Niektóre treści najlepiej oglądać na pełnym ekranie bez żadnych wskaźników na pasku stanu lub pasku nawigacyjnym. Mogą to być na przykład filmy, gry, galerie zdjęć, książki i slajdy prezentacji. Jest to tzw. tryb immersyjny. Na tej stronie dowiesz się, jak zwiększyć zaangażowanie użytkowników w treści wyświetlane na pełnym ekranie.
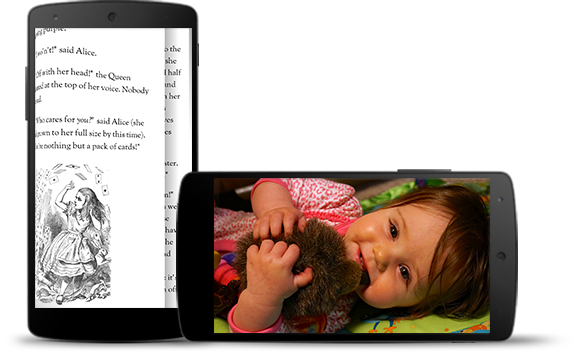
Tryb pełnoekranowy pomaga użytkownikom uniknąć przypadkowego wyjścia z gry i zapewnia wciągające wrażenia podczas oglądania zdjęć i filmów oraz czytania książek. Pamiętaj jednak, jak często użytkownicy wchodzą do aplikacji i z niej wychodzą, aby sprawdzić powiadomienia, przeprowadzić doraźne wyszukiwanie lub wykonać inne działania. Tryb pełnoekranowy utrudnia użytkownikom dostęp do nawigacji systemowej, dlatego należy go używać tylko wtedy, gdy korzyści dla użytkowników wykraczają poza zwykłe wykorzystanie dodatkowej przestrzeni na ekranie.
Użyj WindowInsetsControllerCompat.hide()
, aby ukryć paski systemowe, i WindowInsetsControllerCompat.show()
, aby je przywrócić.
Poniższy fragment kodu pokazuje przykład konfiguracji przycisku, który będzie ukrywać i wyświetlać paski systemowe.
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { ... val windowInsetsController = WindowCompat.getInsetsController(window, window.decorView) // Configure the behavior of the hidden system bars. windowInsetsController.systemBarsBehavior = WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener(window.decorView) { view, windowInsets -> // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()) } } else { binding.toggleFullscreenButton.setOnClickListener { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()) } } ViewCompat.onApplyWindowInsets(view, windowInsets) } }
Java
@Override protected void onCreate(Bundle savedInstanceState) { ... WindowInsetsControllerCompat windowInsetsController = WindowCompat.getInsetsController(getWindow(), getWindow().getDecorView()); // Configure the behavior of the hidden system bars. windowInsetsController.setSystemBarsBehavior( WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE ); // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener( getWindow().getDecorView(), (view, windowInsets) -> { // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener(v -> { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()); }); } else { binding.toggleFullscreenButton.setOnClickListener(v -> { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()); }); } return ViewCompat.onApplyWindowInsets(view, windowInsets); }); }
Opcjonalnie możesz określić typ pasków systemowych do ukrycia i ustalić ich zachowanie, gdy użytkownik wchodzi z nimi w interakcję.
Określanie, które paski systemowe mają być ukryte
Aby określić typ pasków systemowych do ukrycia, przekaż jeden z tych parametrów do funkcji WindowInsetsControllerCompat.hide()
.
Użyj
WindowInsetsCompat.Type.systemBars()
, aby ukryć oba paski systemowe.Użyj
WindowInsetsCompat.Type.statusBars()
, aby ukryć tylko pasek stanu.Użyj
WindowInsetsCompat.Type.navigationBars()
, aby ukryć tylko pasek nawigacyjny.
Określanie zachowania ukrytych pasków systemowych
Użyj WindowInsetsControllerCompat.setSystemBarsBehavior()
, aby określić, jak ukryte paski systemowe mają się zachowywać, gdy użytkownik wchodzi z nimi w interakcję.
Użyj
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_TOUCH
, aby wyświetlić ukryte paski systemowe podczas dowolnych interakcji użytkownika na odpowiednim wyświetlaczu.Użyj
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_SWIPE
, aby wyświetlić ukryte paski systemowe podczas wykonywania dowolnych gestów systemowych, np. przesuwania palcem od krawędzi ekranu, na której jest ukryty pasek.Użyj
WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE
, aby tymczasowo wyświetlić ukryte paski systemowe za pomocą gestów systemowych, np. przesuwając palcem od krawędzi ekranu, na której jest ukryty pasek. Te przejściowe paski systemowe nakładają się na treść aplikacji, mogą być częściowo przezroczyste i są automatycznie ukrywane po krótkim czasie.