Android 14 (API level 34) and higher enable users to write into any text input
field in any app using a stylus. Android text entry fields, including
EditText
components and
WebView
text widgets, support
stylus input by default.
However, if your app requires custom text input fields (see Custom text editors) or has a complex layout with text entry fields overlaying a drawing surface, you'll need to customize your app.
EditText
Stylus handwriting is enabled for all EditText
fields by default on Android 14
and higher. Handwriting mode is started for an EditText
when a stylus motion
event is detected within the handwriting bounds of the view.
The handwriting bounds include 40 dp of vertical padding and 10 dp of horizontal
padding around the view. Adjust the handwriting bounds with
setHandwritingBoundsOffsets()
.
Disable handwriting with
setAutoHandwritingEnabled(false)
.
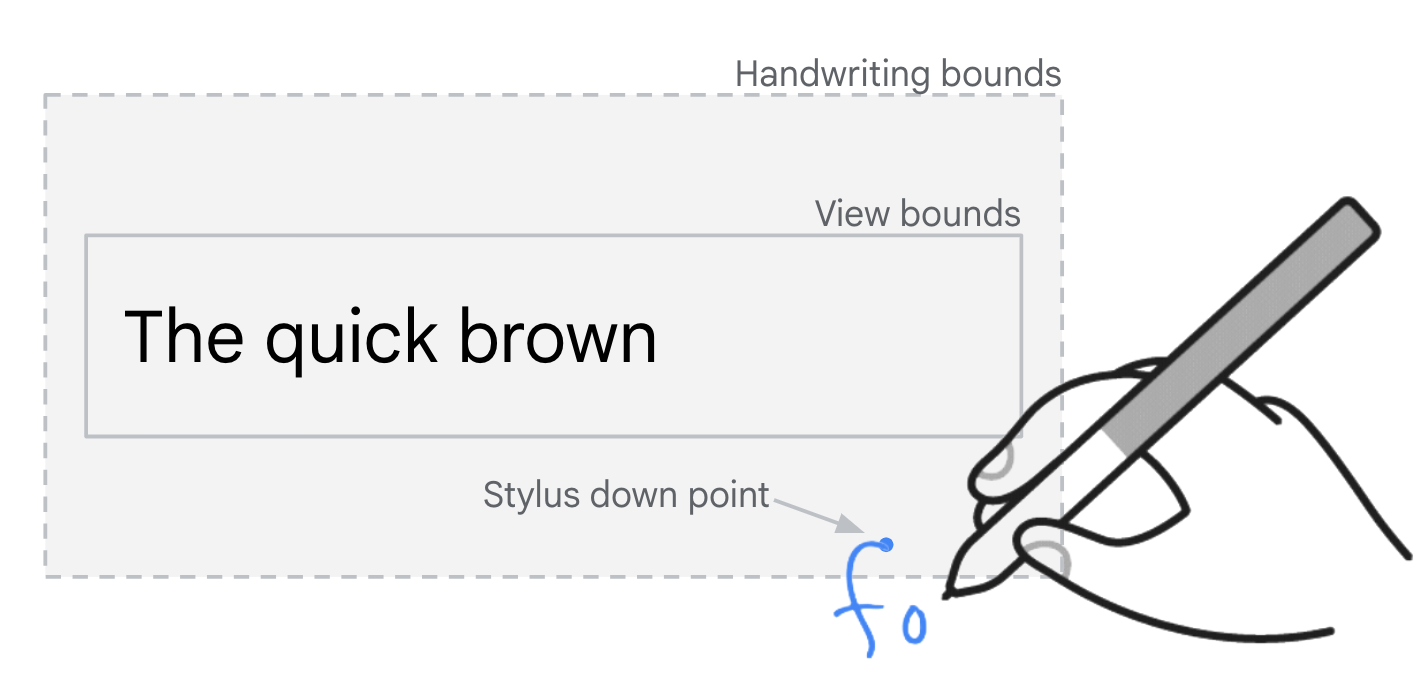
EditText
fields.
Input delegation
Apps can display placeholder UI elements that appear to be text input fields but are actually just static UI elements with no text input capability. Search fields are a common example. Tapping the static UI element triggers a transition to a new UI that contains a functional text input field focused for input.
Stylus input delegation
Use the handwriting delegation APIs to support stylus handwriting input for
placeholder input fields (see
setHandwritingDelegatorCallback()
and
setIsHandwritingDelegate()
).
The placeholder UI element is configured to delegate handwriting to a functional
input field, for example:
Kotlin
if (Build.VERSION.SDK_INT >= 34) { placeholderInputField.setHandwritingDelegatorCallback { showAndFocusDelegateInputField() } delegateInputField.setIsHandwritingDelegate(true) }
Java
if (Build.VERSION.SDK_INT >= 34) { placeholderInputField.setHandwritingDelegatorCallback(this::showAndFocusInputFieldDelegate); delegateInputField.setIsHandwritingDelegate(true); }
Stylus motion over the placeholder text input field view invokes the callback.
The callback triggers the UI transition to show and focus the functional input
field. The callback implementation is typically the same as the implementation
for a click listener on the placeholder element. When the functional input field
creates an
InputConnection
,
stylus handwriting mode starts.
Material Design
The
com.google.android.material.search
library provides the
SearchBar
and
SearchView
classes
to facilitate implementation of the placeholder UI pattern.
Placeholder and functional search views are linked with
setUpWithSearchBar()
.
Handwriting delegation is configured in the Material library with no additional development required in your app.
Overlap with drawing surfaces
If your app has a drawing surface with a text field overlaying the surface, you
may need to disable stylus handwriting to allow the user to draw. See
setAutoHandwritingEnabled()
.
Testing
Stylus handwriting is supported on Android 14 and higher devices with a compatible stylus input device and an input method editor (IME) that supports the Android 14 stylus handwriting APIs.
If you don't have a stylus input device, simulate stylus input on any device with root access (including emulators) using the following Android Debug Bridge (adb) commands:
// Android 14
adb shell setprop persist.debug.input.simulate_stylus_with_touch true && adb shell stop && adb shell start
// Android 15 and higher
// Property takes effect after screen reconfiguration such as orientation change.
adb shell setprop debug.input.simulate_stylus_with_touch true
Use the Gboard beta for testing if you are using a device that doesn't support stylus.
Additional resources
- Material Design — Text fields
- Custom text editors