為了讓通知在各種 Android 版本上呈現最佳視覺效果, 使用標準通知 範本 通知。如要在通知中提供更多內容, 建議使用可展開的通知 範本
不過,如果系統範本不符合您的需求,您可以使用自己的 版面配置
為內容區域建立自訂版面配置
如果需要自訂版面配置
NotificationCompat.DecoratedCustomViewStyle
敬上
通知。這個 API 可讓您為內容提供自訂版面配置
區域通常由標題和文字內容佔用,但您仍是使用系統
通知圖示、時間戳記、子文字和動作按鈕的裝飾。
這個 API 的運作方式類似於可展開式通知範本,也就是以基本通知為基礎建構而成 如下所示:
- 建立基本通知
同時
NotificationCompat.Builder
。 - 致電
setStyle()
、 並向該函式傳送NotificationCompat.DecoratedCustomViewStyle
。 - 將自訂版面配置加載為
RemoteViews
。 - 致電
setCustomContentView()
敬上 設定收合通知的版面配置。 - 或者也可以呼叫
setCustomBigContentView()
敬上 為展開的通知設定不同的版面配置。
準備版面配置
您需要有 small
和 large
版面配置。在此範例中,small
版面配置
看起來會像這樣:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="Small notification, showing only a title" />
</LinearLayout>
large
版面配置可能如下所示:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="300dp"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Large notification, showing a title and a body." />
<TextView
android:id="@+id/notification_body"
style="@style/TextAppearance.Compat.Notification.Line2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="This is the body. The height is manually forced to 300dp." />
</LinearLayout>
建構並顯示通知
版面配置準備就緒後,您可以使用這些版面配置,如以下範例所示:
Kotlin
val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager // Get the layouts to use in the custom notification. val notificationLayout = RemoteViews(packageName, R.layout.notification_small) val notificationLayoutExpanded = RemoteViews(packageName, R.layout.notification_large) // Apply the layouts to the notification. val customNotification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build() notificationManager.notify(666, customNotification)
Java
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); // Get the layouts to use in the custom notification RemoteViews notificationLayout = new RemoteViews(getPackageName(), R.layout.notification_small); RemoteViews notificationLayoutExpanded = new RemoteViews(getPackageName(), R.layout.notification_large); // Apply the layouts to the notification. Notification customNotification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(new NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build(); notificationManager.notify(666, customNotification);
請注意,通知的背景顏色可能因裝置而異
和版本可套用支援資料庫樣式,例如
TextAppearance_Compat_Notification
用於文字和
TextAppearance_Compat_Notification_Title
,做為自訂版面配置中的標題。
如以下範例所示這些樣式會配合顏色變化
但你的文字最後不會有黑色或白色
<TextView android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_weight="1" android:text="@string/notification_title" android:id="@+id/notification_title" style="@style/TextAppearance.Compat.Notification.Title" />
請避免在 RemoteViews
物件上設定背景圖片,因為文字
可能會變得無法讀取
如果使用者在使用應用程式時觸發通知,結果會是 就像圖 1 一樣
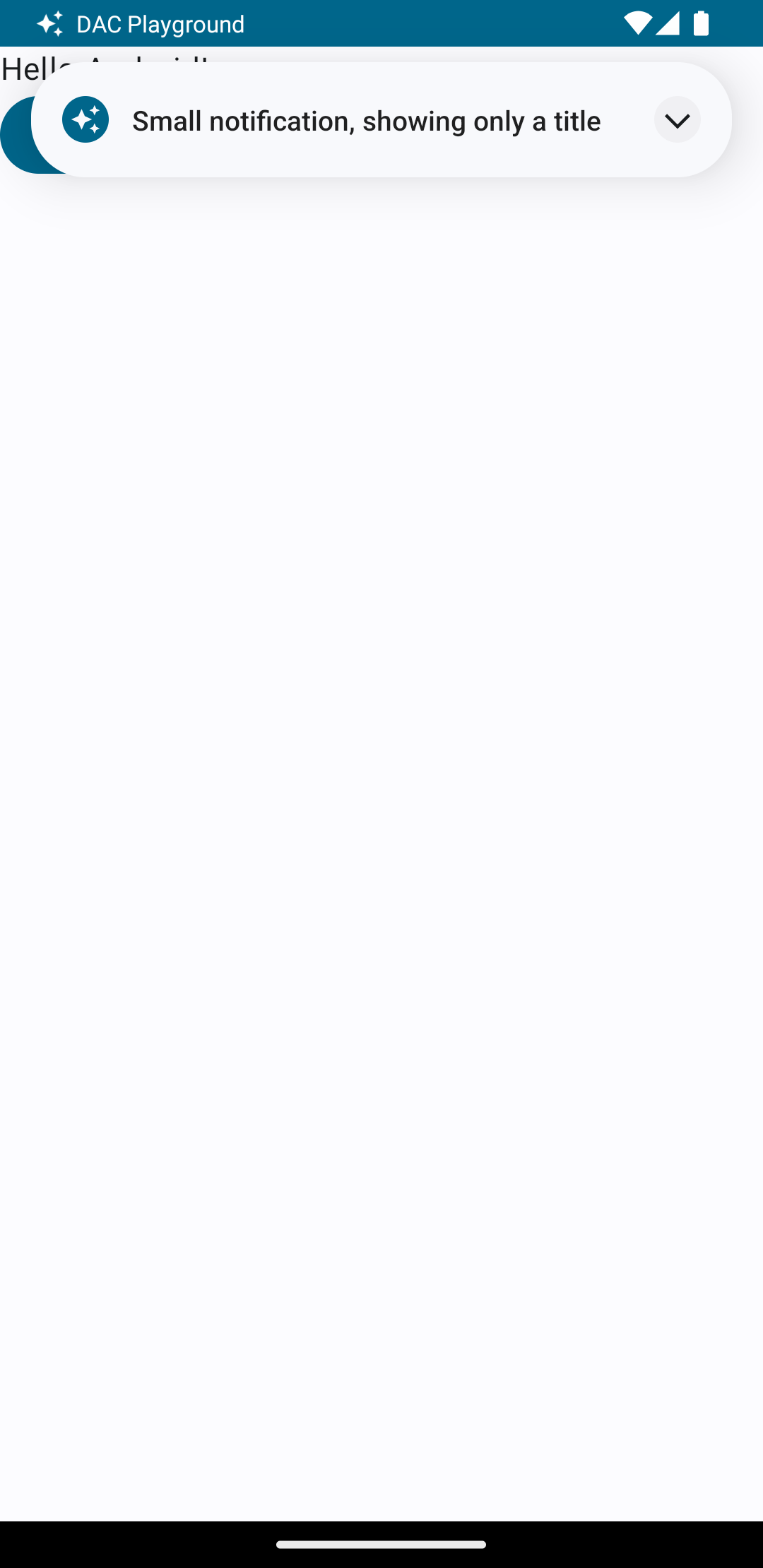
輕觸展開箭頭即可展開通知,如圖 2 所示:
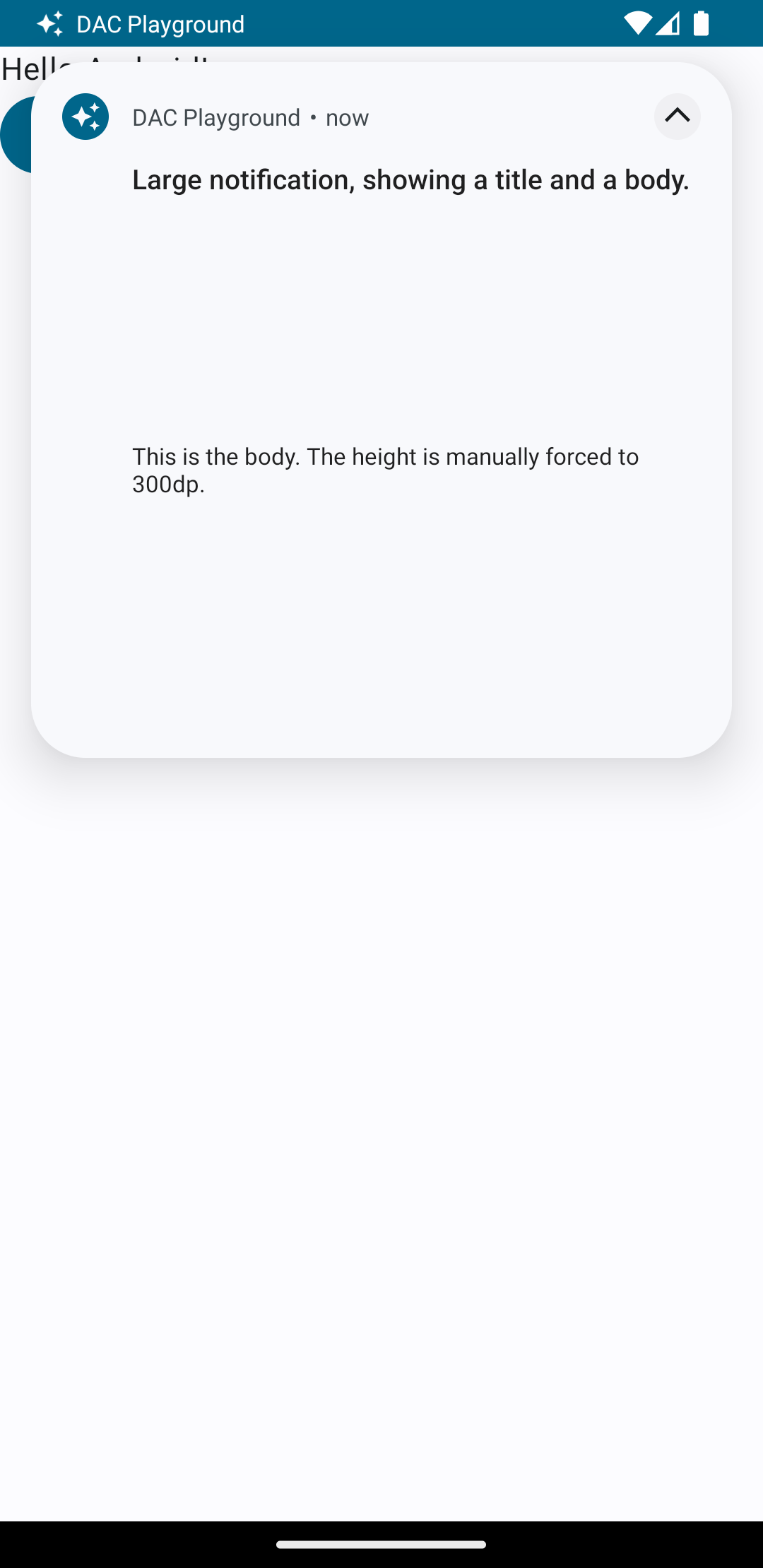
通知逾時屆滿後,通知只會顯示在 系統資訊列,如圖 3 所示:
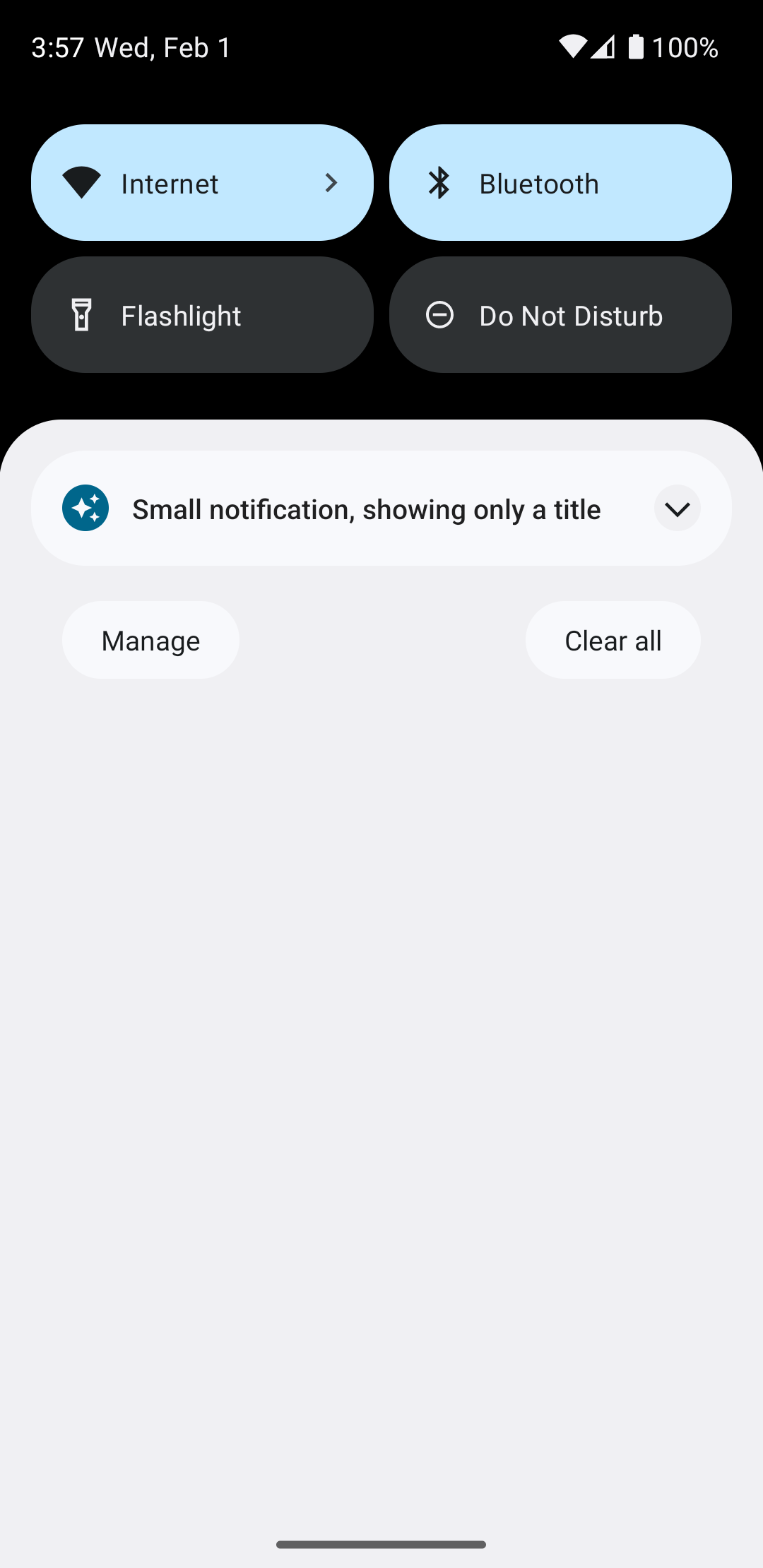
輕觸展開箭頭即可展開通知,如圖 4 所示:
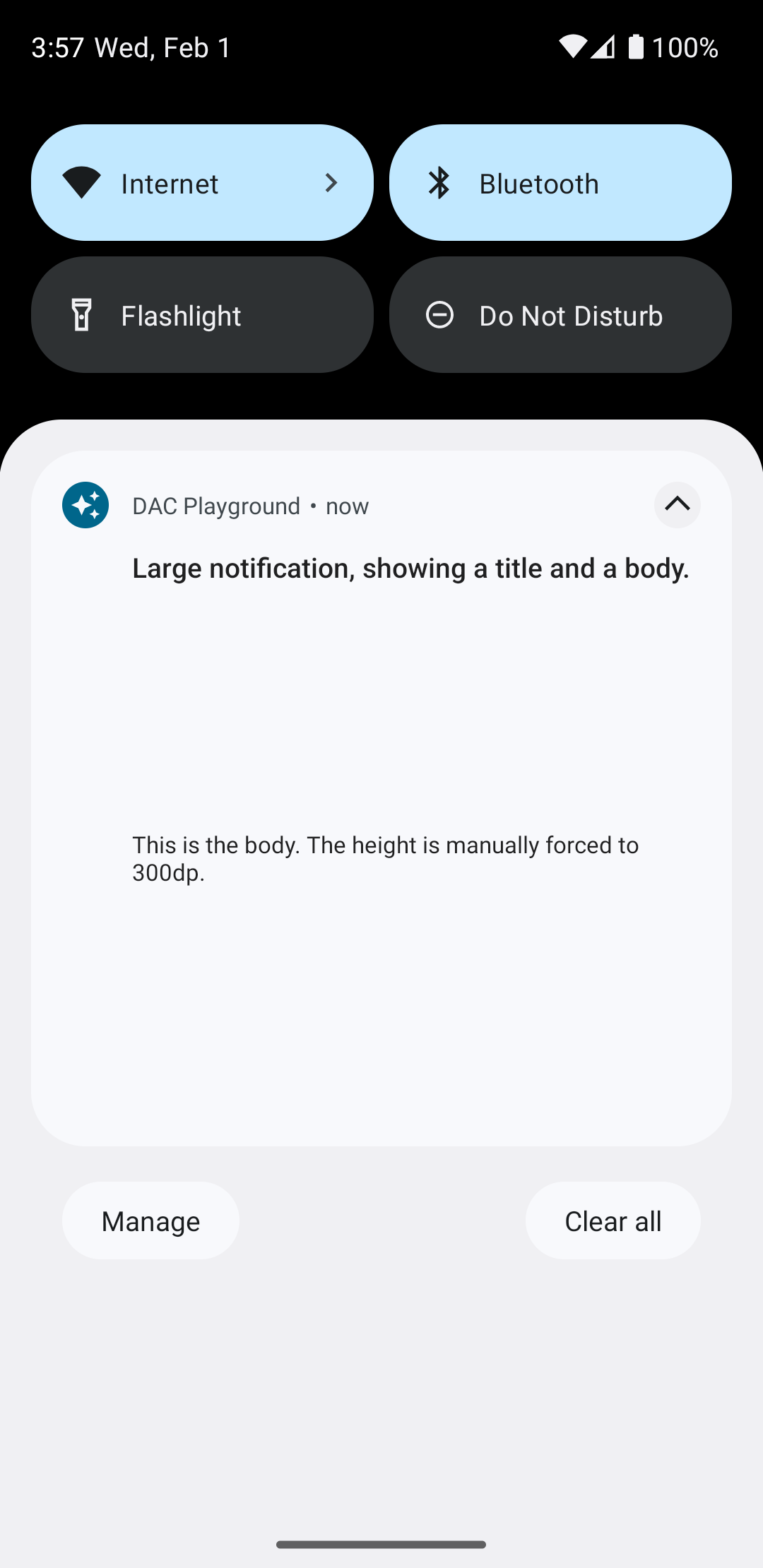
建立完全自訂的通知版面配置
如果不想讓通知加上標準通知裝飾
圖示和標頭,請依上述步驟操作,但「不要」呼叫 setStyle()
。