ऐनिमेशन के इस्तेमाल के सामान्य उदाहरणों को मैनेज करने के लिए, कंपोज़ेबल में पहले से मौजूद कंपोज़ेबल और मॉडिफ़ायर की सुविधा मिलती है.
पहले से मौजूद, ऐनिमेटेड कंपोज़ेबल
AnimatedVisibility
की मदद से, अपनी स्क्रीन पर कॉन्टेंट के दिखने और गायब होने से जुड़ी जानकारी पाएं
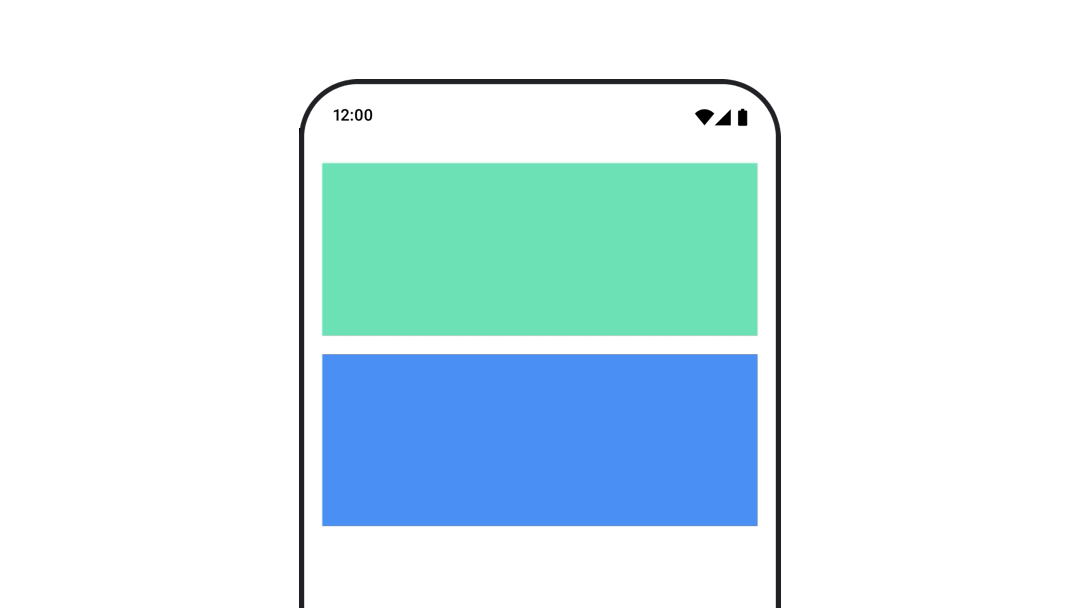
कॉन्टेंट बनाने
AnimatedVisibility
इस तरह के कॉन्टेंट से, कॉन्टेंट के दिखने और गायब होने का ऐनिमेशन तैयार होता है.
var visible by remember { mutableStateOf(true) } // Animated visibility will eventually remove the item from the composition once the animation has finished. AnimatedVisibility(visible) { // your composable here // ... }
डिफ़ॉल्ट रूप से, कॉन्टेंट फ़ेड इन और बड़ा होता हुआ दिखता है और इतनी देर के बाद दिखना बंद हो जाता है
धीरे-धीरे खत्म हो रहा है और सिकुड़ने लगा है. इस ट्रांज़िशन को अपनी ज़रूरत के हिसाब से,
EnterTransition
और
ExitTransition
.
var visible by remember { mutableStateOf(true) } val density = LocalDensity.current AnimatedVisibility( visible = visible, enter = slideInVertically { // Slide in from 40 dp from the top. with(density) { -40.dp.roundToPx() } } + expandVertically( // Expand from the top. expandFrom = Alignment.Top ) + fadeIn( // Fade in with the initial alpha of 0.3f. initialAlpha = 0.3f ), exit = slideOutVertically() + shrinkVertically() + fadeOut() ) { Text("Hello", Modifier.fillMaxWidth().height(200.dp)) }
जैसा कि ऊपर दिए गए उदाहरण में देखा जा सकता है, एक से ज़्यादा EnterTransition
को जोड़ा जा सकता है
या +
ऑपरेटर वाले ExitTransition
ऑब्जेक्ट और हर वैकल्पिक ऑपरेटर को अनुमति देता है
पैरामीटर का इस्तेमाल करें. ज़्यादा जानकारी के लिए, रेफ़रंस देखें.
EnterTransition
और ExitTransition
के उदाहरण
ट्रांज़िशन शुरू करें | ट्रांज़िशन से बाहर निकलें |
---|---|
fadeIn
![]() |
fadeOut
![]() |
slideIn
![]() |
slideOut
![]() |
slideInHorizontally
![]() |
slideOutHorizontally
![]() |
slideInVertically
![]() |
slideOutVertically
![]() |
scaleIn
![]() |
scaleOut
![]() |
expandIn
![]() |
shrinkOut
![]() |
expandHorizontally
![]() |
shrinkHorizontally
![]() |
expandVertically
![]() |
shrinkVertically
![]() |
AnimatedVisibility
में एक ऐसा वैरिएंट भी मिलता है जो
MutableTransitionState
. इससे आप एनीमेशन को तुरंत स्ट्रीम कर सकते हैं
AnimatedVisibility
को कंपोज़िशन ट्री में जोड़ा गया. यह इन कामों के लिए भी फ़ायदेमंद है
ऐनिमेशन की स्थिति देखी जा रही है.
// Create a MutableTransitionState<Boolean> for the AnimatedVisibility. val state = remember { MutableTransitionState(false).apply { // Start the animation immediately. targetState = true } } Column { AnimatedVisibility(visibleState = state) { Text(text = "Hello, world!") } // Use the MutableTransitionState to know the current animation state // of the AnimatedVisibility. Text( text = when { state.isIdle && state.currentState -> "Visible" !state.isIdle && state.currentState -> "Disappearing" state.isIdle && !state.currentState -> "Invisible" else -> "Appearing" } ) }
बच्चों के लिए अंदर और बाहर जाने का ऐनिमेशन
AnimatedVisibility
में शामिल कॉन्टेंट (सीधे तौर पर या अन्य तरीके से बच्चे)
animateEnterExit
मॉडिफ़ायर का इस्तेमाल करें. विज़ुअल
इनमें से हर बच्चे पर इन चीज़ों का असर, इन ऐनिमेशन के ज़रिए दिया गया है
AnimatedVisibility
कंपोज़ेबल में सबमिट किया गया हो और बच्चे ने खुद ही लॉग इन किया हो और
ऐनिमेशन बंद करें.
var visible by remember { mutableStateOf(true) } AnimatedVisibility( visible = visible, enter = fadeIn(), exit = fadeOut() ) { // Fade in/out the background and the foreground. Box(Modifier.fillMaxSize().background(Color.DarkGray)) { Box( Modifier .align(Alignment.Center) .animateEnterExit( // Slide in/out the inner box. enter = slideInVertically(), exit = slideOutVertically() ) .sizeIn(minWidth = 256.dp, minHeight = 64.dp) .background(Color.Red) ) { // Content of the notification… } } }
कुछ मामलों में, हो सकता है कि आप AnimatedVisibility
को कोई ऐनिमेशन लागू न करना चाहें
इससे बच्चों को एक अलग ऐनिमेशन बनाने की सुविधा मिलती है.
animateEnterExit
. इसके लिए, EnterTransition.None
और
AnimatedVisibility
कंपोज़ेबल में ExitTransition.None
.
कस्टम ऐनिमेशन जोड़ें
अगर आपको ऐप्लिकेशन में पहले से मौजूद एंट्री और एग्ज़िट के अलावा, कस्टम ऐनिमेशन इफ़ेक्ट जोड़ना है, तो
ऐनिमेशन, transition
के ज़रिए दिए गए Transition
इंस्टेंस को ऐक्सेस करें
AnimatedVisibility
के लिए सामग्री lambda के अंदर प्रॉपर्टी. कोई भी ऐनिमेशन
ट्रांज़िशन इंस्टेंस में जोड़ी गई स्थितियां, Enter के साथ एक साथ चलेंगी
और AnimatedVisibility
के ऐनिमेशन से बाहर निकलें. AnimatedVisibility
तक इंतज़ार करें
Transition
के सभी एनिमेशन इसकी सामग्री हटाने से पहले खत्म हो गए हैं.
Transition
से अलग बनाए गए एग्ज़िट ऐनिमेशन के लिए (जैसे, इस्तेमाल करके
animate*AsState
), AnimatedVisibility
उनके लिए ज़िम्मेदार नहीं होगा,
इसलिए, कॉन्टेंट अपलोड करने की प्रोसेस पूरी होने से पहले ही उसे हटाया जा सकता है.
var visible by remember { mutableStateOf(true) } AnimatedVisibility( visible = visible, enter = fadeIn(), exit = fadeOut() ) { // this: AnimatedVisibilityScope // Use AnimatedVisibilityScope#transition to add a custom animation // to the AnimatedVisibility. val background by transition.animateColor(label = "color") { state -> if (state == EnterExitState.Visible) Color.Blue else Color.Gray } Box(modifier = Modifier.size(128.dp).background(background)) }
Transition
के बारे में ज़्यादा जानकारी के लिए, updatetransit देखें.
AnimatedContent
के साथ टारगेट की स्थिति के हिसाब से ऐनिमेट करें
AnimatedContent
कंपोज़ेबल में कॉन्टेंट देखते समय, शॉर्ट वीडियो में दिखाए गए
टारगेट की स्थिति.
Row { var count by remember { mutableStateOf(0) } Button(onClick = { count++ }) { Text("Add") } AnimatedContent(targetState = count) { targetCount -> // Make sure to use `targetCount`, not `count`. Text(text = "Count: $targetCount") } }
ध्यान दें कि आपको हमेशा lambda पैरामीटर का इस्तेमाल करना चाहिए और इसे कॉन्टेंट. एपीआई इस वैल्यू का इस्तेमाल कुंजी के तौर पर, ऐसे कॉन्टेंट की पहचान करने के लिए करता है अभी दिखाया गया है.
डिफ़ॉल्ट रूप से, शुरुआती कॉन्टेंट फ़ेड आउट हो जाता है और फिर टारगेट किया गया कॉन्टेंट फ़ेड इन हो जाता है
(इस व्यवहार को फ़ेड थ्रू कहा जाता है). आपने लोगों तक पहुंचाया मुफ़्त में
इस एनीमेशन के व्यवहार को ContentTransform
ऑब्जेक्ट को
transitionSpec
पैरामीटर. आप एक-दूसरे से जोड़कर ContentTransform
बना सकते हैं
EnterTransition
ExitTransition
के साथ
with
इन्फ़िक्स फ़ंक्शन का इस्तेमाल करके. SizeTransform
को लागू किया जा सकता है
ContentTransform
के साथ अटैच करके
using
इन्फ़िक्स फ़ंक्शन.
AnimatedContent( targetState = count, transitionSpec = { // Compare the incoming number with the previous number. if (targetState > initialState) { // If the target number is larger, it slides up and fades in // while the initial (smaller) number slides up and fades out. slideInVertically { height -> height } + fadeIn() with slideOutVertically { height -> -height } + fadeOut() } else { // If the target number is smaller, it slides down and fades in // while the initial number slides down and fades out. slideInVertically { height -> -height } + fadeIn() with slideOutVertically { height -> height } + fadeOut() }.using( // Disable clipping since the faded slide-in/out should // be displayed out of bounds. SizeTransform(clip = false) ) } ) { targetCount -> Text(text = "$targetCount") }
EnterTransition
तय करता है कि टारगेट कॉन्टेंट कैसा दिखना चाहिए, और
ExitTransition
बताता है कि शुरुआती कॉन्टेंट किस तरह गायब होना चाहिए. इसके अलावा
इसके लिए उपलब्ध सभी EnterTransition
और ExitTransition
फ़ंक्शन का
AnimatedVisibility
, AnimatedContent
ऑफ़र slideIntoContainer
और slideOutOfContainer
.
ये slideInHorizontally/Vertically
और
slideOutHorizontally/Vertically
जो इसके आधार पर स्लाइड की दूरी का हिसाब लगाता है
शुरुआती कॉन्टेंट का साइज़ और टारगेट किया गया कॉन्टेंट
AnimatedContent
कॉन्टेंट.
SizeTransform
तय करता है कि
साइज़, शुरुआती और टारगेट कॉन्टेंट के बीच ऐनिमेट होना चाहिए. आपके बकाया पैसे
बनाते समय शुरुआती साइज़ और टारगेट साइज़, दोनों को ऐक्सेस किया जा सकता है
ऐनिमेशन. SizeTransform
यह भी कंट्रोल करता है कि कॉन्टेंट से क्लिप बनाई जानी चाहिए या नहीं
कॉम्पोनेंट के साइज़ में बदलाव कर देते हैं.
var expanded by remember { mutableStateOf(false) } Surface( color = MaterialTheme.colorScheme.primary, onClick = { expanded = !expanded } ) { AnimatedContent( targetState = expanded, transitionSpec = { fadeIn(animationSpec = tween(150, 150)) with fadeOut(animationSpec = tween(150)) using SizeTransform { initialSize, targetSize -> if (targetState) { keyframes { // Expand horizontally first. IntSize(targetSize.width, initialSize.height) at 150 durationMillis = 300 } } else { keyframes { // Shrink vertically first. IntSize(initialSize.width, targetSize.height) at 150 durationMillis = 300 } } } } ) { targetExpanded -> if (targetExpanded) { Expanded() } else { ContentIcon() } } }
बच्चे के अंदर और बाहर निकलने के ट्रांज़िशन को ऐनिमेट करें
AnimatedVisibility
की तरह ही, animateEnterExit
कार्रवाई बदलने वाली कुंजी, AnimatedContent
के कॉन्टेंट lambda के अंदर उपलब्ध है. इसका इस्तेमाल करें
हर डायरेक्ट या इनडायरेक्ट ट्रैफ़िक पर EnterAnimation
और ExitAnimation
लागू करने के लिए
बच्चों को अलग-अलग रखने के लिए.
कस्टम ऐनिमेशन जोड़ें
AnimatedVisibility
की तरह ही, transition
फ़ील्ड
AnimatedContent
का कॉन्टेंट lambda. कस्टम ऐनिमेशन बनाने के लिए इसका इस्तेमाल करें
यह इफ़ेक्ट AnimatedContent
ट्रांज़िशन के साथ-साथ चलता है. यहां जाएं:
ज़्यादा जानकारी के लिए, updatetransit पर जाएं.
Crossfade
के साथ दो लेआउट के बीच ऐनिमेट करें
Crossfade
, क्रॉसफ़ेड ऐनिमेशन के साथ दो लेआउट के बीच ऐनिमेट होता है. टॉगल करके
current
पैरामीटर को पास की गई वैल्यू, कॉन्टेंट को
क्रॉसफ़ेड ऐनिमेशन भी दिखाता है.
var currentPage by remember { mutableStateOf("A") } Crossfade(targetState = currentPage) { screen -> when (screen) { "A" -> Text("Page A") "B" -> Text("Page B") } }
पहले से मौजूद ऐनिमेशन मॉडिफ़ायर
animateContentSize
की मदद से, कंपोज़ेबल साइज़ में होने वाले बदलावों को ऐनिमेट करें
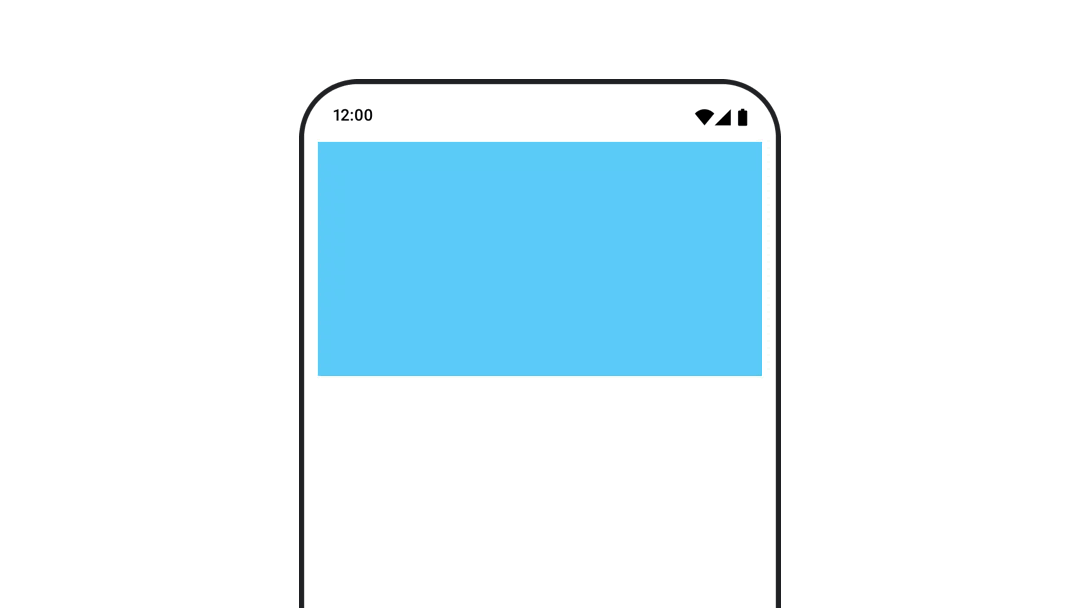
animateContentSize
मॉडिफ़ायर, साइज़ में बदलाव को ऐनिमेट करता है.
var expanded by remember { mutableStateOf(false) } Box( modifier = Modifier .background(colorBlue) .animateContentSize() .height(if (expanded) 400.dp else 200.dp) .fillMaxWidth() .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { expanded = !expanded } ) { }
सूची आइटम के ऐनिमेशन
अगर आपको लेज़ी लिस्ट या ग्रिड में, आइटम का क्रम बदलने की सुविधा को ऐनिमेट करना है, तो लेज़ी लेआउट आइटम के ऐनिमेशन से जुड़े दस्तावेज़.
आपके लिए सुझाव
- ध्यान दें: JavaScript बंद होने पर लिंक टेक्स्ट दिखता है
- वैल्यू के हिसाब से ऐनिमेशन
- Compose में ऐनिमेशन
- ऐनिमेशन टूल की सुविधा {:#tooling}