Có trong Android 9 (API cấp 28) trở lên, tiện ích phóng to là một
kính lúp ảo cho thấy bản sao phóng to của View
thông qua một
ngăn lớp phủ thể hiện ống kính. Tính năng này cải thiện tính năng chèn văn bản
và lựa chọn trải nghiệm người dùng. Khi áp dụng trình phóng to cho văn bản, người dùng có thể
định vị chính xác con trỏ hoặc các ô điều khiển lựa chọn bằng cách xem phóng to
trong ngăn theo dõi ngón tay của họ.
Hình 1 cho thấy cách kính lúp hỗ trợ việc chọn văn bản. API trình phóng to không gắn liền với văn bản và bạn có thể sử dụng tiện ích này trong nhiều trường hợp sử dụng, chẳng hạn như như đọc văn bản nhỏ hoặc phóng to tên địa điểm khó nhìn thấy trên bản đồ.
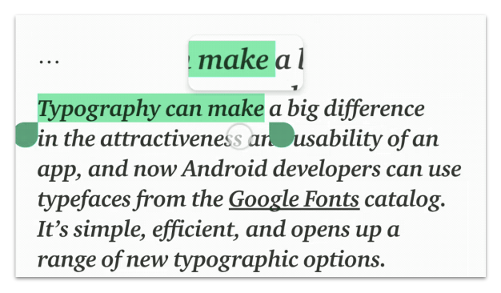
Trình phóng to đã được tích hợp với các tiện ích nền tảng như TextView
,
EditText
và WebView
. Giúp thao tác văn bản nhất quán trên các ứng dụng.
Tiện ích này đi kèm một API đơn giản và có thể dùng để phóng to bất kỳ View
nào
tuỳ thuộc vào ngữ cảnh của ứng dụng.
Sử dụng API
Bạn có thể dùng trình phóng to theo phương thức lập trình ở chế độ xem tuỳ ý như sau:
Kotlin
val view: View = findViewById(R.id.view) val magnifier = Magnifier.Builder(view).build() magnifier.show(view.width / 2.0f, view.height / 2.0f)
Java
View view = findViewById(R.id.view); Magnifier magnifier = new Magnifier.Builder(view).build(); magnifier.show(view.getWidth() / 2, view.getHeight() / 2);
Giả sử hệ phân cấp chế độ xem có bố cục đầu tiên, trình phóng to sẽ hiển thị trên màn hình chứa một khu vực tập trung vào toạ độ đã cho trong khung hiển thị. Ngăn này xuất hiện phía trên điểm giữa của nội dung đang được sao chép. Chiến lược phát hành đĩa đơn Kính lúp vẫn hoạt động vô thời hạn cho đến khi người dùng đóng.
Đoạn mã sau đây cho biết cách thay đổi nền của tính năng phóng to chế độ xem:
Kotlin
view.setBackgroundColor(...)
Java
view.setBackgroundColor(...);
Giả sử màu nền có thể nhìn thấy trong kính lúp, thì
nội dung đã lỗi thời do một khu vực của chế độ xem vẫn có nền cũ
màn hình. Để làm mới nội dung, hãy sử dụng
update()
như sau:
Kotlin
view.post { magnifier.update() }
Java
view.post(magnifier::update);
Khi hoàn tất, hãy đóng kính lúp bằng cách gọi phương thức dismiss()
:
Kotlin
magnifier.dismiss()
Java
magnifier.dismiss();
Phóng to khi người dùng tương tác
Một trường hợp sử dụng phổ biến của kính lúp là cho phép người dùng phóng to khu vực xem bằng cách chạm vào thiết bị đó, như minh hoạ trong hình 2.
ViewGroup
có chứa "ImageView" ở bên trái
và một TextView
ở bên phải.Bạn có thể thực hiện việc này bằng cách cập nhật kính lúp theo sự kiện chạm nhận được theo chế độ xem, như sau:
Kotlin
imageView.setOnTouchListener { v, event -> when (event.actionMasked) { MotionEvent.ACTION_DOWN, MotionEvent.ACTION_MOVE -> { val viewPosition = IntArray(2) v.getLocationOnScreen(viewPosition) magnifier.show(event.rawX - viewPosition[0], event.rawY - viewPosition[1]) } MotionEvent.ACTION_CANCEL, MotionEvent.ACTION_UP -> { magnifier.dismiss() } } true }
Java
imageView.setOnTouchListener(new View.OnTouchListener() { @Override public boolean onTouch(View v, MotionEvent event) { switch (event.getActionMasked()) { case MotionEvent.ACTION_DOWN: // Fall through. case MotionEvent.ACTION_MOVE: { final int[] viewPosition = new int[2]; v.getLocationOnScreen(viewPosition); magnifier.show(event.getRawX() - viewPosition[0], event.getRawY() - viewPosition[1]); break; } case MotionEvent.ACTION_CANCEL: // Fall through. case MotionEvent.ACTION_UP: { magnifier.dismiss(); } } return true; } });
Những cân nhắc khác khi phóng to văn bản
Đối với các tiện ích văn bản trên nền tảng, bạn cần phải hiểu được tính năng phóng to cụ thể và để bật tính năng phóng to cho chế độ xem văn bản tuỳ chỉnh một cách nhất quán trên nền tảng Android. Hãy cân nhắc thực hiện những bước sau:
- Kính lúp sẽ được kích hoạt ngay lập tức khi người dùng tóm lấy phần chèn hoặc ô điều khiển lựa chọn.
- Kính lúp luôn di chuyển mượt mà theo chiều ngang ngón tay của người dùng, trong khi theo chiều dọc nó được cố định vào chính giữa của dòng văn bản hiện tại.
- Khi di chuyển theo chiều ngang, kính lúp chỉ di chuyển giữa bên trái và ranh giới bên phải của dòng hiện tại. Hơn nữa, khi thao tác chạm của người dùng rời khỏi các giới hạn này và khoảng cách theo chiều ngang giữa điểm chạm và điểm gần nhất giới hạn lớn hơn một nửa chiều rộng ban đầu của nội dung phóng to. trình phóng to bị đóng vì con trỏ không còn hiển thị bên trong phóng to.
- Không thể kích hoạt kính lúp khi phông chữ văn bản quá lớn. Văn bản là được coi là quá lớn khi sự khác biệt giữa độ lệch chuẩn của phông chữ và độ cao lớn hơn chiều cao của nội dung vừa với trình phóng to. Việc kích hoạt trình phóng to trong trường hợp này không làm tăng thêm giá trị.