Mit der EmojiCompat
-Supportbibliothek sollen Android-Geräte immer mit den neuesten Emojis auf dem neuesten Stand gehalten werden. So wird verhindert, dass in Ihrer App fehlende Emoji-Zeichen in Form von ☐ angezeigt werden, was darauf hinweist, dass auf Ihrem Gerät keine Schriftart für die Anzeige des Texts vorhanden ist. Wenn Sie die EmojiCompat
-Supportbibliothek verwenden, müssen Ihre App-Nutzer nicht auf Android-Betriebssystemupdates warten, um die neuesten Emojis zu erhalten.
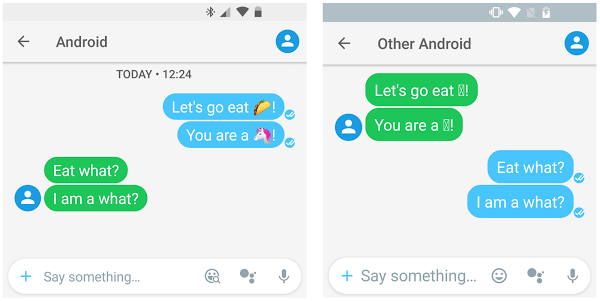
Weitere Informationen finden Sie unter den folgenden Links:
Wie funktioniert EmojiCompat?
Die EmojiCompat
-Supportbibliothek bietet Klassen zur Implementierung einer abwärtskompatiblen Emoji-Unterstützung auf Geräten mit Android 4.4 (API-Level 19) und höher. Sie können EmojiCompat
entweder mit im Lieferumfang enthaltenen oder herunterladbaren Schriftarten konfigurieren. Weitere Informationen zur Konfiguration finden Sie in den folgenden Abschnitten:
EmojiCompat
identifiziert Emojis für eine bestimmte CharSequence
, ersetzt sie bei Bedarf durch EmojiSpans
und rendert schließlich die Emoji-Glyphen. Abbildung 2 veranschaulicht den Vorgang.
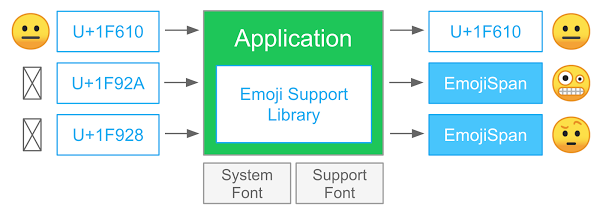
Konfiguration für herunterladbare Schriftarten
Bei der Konfiguration der herunterladbaren Schriftarten wird die Bibliotheksfunktion „Herunterladbare Schriftarten“ verwendet, um eine Emoji-Schriftart herunterzuladen. Außerdem werden die erforderlichen Emoji-Metadaten aktualisiert, die die EmojiCompat
-Unterstützungsbibliothek benötigt, um mit den neuesten Versionen der Unicode-Spezifikation Schritt zu halten.
Abhängigkeit von der Supportbibliothek hinzufügen
Wenn Sie die EmojiCompat
-Unterstützungsbibliothek verwenden möchten, müssen Sie die ClassPath-Abhängigkeiten Ihres App-Projekts in Ihrer Entwicklungsumgebung ändern.
So fügen Sie Ihrem Anwendungsprojekt eine Supportbibliothek hinzu:
- Öffnen Sie die
build.gradle
-Datei Ihrer Anwendung. - Fügen Sie die Supportbibliothek dem Abschnitt
dependencies
hinzu.
Groovy
dependencies { ... implementation "androidx.emoji:emoji:28.0.0" }
Kotlin
dependencies { ... implementation("androidx.emoji:emoji:28.0.0") }
Konfiguration der herunterladbaren Schriftart initialisieren
Sie müssen EmojiCompat
initialisieren, um die Metadaten und die Schriftart zu laden. Da die Initialisierung einige Zeit dauern kann, wird der Initialisierungsprozess in einem Hintergrund-Thread ausgeführt.
So initialisieren Sie EmojiCompat
mit der herunterladbaren Schriftkonfiguration:
- Erstellen Sie eine Instanz der Klasse
FontRequest
und geben Sie die Zertifizierungsstelle des Schriftanbieters, das Paket des Schriftanbieters, die Schriftabfrage und eine Liste von Hash-Sätzen für das Zertifikat an. Weitere Informationen zuFontRequest
finden Sie im Abschnitt Herunterladbare Schriftarten programmatisch verwenden der Dokumentation zu Herunterladbare Schriftarten. - Erstellen Sie eine Instanz von
FontRequestEmojiCompatConfig
und geben Sie Instanzen vonContext
undFontRequest
an. - Initialisieren Sie
EmojiCompat
, indem Sie die Methodeinit()
aufrufen und die Instanz vonFontRequestEmojiCompatConfig
übergeben. - Verwenden Sie
EmojiCompat
-Widgets in Layout-XMLs. Wenn SieAppCompat
verwenden, lesen Sie den Abschnitt EmojiCompat-Widgets mit AppCompat verwenden.
Kotlin
class MyApplication : Application() { override fun onCreate() { super.onCreate() val fontRequest = FontRequest( "com.example.fontprovider", "com.example", "emoji compat Font Query", CERTIFICATES ) val config = FontRequestEmojiCompatConfig(this, fontRequest) EmojiCompat.init(config) } }
Java
public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); FontRequest fontRequest = new FontRequest( "com.example.fontprovider", "com.example", "emoji compat Font Query", CERTIFICATES); EmojiCompat.Config config = new FontRequestEmojiCompatConfig(this, fontRequest); EmojiCompat.init(config); } }
<android.support.text.emoji.widget.EmojiTextView android:layout_width="wrap_content" android:layout_height="wrap_content"/> <android.support.text.emoji.widget.EmojiEditText android:layout_width="wrap_content" android:layout_height="wrap_content"/> <android.support.text.emoji.widget.EmojiButton android:layout_width="wrap_content" android:layout_height="wrap_content"/>
Weitere Informationen zum Konfigurieren von EmojiCompat
mit der herunterladbaren Schriftkonfiguration finden Sie in der Beispiel-App zur Emoji-Kompatibilität Java
| Kotlin.
Bibliothekskomponenten
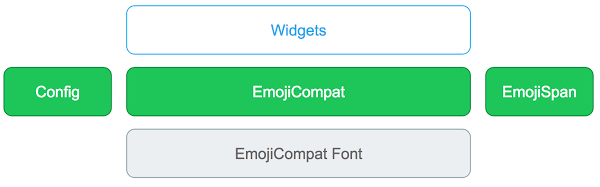
- Widgets:
EmojiEditText
,EmojiTextView
,EmojiButton
- Standard-Widget-Implementierungen, um
EmojiCompat
mitTextView
,EditText
undButton
zu verwenden. EmojiCompat
- Hauptoberfläche der Supportbibliothek. Er führt alle externen Aufrufe aus und koordiniert sich mit den anderen Teilen des Systems.
EmojiCompat.Config
- Konfiguriert die zu erstellende Singleton-Instanz.
EmojiSpan
- Eine
ReplacementSpan
-Unterklasse, die das Zeichen (die Zeichensequenz) ersetzt und das Schriftzeichen rendert. EmojiCompat
SchriftartEmojiCompat
verwendet eine Schriftart, um Emojis anzuzeigen. Diese Schriftart ist eine modifizierte Version der Android-Emoji-Schriftart. Die Schriftart wird so geändert:- Für die Abwärtskompatibilität beim Rendern von Emojis werden alle Emoji-Zeichen mit einem einzelnen Unicode-Codepunkt im Supplemental Private Use Area-A von Unicode dargestellt, beginnend mit U+F0001.
-
Zusätzliche Emoji-Metadaten werden in einem Binärformat in die Schriftart eingefügt und bei der Laufzeit von
EmojiCompat
geparst. Die Daten sind in dermeta
-Tabelle der Schriftart mit dem privaten Tag Emji eingebettet.
Konfigurationsoptionen
Mit der EmojiCompat
-Instanz können Sie das Verhalten von EmojiCompat
ändern. Sie können die folgenden Methoden aus der Basisklasse verwenden, um die Konfiguration festzulegen:
setReplaceAll()
: Bestimmt, obEmojiCompat
alle gefundenen Emojis durchEmojiSpans
ersetzen soll. Standardmäßig versuchtEmojiCompat
, herauszufinden, ob das System ein Emoji darstellen kann, und ersetzt diese Emojis nicht. Wenntrue
festgelegt ist, ersetztEmojiCompat
alle gefundenen Emojis durchEmojiSpans
.setEmojiSpanIndicatorEnabled()
: Gibt an, obEmojiCompat
ein Emoji durch einEmojiSpan
ersetzt hat. Wenn dieser Parameter auftrue
gesetzt ist, zeichnetEmojiCompat
einen Hintergrund für dieEmojiSpan
. Diese Methode wird hauptsächlich zum Debugging verwendet.setEmojiSpanIndicatorColor()
: Legt die Farbe für eineEmojiSpan
fest. Der Standardwert istGREEN
.registerInitCallback
: Informiert die App über den Status derEmojiCompat
-Initialisierung.
Kotlin
val config = FontRequestEmojiCompatConfig(...) .setReplaceAll(true) .setEmojiSpanIndicatorEnabled(true) .setEmojiSpanIndicatorColor(Color.GREEN) .registerInitCallback(object: EmojiCompat.InitCallback() { ... })
Java
EmojiCompat.Config config = new FontRequestEmojiCompatConfig(...) .setReplaceAll(true) .setEmojiSpanIndicatorEnabled(true) .setEmojiSpanIndicatorColor(Color.GREEN) .registerInitCallback(new InitCallback() {...})
Initialisierungs-Listener hinzufügen
Die Klassen EmojiCompat
und EmojiCompat
bieten die Methoden registerInitCallback()
und unregisterInitCallback()
, um einen Initialisierungs-Callback zu registrieren. Wenn Sie diese Methoden verwenden möchten, erstellen Sie eine Instanz der Klasse EmojiCompat.InitCallback
. Rufen Sie diese Methoden auf und übergeben Sie die Instanz der EmojiCompat.InitCallback
-Klasse. Wenn die Initialisierung der EmojiCompat
-Unterstützungsbibliothek erfolgreich war, ruft die EmojiCompat
-Klasse die Methode onInitialized()
auf. Wenn die Bibliothek nicht initialisiert werden kann, ruft die EmojiCompat
-Klasse die Methode onFailed()
auf.
Wenn Sie den Initialisierungsstatus jederzeit prüfen möchten, rufen Sie die Methode getLoadState()
auf. Es wird einer der folgenden Werte zurückgegeben: LOAD_STATE_LOADING
, LOAD_STATE_SUCCEEDED
oder LOAD_STATE_FAILED
.
EmojiCompat mit AppCompat-Widgets verwenden
Wenn Sie AppCompat widgets
verwenden, können Sie EmojiCompat
-Widgets verwenden, die von AppCompat widgets
abgeleitet sind.
- Fügen Sie die Supportbibliothek dem Abschnitt „Abhängigkeiten“ hinzu.
Groovy
dependencies { ... implementation "androidx.emoji:emoji-bundled:$version" }
Kotlin
dependencies { implementation("androidx.emoji:emoji-appcompat:$version") }
Groovy
dependencies { implementation "androidx.emoji:emoji-appcompat:$version" }
- Verwende
EmojiCompat
AppCompat Widget
-Widgets in Layout-XMLs.
<android.support.text.emoji.widget.EmojiAppCompatTextView android:layout_width="wrap_content" android:layout_height="wrap_content"/> <android.support.text.emoji.widget.EmojiAppCompatEditText android:layout_width="wrap_content" android:layout_height="wrap_content"/> <android.support.text.emoji.widget.EmojiAppCompatButton android:layout_width="wrap_content" android:layout_height="wrap_content"/>
Konfiguration der bereitgestellten Schriftarten
Die EmojiCompat
-Unterstützungsbibliothek ist auch als Paket mit Fonts verfügbar. Dieses Paket enthält die Schriftart mit den eingebetteten Metadaten. Das Paket enthält außerdem eine BundledEmojiCompatConfig
, mit der die Metadaten und Schriftarten über die AssetManager
geladen werden.
Hinweis:Die Größe der Schrift wird in mehreren Megabyte angegeben.
Abhängigkeit von der Supportbibliothek hinzufügen
Wenn Sie die EmojiCompat
-Unterstützungsbibliothek mit der bereitgestellten Schriftkonfiguration verwenden möchten, müssen Sie die Pfadabhängigkeiten Ihres App-Projekts in Ihrer Entwicklungsumgebung ändern.
So fügen Sie Ihrem Anwendungsprojekt eine Supportbibliothek hinzu:
- Öffnen Sie die
build.gradle
-Datei Ihrer Anwendung. - Fügen Sie die Supportbibliothek dem Abschnitt
dependencies
hinzu.
Groovy
dependencies { ... implementation "androidx.emoji:emoji:28.0.0" }
Kotlin
dependencies { ... implementation("androidx.emoji:emoji:28.0.0") }
Mithilfe von bereitgestellten Schriftarten EmojiCompat konfigurieren
So konfigurierst du EmojiCompat
mithilfe von bereitgestellten Schriftarten:
- Verwenden Sie
BundledEmojiCompatConfig
, um eine Instanz vonEmojiCompat
zu erstellen, und geben Sie eine Instanz vonContext
an. - Rufen Sie die Methode
init()
auf, umEmojiCompat
zu initialisieren und die Instanz vonBundledEmojiCompatConfig
zu übergeben.
Kotlin
class MyApplication : Application() { override fun onCreate() { super.onCreate() val config = BundledEmojiCompatConfig(this) EmojiCompat.init(config) } }
Java
public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); EmojiCompat.Config config = new BundledEmojiCompatConfig(this); EmojiCompat.init(config); ... } }
EmojiCompat ohne Widgets verwenden
EmojiCompat
verwendet EmojiSpan
, um korrekte Bilder zu rendern.
Daher muss jede CharSequence
in Spanned
-Instanzen mit EmojiSpans
umgewandelt werden. Die Klasse EmojiCompat
bietet eine Methode, mit der CharSequences
-Objekte mit EmojiSpans
in Spanned
-Instanzen umgewandelt werden können. Mit dieser Methode können Sie die verarbeiteten Instanzen anstelle des Rohstrings verarbeiten und im Cache speichern, was die Leistung Ihrer Anwendung verbessert.
Kotlin
val processed = EmojiCompat.get().process("neutral face \uD83D\uDE10")
Java
CharSequence processed = EmojiCompat.get().process("neutral face \uD83D\uDE10");
EmojiCompat für Eingabemethoden verwenden
Mithilfe der EmojiCompat
-Unterstützungsbibliothek können Tastaturen die Emojis rendern, die von der Anwendung unterstützt werden, mit der sie interagieren. Mit der Methode hasEmojiGlyph()
können IMEs prüfen, ob EmojiCompat
ein Emoji rendern kann. Diese Methode nimmt eine CharSequence
eines Emojis an und gibt true
zurück, wenn EmojiCompat
das Emoji erkennen und rendern kann.
Die Tastatur kann auch die Version der EmojiCompat
-Unterstützungsbibliothek prüfen, die von der App unterstützt wird, um zu bestimmen, welche Emojis in der Palette gerendert werden sollen. Um die Version zu prüfen, muss die Tastatur prüfen, ob die folgenden Schlüssel im EditorInfo.extras
-Bundle vorhanden sind:
EDITOR_INFO_METAVERSION_KEY
EDITOR_INFO_REPLACE_ALL_KEY
Wenn der Schlüssel im Bundle vorhanden ist, entspricht der Wert der Version der Emoji-Metadaten, die von der App verwendet wird. Wenn dieser Schlüssel nicht vorhanden ist, verwendet die App EmojiCompat
nicht.
Wenn der Schlüssel vorhanden ist und auf true
gesetzt ist, hat die App die Methode SetReplaceAll()
aufgerufen. Weitere Informationen zur EmojiCompat
-Konfiguration finden Sie im Abschnitt Konfigurationsoptionen.
Nachdem die Tastatur die Schlüssel im EditorInfo.extras
-Bundle erhalten hat, kann sie die Methode hasEmojiGlyph()
verwenden, wobei metadataVersion
der Wert für EDITOR_INFO_METAVERSION_KEY
ist, um zu prüfen, ob die App ein bestimmtes Emoji rendern kann.
EmojiCompat mit benutzerdefinierten Widgets verwenden
Sie können die process()
-Methode verwenden, um die CharSequence
in Ihrer App vorzuverarbeiten und sie jedem Widget hinzuzufügen, das Spanned
-Instanzen rendern kann, z. B. TextView
. Außerdem bietet EmojiCompat
die folgenden Widget-Hilfsklassen, mit denen Sie Ihre benutzerdefinierten Widgets mit minimalem Aufwand um Emoji-Unterstützung ergänzen können.
- Beispiel für TextView
- Beispiel für EditText
Kotlin
class MyTextView(context: Context) : AppCompatTextView(context) { private val emojiTextViewHelper: EmojiTextViewHelper by lazy(LazyThreadSafetyMode.NONE) { EmojiTextViewHelper(this).apply { updateTransformationMethod() } } override fun setFilters(filters: Array<InputFilter>) { super.setFilters(emojiTextViewHelper.getFilters(filters)) } override fun setAllCaps(allCaps: Boolean) { super.setAllCaps(allCaps) emojiTextViewHelper.setAllCaps(allCaps) } }
Java
public class MyTextView extends AppCompatTextView { ... public MyTextView(Context context) { super(context); init(); } ... private void init() { getEmojiTextViewHelper().updateTransformationMethod(); } @Override public void setFilters(InputFilter[] filters) { super.setFilters(getEmojiTextViewHelper().getFilters(filters)); } @Override public void setAllCaps(boolean allCaps) { super.setAllCaps(allCaps); getEmojiTextViewHelper().setAllCaps(allCaps); } private EmojiTextViewHelper getEmojiTextViewHelper() { ... } }
Kotlin
class MyEditText(context: Context) : AppCompatEditText(context) { private val emojiEditTextHelper: EmojiEditTextHelper by lazy(LazyThreadSafetyMode.NONE) { EmojiEditTextHelper(this).also { super.setKeyListener(it.getKeyListener(keyListener)) } } override fun setKeyListener(input: KeyListener?) { input?.also { super.setKeyListener(emojiEditTextHelper.getKeyListener(it)) } } override fun onCreateInputConnection(outAttrs: EditorInfo): InputConnection { val inputConnection: InputConnection = super.onCreateInputConnection(outAttrs) return emojiEditTextHelper.onCreateInputConnection( inputConnection, outAttrs ) as InputConnection } }
Java
public class MyEditText extends AppCompatEditText { ... public MyEditText(Context context) { super(context); init(); } ... private void init() { super.setKeyListener(getEmojiEditTextHelper().getKeyListener(getKeyListener())); } @Override public void setKeyListener(android.text.method.KeyListener keyListener) { super.setKeyListener(getEmojiEditTextHelper().getKeyListener(keyListener)); } @Override public InputConnection onCreateInputConnection(EditorInfo outAttrs) { InputConnection inputConnection = super.onCreateInputConnection(outAttrs); return getEmojiEditTextHelper().onCreateInputConnection(inputConnection, outAttrs); } private EmojiEditTextHelper getEmojiEditTextHelper() { ... } }
Häufig gestellte Fragen
- Wie starte ich den Schriftartendownload?
- Wie lange dauert die Initialisierung?
- Wie viel Arbeitsspeicher belegt die EmojiCompat-Unterstützungsbibliothek?
- Kann ich EmojiCompat für eine benutzerdefinierte TextView verwenden?
- Was passiert, wenn ich Widgets in Layout-XMLs auf Geräten hinzufüge, auf denen Android 4.4 (API-Level 19) oder niedriger ausgeführt wird?
Die Emoji-Schriftarten werden bei der ersten Anfrage heruntergeladen, wenn sie nicht auf dem Gerät vorhanden sind. Die Planung des Downloads ist für die App transparent.
Nach dem Herunterladen der Schriftart dauert es etwa 150 Millisekunden, bis EmojiCompat
initialisiert ist.
Derzeit wird die Datenstruktur zum Finden des Emojis in den Arbeitsspeicher der App geladen und belegt etwa 200 KB.
Ja. EmojiCompat bietet Hilfsklassen für benutzerdefinierte Widgets. Es ist auch möglich, einen bestimmten String vorzuverarbeiten und in Spanned
umzuwandeln. Weitere Informationen zu Widget-Hilfsklassen finden Sie im Abschnitt EmojiCompat mit benutzerdefinierten Widgets verwenden.
Sie können die EmojiCompat
Supportbibliothek oder ihre Widgets in Ihre Anwendungen einbinden, die Geräte mit Android 4.4 (API-Level 19) oder niedriger unterstützen. Wenn auf einem Gerät jedoch eine Android-Version vor API-Level 19 ausgeführt wird, befinden sich EmojiCompat
und seine Widgets im Status „Kein Betrieb“. Das bedeutet, dass sich EmojiTextView
genau wie ein normaler TextView
verhält.
EmojiCompat
-Instanz; sie wechselt sofort in den Status LOAD_STATE_SUCCEEDED
, wenn Sie die Methode init()
aufrufen.
Zusätzliche Ressourcen
Weitere Informationen zur Verwendung der EmojiCompat
-Bibliothek finden Sie im Video EmojiCompat.