As a mobile developer, you're often developing your app's UI step by step rather than developing everything at once. Android Studio embraces this approach with Jetpack Compose by providing tools that don't require a full build to inspect, modify values, and verify the final result.
Live Edit
Live Edit is a feature that lets you update composables in emulators and physical devices in real time. This functionality minimizes context switches between writing and building your app, letting you focus on writing code longer without interruption.
Live Edit has three modes:
- Manual: Code changes are applied when they're manually pushed using Control+\ (Command+\ on macOS)
- Manual on Save: Code changes are applied when they're manually saved using Control+S (Command+S on macOS).
- Automatic: Changes are applied in your device or emulator when you update a composable function.
Live Edit is focused on UI- and UX-related code changes. Live Edit doesn't support changes such as method signature updates, adding new methods, or class hierarchy changes. For more information, see the list of Limitations of Live Edit.
This feature is not a replacement for building and running your app or for Apply Changes. Instead, it's designed to optimize your workflow as you build, deploy, and iterate to develop Compose UI.
The best practice workflow is as follows:
- Set up your application so that it can be run.
- Live Edit as much as possible until you need to make a change that Live Edit doesn't support, such as adding new methods while the app is running.
- After you make an unsupported change, click Run
to restart your app and resume Live Edit.
Get started with Live Edit
To get started, follow these steps to create an empty Compose Activity, enable Live Edit for your project, and make changes with Live Edit.
Set up your new project
Before you begin, make sure that you have Android Studio Giraffe or higher installed and that the API level of your physical device or emulator is at least 30.
Open Android Studio and select New Project in the Welcome to Android Studio dialog. If you already have a project open, you can create a new one by navigating to File > New > New Project.
Choose the Empty Compose Activity template for Phone and Tablet, and then click Next.
Figure 1. Templates you can choose from. For Live Edit, choose Empty Compose Activity. Complete the New Project dialog with the required information: name, package name, save location, minimum SDK, and build configuration language.
Figure 2. Example project settings. Click Finish.
Enable Live Edit
Navigate to the settings to enable Live Edit.
- On Windows or Linux, navigate to File > Settings > Editor > Live Edit.
- On macOS, navigate to Android Studio > Settings > Editor > Live Edit.
Select the Live Edit option and the mode you want to run from the settings.
In manual mode, your code changes are pushed every time you press Control+\ (Command+\ on macOS). In manual mode on save, your code changes are applied every time you manually save, using Control+S (Command+S on macOS). In automatic mode, your code changes are applied in your device or emulator as you make your changes.
Figure 3. Live Edit settings. In the editor, open the
MainActivity
file, which is the entry point for your app.Click Run
to deploy your app.
After you turn on Live Edit, the Up-to-date green checkmark appears in the top right of the Running Devices tool window:
Make and review changes
As you make supported changes in the editor, the virtual or physical test device updates automatically.
For example, edit the existing Greeting
method in MainActivity
to the
following:
@Composable fun Greeting(name: String) { Text( text = "Hello $name!", Modifier .padding(80.dp) // Outer padding; outside background .background(color = Color.Cyan) // Solid element background color .padding(16.dp) // Inner padding; inside background, around text) ) }
Your changes appear instantaneously on the test device, as shown in figure 4.
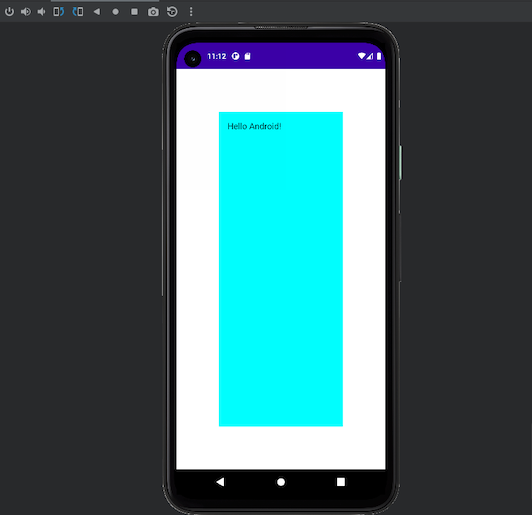
Greeting
method.Troubleshoot Live Edit
If you don't see your edits on the test device, Android Studio might have failed to update your edits. Check whether the Live Edit indicator says Out Of Date as shown in figure 5, which indicates a compilation error. For information about the error and suggestions for how to resolve it, click the indicator.
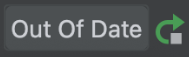
Limitations of Live Edit
The following is a list of current limitations.
[Only applies to Android Studio Giraffe and higher] Live Edit requires Compose Runtime 1.3.0 or higher. If your project uses a lower version of Compose, Live Edit is disabled.
[Only applies to Android Studio Giraffe and higher] Live Edit requires AGP 8.1.0-alpha05 or higher. If your project uses a lower version of AGP, Live Edit is disabled.
Live Edit requires a physical device or emulator that is running API level 30 or higher.
Live Edit only supports editing a function body, which means that you can't change the function name or the signature, add or remove a function, or change non-function fields.
Live Edit resets the app's state the first time you change a Compose function in a file. This only happens after the first code change—the app state isn't reset by subsequent code changes you make to Compose functions in that file.
Live Edit-modified classes might incur some performance penalty. Run your app and use a clean release build if you are evaluating its performance.
You must perform a full run for the debugger to operate on classes that you have modified with Live Edit.
A running app might crash when you edit it with Live Edit. If this happens, you can redeploy the app with the Run
button.
Live Edit doesn't perform any bytecode manipulation that's defined in your project's build file—for example, bytecode manipulation that would be applied when the project is built using the options in the Build menu or by clicking the Build or Run buttons.
Non-Composable functions are updated live on the device or emulator, and a full recomposition is triggered. The full recomposition might not invoke the updated function. For non-composable functions, you must trigger the newly updated functions or run the app again.
Live Edit doesn't resume on app restarts. You must run the app again.
Live Edit only supports debuggable processes.
Live Edit does not support projects that use custom values for
moduleName
underkotlinOptions
in build configuration.Live Edit doesn't work with multi-deploy deployments. This means that you can't deploy to one device, and then to another. Live Edit is only active on the last set of devices the app was deployed to.
Live Edit works with multidevice deployments (deployments to multiple devices that were created through Select multiple devices in the target device dropdown). However, it's not officially supported and there might be issues. If you experience issues, please report them.
Apply Changes/Apply Code Changes are not compatible with Live Edit and require the running app to be restarted.
Live Edit currently does not support Android Automotive projects.
Frequently asked questions about Live Edit
What is the current status of Live Edit?
Live Edit is available in Android Studio Giraffe. To turn it on, navigate to File > Settings > Editor > Live Edit (Android Studio > Settings > Editor > Live Edit on macOS).
When should I use Live Edit?
Use Live Edit when you want to quickly see the effect of updates to UX elements (such as modifier updates and animations) on the overall app experience.
When should I avoid using Live Edit?
Live Edit is focused on UI- and UX-related code changes. It doesn't support changes such as method signature updates, adding new methods, or class hierarchy changes. For more information, see Limitations of Live Edit.
When should I use Compose Preview?
Use Compose Preview when you're developing individual composables. Preview visualizes Compose elements and automatically refreshes to display the effect of code changes. Preview also supports viewing UI elements under different configurations and states, such as dark theme, locales, and font scale.
Live Edit of literals (deprecated)
Android Studio can update in real time some constant literals used in composables within previews, emulator, and physical device. Here are some supported types:
Int
String
Color
Dp
Boolean
You can view constant literals that trigger real time updates without the compilation step by enabling literal decorations through the Live Edit of literals UI indicator:
Apply Changes
Apply Changes lets you update code and resources without having to redeploy your app to an emulator or physical device (with some limitations).
Whenever you add, modify, or delete composables, you can update your app without having to redeploy it by clicking on the Apply Code Changes button:
Recommended for you
- Note: link text is displayed when JavaScript is off
- Customize animations {:#customize-animations}
- Value-based animations
- Add parameters