlin
if (vibrator.hasAmplitudeControl()) {
vibrator.vibrate(VibrationEffect.createWaveform(
smoothTimings, amplitudes, smoothRepeatIdx))
} else {
vibrator.vibrate(VibrationEffect.createWaveform(
onOffTimings, onOffRepeatIdx))
}
Java
if (vibrator.hasAmplitudeControl()) {
vibrator.vibrate(VibrationEffect.createWaveform(
smoothTimings, amplitudes, smoothRepeatIdx));
} else {
vibrator.vibrate(VibrationEffect.createWaveform(
onOffTimings, onOffRepeatIdx));
}
建立震動組合
本節將說明如何將震動組合成更長、更複雜的自訂效果,並進一步探索如何使用更先進的硬體功能,體驗豐富的觸覺回饋。您可以使用不同振幅和頻率的效果組合,在具備頻率頻寬較寬的觸覺致動器的裝置上,創造更複雜的觸覺效果。
如前文所述,建立自訂振動模式的程序說明瞭如何控制振動幅度,以便產生平滑的升降效果。豐富的觸覺回饋功能則是透過探索裝置震動器的更廣頻率範圍,進一步強化這項概念,讓效果更流暢。這些波形特別適合用來創造漸強或漸弱效果。
本頁稍早所述的組合原語元素是由裝置製造商實作。這些震動會提供清晰、短促且悅耳的震動,符合觸覺回饋原則,可提供清晰的觸覺回饋。如要進一步瞭解這些功能及其運作方式,請參閱「震動致動器入門指南」。
Android 不會為含有不支援的元素的組合提供備用方案。因此,請執行下列步驟:
啟用進階觸覺回饋功能前,請確認特定裝置支援您使用的所有基本元素。
停用系統不支援的一致體驗組合,而非僅停用缺少原始元素的效果。
如要進一步瞭解如何確認裝置支援狀況,請參閱下列章節。
建立合成的震動效果
您可以使用 VibrationEffect.Composition
建立組合振動效果。以下是慢慢升起效果,接著是銳利的點擊效果的範例:
Kotlin
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SLOW_RISE
).addPrimitive(
VibrationEffect.Composition.PRIMITIVE_CLICK
).compose()
)
Java
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_SLOW_RISE)
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_CLICK)
.compose());
您可以新增要依序播放的原始元素,建立組合。每個原始元素也都可調整,因此您可以控制每個原始元素產生的振動幅度。比例定義為介於 0 和 1 之間的值,其中 0 實際對應至最小振幅,使用者幾乎無法察覺此原始元素。
在震動原型中建立變化版本
如果您想為同一個原始物件建立弱和強版本,請建立強度比率為 1.4 以上的版本,這樣一來就能輕易分辨強度差異。請勿嘗試為相同的圖元建立超過三個強度層級,因為這些層級在感知上並無差異。例如,使用 0.5、0.7 和 1.0 的比例,建立基本圖形的低、中和高強度版本。
在震動原語之間加入間隔
組合也能指定要新增的連續原始元素間延遲時間。這項延遲時間會以毫秒為單位,自上一個基本元素結束後開始計算。一般來說,兩個原始元素之間的 5 到 10 毫秒差距太短,無法偵測。如要讓兩個圖元之間有明顯的間隔,請使用 50 毫秒或更長的間隔。以下是含有延遲的組合範例:
Kotlin
val delayMs = 100
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SPIN, 0.8f
).addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SPIN, 0.6f
).addPrimitive(
VibrationEffect.Composition.PRIMITIVE_THUD, 1.0f, delayMs
).compose()
)
Java
int delayMs = 100;
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_SPIN, 0.8f)
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_SPIN, 0.6f)
.addPrimitive(
VibrationEffect.Composition.PRIMITIVE_THUD, 1.0f, delayMs)
.compose());
查看系統支援哪些基本元素
您可以使用下列 API 驗證裝置是否支援特定原始元素:
Kotlin
val primitive = VibrationEffect.Composition.PRIMITIVE_LOW_TICK
if (vibrator.areAllPrimitivesSupported(primitive)) {
vibrator.vibrate(VibrationEffect.startComposition()
.addPrimitive(primitive).compose())
} else {
// Play a predefined effect or custom pattern as a fallback.
}
Java
int primitive = VibrationEffect.Composition.PRIMITIVE_LOW_TICK;
if (vibrator.areAllPrimitivesSupported(primitive)) {
vibrator.vibrate(VibrationEffect.startComposition()
.addPrimitive(primitive).compose());
} else {
// Play a predefined effect or custom pattern as a fallback.
}
您也可以檢查多個原始元素,然後根據裝置支援等級決定要組合哪些原始元素:
Kotlin
val effects: IntArray = intArrayOf(
VibrationEffect.Composition.PRIMITIVE_LOW_TICK,
VibrationEffect.Composition.PRIMITIVE_TICK,
VibrationEffect.Composition.PRIMITIVE_CLICK
)
val supported: BooleanArray = vibrator.arePrimitivesSupported(primitives)
Java
int[] primitives = new int[] {
VibrationEffect.Composition.PRIMITIVE_LOW_TICK,
VibrationEffect.Composition.PRIMITIVE_TICK,
VibrationEffect.Composition.PRIMITIVE_CLICK
};
boolean[] supported = vibrator.arePrimitivesSupported(effects);
振動組合示例
以下各節提供幾個振動組合範例,這些範例取自 GitHub 上的觸覺回饋範例應用程式。
電阻 (低電流)
您可以控制原始震動的振幅,向進行中的動作傳達實用的回饋。您可以使用密集的縮放值,為基本圖形建立平滑的漸強效果。您也可以根據使用者互動,動態設定連續原語法之間的延遲時間。以下範例說明瞭這個概念,其中的檢視畫面動畫是由拖曳手勢控制,並透過觸覺回饋增強。
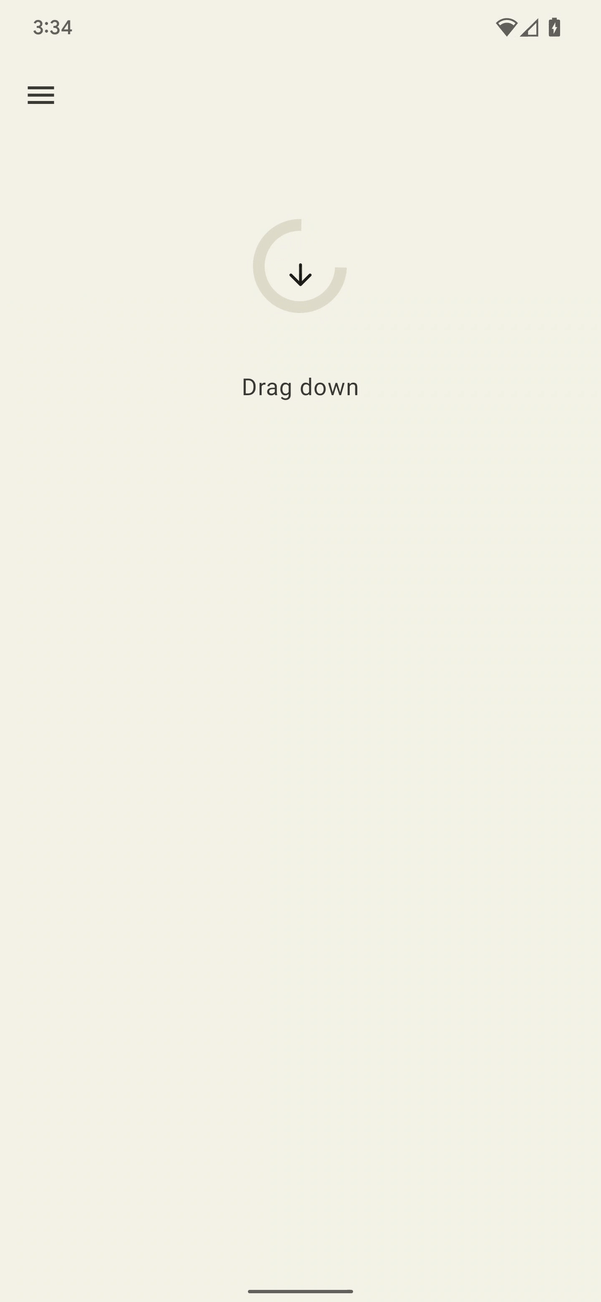
圖 1. 這張波形圖代表裝置上震動輸出的加速度。
Kotlin
@Composable
fun ResistScreen() {
// Control variables for the dragging of the indicator.
var isDragging by remember { mutableStateOf(false) }
var dragOffset by remember { mutableStateOf(0f) }
// Only vibrates while the user is dragging
if (isDragging) {
LaunchedEffect(Unit) {
// Continuously run the effect for vibration to occur even when the view
// is not being drawn, when user stops dragging midway through gesture.
while (true) {
// Calculate the interval inversely proportional to the drag offset.
val vibrationInterval = calculateVibrationInterval(dragOffset)
// Calculate the scale directly proportional to the drag offset.
val vibrationScale = calculateVibrationScale(dragOffset)
delay(vibrationInterval)
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_LOW_TICK,
vibrationScale
).compose()
)
}
}
}
Screen() {
Column(
Modifier
.draggable(
orientation = Orientation.Vertical,
onDragStarted = {
isDragging = true
},
onDragStopped = {
isDragging = false
},
state = rememberDraggableState { delta ->
dragOffset += delta
}
)
) {
// Build the indicator UI based on how much the user has dragged it.
ResistIndicator(dragOffset)
}
}
}
Java
class DragListener implements View.OnTouchListener {
// Control variables for the dragging of the indicator.
private int startY;
private int vibrationInterval;
private float vibrationScale;
@Override
public boolean onTouch(View view, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
startY = event.getRawY();
vibrationInterval = calculateVibrationInterval(0);
vibrationScale = calculateVibrationScale(0);
startVibration();
break;
case MotionEvent.ACTION_MOVE:
float dragOffset = event.getRawY() - startY;
// Calculate the interval inversely proportional to the drag offset.
vibrationInterval = calculateVibrationInterval(dragOffset);
// Calculate the scale directly proportional to the drag offset.
vibrationScale = calculateVibrationScale(dragOffset);
// Build the indicator UI based on how much the user has dragged it.
updateIndicator(dragOffset);
break;
case MotionEvent.ACTION_CANCEL:
case MotionEvent.ACTION_UP:
// Only vibrates while the user is dragging
cancelVibration();
break;
}
return true;
}
private void startVibration() {
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_LOW_TICK,
vibrationScale)
.compose());
// Continuously run the effect for vibration to occur even when the view
// is not being drawn, when user stops dragging midway through gesture.
handler.postDelayed(this::startVibration, vibrationInterval);
}
private void cancelVibration() {
handler.removeCallbacksAndMessages(null);
}
}
展開 (包含上升和下降)
有兩個原始碼可用來提高感知到的震動強度:PRIMITIVE_QUICK_RISE
和 PRIMITIVE_SLOW_RISE
。兩者都會觸及相同的目標,但時間長度不同。只有一個基本類型可用於降速,即 PRIMITIVE_QUICK_FALL
。這些基本元素搭配使用時,效果會更佳,可建立波形區段,讓波形強度逐漸增加,然後消失。您可以對齊經過調整大小的基本元素,避免兩者之間的振幅突然跳躍,這麼做也能延長整體效果的時間長度。在感知上,使用者總是比較注意上升部分,而非下降部分,因此讓上升部分比下降部分短,可以將重點轉移至下降部分。
以下是應用此組合來展開和收合圓形的範例。升起效果可強化動畫期間的擴展感。結合上升和下降效果,有助於強調動畫結尾的收合效果。
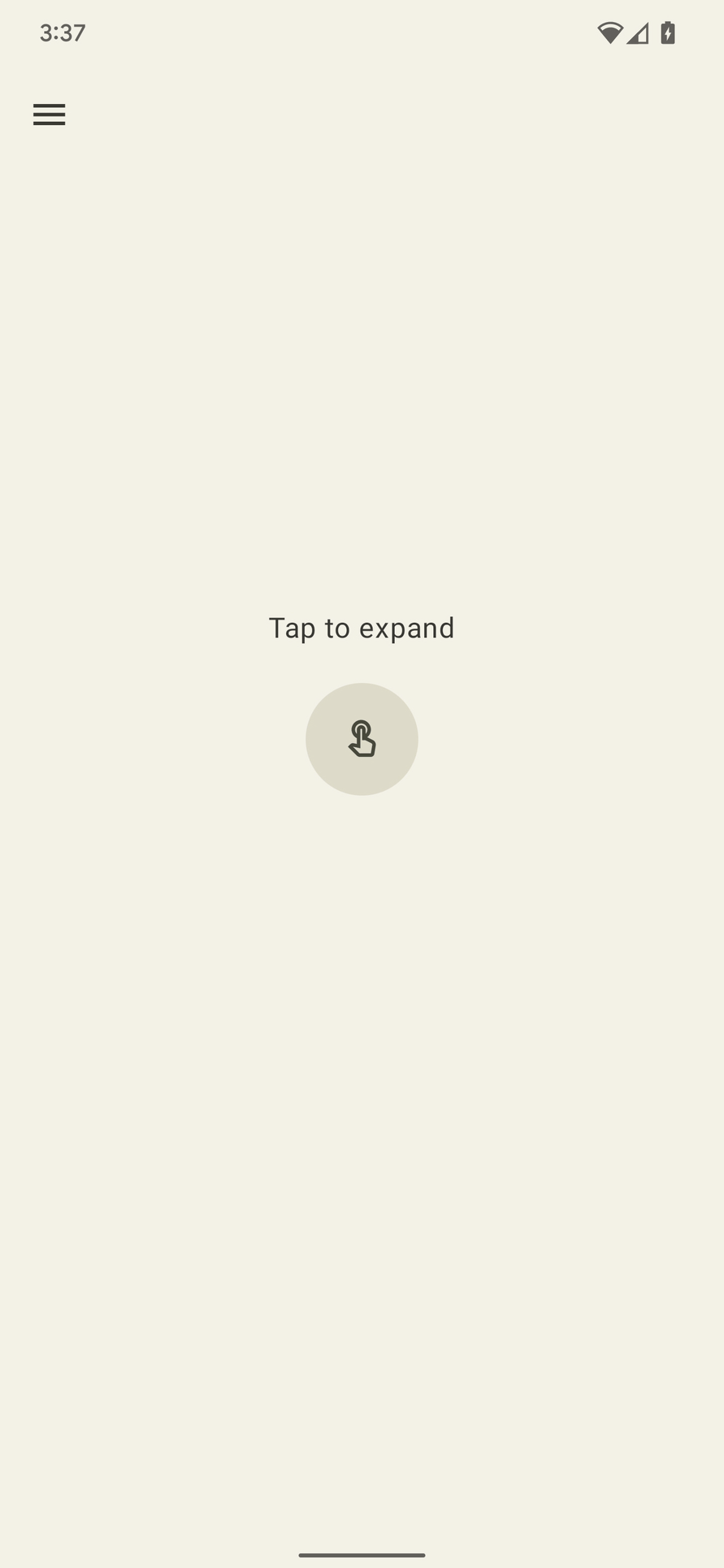
圖 2.這張波形圖代表裝置上振動輸出的加速度。
Kotlin
enum class ExpandShapeState {
Collapsed,
Expanded
}
@Composable
fun ExpandScreen() {
// Control variable for the state of the indicator.
var currentState by remember { mutableStateOf(ExpandShapeState.Collapsed) }
// Animation between expanded and collapsed states.
val transitionData = updateTransitionData(currentState)
Screen() {
Column(
Modifier
.clickable(
{
if (currentState == ExpandShapeState.Collapsed) {
currentState = ExpandShapeState.Expanded
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SLOW_RISE,
0.3f
).addPrimitive(
VibrationEffect.Composition.PRIMITIVE_QUICK_FALL,
0.3f
).compose()
)
} else {
currentState = ExpandShapeState.Collapsed
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SLOW_RISE
).compose()
)
}
)
) {
// Build the indicator UI based on the current state.
ExpandIndicator(transitionData)
}
}
}
Java
class ClickListener implements View.OnClickListener {
private final Animation expandAnimation;
private final Animation collapseAnimation;
private boolean isExpanded;
ClickListener(Context context) {
expandAnimation = AnimationUtils.loadAnimation(context, R.anim.expand);
expandAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SLOW_RISE, 0.3f)
.addPrimitive(
VibrationEffect.Composition.PRIMITIVE_QUICK_FALL, 0.3f)
.compose());
}
});
collapseAnimation = AnimationUtils
.loadAnimation(context, R.anim.collapse);
collapseAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SLOW_RISE)
.compose());
}
});
}
@Override
public void onClick(View view) {
view.startAnimation(isExpanded ? collapseAnimation : expandAnimation);
isExpanded = !isExpanded;
}
}
擺動 (旋轉)
觸覺技術原則之一就是要讓使用者感到愉悅。如要帶來令人驚喜的振動效果,不妨使用 PRIMITIVE_SPIN
。這個原始元素最適合多次呼叫。將多個旋轉連接起來,可能會產生搖晃和不穩定的效果,您可以對每個基本圖形套用某種隨機縮放比例,進一步強化效果。您也可以嘗試調整連續旋轉基本元素之間的間距。兩次旋轉之間沒有任何間隔 (0 毫秒),會造成緊密旋轉的感受。將旋轉間隔從 10 毫秒增加至 50 毫秒,可產生較鬆散的旋轉效果,並可用於配合影片或動畫的時間長度。
請勿使用超過 100 毫秒的間隔,因為連續旋轉的效果會變得無法整合,並開始讓人感覺像是個別特效。
以下是彈性形狀的範例,當您將其向下拖曳並放開時,形狀會彈回。動畫會搭配一組旋轉效果,以不同的強度播放,強度與彈跳位移成正比。
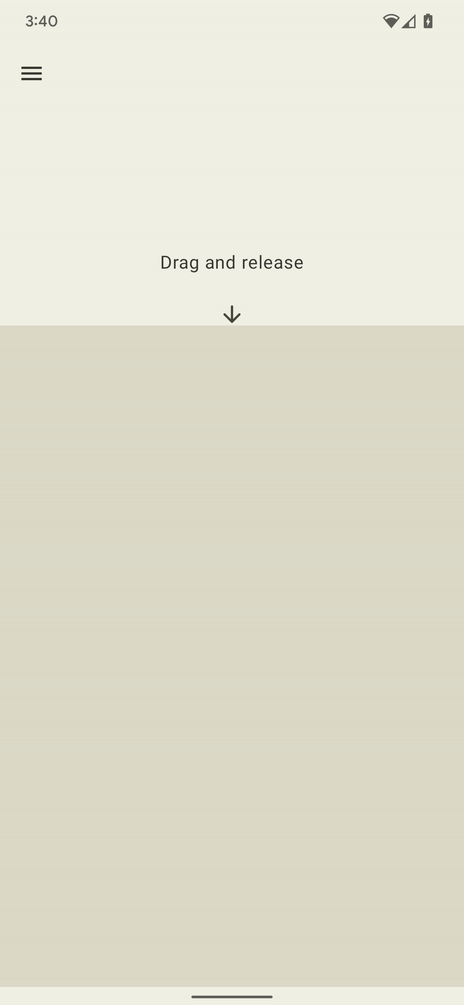
圖 3. 這張波形圖代表裝置上震動輸出的加速度。
Kotlin
@Composable
fun WobbleScreen() {
// Control variables for the dragging and animating state of the elastic.
var dragDistance by remember { mutableStateOf(0f) }
var isWobbling by remember { mutableStateOf(false) }
// Use drag distance to create an animated float value behaving like a spring.
val dragDistanceAnimated by animateFloatAsState(
targetValue = if (dragDistance > 0f) dragDistance else 0f,
animationSpec = spring(
dampingRatio = Spring.DampingRatioHighBouncy,
stiffness = Spring.StiffnessMedium
),
)
if (isWobbling) {
LaunchedEffect(Unit) {
while (true) {
val displacement = dragDistanceAnimated / MAX_DRAG_DISTANCE
// Use some sort of minimum displacement so the final few frames
// of animation don't generate a vibration.
if (displacement > SPIN_MIN_DISPLACEMENT) {
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SPIN,
nextSpinScale(displacement)
).addPrimitive(
VibrationEffect.Composition.PRIMITIVE_SPIN,
nextSpinScale(displacement)
).compose()
)
}
// Delay the next check for a sufficient duration until the
// current composition finishes. Note that you can use
// Vibrator.getPrimitiveDurations API to calculcate the delay.
delay(VIBRATION_DURATION)
}
}
}
Box(
Modifier
.fillMaxSize()
.draggable(
onDragStopped = {
isWobbling = true
dragDistance = 0f
},
orientation = Orientation.Vertical,
state = rememberDraggableState { delta ->
isWobbling = false
dragDistance += delta
}
)
) {
// Draw the wobbling shape using the animated spring-like value.
WobbleShape(dragDistanceAnimated)
}
}
// Calculate a random scale for each spin to vary the full effect.
fun nextSpinScale(displacement: Float): Float {
// Generate a random offset in the range [-0.1, +0.1] to be added to the
// vibration scale so the spin effects have slightly different values.
val randomOffset: Float = Random.Default.nextFloat() * 0.2f - 0.1f
return (displacement + randomOffset).absoluteValue.coerceIn(0f, 1f)
}
Java
class AnimationListener implements DynamicAnimation.OnAnimationUpdateListener {
private final Random vibrationRandom = new Random(seed);
private final long lastVibrationUptime;
@Override
public void onAnimationUpdate(
DynamicAnimation animation, float value, float velocity) {
// Delay the next check for a sufficient duration until the current
// composition finishes. Note that you can use
// Vibrator.getPrimitiveDurations API to calculcate the delay.
if (SystemClock.uptimeMillis() - lastVibrationUptime < VIBRATION_DURATION) {
return;
}
float displacement = calculateRelativeDisplacement(value);
// Use some sort of minimum displacement so the final few frames
// of animation don't generate a vibration.
if (displacement < SPIN_MIN_DISPLACEMENT) {
return;
}
lastVibrationUptime = SystemClock.uptimeMillis();
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_SPIN,
nextSpinScale(displacement))
.addPrimitive(VibrationEffect.Composition.PRIMITIVE_SPIN,
nextSpinScale(displacement))
.compose());
}
// Calculate a random scale for each spin to vary the full effect.
float nextSpinScale(float displacement) {
// Generate a random offset in the range [-0.1,+0.1] to be added to
// the vibration scale so the spin effects have slightly different
// values.
float randomOffset = vibrationRandom.nextFloat() * 0.2f - 0.1f
return MathUtils.clamp(displacement + randomOffset, 0f, 1f)
}
}
彈跳 (含 thud)
振動效果的另一個進階用途是模擬物理互動。PRIMITIVE_THUD
可產生強烈的迴盪效果,並搭配視覺效果 (例如影片或動畫中的撞擊聲),提升整體體驗。
以下是球落下動畫的範例,每次球彈跳至畫面底部時,都會播放 thud 效果:
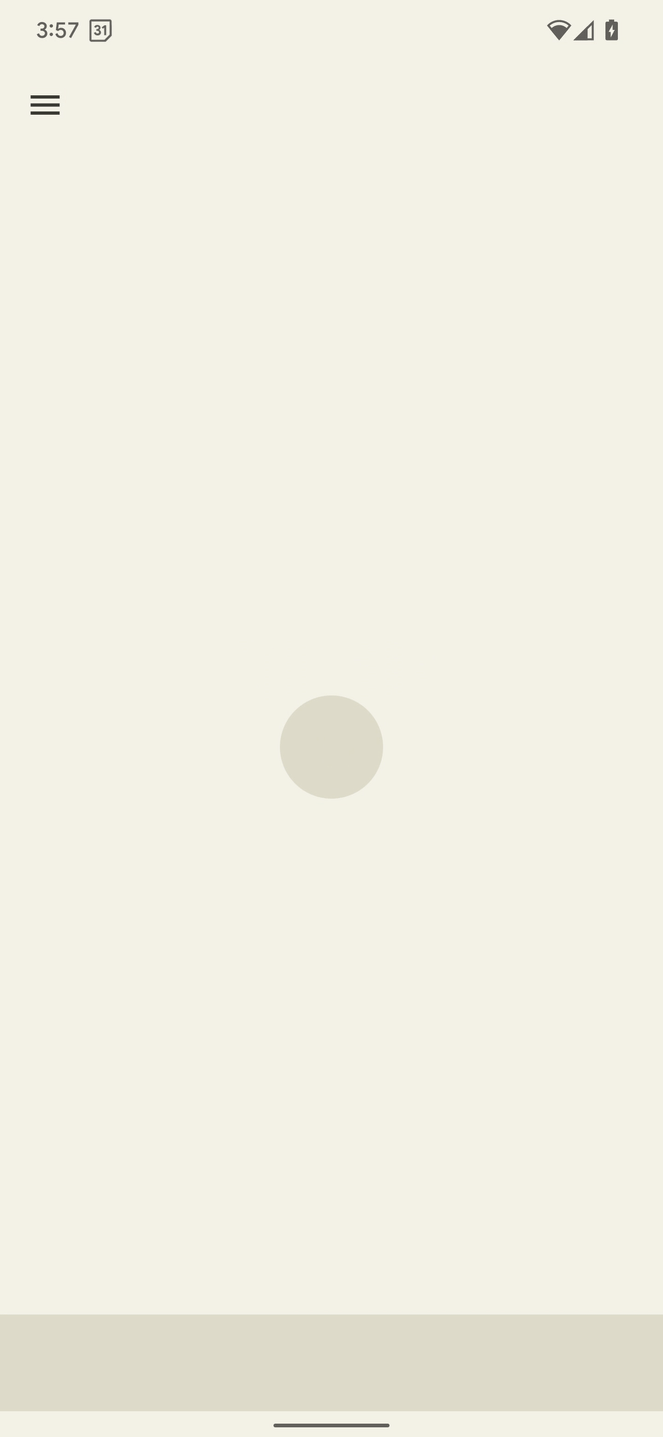
圖 4. 這張波形圖代表裝置上震動輸出的加速度。
Kotlin
enum class BallPosition {
Start,
End
}
@Composable
fun BounceScreen() {
// Control variable for the state of the ball.
var ballPosition by remember { mutableStateOf(BallPosition.Start) }
var bounceCount by remember { mutableStateOf(0) }
// Animation for the bouncing ball.
var transitionData = updateTransitionData(ballPosition)
val collisionData = updateCollisionData(transitionData)
// Ball is about to contact floor, only vibrating once per collision.
var hasVibratedForBallContact by remember { mutableStateOf(false) }
if (collisionData.collisionWithFloor) {
if (!hasVibratedForBallContact) {
val vibrationScale = 0.7.pow(bounceCount++).toFloat()
vibrator.vibrate(
VibrationEffect.startComposition().addPrimitive(
VibrationEffect.Composition.PRIMITIVE_THUD,
vibrationScale
).compose()
)
hasVibratedForBallContact = true
}
} else {
// Reset for next contact with floor.
hasVibratedForBallContact = false
}
Screen() {
Box(
Modifier
.fillMaxSize()
.clickable {
if (transitionData.isAtStart) {
ballPosition = BallPosition.End
} else {
ballPosition = BallPosition.Start
bounceCount = 0
}
},
) {
// Build the ball UI based on the current state.
BouncingBall(transitionData)
}
}
}
Java
class ClickListener implements View.OnClickListener {
@Override
public void onClick(View view) {
view.animate()
.translationY(targetY)
.setDuration(3000)
.setInterpolator(new BounceInterpolator())
.setUpdateListener(new AnimatorUpdateListener() {
boolean hasVibratedForBallContact = false;
int bounceCount = 0;
@Override
public void onAnimationUpdate(ValueAnimator animator) {
boolean valueBeyondThreshold = (float) animator.getAnimatedValue() > 0.98;
if (valueBeyondThreshold) {
if (!hasVibratedForBallContact) {
float vibrationScale = (float) Math.pow(0.7, bounceCount++);
vibrator.vibrate(
VibrationEffect.startComposition()
.addPrimitive(
VibrationEffect.Composition.PRIMITIVE_THUD,
vibrationScale)
.compose());
hasVibratedForBallContact = true;
}
} else {
// Reset for next contact with floor.
hasVibratedForBallContact = false;
}
}
});
}
}