基本通知通常包含標題、一行文字,以及 回應中的應用程式如要提供更多資訊,您可以將大型 可展開通知,方法是將其中一個通知範本套用於 。
首先,請建構一則通知,其中包含
建立通知。接著:
通話
setStyle()
敬上
,並提供每個範本對應的資訊,
如以下範例所示
新增大型圖片
如要在通知中新增圖像,請傳送
NotificationCompat.BigPictureStyle
敬上
至 setStyle()
。
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setStyle(NotificationCompat.BigPictureStyle() .bigPicture(myBitmap)) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(myBitmap)) .build();
如何讓通知只在通知出現時以縮圖顯示
如下圖所示
setLargeIcon()
敬上
再將圖片傳送給客戶接著,呼叫
BigPictureStyle.bigLargeIcon()
敬上
並傳遞 null
,這樣大型圖示就會在通知
已展開:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setLargeIcon(myBitmap) .setStyle(NotificationCompat.BigPictureStyle() .bigPicture(myBitmap) .bigLargeIcon(null)) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setLargeIcon(myBitmap) .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(myBitmap) .bigLargeIcon(null)) .build();
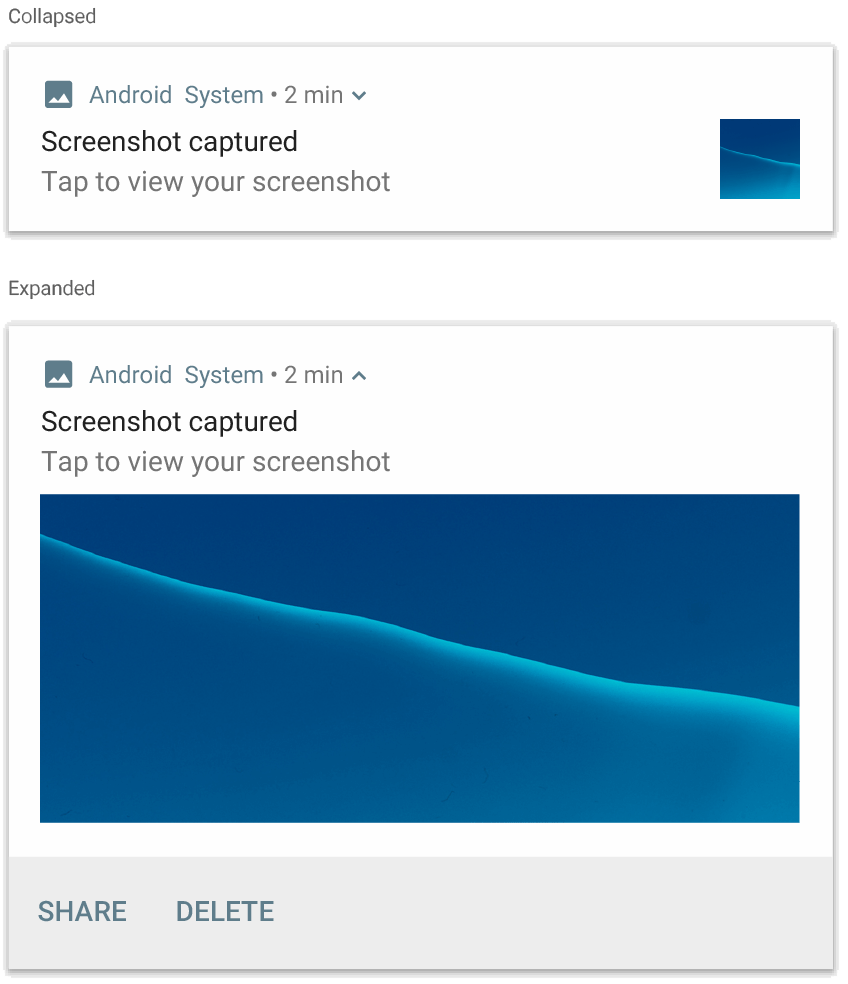
NotificationCompat.BigPictureStyle
。
新增一大段文字
套用
NotificationCompat.BigTextStyle
敬上
在通知的展開內容區域中顯示文字:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_mail) .setContentTitle(emailObject.getSenderName()) .setContentText(emailObject.getSubject()) .setLargeIcon(emailObject.getSenderAvatar()) .setStyle(NotificationCompat.BigTextStyle() .bigText(emailObject.getSubjectAndSnippet())) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_mail) .setContentTitle(emailObject.getSenderName()) .setContentText(emailObject.getSubject()) .setLargeIcon(emailObject.getSenderAvatar()) .setStyle(new NotificationCompat.BigTextStyle() .bigText(emailObject.getSubjectAndSnippet())) .build();
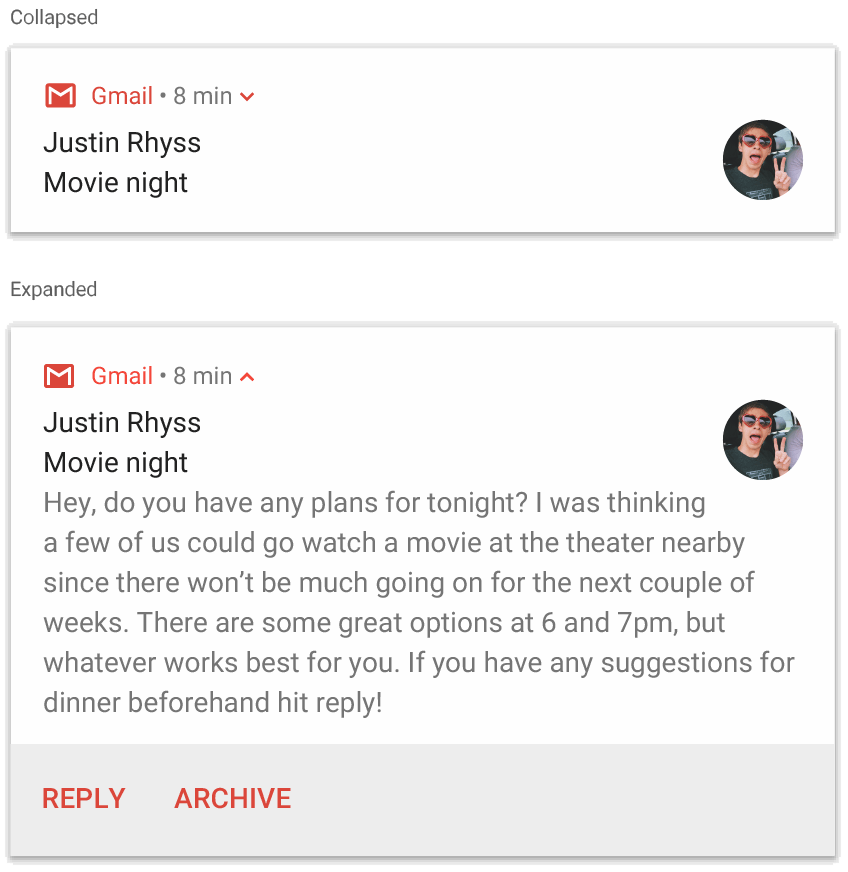
NotificationCompat.BigTextStyle
。
建立收件匣樣式通知
套用
NotificationCompat.InboxStyle
敬上
如果您想新增多行簡短摘要行 (例如
節錄自傳入的電子郵件。這樣就能新增多段內容文字
這些文字會截斷成一行,而不是一行連續文字
提供者:NotificationCompat.BigTextStyle
。
如要新增行,請呼叫
addLine()
敬上
最多六次,如以下範例所示。如果新增超過 6 個
只會顯示前 6 行。
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_email_24) .setContentTitle("5 New mails from Frank") .setContentText("Check them out") .setLargeIcon(BitmapFactory.decodeResource(resources, R.drawable.logo)) .setStyle( NotificationCompat.InboxStyle() .addLine("Re: Planning") .addLine("Delivery on its way") .addLine("Follow-up") ) .build()
Java
Notification notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_email_24) .setContentTitle("5 New mails from Frank") .setContentText("Check them out") .setLargeIcon(BitmapFactory.decodeResource(resources, R.drawable.logo)) .setStyle( NotificationCompat.InboxStyle() .addLine("Re: Planning") .addLine("Delivery on its way") .addLine("Follow-up") ) .build();
結果如下圖所示:
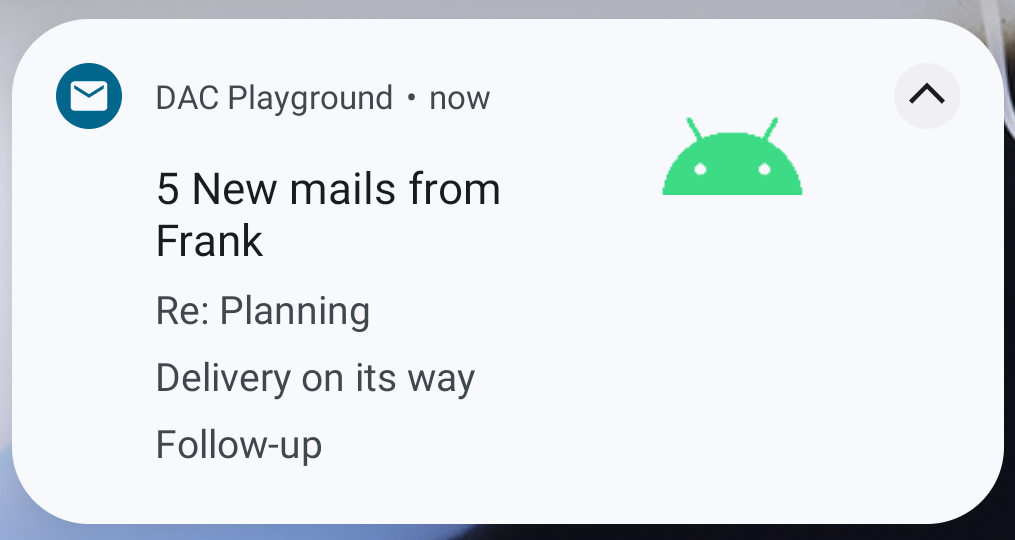
在通知中顯示對話
套用
NotificationCompat.MessagingStyle
敬上
以便在任意數量的使用者之間依序顯示訊息這很適合
訊息應用程式,因為它能為每個訊息提供一致的版面配置
會分別處理寄件者名稱和訊息文字,且每則訊息都可以
多行內容
如要新增訊息,請呼叫
addMessage()
、
傳送訊息文字、接收時間以及傳送者姓名。你也可以
會將這項資訊
NotificationCompat.MessagingStyle.Message
敬上
物件,如以下範例所示:
Kotlin
val message1 = NotificationCompat.MessagingStyle.Message( messages[0].getText(), messages[0].getTime(), messages[0].getSender()) val message2 = NotificationCompat.MessagingStyle.Message( messages[1].getText(), messages[1].getTime(), messages[1].getSender()) val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_message) .setStyle( NotificationCompat.MessagingStyle(resources.getString(R.string.reply_name)) .addMessage(message1) .addMessage(message2)) .build()
Java
NotificationCompat.MessagingStyle.Message message1 = new NotificationCompat.MessagingStyle.Message(messages[0].getText(), messages[0].getTime(), messages[0].getSender()); NotificationCompat.MessagingStyle.Message message2 = new NotificationCompat.MessagingStyle.Message(messages[1].getText(), messages[1].getTime(), messages[1].getSender()); Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_message) .setStyle(new NotificationCompat.MessagingStyle(resources.getString(R.string.reply_name)) .addMessage(message1) .addMessage(message2)) .build();
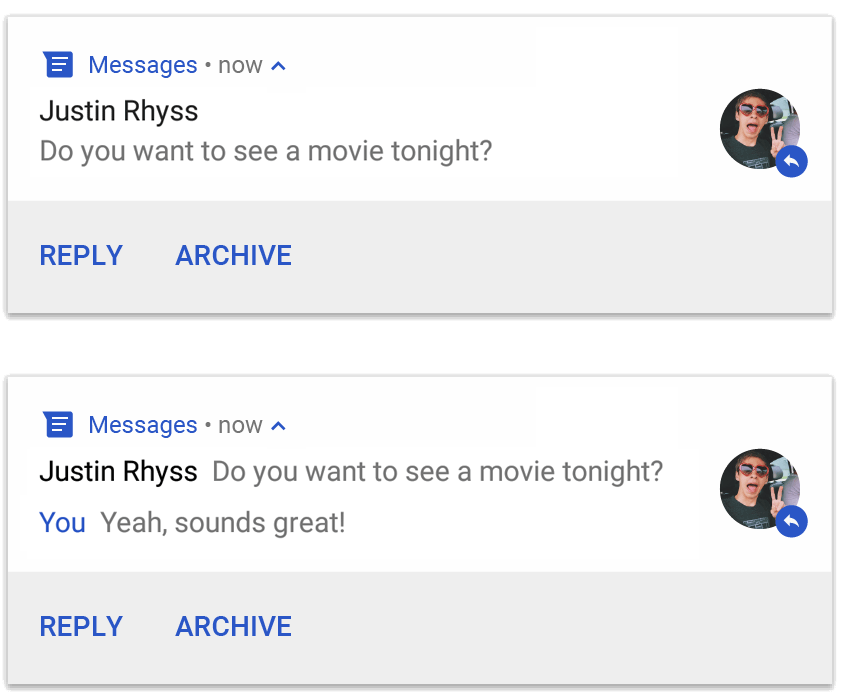
NotificationCompat.MessagingStyle
。
使用 NotificationCompat.MessagingStyle
時,
setContentTitle()
和
setContentText()
系統會忽略此值。
你可以打電話
setConversationTitle()
敬上
新增對話上方顯示的標題。這可能是
使用者建立的群組名稱。如果沒有特定名稱,則將
對話中的參與者不要為下列項目設定對話標題:
進行一對一即時通訊,因為系統會使用這個欄位的提示
群組對話
這個樣式僅適用於搭載 Android 7.0 (API 級別 24) 以上版本的裝置。
使用相容性程式庫時
(NotificationCompat
),
如先前所述,系統會退回 MessagingStyle
的通知
自動轉換成支援的展開通知樣式。
如果您要為即時通訊對話建立這類通知,新增 回覆動作。
使用媒體控制選項建立通知
套用
MediaStyleNotificationHelper.MediaStyle
敬上
顯示媒體播放控制項及追蹤資訊。
請指明您的
MediaSession
位於
建構函式中設定。這樣一來,Android 裝置就能根據使用者的
媒體。
致電
addAction()
敬上
最多顯示 5 個圖示按鈕。撥打電話給「setLargeIcon()
」:
設定專輯封面。
有別於其他通知樣式,MediaStyle
也可讓您修改
指定同時出現的三個動作按鈕,即可調整收合大小的內容檢視畫面
顯示在收合檢視畫面中為此,請提供動作按鈕索引
setShowActionsInCompactView()
。
以下範例說明如何建立具有媒體控制項的通知:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) // Show controls on lock screen even when user hides sensitive content. .setVisibility(NotificationCompat.VISIBILITY_PUBLIC) .setSmallIcon(R.drawable.ic_stat_player) // Add media control buttons that invoke intents in your media service .addAction(R.drawable.ic_prev, "Previous", prevPendingIntent) // #0 .addAction(R.drawable.ic_pause, "Pause", pausePendingIntent) // #1 .addAction(R.drawable.ic_next, "Next", nextPendingIntent) // #2 // Apply the media style template. .setStyle(MediaStyleNotificationHelper.MediaStyle(mediaSession) .setShowActionsInCompactView(1 /* #1: pause button \*/)) .setContentTitle("Wonderful music") .setContentText("My Awesome Band") .setLargeIcon(albumArtBitmap) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) // Show controls on lock screen even when user hides sensitive content. .setVisibility(NotificationCompat.VISIBILITY_PUBLIC) .setSmallIcon(R.drawable.ic_stat_player) // Add media control buttons that invoke intents in your media service .addAction(R.drawable.ic_prev, "Previous", prevPendingIntent) // #0 .addAction(R.drawable.ic_pause, "Pause", pausePendingIntent) // #1 .addAction(R.drawable.ic_next, "Next", nextPendingIntent) // #2 // Apply the media style template. .setStyle(new MediaStyleNotificationHelper.MediaStyle(mediaSession) .setShowActionsInCompactView(1 /* #1: pause button */)) .setContentTitle("Wonderful music") .setContentText("My Awesome Band") .setLargeIcon(albumArtBitmap) .build();
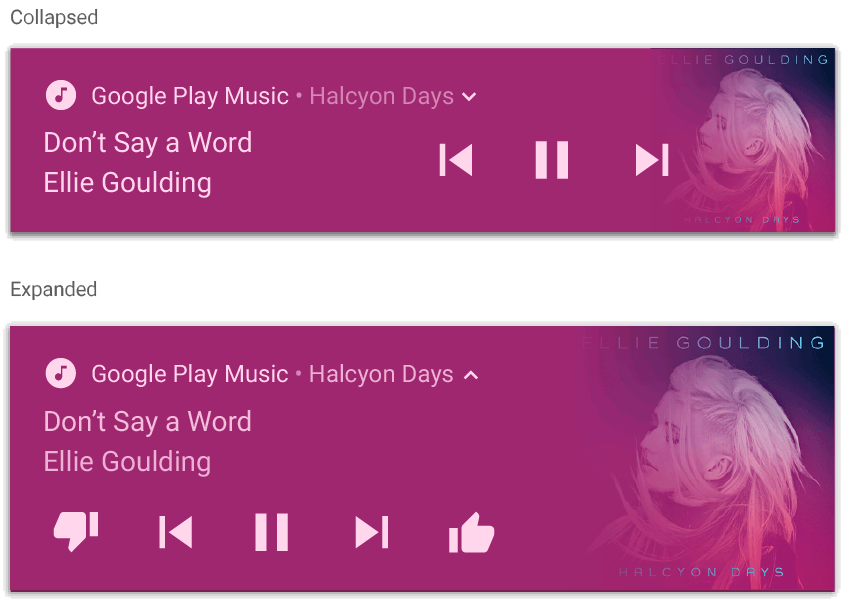
MediaStyleNotificationHelper.MediaStyle
。
其他資源
如要進一步瞭解 MediaStyle
和
可展開式通知