Android 8.0(API 级别 26)引入了 XML 中的字体,此功能允许您将字体用作资源。您可以在 res/font/
文件夹中添加 font
文件,将字体捆绑为资源。这些字体是在您的 R
文件中编译的,可直接在 Android Studio 中使用。您可以使用 font
资源类型访问字体资源。例如,要访问字体资源,请使用 @font/myfont
或 R.font.myfont
。
要在搭载 Android 4.1(API 级别 16)及更高版本的设备上使用 XML 功能中的字体,请使用支持库 26.0。如需详细了解如何使用支持库,请参阅使用支持库部分。
如需将字体添加为资源,请在 Android Studio 中执行以下步骤:
- 右键点击 res 文件夹,然后转到 New > Android resource directory。此时将显示 New Resource Directory 窗口。
- 在“Resource type”列表中,选择“font”,然后点击 OK。
注意:资源目录的名称必须为 font。
图 1. 添加字体资源目录。
- 在
font
文件夹中添加字体文件。以下文件夹结构生成了
R.font.dancing_script
、R.font.lobster
和R.font.typo_graphica
。图 2. 在
res/font
目录中添加字体文件。 - 双击字体文件可在编辑器中预览文件的字体。
图 3. 预览字体文件。
创建字体系列
字体系列是一组字体文件及其样式和字体粗细详情。 在 Android 中,您可以创建新的字体系列作为 XML 资源,并将其作为单个单元使用,而不是将每个样式和字体粗细作为单独的资源引用。这样,系统就可以根据您使用的文本样式选择正确的字体。
如需创建字体系列,请在 Android Studio 中执行以下步骤:
- 右键点击
font
文件夹,然后依次选择 New > Font resource file。此时将显示“New Resource File”窗口。 - 输入文件名,然后点击 OK。新的字体资源 XML 会在编辑器中打开。
- 将各个字体文件、样式和粗细属性都封装在
<font>
元素中。以下 XML 演示了如何在字体资源 XML 中添加与字体相关的属性:<?xml version="1.0" encoding="utf-8"?> <font-family xmlns:android="http://schemas.android.com/apk/res/android"> <font android:fontStyle="normal" android:fontWeight="400" android:font="@font/lobster_regular" /> <font android:fontStyle="italic" android:fontWeight="400" android:font="@font/lobster_italic" /> </font-family>
在 XML 布局中使用字体
您可以使用 fontFamily
属性在 TextView
对象或样式中使用单个字体文件形式的字体或某个字体系列中的字体。
注意:如果您使用字体系列,TextView
会根据需要自行切换,以使用该系列中的字体文件。
向 TextView 添加字体
如需为 TextView
设置字体,请执行以下操作之一:
- 在布局 XML 文件中,将
fontFamily
属性设置为您要访问的字体文件。<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:fontFamily="@font/lobster"/>
- 打开 Properties 窗口,为
TextView
设置字体。- 选择一个视图以打开 Properties 窗口。
注意:Properties 窗口仅在设计编辑器打开时可用。选择窗口底部的 Design 标签。
- 展开 textAppearance 属性,然后从“fontFamily”列表中选择字体。
-
图 4. 从 Properties 窗口中选择字体。
- 选择一个视图以打开 Properties 窗口。
借助 Android Studio 布局预览(如图 5 最右侧的窗格所示),您可以预览 TextView
中的字体集。
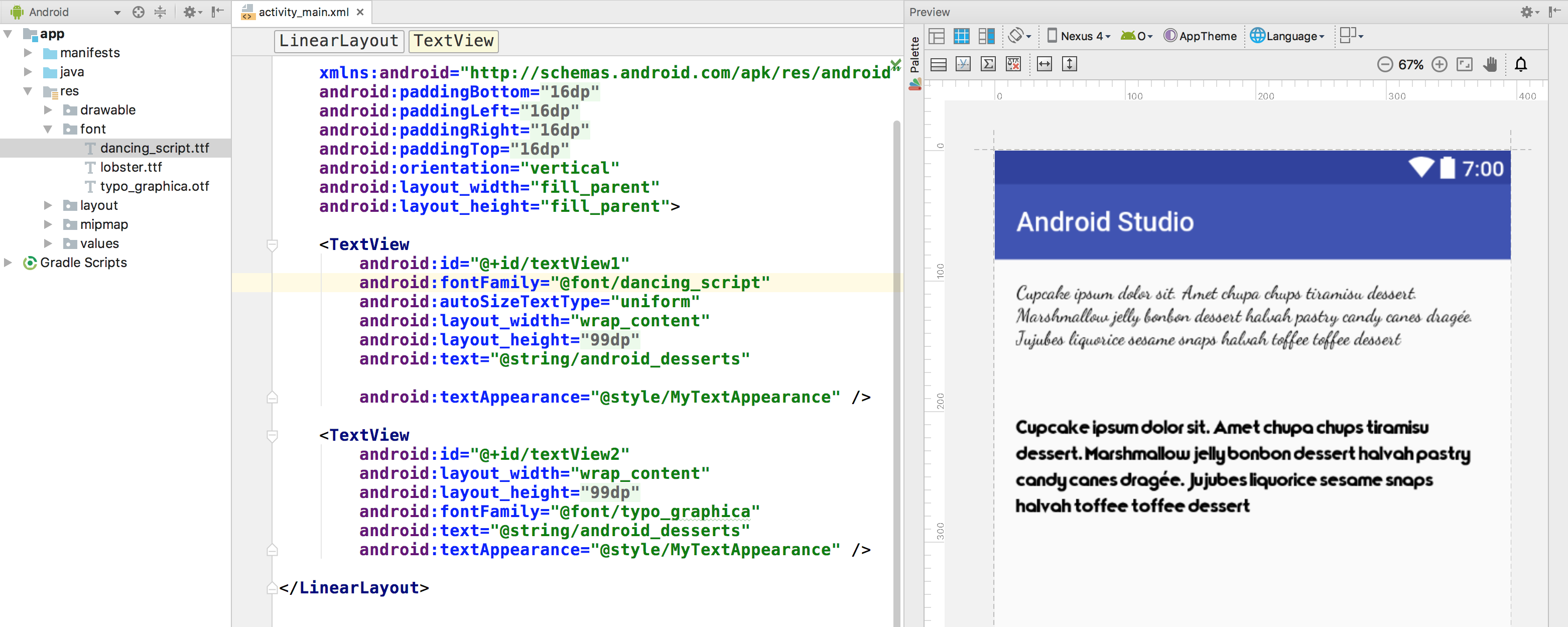
图 5. 在布局预览中预览字体。
向样式添加字体
打开 styles.xml
文件,并将 fontFamily
属性设置为您要访问的字体文件。
<style name="customfontstyle" parent="@android:style/TextAppearance.Small"> <item name="android:fontFamily">@font/lobster</item> </style>
以编程方式使用字体
要以程序化方式检索字体,请调用 getFont(int)
方法,并提供要检索的字体的资源标识符。此方法会返回一个 Typeface
对象。虽然系统会根据字体信息选择最适合您的样式,但您可以使用 setTypeface(android.graphics.Typeface, int)
方法设置具有特定样式的字体。
注意:TextView
会为您执行此操作。
Kotlin
val typeface = resources.getFont(R.font.myfont) textView.typeface = typeface
Java
Typeface typeface = getResources().getFont(R.font.myfont); textView.setTypeface(typeface);
使用支持内容库
支持库 26.0 支持在搭载 Android 4.1(API 级别 16)及更高版本的设备上采用 XML 字体。
注意:当您通过支持库在 XML 布局中声明字体系列时,请使用 app 命名空间,以确保系统能够顺利加载字体。
<?xml version="1.0" encoding="utf-8"?> <font-family xmlns:app="http://schemas.android.com/apk/res-auto"> <font app:fontStyle="normal" app:fontWeight="400" app:font="@font/myfont-Regular"/> <font app:fontStyle="italic" app:fontWeight="400" app:font="@font/myfont-Italic" /> </font-family>
要以编程方式检索字体,请调用 ResourceCompat.getFont(Context, int)
方法,并提供 Context
的实例和资源标识符。
Kotlin
val typeface = ResourcesCompat.getFont(context, R.font.myfont)
Java
Typeface typeface = ResourcesCompat.getFont(context, R.font.myfont);