Nút hành động nổi (FAB) là một nút tròn kích hoạt hành động chính trong giao diện người dùng của ứng dụng. Tài liệu này trình bày cách thêm một FAB vào bố cục, tuỳ chỉnh một số giao diện của ứng dụng và phản hồi khi nhấn nút.
Để tìm hiểu thêm về cách thiết kế FAB cho ứng dụng theo Nguyên tắc thiết kế Material Design, hãy xem phần FAB Material Design.
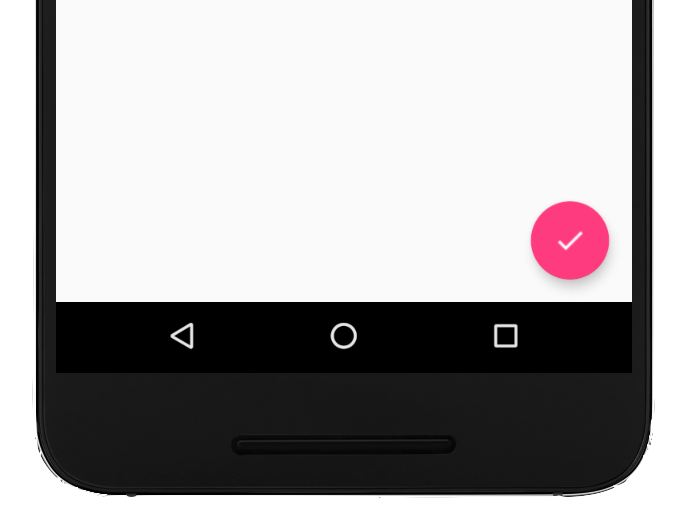
Thêm nút hành động nổi vào bố cục
Đoạn mã sau cho biết cách FloatingActionButton
xuất hiện trong tệp bố cục:
<com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/fab" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="end|bottom" android:src="@drawable/ic_my_icon" android:contentDescription="@string/submit" android:layout_margin="16dp" />
Theo mặc định, FAB được tô màu theo thuộc tính colorAccent
, bạn có thể tuỳ chỉnh bằng bảng màu của giao diện.
Bạn có thể định cấu hình các thuộc tính FAB khác bằng các thuộc tính XML hoặc phương thức tương ứng, chẳng hạn như sau:
- Kích thước của FAB, sử dụng thuộc tính
app:fabSize
hoặc phương thứcsetSize()
- Màu gợn sóng của FAB, sử dụng thuộc tính
app:rippleColor
hoặc phương thứcsetRippleColor()
- Biểu tượng FAB, sử dụng thuộc tính
android:src
hoặc phương thứcsetImageDrawable()
Phản hồi khi nhấn nút
Sau đó, bạn có thể áp dụng View.OnClickListener
để xử lý các lượt nhấn vào FAB. Ví dụ: mã sau đây hiển thị một Snackbar
khi người dùng nhấn vào FAB:
Kotlin
val fab: View = findViewById(R.id.fab) fab.setOnClickListener { view -> Snackbar.make(view, "Here's a Snackbar", Snackbar.LENGTH_LONG) .setAction("Action", null) .show() }
Java
FloatingActionButton fab = findViewById(R.id.fab); fab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Snackbar.make(view, "Here's a Snackbar", Snackbar.LENGTH_LONG) .setAction("Action", null).show(); } });
Để biết thêm thông tin về các tính năng của FAB, hãy xem tài liệu tham khảo về API cho FloatingActionButton
.