Compose มีกลไกภาพเคลื่อนไหวในตัวมากมาย ซึ่งอาจทำให้คุณสับสนว่าจะเลือกกลไกใด ด้านล่างนี้คือรายการ Use Case ทั่วไปของแอนิเมชัน อ่านข้อมูลโดยละเอียดเพิ่มเติมเกี่ยวกับตัวเลือก API ต่างๆ ทั้งหมดที่ใช้ได้ได้ที่เอกสารประกอบเกี่ยวกับการสร้างภาพเคลื่อนไหวฉบับเต็ม
ทำให้คุณสมบัติที่ประกอบได้ทั่วไปเคลื่อนไหว
Compose มี API ที่สะดวกซึ่งช่วยให้คุณแก้ปัญหาสำหรับ Use Case ภาพเคลื่อนไหวที่พบบ่อยจำนวนมาก ส่วนนี้จะสาธิตวิธีสร้างภาพเคลื่อนไหวให้กับพร็อพเพอร์ตี้ทั่วไปของคอมโพสิเบิล
ภาพเคลื่อนไหวที่ปรากฏ / หายไป
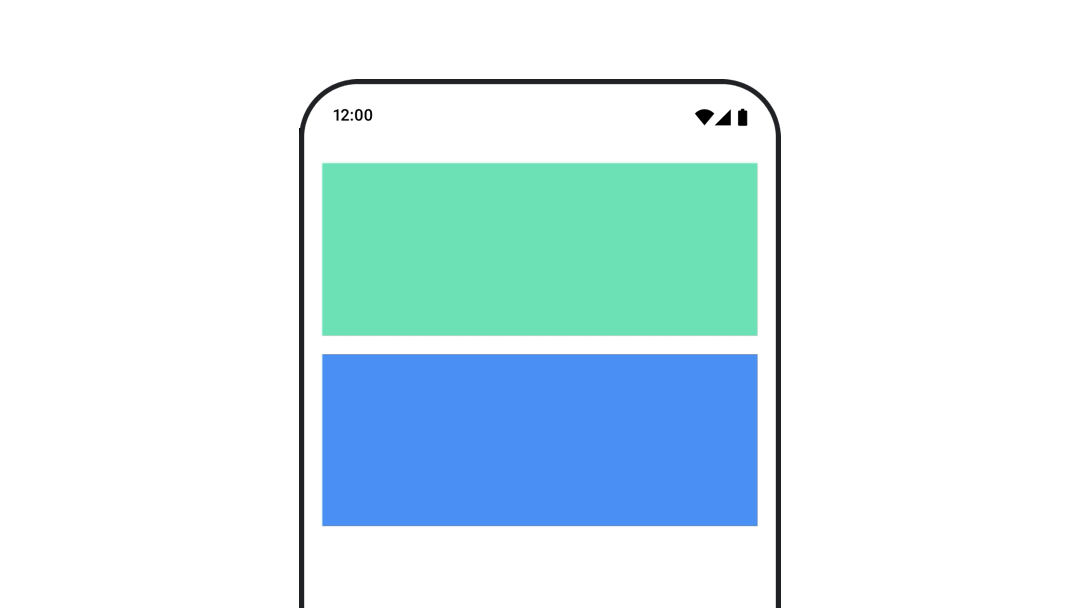
ใช้ AnimatedVisibility
เพื่อซ่อนหรือแสดง Composable เด็กที่อยู่ใน AnimatedVisibility
สามารถใช้ Modifier.animateEnterExit()
สำหรับทรานซิชันเข้าหรือออกของตนเอง
var visible by remember { mutableStateOf(true) } // Animated visibility will eventually remove the item from the composition once the animation has finished. AnimatedVisibility(visible) { // your composable here // ... }
พารามิเตอร์ "เข้า" และ "ออก" ของ AnimatedVisibility
ช่วยให้คุณกําหนดค่าลักษณะการทํางานของคอมโพสิเบิลเมื่อปรากฏขึ้นและหายไป อ่านข้อมูลเพิ่มเติมในเอกสารประกอบฉบับเต็ม
อีกทางเลือกหนึ่งในการทำให้การแสดงผลของคอมโพสิเบิลเคลื่อนไหวคือการทำให้ค่าอัลฟ่าเคลื่อนไหวตามเวลาโดยใช้ animateFloatAsState
ดังนี้
var visible by remember { mutableStateOf(true) } val animatedAlpha by animateFloatAsState( targetValue = if (visible) 1.0f else 0f, label = "alpha" ) Box( modifier = Modifier .size(200.dp) .graphicsLayer { alpha = animatedAlpha } .clip(RoundedCornerShape(8.dp)) .background(colorGreen) .align(Alignment.TopCenter) ) { }
อย่างไรก็ตาม การเปลี่ยนค่าอัลฟ่ามีข้อควรระวังคือคอมโพสิชันที่คอมโพสได้จะยังคงอยู่ในองค์ประกอบและยังคงใช้พื้นที่ที่วางไว้ต่อไป ซึ่งอาจทำให้โปรแกรมอ่านหน้าจอและกลไกการช่วยเหลือพิเศษอื่นๆ ยังคงพิจารณารายการบนหน้าจอ ในทางกลับกัน AnimatedVisibility
จะนํารายการออกจากการประพันธ์ในท้ายที่สุด
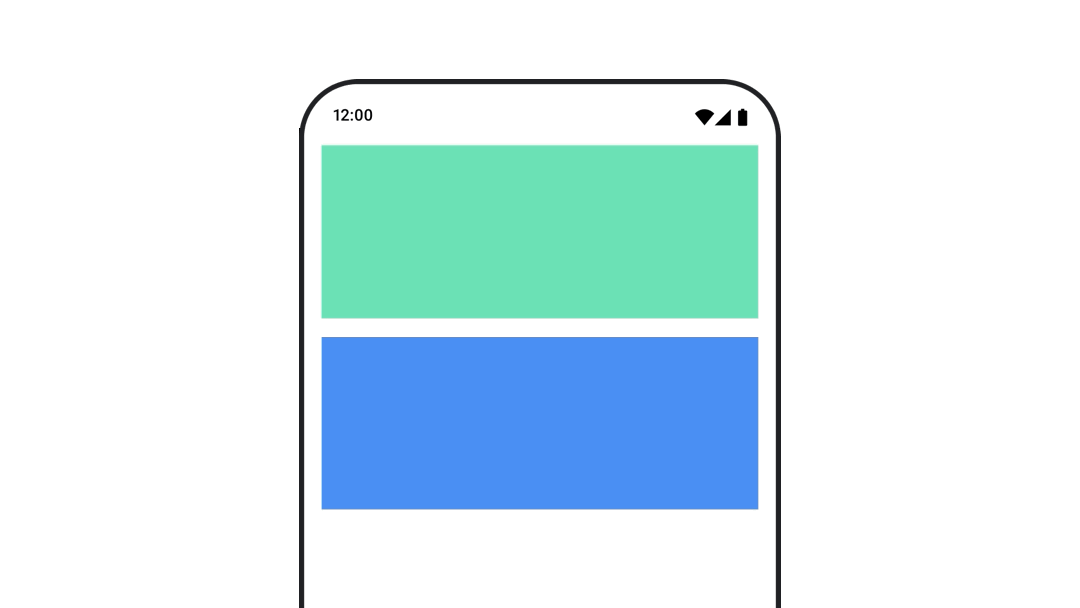
ทำให้สีพื้นหลังเคลื่อนไหว
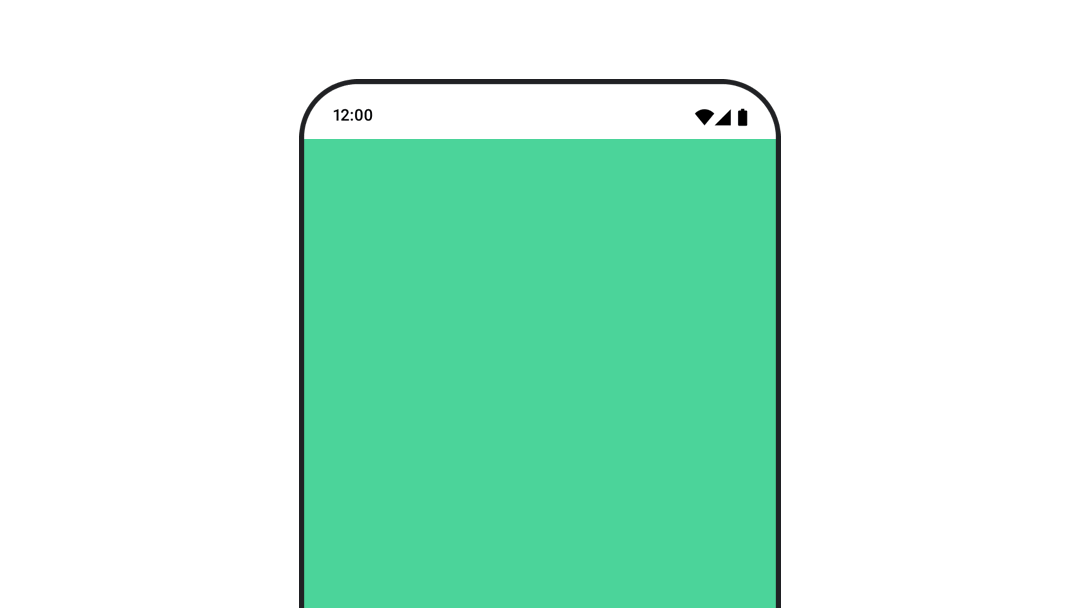
val animatedColor by animateColorAsState( if (animateBackgroundColor) colorGreen else colorBlue, label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(animatedColor) } ) { // your composable here }
ตัวเลือกนี้มีประสิทธิภาพมากกว่าการใช้ Modifier.background()
Modifier.background()
ยอมรับได้สำหรับการตั้งค่าสีแบบครั้งเดียว แต่เมื่อสร้างภาพเคลื่อนไหวของสีเมื่อเวลาผ่านไป การตั้งค่านี้อาจทําให้ต้องจัดองค์ประกอบใหม่มากกว่าที่จําเป็น
หากต้องการทำให้สีพื้นหลังเคลื่อนไหวแบบไม่สิ้นสุด โปรดดูส่วนการทำภาพเคลื่อนไหวซ้ำ
แสดงขนาดของคอมโพสิเบิลเป็นภาพเคลื่อนไหว
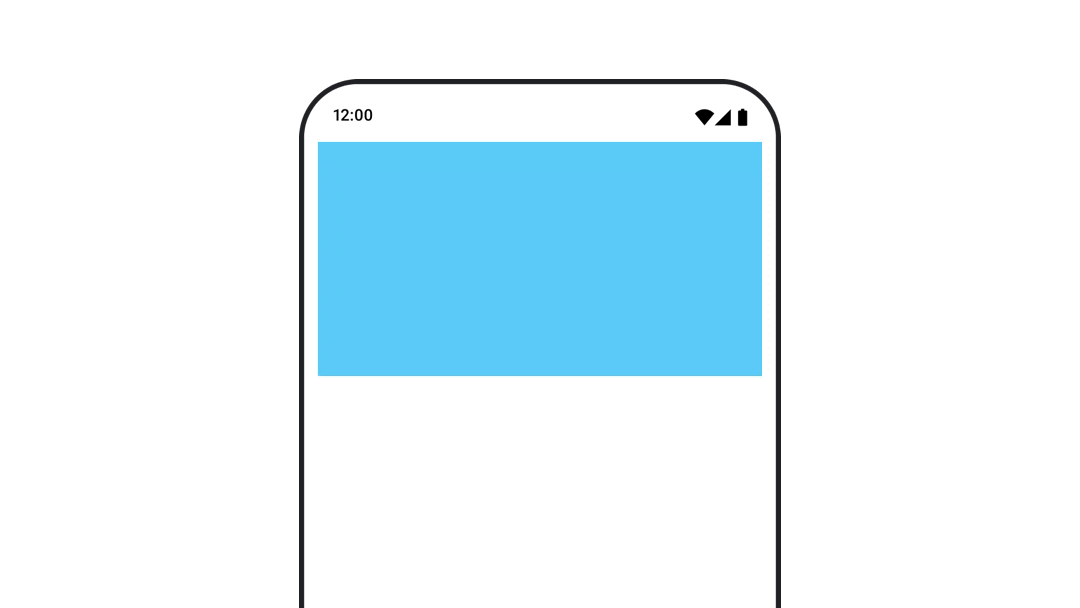
คอมโพสิชันช่วยให้คุณสร้างภาพเคลื่อนไหวขนาดของคอมโพสิเบิลได้หลายวิธี ใช้
animateContentSize()
สำหรับภาพเคลื่อนไหวระหว่างการเปลี่ยนแปลงขนาดที่คอมโพสิเบิล
เช่น หากคุณมีกล่องข้อความที่มีข้อความซึ่งขยายจาก 1 บรรทัดเป็นหลายบรรทัด ก็ใช้ Modifier.animateContentSize()
เพื่อทำให้การเปลี่ยนราบรื่นขึ้นได้ ดังนี้
var expanded by remember { mutableStateOf(false) } Box( modifier = Modifier .background(colorBlue) .animateContentSize() .height(if (expanded) 400.dp else 200.dp) .fillMaxWidth() .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { expanded = !expanded } ) { }
คุณยังใช้ AnimatedContent
ร่วมกับ SizeTransform
เพื่ออธิบายวิธีเปลี่ยนขนาดได้ด้วย
เคลื่อนไหวตําแหน่งของคอมโพสิเบิล
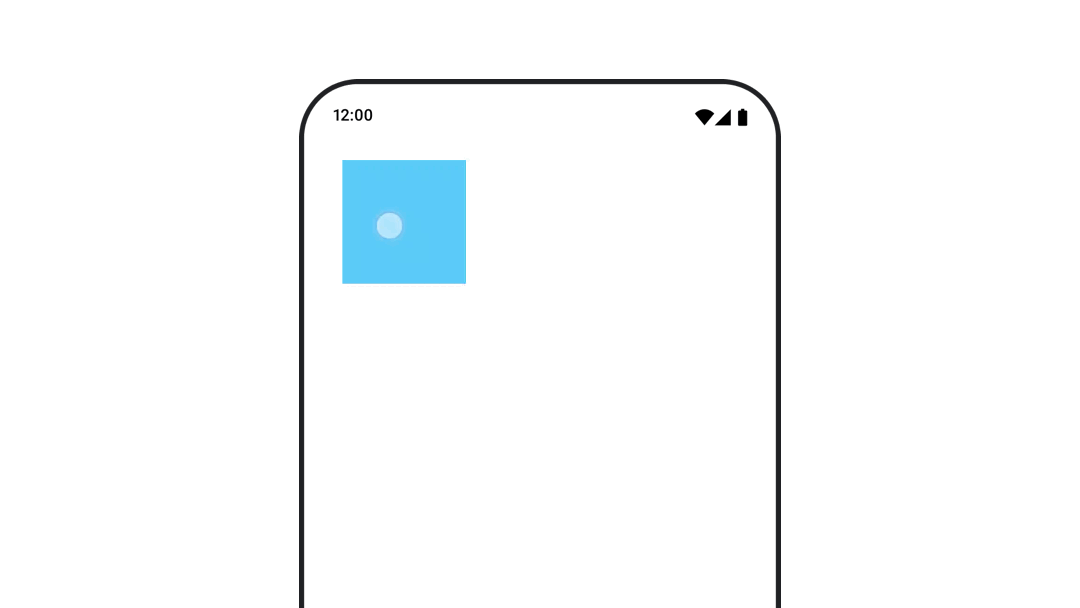
หากต้องการทำให้ตำแหน่งของคอมโพสิเบิลเคลื่อนไหว ให้ใช้ Modifier.offset{ }
ร่วมกับ
animateIntOffsetAsState()
var moved by remember { mutableStateOf(false) } val pxToMove = with(LocalDensity.current) { 100.dp.toPx().roundToInt() } val offset by animateIntOffsetAsState( targetValue = if (moved) { IntOffset(pxToMove, pxToMove) } else { IntOffset.Zero }, label = "offset" ) Box( modifier = Modifier .offset { offset } .background(colorBlue) .size(100.dp) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { moved = !moved } )
หากต้องการให้คอมโพสิเบิลไม่วาดทับหรืออยู่ใต้คอมโพสิเบิลอื่นๆ เมื่อแสดงภาพเคลื่อนไหวตำแหน่งหรือขนาด ให้ใช้ Modifier.layout{ }
ตัวแก้ไขนี้จะส่งต่อการเปลี่ยนแปลงขนาดและตําแหน่งไปยังองค์ประกอบหลัก ซึ่งจะส่งผลต่อองค์ประกอบย่อยอื่นๆ
ตัวอย่างเช่น หากคุณย้าย Box
ภายใน Column
และรายการย่อยอื่นๆ ต้องย้ายด้วยเมื่อ Box
ย้าย ให้ใส่ข้อมูลออฟเซตพร้อมกับ Modifier.layout{ }
ดังนี้
var toggled by remember { mutableStateOf(false) } val interactionSource = remember { MutableInteractionSource() } Column( modifier = Modifier .padding(16.dp) .fillMaxSize() .clickable(indication = null, interactionSource = interactionSource) { toggled = !toggled } ) { val offsetTarget = if (toggled) { IntOffset(150, 150) } else { IntOffset.Zero } val offset = animateIntOffsetAsState( targetValue = offsetTarget, label = "offset" ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) Box( modifier = Modifier .layout { measurable, constraints -> val offsetValue = if (isLookingAhead) offsetTarget else offset.value val placeable = measurable.measure(constraints) layout(placeable.width + offsetValue.x, placeable.height + offsetValue.y) { placeable.placeRelative(offsetValue) } } .size(100.dp) .background(colorGreen) ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) }
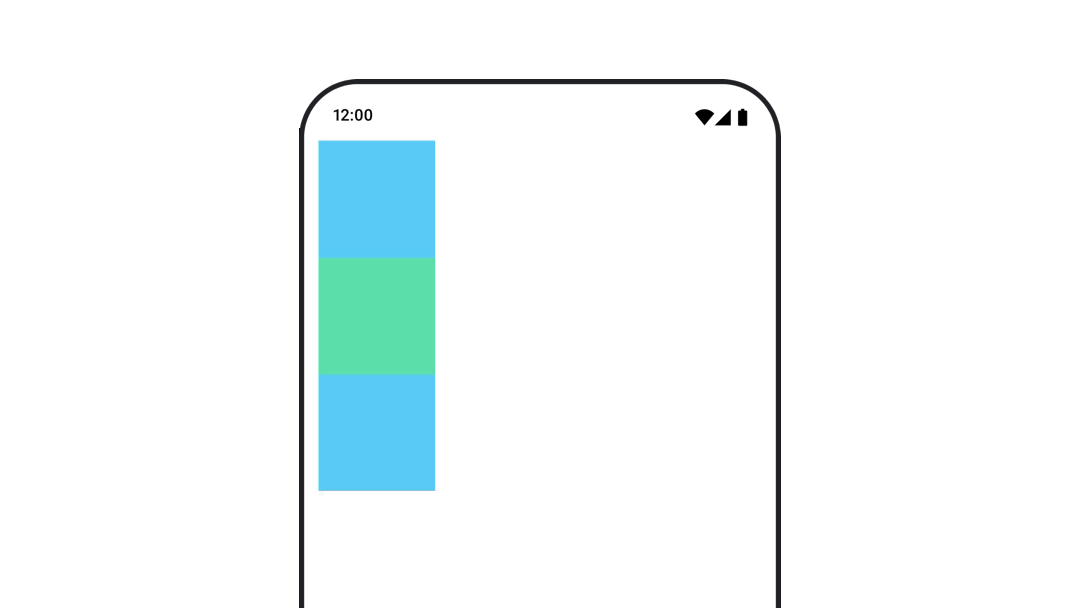
Modifier.layout{ }
ทำให้ระยะห่างจากขอบของ Composable เคลื่อนไหว
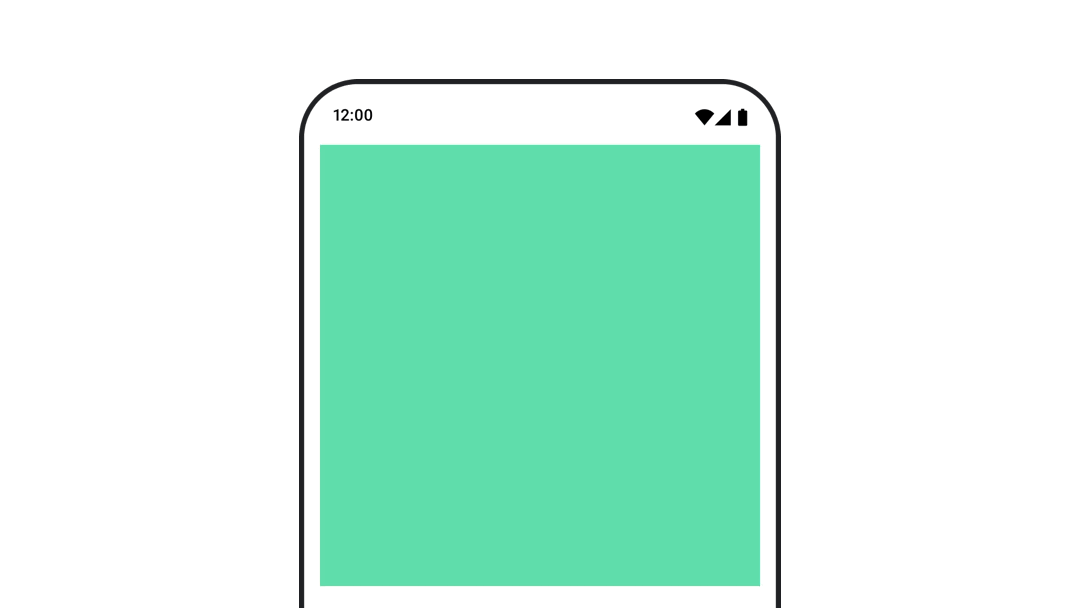
หากต้องการทำให้การเว้นวรรคของคอมโพสิเบิลเคลื่อนไหว ให้ใช้ animateDpAsState
ร่วมกับ Modifier.padding()
ดังนี้
var toggled by remember { mutableStateOf(false) } val animatedPadding by animateDpAsState( if (toggled) { 0.dp } else { 20.dp }, label = "padding" ) Box( modifier = Modifier .aspectRatio(1f) .fillMaxSize() .padding(animatedPadding) .background(Color(0xff53D9A1)) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { toggled = !toggled } )
แสดงภาพเคลื่อนไหวการยกระดับคอมโพเนนต์
หากต้องการสร้างการเคลื่อนไหวของ Composable ให้ใช้ animateDpAsState
ร่วมกับ Modifier.graphicsLayer{ }
สำหรับการเปลี่ยนแปลงระดับความสูงแบบครั้งเดียว ให้ใช้
Modifier.shadow()
หากต้องการสร้างภาพเคลื่อนไหวเงา การใช้ตัวแก้ไข Modifier.graphicsLayer{ }
เป็นตัวเลือกที่มีประสิทธิภาพมากกว่า
val mutableInteractionSource = remember { MutableInteractionSource() } val pressed = mutableInteractionSource.collectIsPressedAsState() val elevation = animateDpAsState( targetValue = if (pressed.value) { 32.dp } else { 8.dp }, label = "elevation" ) Box( modifier = Modifier .size(100.dp) .align(Alignment.Center) .graphicsLayer { this.shadowElevation = elevation.value.toPx() } .clickable(interactionSource = mutableInteractionSource, indication = null) { } .background(colorGreen) ) { }
หรือจะใช้คอมโพสิเบิล Card
แล้วตั้งค่าพร็อพเพอร์ตี้ระดับเป็นค่าที่แตกต่างกันในแต่ละสถานะก็ได้
ทำให้การปรับขนาด การเปลี่ยนตำแหน่ง หรือการหมุนข้อความเคลื่อนไหว
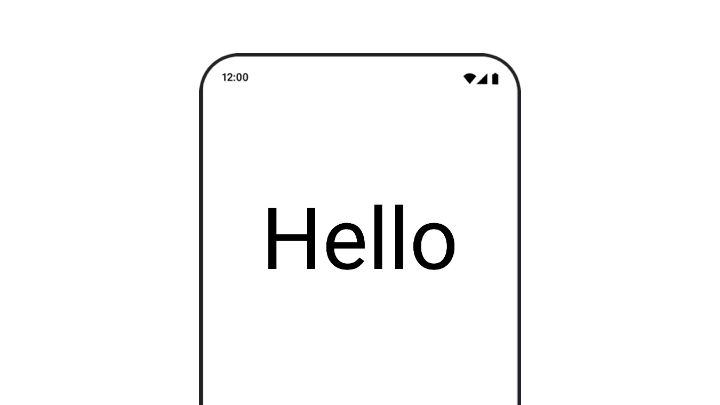
เมื่อแสดงภาพเคลื่อนไหวการปรับขนาด การเปลี่ยนตำแหน่ง หรือการหมุนข้อความ ให้ตั้งค่าพารามิเตอร์ textMotion
ใน TextStyle
เป็น TextMotion.Animated
เพื่อให้การเปลี่ยนระหว่างภาพเคลื่อนไหวข้อความ
ราบรื่นขึ้น ใช้ Modifier.graphicsLayer{ }
เพื่อแปล หมุน หรือปรับขนาดข้อความ
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val scale by infiniteTransition.animateFloat( initialValue = 1f, targetValue = 8f, animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "scale" ) Box(modifier = Modifier.fillMaxSize()) { Text( text = "Hello", modifier = Modifier .graphicsLayer { scaleX = scale scaleY = scale transformOrigin = TransformOrigin.Center } .align(Alignment.Center), // Text composable does not take TextMotion as a parameter. // Provide it via style argument but make sure that we are copying from current theme style = LocalTextStyle.current.copy(textMotion = TextMotion.Animated) ) }
สีข้อความแบบเคลื่อนไหว
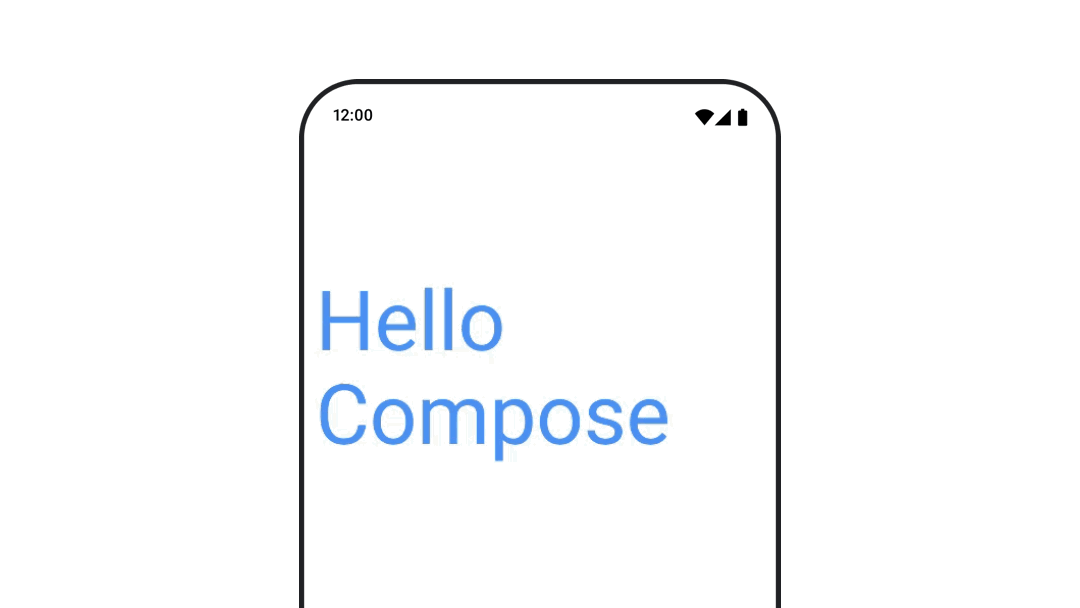
หากต้องการทำให้สีข้อความเคลื่อนไหว ให้ใช้ color
lambda ในคอมโพสิชัน BasicText
ดังนี้
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val animatedColor by infiniteTransition.animateColor( initialValue = Color(0xFF60DDAD), targetValue = Color(0xFF4285F4), animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "color" ) BasicText( text = "Hello Compose", color = { animatedColor }, // ... )
สลับระหว่างเนื้อหาประเภทต่างๆ
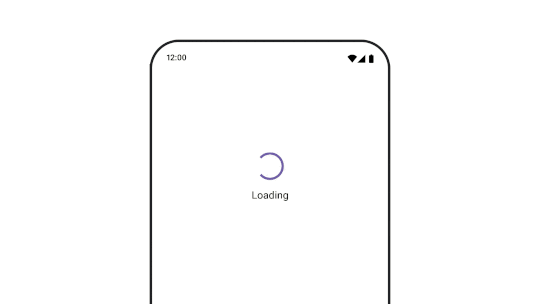
ใช้ AnimatedContent
เพื่อแสดงภาพเคลื่อนไหวระหว่างคอมโพสิเบิลต่างๆ หากต้องการใช้การเฟดมาตรฐานระหว่างคอมโพสิเบิล ให้ใช้ Crossfade
var state by remember { mutableStateOf(UiState.Loading) } AnimatedContent( state, transitionSpec = { fadeIn( animationSpec = tween(3000) ) togetherWith fadeOut(animationSpec = tween(3000)) }, modifier = Modifier.clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { state = when (state) { UiState.Loading -> UiState.Loaded UiState.Loaded -> UiState.Error UiState.Error -> UiState.Loading } }, label = "Animated Content" ) { targetState -> when (targetState) { UiState.Loading -> { LoadingScreen() } UiState.Loaded -> { LoadedScreen() } UiState.Error -> { ErrorScreen() } } }
AnimatedContent
สามารถปรับแต่งให้แสดงทรานซิชันของช่วงเข้าและออกได้หลายประเภท อ่านข้อมูลเพิ่มเติมได้ในเอกสารใน AnimatedContent
หรืออ่านบล็อกโพสต์นี้ใน
AnimatedContent
เคลื่อนไหวขณะนำทางไปยังจุดหมายต่างๆ
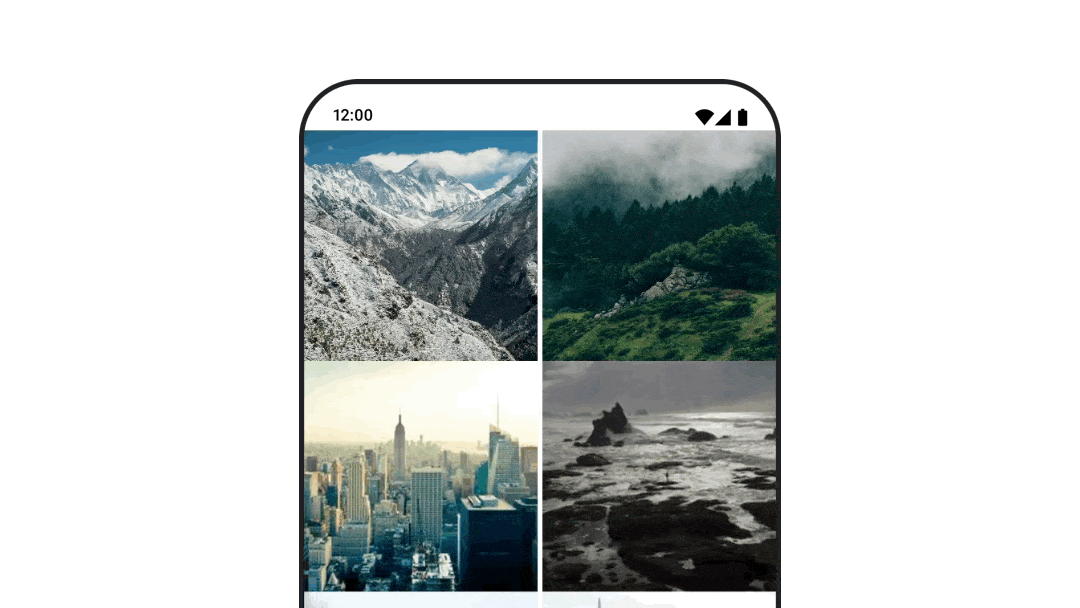
หากต้องการแสดงภาพเคลื่อนไหวของการเปลี่ยนระหว่างคอมโพสิเบิลเมื่อใช้อาร์ติแฟกต์ navigation-compose ให้ระบุ enterTransition
และ exitTransition
ในคอมโพสิเบิล นอกจากนี้ คุณยังตั้งค่าภาพเคลื่อนไหวเริ่มต้นให้ใช้กับปลายทางทั้งหมดที่ระดับบนสุด NavHost
ได้ด้วย โดยทำดังนี้
val navController = rememberNavController() NavHost( navController = navController, startDestination = "landing", enterTransition = { EnterTransition.None }, exitTransition = { ExitTransition.None } ) { composable("landing") { ScreenLanding( // ... ) } composable( "detail/{photoUrl}", arguments = listOf(navArgument("photoUrl") { type = NavType.StringType }), enterTransition = { fadeIn( animationSpec = tween( 300, easing = LinearEasing ) ) + slideIntoContainer( animationSpec = tween(300, easing = EaseIn), towards = AnimatedContentTransitionScope.SlideDirection.Start ) }, exitTransition = { fadeOut( animationSpec = tween( 300, easing = LinearEasing ) ) + slideOutOfContainer( animationSpec = tween(300, easing = EaseOut), towards = AnimatedContentTransitionScope.SlideDirection.End ) } ) { backStackEntry -> ScreenDetails( // ... ) } }
ทรานซิชันของช่วงเข้าและช่วงออกมีหลายประเภทที่ใช้เอฟเฟกต์ที่แตกต่างกันกับเนื้อหาขาเข้าและขาออก โปรดดูข้อมูลเพิ่มเติมในเอกสารประกอบ
เล่นภาพเคลื่อนไหวซ้ำ
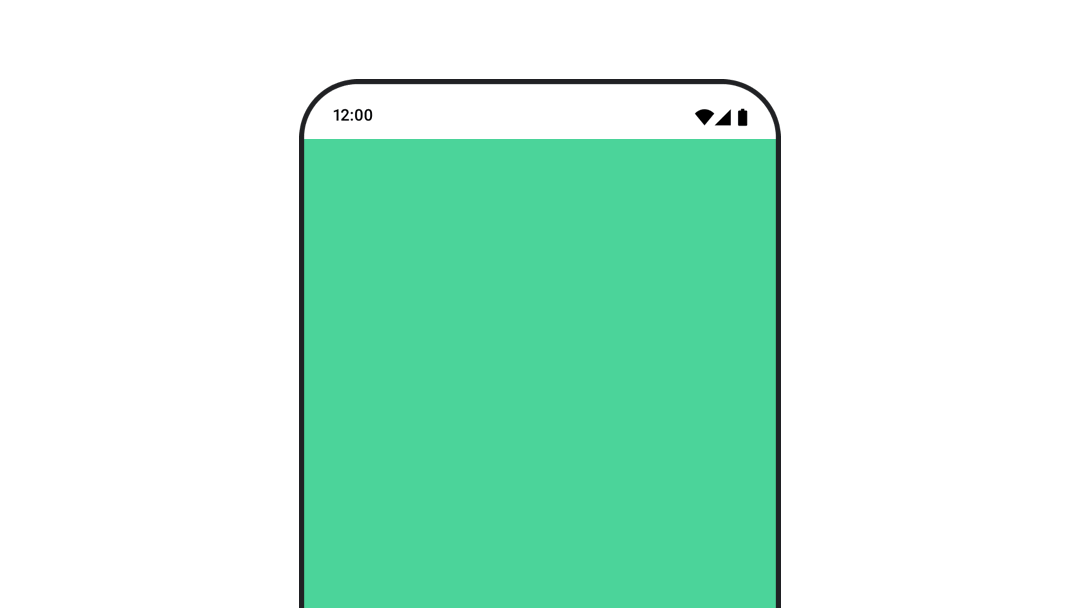
ใช้ rememberInfiniteTransition
กับ infiniteRepeatable
animationSpec
เพื่อแสดงภาพเคลื่อนไหวซ้ำไปเรื่อยๆ เปลี่ยน RepeatModes
เพื่อระบุวิธีเลื่อนไปมา
ใช้ finiteRepeatable
เพื่อทำซ้ำตามจำนวนครั้งที่กำหนด
val infiniteTransition = rememberInfiniteTransition(label = "infinite") val color by infiniteTransition.animateColor( initialValue = Color.Green, targetValue = Color.Blue, animationSpec = infiniteRepeatable( animation = tween(1000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(color) } ) { // your composable here }
เริ่มภาพเคลื่อนไหวเมื่อเปิดใช้งานคอมโพสิเบิล
LaunchedEffect
จะทำงานเมื่อ Composable เข้าสู่การเรียบเรียง แท็กจะเริ่มต้นภาพเคลื่อนไหวเมื่อเปิดใช้งาน Composable ที่คุณสามารถใช้เพื่อขับเคลื่อนการเปลี่ยนแปลงสถานะของภาพเคลื่อนไหว การใช้ Animatable
กับเมธอด animateTo
เพื่อเริ่มภาพเคลื่อนไหวเมื่อเปิด
val alphaAnimation = remember { Animatable(0f) } LaunchedEffect(Unit) { alphaAnimation.animateTo(1f) } Box( modifier = Modifier.graphicsLayer { alpha = alphaAnimation.value } )
สร้างภาพเคลื่อนไหวตามลำดับ
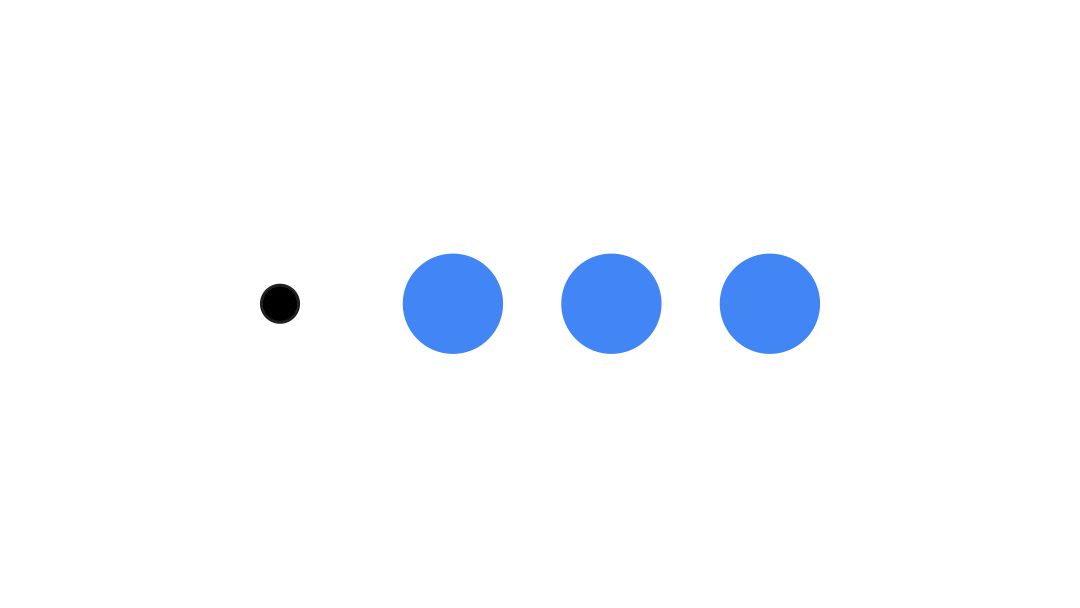
ใช้ Animatable
coroutine API เพื่อแสดงภาพเคลื่อนไหวตามลำดับหรือพร้อมกัน การเรียก animateTo
ใน Animatable
ทีละรายการจะทำให้ภาพเคลื่อนไหวแต่ละรายการรอให้ภาพเคลื่อนไหวก่อนหน้าเสร็จสิ้นก่อนดำเนินการต่อ
เนื่องจากเป็นฟังก์ชันการระงับ
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { alphaAnimation.animateTo(1f) yAnimation.animateTo(100f) yAnimation.animateTo(500f, animationSpec = tween(100)) }
สร้างภาพเคลื่อนไหวพร้อมกัน
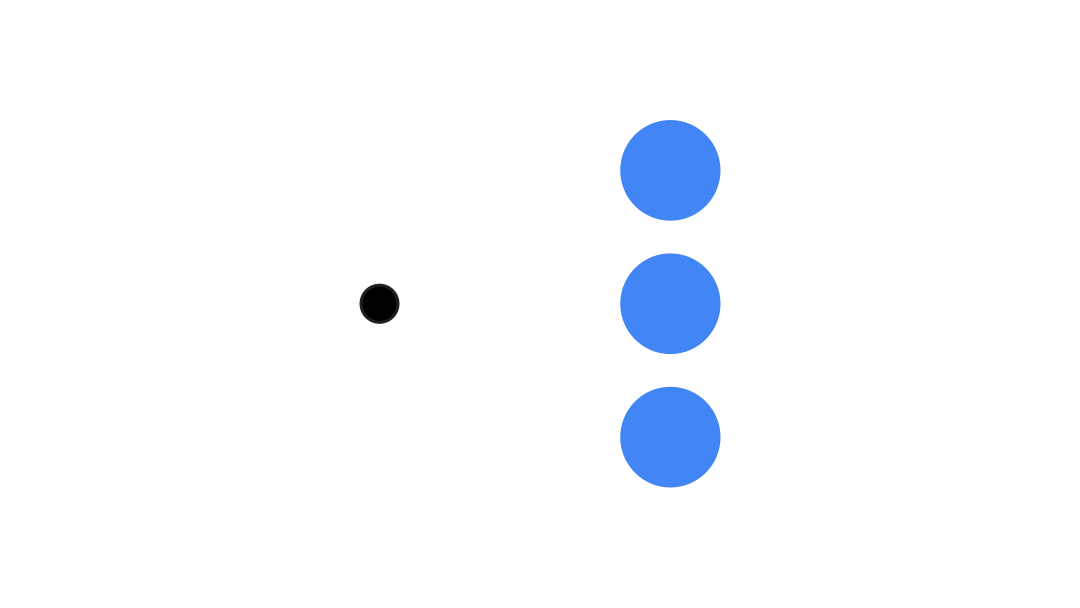
ใช้ coroutine API (Animatable#animateTo()
หรือ animate
) หรือ Transition
API เพื่อให้ได้ภาพเคลื่อนไหวพร้อมกัน หากคุณใช้ฟังก์ชันการเริ่มหลายรายการในบริบท coroutine ระบบจะเปิดภาพเคลื่อนไหวพร้อมกัน
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { launch { alphaAnimation.animateTo(1f) } launch { yAnimation.animateTo(100f) } }
คุณสามารถใช้ updateTransition
API เพื่อใช้สถานะเดียวกันเพื่อขับเคลื่อนภาพเคลื่อนไหวของพร็อพเพอร์ตี้หลายรายการพร้อมกัน ตัวอย่างด้านล่างแสดงภาพเคลื่อนไหวของพร็อพเพอร์ตี้ 2 รายการที่ควบคุมโดยการเปลี่ยนแปลงสถานะ rect
และ borderWidth
var currentState by remember { mutableStateOf(BoxState.Collapsed) } val transition = updateTransition(currentState, label = "transition") val rect by transition.animateRect(label = "rect") { state -> when (state) { BoxState.Collapsed -> Rect(0f, 0f, 100f, 100f) BoxState.Expanded -> Rect(100f, 100f, 300f, 300f) } } val borderWidth by transition.animateDp(label = "borderWidth") { state -> when (state) { BoxState.Collapsed -> 1.dp BoxState.Expanded -> 0.dp } }
เพิ่มประสิทธิภาพของภาพเคลื่อนไหว
ภาพเคลื่อนไหวในเครื่องมือเขียนอาจทำให้เกิดปัญหาด้านประสิทธิภาพ สาเหตุเป็นเพราะลักษณะของภาพเคลื่อนไหวที่เป็นการย้ายหรือเปลี่ยนพิกเซลบนหน้าจออย่างรวดเร็วทีละเฟรมเพื่อสร้างภาพลวงตาการเคลื่อนไหว
ลองพิจารณาระยะต่างๆ ขององค์ประกอบ ได้แก่ การจัดองค์ประกอบ เลย์เอาต์ และการวาด หากภาพเคลื่อนไหวเปลี่ยนระยะของเลย์เอาต์ ต้องใช้ Composable ที่ได้รับผลกระทบทั้งหมดเพื่อรีเลย์และวาดใหม่ หากภาพเคลื่อนไหวเกิดขึ้นในระยะวาด การแสดงภาพเคลื่อนไหวจะมีประสิทธิภาพมากกว่าโดยค่าเริ่มต้นเมื่อเทียบกับการเรียกใช้ภาพเคลื่อนไหวในระยะเลย์เอาต์ เนื่องจากมีงานน้อยลงโดยรวม
เลือกModifier
เวอร์ชัน Lambda หากเป็นไปได้ เพื่อให้แอปทำงานน้อยที่สุดขณะแสดงภาพเคลื่อนไหว ซึ่งจะข้ามการจัดองค์ประกอบใหม่และแสดงภาพเคลื่อนไหวนอกระยะการจัดองค์ประกอบ หรือใช้ Modifier.graphicsLayer{ }
เนื่องจากตัวแก้ไขนี้จะทำงานในระยะวาดเสมอ ดูข้อมูลเพิ่มเติมเกี่ยวกับเรื่องนี้ได้ในส่วนการเลื่อนการอ่านในเอกสารประกอบเกี่ยวกับประสิทธิภาพ
เปลี่ยนเวลาของภาพเคลื่อนไหว
โดยค่าเริ่มต้น เครื่องมือแต่งจะใช้ภาพเคลื่อนไหวสปริงสำหรับภาพเคลื่อนไหวส่วนใหญ่ ภาพสปริงหรือภาพเคลื่อนไหว
แบบฟิสิกส์จะเป็นธรรมชาติมากขึ้น และยังขัดจังหวะได้ด้วย
เนื่องจากจะพิจารณาความเร็วปัจจุบันของวัตถุ แทนที่จะเป็นเวลาคงที่
หากต้องการลบล้างค่าเริ่มต้น API ภาพเคลื่อนไหวทั้งหมดที่แสดงด้านบนจะตั้งค่า animationSpec
เพื่อปรับแต่งวิธีการทำงานของภาพเคลื่อนไหวได้ ไม่ว่าคุณจะต้องการให้ภาพเคลื่อนไหวทำงานเป็นระยะเวลาหนึ่งหรือให้เด้งมากขึ้น
ต่อไปนี้เป็นข้อมูลสรุปเกี่ยวกับตัวเลือกต่างๆ ของ animationSpec
:
spring
: ภาพเคลื่อนไหวตามฟิสิกส์ ซึ่งเป็นค่าเริ่มต้นสำหรับภาพเคลื่อนไหวทั้งหมด คุณเปลี่ยนค่า stiffness หรือ dampingRatio เพื่อให้ภาพเคลื่อนไหวมีรูปลักษณ์และความรู้สึกแตกต่างกันได้tween
(ย่อมาจาก between): ภาพเคลื่อนไหวที่อิงตามระยะเวลา ภาพเคลื่อนไหวระหว่าง 2 ค่าที่มีฟังก์ชันEasing
keyframes
: ข้อกำหนดสำหรับการระบุค่าในจุดสำคัญๆ ของภาพเคลื่อนไหวrepeatable
: ข้อมูลจำเพาะตามระยะเวลาที่ทำงานเป็นจํานวนครั้งที่ระบุโดยRepeatMode
infiniteRepeatable
: ข้อกำหนดตามระยะเวลาที่ทำงานตลอดไปsnap
: ข้ามไปยังค่าสุดท้ายทันทีโดยไม่มีภาพเคลื่อนไหว
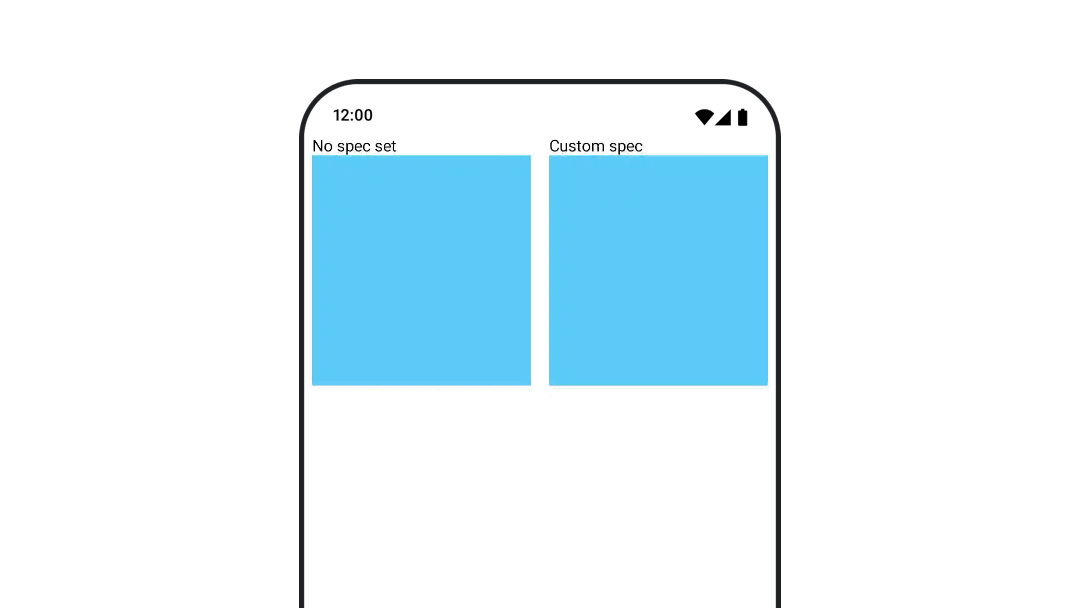
อ่านเอกสารประกอบฉบับเต็มเพื่อดูข้อมูลเพิ่มเติมเกี่ยวกับ animationSpecs
แหล่งข้อมูลเพิ่มเติม
ดูตัวอย่างภาพเคลื่อนไหวสนุกๆ เพิ่มเติมในเครื่องมือเขียนได้ที่ด้านล่าง
- ภาพเคลื่อนไหวสั้นๆ 5 แบบใน Compose
- การทำให้แมงกะพรุนเคลื่อนไหวใน Compose
- การปรับแต่ง
AnimatedContent
ในเขียน - การค่อยๆ เปลี่ยนฟังก์ชันการค่อยๆ เปลี่ยนใน Compose