Glance पर गड़बड़ी को मैनेज करने की सुविधा को बेहतर बनाने के लिए, Android 15 में एपीआई की सुविधाएं शामिल की गई हैं. इस पेज पर, इन एपीआई के इस्तेमाल के सबसे सही तरीके बताए गए हैं.
ऐसे कॉम्पोनेंट के लिए try-catch ब्लॉक का इस्तेमाल करना जो कॉम्पोज़ नहीं किए जा सकते
Compose, कॉम्पोज़ेबल के आस-पास try-catch ब्लॉक की अनुमति नहीं देता. हालांकि, इन ब्लॉक में अपने ऐप्लिकेशन के दूसरे लॉजिक को रैप किया जा सकता है. इससे गड़बड़ी के व्यू के लिए, लिखें का इस्तेमाल किया जा सकता है, जैसा कि इस उदाहरण में दिखाया गया है:
provideContent {
var isError = false;
var data = null
try {
val repository = (context.applicationContext as MyApplication).myRepository
data = repository.loadData()
} catch (e: Exception) {
isError = true;
//handleError
}
if (isError) {
ErrorView()
} else {
Content(data)
}
}
गड़बड़ी का डिफ़ॉल्ट लेआउट
अगर कोई अपवाद नहीं मिला है या Compose में कोई गड़बड़ी हुई है, तो Glance में गड़बड़ी का डिफ़ॉल्ट लेआउट दिखता है:
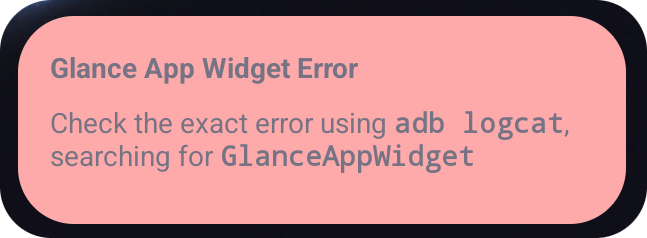
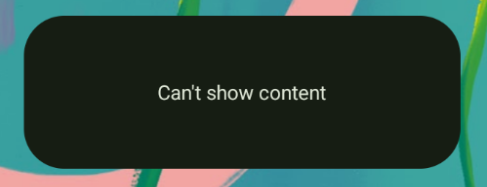
Glance की मदद से, डेवलपर फ़ॉलबैक के तौर पर एक्सएमएल लेआउट उपलब्ध करा सकते हैं. ऐसा तब किया जाता है, जब कॉम्पोज़िशन काम न करे. इसका मतलब है कि Compose कोड में कोई गड़बड़ी थी. गड़बड़ी का यह यूज़र इंटरफ़ेस (यूआई) तब भी दिखता है, जब आपके ऐप्लिकेशन के कोड में कोई ऐसी गड़बड़ी हो जिसे ठीक नहीं किया गया है.
class UpgradeWidget : GlanceAppWidget(errorUiLayout = R.layout.error_layout)
यह एक स्टैटिक लेआउट है. इसमें उपयोगकर्ता इंटरैक्ट नहीं कर सकता. हालांकि, यह आपातकालीन मामलों में अच्छा होता है.
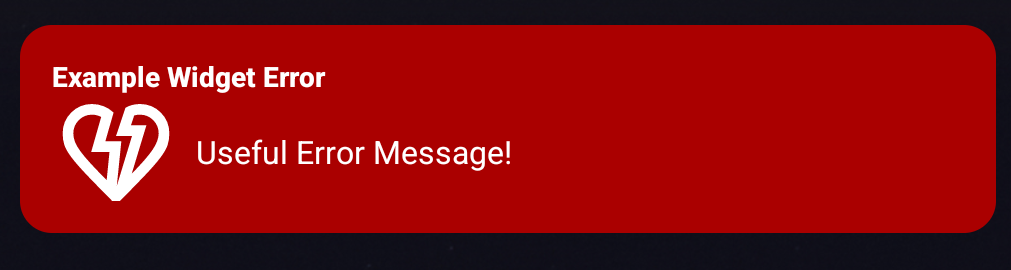
गड़बड़ी के डिफ़ॉल्ट यूज़र इंटरफ़ेस (यूआई) में कार्रवाइयां जोड़ना
Glance 1.1.0 में, गड़बड़ी को मैनेज करने के लिए डिफ़ॉल्ट कोड को बदला जा सकता है. इस तरह, कॉम्पोज़िशन में किसी अपवाद या गड़बड़ी का पता चलने पर, ऐक्शन कॉलबैक जोड़े जा सकते हैं.
इस सुविधा का इस्तेमाल करने के लिए, onCompositionError()
फ़ंक्शन को बदलें:
GlanceAppWidget.onCompositionError(
context: Context,
glanceId: GlanceId,
appWidgetId: Int,
throwable: Throwable
)
इस फ़ंक्शन में, गड़बड़ी को ठीक करने के लिए Glance, RemoteViews
एपीआई का इस्तेमाल करता है.
इससे, एक्सएमएल का इस्तेमाल करके लेआउट और ऐक्शन हैंडलर तय किए जा सकते हैं.
यहां दिए गए उदाहरणों में, गड़बड़ी का ऐसा यूज़र इंटरफ़ेस (यूआई) बनाने का तरीका बताया गया है जिसमें सुझाव/राय/शिकायत भेजने के लिए बटन शामिल हो:
error_layout.xml
फ़ाइल लिखें:<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" style="@style/Widget.MyApplication.AppWidget.Error" android:id="@android:id/background" android:layout_width="match_parent" android:textSize="24sp" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/error_title_view" android:layout_width="match_parent" android:textColor="@color/white" android:textFontWeight="800" android:layout_height="wrap_content" android:text="Example Widget Error" /> <LinearLayout android:layout_width="match_parent" android:orientation="horizontal" android:paddingTop="4dp" android:layout_height="match_parent"> <ImageButton android:layout_width="64dp" android:layout_height="64dp" android:layout_gravity="center" android:tint="@color/white" android:id="@+id/error_icon" android:src="@drawable/heart_broken_fill0_wght400_grad0_opsz24" /> <TextView android:id="@+id/error_text_view" android:layout_width="wrap_content" android:textColor="@color/white" android:layout_height="wrap_content" android:layout_gravity="center" android:padding="8dp" android:textSize="16sp" android:layout_weight="1" android:text="Useful Error Message!" /> </LinearLayout> </LinearLayout>
onCompositionError
फ़ंक्शन को बदलना:override fun onCompositionError( context: Context, glanceId: GlanceId, appWidgetId: Int, throwable: Throwable ) { val rv = RemoteViews(context.packageName, R.layout.error_layout) rv.setTextViewText( R.id.error_text_view, "Error was thrown. \nThis is a custom view \nError Message: `${throwable.message}`" ) rv.setOnClickPendingIntent(R.id.error_icon, getErrorIntent(context, throwable)) AppWidgetManager.getInstance(context).updateAppWidget(appWidgetId, rv) }
ऐसा पेंडिंग इंटेंट बनाएं जिसमें आपके
GlanceAppWidgetReceiver
का रेफ़रंस हो:private fun getErrorIntent(context: Context, throwable: Throwable): PendingIntent { val intent = Intent(context, UpgradeToHelloWorldPro::class.java) intent.setAction("widgetError") return PendingIntent.getBroadcast(context, 0, intent, PendingIntent.FLAG_IMMUTABLE) }
अपने
GlanceAppWidgetReceiver
में इंटेंट को मैनेज करें:override fun onReceive(context: Context, intent: Intent) { super.onReceive(context, intent) Log.e("ErrorOnClick", "Button was clicked."); }