To see how your app looks and behaves on a device, you need to build and run it. Android Studio sets up new projects so that you can deploy your app to a virtual or a physical device with just a few clicks.
This overview focuses on how to use Android Studio to build and run your app for testing and debugging. For information on how to use Android Studio to build your app so that it can be released to users, see Build your app for release to users. For more detailed information about managing and customizing your build with or without Android Studio, see Configure your build.
Basic build and run
To build and run your app, follow these steps:
- In the toolbar, select your app from the run configurations menu.
In the target device menu, select the device that you want to run your app on.
If you don't have any devices configured, you need to either create an Android Virtual Device to use the Android Emulator or connect a physical device.
Click Run
.
Android Studio warns you if you attempt to launch your project to a device that has an error or a warning associated with it. Iconography and stylistic changes differentiate between errors (device selections that result in a broken configuration) and warnings (device selections that might result in unexpected behavior but are still runnable).
Monitor the build process
To view details about the build process, select View > Tool
Windows > Build or click Build
in the tool window bar. The Build tool window
displays the
tasks that Gradle executes to build your app, as shown in figure 1.
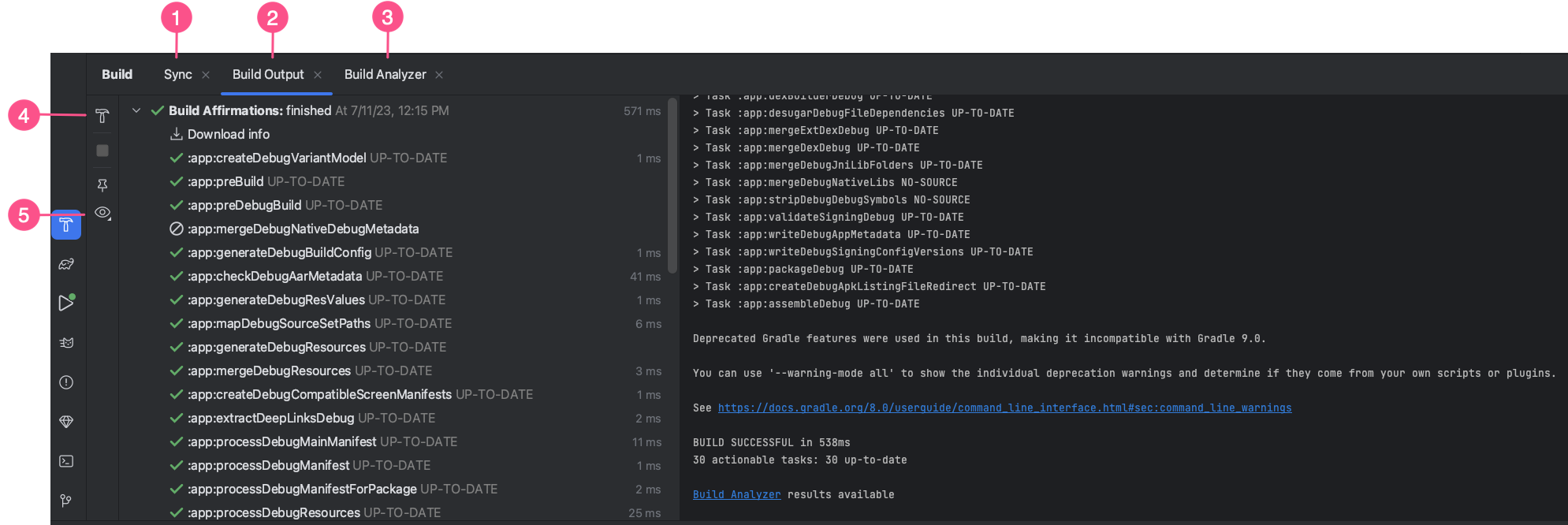
- Sync tab: Displays tasks that Gradle executes to sync with your project files. Similar to the Build Output tab, if you encounter a sync error, select elements in the tree to get more information about the error. Also displays a summary of download impact to determine whether dependency downloads are negatively affecting your build.
- Build Output tab: Displays the tasks that Gradle executes as a tree,
where each node represents either a build phase or a group
of task dependencies. If you receive build-time or compile-time errors,
inspect the tree and select an element to read the error output, as shown in
figure 2.
Figure 2. Inspect the Build Output tab for error messages. - Build Analyzer tab: Provides build performance analysis information about your build. See Troubleshoot build performance with Build Analyzer for more information.
- Restart: Performs the last build action again. If you last ran Build > Make Selected Module, it'll build the current module. If you last ran Build > Make Project, it'll generate intermediate build files for all modules in your project.
- Filters: Filters out warnings, tasks, or both that completed successfully. This can make it easier to find issues in the output.
If your build variants use product flavors, Gradle also invokes tasks to
build those product flavors. To view the list of all available build tasks,
click View > Tool Windows > Gradle or click Gradle
in the tool window bar.
If an error occurs during the build process, Gradle may recommend
command-line options to help you resolve the issue, such as
--stacktrace
or --debug
. To use command-line options
with your build process:
- Open the Settings or Preferences
dialog:
- On Windows or Linux, select File > Settings from the menu bar.
- On macOS, select Android Studio > Preferences from the menu bar.
- Navigate to Build, Execution, Deployment > Compiler.
- In the text field next to Command-line Options, enter your command-line options.
- Click OK to save and exit.
Gradle applies these command-line options the next time you try building your app.
Advanced build and run features
The default way to build and run your app in Android Studio should be sufficient to test a simple app. However, you can use these build and run features for more advanced use cases:
To deploy your app in debug mode, click Debug
. Running your app in debug mode lets you set breakpoints in your code, examine variables and evaluate expressions at run-time, and run debugging tools. To learn more, see Debug your app.
If you have a larger, more complex app, use Apply Changes instead of clicking Run
. This saves time, because you avoid restarting your app every time you want to deploy a change. For more information about Apply Changes, see the Deploy incrementally with Apply Changes section.
If you're using Jetpack Compose, Live Edit is an experimental feature that lets you update composables in real time without re-clicking Run
. This lets you focus on writing UI code with minimal interruption. For more information, see the Live Edit (experimental) section.
If you have an app with multiple build variants or versions, you can choose which build variant to deploy by using the Build Variants tool window. For more information about running a specific build variant, see the Change the build variant section.
To fine-tune app installation, launch, and test options, you can change the run/debug configuration. For more information about creating custom run/debug configurations, see the Create run/debug configurations section.
We recommend that you use Android Studio for your development needs, but you can also deploy your app to a virtual or physical device from the command line. For more information, see Build your app from the command line.
Deploy incrementally with Apply Changes
In Android Studio 3.5 and higher, Apply Changes lets you push code and resource changes to your running app without restarting your app—and, in some cases, without restarting the current activity. This flexibility helps you control how much of your app is restarted when you want to deploy and test small, incremental changes while preserving your device's current state.
Apply Changes uses capabilities in the Android JVMTI implementation that are supported on devices running Android 8.0 (API level 26) or higher. To learn more about how Apply Changes works, see Android Studio Project Marble: Apply Changes.
Requirements
Apply Changes actions are only available when you meet the following conditions:
- You build the APK of your app using a debug build variant.
- You deploy your app to a target device or emulator that runs Android 8.0 (API level 26) or higher.
Use Apply Changes
Use the following options when you want to deploy your changes to a compatible device:
Apply Changes and Restart Activity : Attempts
to apply both your resource and code changes by restarting your activity but
without restarting your app. Generally, you can use this option when you've
modified code in the body of a method or modified an existing resource.
You can also perform this action by pressing Control+Alt+F10 (Control+Command+Shift+R on macOS).
Apply Code Changes : Attempts to apply only
your code changes without restarting anything. Generally, you can use this
option when you've modified code in the body of a method but you haven't
modified any resources. If you've modified both code and resources, use
Apply Changes and Restart Activity instead.
You can also perform this action by pressing Control+F10 (Control+Command+R on macOS).
Run : Deploys all changes and restarts the app. Use this
option when the changes you've made can't be applied using either of the Apply
Changes options. To learn more about the types of changes that require an app
restart, see the Limitations of Apply Changes
section.
Enable run fallback for Apply Changes
When you click either Apply Changes and Restart Activity or
Apply Code Changes, Android Studio builds a new APK and determines whether
the changes can be applied. If the changes can't be applied and would cause
Apply Changes to fail, Android Studio prompts you to Run your app again instead.
If you don't want to be prompted every time this occurs, you can configure Android Studio to automatically rerun your app when changes can't be applied. To enable this behavior, follow these steps:
Open the Settings or Preferences dialog:
- On Windows or Linux, select File > Settings from the menu.
- On macOS, select Android Studio > Preferences from the menu.
Navigate to Build, Execution, Deployment > Deployment.
Select the checkboxes to enable automatic run fallback for either or both of the Apply Changes actions.
Click OK.
Platform-dependent changes
Some features of Apply Changes depend on specific versions of the Android platform. To apply these kinds of changes, your app must be deployed to a device running that version of Android (or higher). For example, adding a method requires Android 11 or higher.
Limitations of Apply Changes
Apply Changes is designed to speed up the app deployment process. However, there are some limitations on when it can be used.
Code changes that require app restart
Some code and resource changes can't be applied until the app is restarted, including the following:
- Adding or removing a field
- Removing a method
- Changing method signatures
- Changing modifiers of methods or classes
- Changing class inheritance
- Changing values in enums
- Adding or removing a resource
- Changing the app manifest
- Changing native libraries (SO files)
Libraries and plugins
Some libraries and plugins automatically make changes to your app's manifest files or to resources that are referenced in the manifest. These automatic updates can interfere with Apply Changes in the following ways:
- If a library or plugin makes changes to your app's manifest, you can't use Apply Changes. You must restart your app to see your changes.
- If a library or plugin makes changes to your app's resource files, you can't
use Apply Code Changes
. You must use Apply Changes and Restart Activity
(or restart your app) to see your changes.
To avoid these limitations, disable all automatic updates for your debug build variants.
For example, Firebase
Crashlytics updates app
resources with a unique build ID during every build, which prevents you from
using Apply Code Changes
and requires you to restart your app's activity to see your changes. Disable
this behavior to use Apply Code Changes alongside Crashlytics with your
debug builds.
Code that directly references content in an installed APK
If your code directly references content from your app's APK that's installed on
the device, that code can cause crashes or misbehave after clicking Apply Code
Changes . This behavior occurs
because when you click Apply Code Changes the underlying APK on the device
is replaced during installation. In these cases, you can click Apply Changes
and Restart Activity
or Run
instead.
If you encounter any other issues while using Apply Changes, file a bug.
Live Edit
Live Edit is an experimental feature in the Android Studio that lets you update composables in emulators and physical devices in real time. This functionality minimizes context switches between writing and building your app, letting you focus on writing code longer without interruption.
Change the build variant
By default, Android Studio builds the debug version of your app, which is
intended for use only during development, when you click Run .
To change the build variant Android Studio uses, do one of the following:
- Select Build > Select Build Variant in the menu.
- Select View > Tool Windows > Build Variants in the menu.
- Click the Build Variants tab on the tool window bar.
For projects without native/C++ code, the Build Variants panel has two columns: Module and Active Build Variant. The Active Build Variant value for the module determines which build variant the IDE deploys to your connected device and is visible in the editor.
Figure 9. The Build Variants panel has two columns for projects that don't have native/C++ code.
To switch between variants, click the Active Build Variant cell for a module and choose the desired variant from the list.
For projects with native/C++ code, the Build Variants panel has three columns:
- Module
- Active Build Variant
- Active ABI
The Active Build Variant value for the module determines the build variant that the IDE deploys to your device and is visible in the editor. For native modules, the Active ABI value determines the ABI that the editor uses, but doesn't impact what is deployed.
Figure 10. The Build Variants panel adds the Active ABI column for projects with native/C++ code.
To change the build variant or ABI, click the cell for the Active Build Variant or Active ABI column and choose the desired variant or ABI from the list. After you change the selection, the IDE syncs your project automatically. Changing either column for an app or library module applies the change to all dependent rows.
By default, new projects are set up with two build variants: a debug variant and release variant. You need to build the release variant to prepare your app for public release. To define other variations of your app with different features or device requirements, you can define additional build variants.
Conflicts in Android Studio Build Variants dialog
In the Android Studio Build Variants dialog, you might see error messages indicating conflicts between build variants, such as the following:
This error doesn't indicate a build issue with Gradle. It indicates that the Android Studio IDE can't resolve symbols between the variants of the selected modules.
For example, if you have a module M1
that depends on variant v1
of module
M2
, but M2
has variant v2
selected in the IDE, you have unresolved symbols
in the IDE. Suppose M1
depends on a class that is only available in v1
; when
v2
is selected, that class is not known by the IDE. Therefore it fails to
resolve the class name and shows errors in the M1
module's code.
These error messages appear because the IDE can't load code for multiple variants simultaneously. In terms of your app’s build, however, the variant selected in this dialog has no effect, because Gradle builds your app with the source code specified in your Gradle build recipes, not based on what’s currently loaded in the IDE.
Change the run/debug configuration
When you run your app for the first time, Android Studio uses a default run configuration. The run configuration specifies whether to deploy your app from an APK or an Android App Bundle as well as the module to run, package to deploy, activity to start, target device, emulator settings, Logcat options, and more.
The default run/debug configuration builds an APK, launches the default project activity, and uses the Select Deployment Target dialog for target device selection. If the default settings don't suit your project or module, you can customize the run/debug configuration or create a new one at the project, default, and module levels.
To edit a run/debug configuration, select Run > Edit Configurations. For more information, see Create and edit run/debug configurations.