建議您將 SearchView
小工具用作應用程式列中的項目,在應用程式中提供搜尋功能。如同應用程式列中的所有項目,您可以將 SearchView
定義為一律顯示,或僅在有空間時顯示。您也可以將其定義為可摺疊的動作,讓 SearchView
一開始顯示為圖示,然後在使用者輕觸圖示時,將整個應用程式列用作搜尋欄位。
將 SearchView 新增至應用程式列
如要將 SearchView
小工具新增至應用程式列,請在專案中建立名為 res/menu/options_menu.xml
的檔案,然後將下列程式碼新增至檔案。此程式碼會定義建立搜尋項目的方式,例如要使用的圖示和項目標題。collapseActionView
屬性可讓 SearchView
展開,占用整個應用程式列,並在未使用時折疊回一般應用程式列項目。由於手機裝置上的應用程式列空間有限,建議您使用 collapsibleActionView
屬性,提供更優質的使用者體驗。
<?xml version="1.0" encoding="utf-8"?> <menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@+id/search" android:title="@string/search_title" android:icon="@drawable/ic_search" android:showAsAction="collapseActionView|ifRoom" android:actionViewClass="androidx.appcompat.widget.SearchView" /> </menu>
如要使用更容易存取的搜尋圖示,請在 /res/drawable
資料夾中建立 ic_search.xml
檔案,並在其中加入下列程式碼:
<vector android:height="24dp" android:tint="#000000" android:viewportHeight="24" android:viewportWidth="24" android:width="24dp" xmlns:android="http://schemas.android.com/apk/res/android"> <path android:fillColor="@android:color/white" android:pathData="M15.5,14h-0.79l-0.28,-0.27C15.41,12.59 16,11.11 16,9.5 16,5.91 13.09,3 9.5,3S3,5.91 3,9.5 5.91,16 9.5,16c1.61,0 3.09,-0.59 4.23,-1.57l0.27,0.28v0.79l5,4.99L20.49,19l-4.99,-5zM9.5,14C7.01,14 5,11.99 5,9.5S7.01,5 9.5,5 14,7.01 14,9.5 11.99,14 9.5,14z"/> </vector>
如要在應用程式列中顯示 SearchView
,請在活動的 onCreateOptionsMenu()
方法中展開 XML 選單資源 res/menu/options_menu.xml
:
Kotlin
override fun onCreateOptionsMenu(menu: Menu): Boolean { menuInflater.inflate(R.menu.options_menu, menu) return true }
執行應用程式時會產生類似以下內容:
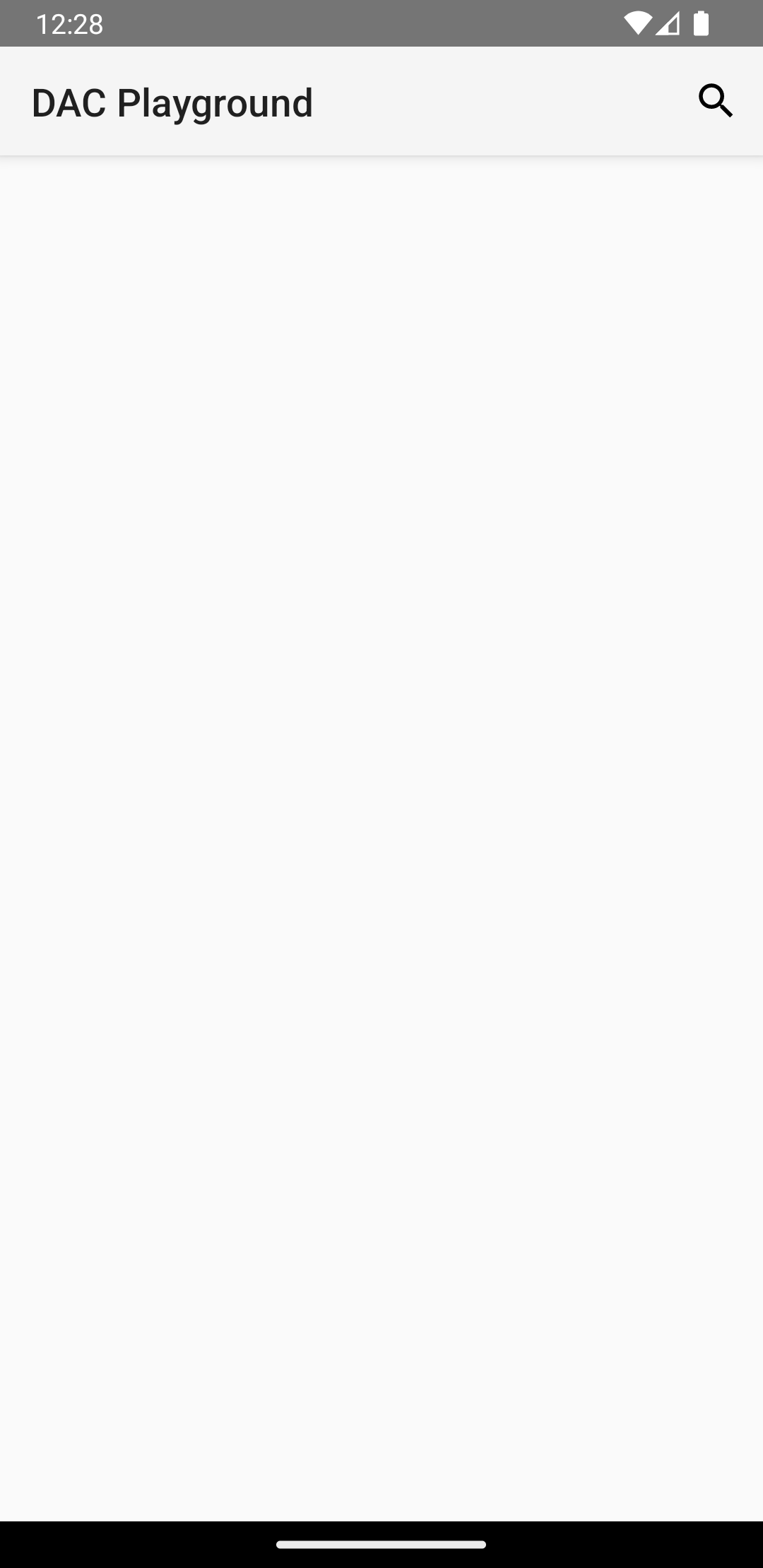
SearchView
會顯示在應用程式的應用程式列中,但無法運作。輕觸搜尋圖示後,畫面會顯示類似下列的畫面:
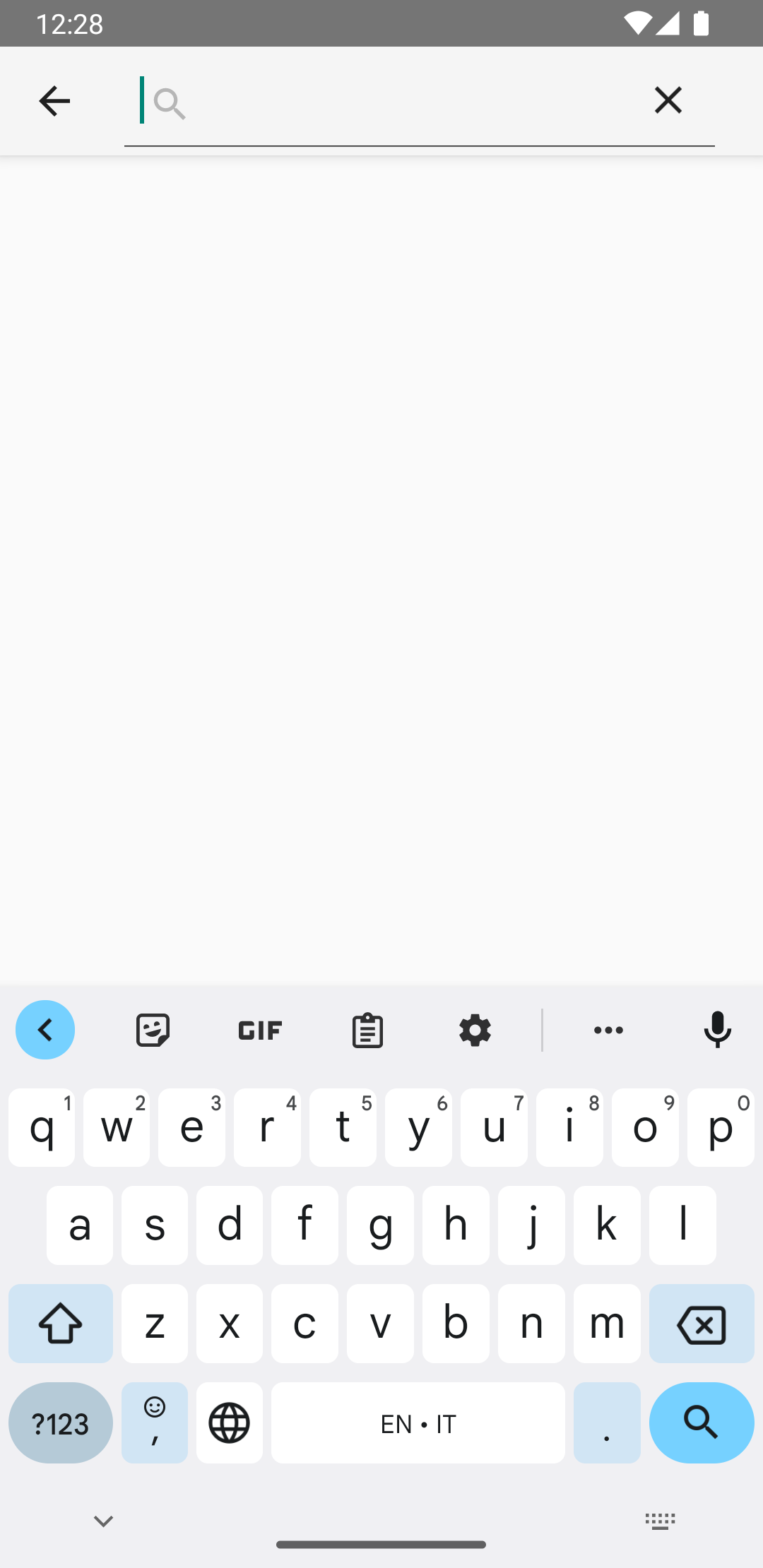
SearchView
的運作情形。如要讓 SearchView
運作,您必須定義 SearchView
的行為方式。
建立搜尋設定
搜尋設定會指定 SearchView
的行為,並在 res/xml/searchable.xml
檔案中定義。搜尋設定至少必須包含一個 android:label
屬性,其值與 Android 資訊清單中 <application> 或 <activity> 元素的 android:label
屬性相同。不過,我們也建議您新增 android:hint
屬性,讓使用者知道該在搜尋框中輸入什麼內容。
<?xml version="1.0" encoding="utf-8"?> <searchable xmlns:android="http://schemas.android.com/apk/res/android" android:label="@string/app_name" android:hint="@string/search_hint" />
在應用程式的資訊清單檔案中,宣告指向 res/xml/searchable.xml
檔案的 <meta-data>
元素。在您要顯示 SearchView
的 <activity>
中宣告元素。
<activity android:name=".SearchResultsActivity" android:exported="false" android:label="@string/title_activity_search_results" android:launchMode="singleTop" android:theme="@style/Theme.AppCompat.Light"> <intent-filter> <action android:name="android.intent.action.SEARCH" /> </intent-filter> <meta-data android:name="android.app.searchable" android:resource="@xml/searchable" /> </activity>
在您建立的 onCreateOptionsMenu()
方法中,呼叫 setSearchableInfo(SearchableInfo)
將搜尋設定與 SearchView
建立關聯:
Kotlin
override fun onCreateOptionsMenu(menu: Menu): Boolean { menuInflater.inflate(R.menu.options_menu, menu) val searchManager = getSystemService(Context.SEARCH_SERVICE) as SearchManager val searchView = menu.findItem(R.id.search).actionView as SearchView val component = ComponentName(this, SearchResultsActivity::class.java) val searchableInfo = searchManager.getSearchableInfo(component) searchView.setSearchableInfo(searchableInfo) return true }
對 getSearchableInfo()
的呼叫會取得從搜尋設定 XML 檔案建立的 SearchableInfo
物件。當搜尋設定與 SearchView
正確建立關聯,且使用者提交查詢時,SearchView
會啟動含有 ACTION_SEARCH
意圖的活動。接著,您需要一個活動,能夠篩選這個意圖並處理搜尋查詢。
建立可搜尋的活動
可搜尋的活動會為 ACTION_SEARCH
意圖篩選,並在資料集中搜尋查詢。如要建立可搜尋的活動,請宣告所選活動,以便篩選 ACTION_SEARCH
意圖:
<activity android:name=".SearchResultsActivity" ... > ... <intent-filter> <action android:name="android.intent.action.SEARCH" /> </intent-filter> ... </activity>
在可搜尋的活動中,請在 onCreate()
方法中檢查 ACTION_SEARCH
意圖,以便處理該意圖。
Kotlin
class SearchResultsActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_search_results) handleIntent(intent) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) handleIntent(intent) } private fun handleIntent(intent: Intent) { if (Intent.ACTION_SEARCH == intent.action) { val query = intent.getStringExtra(SearchManager.QUERY) Log.d("SEARCH", "Search query was: $query") } } }
SearchView
現在可以接受使用者的查詢,並透過 ACTION_SEARCH
意圖啟動可搜尋的活動。
取得搜尋查詢後,您可以將其傳遞至 ViewModel
,並在架構的其他層中使用,以便擷取要顯示的搜尋結果。