Bạn có thể cung cấp cụm từ tìm kiếm được đề xuất dựa trên các cụm từ tìm kiếm gần đây trong hộp thoại tìm kiếm trên Android hoặc tiện ích tìm kiếm. Ví dụ: nếu người dùng truy vấn "chó con" thì cụm từ tìm kiếm sẽ xuất hiện dưới dạng một gợi ý khi nhập lại chính truy vấn đó. Hình 1 cho thấy ví dụ về một hộp thoại tìm kiếm với truy vấn gần đây nội dung đề xuất.
Trước khi bạn bắt đầu, hãy triển khai hộp thoại tìm kiếm hoặc một tiện ích tìm kiếm cho các nội dung tìm kiếm cơ bản trong ứng dụng của bạn. Để tìm hiểu cách thực hiện, hãy xem Tạo giao diện tìm kiếm.
Thông tin cơ bản
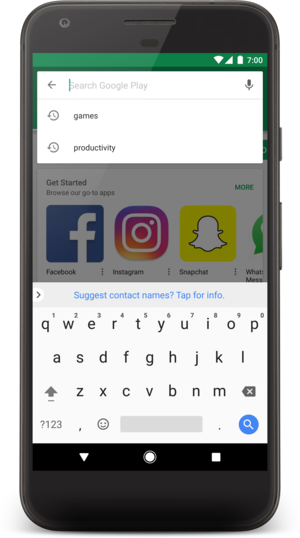
Hình 1. Ảnh chụp màn hình hộp thoại tìm kiếm với cụm từ tìm kiếm gần đây nội dung đề xuất.
Các cụm từ đề xuất gần đây là các cụm từ tìm kiếm đã lưu. Khi người dùng chọn một đề xuất, tính năng
một hoạt động nhận được một
Ý định ACTION_SEARCH
với gợi ý dưới dạng cụm từ tìm kiếm mà hoạt động có thể tìm kiếm của bạn đã xử lý.
Để đưa ra đề xuất cho các truy vấn gần đây, bạn cần:
- Triển khai một hoạt động có thể tìm kiếm.
- Tạo một trình cung cấp nội dung mở rộng
SearchRecentSuggestionsProvider
rồi khai báo mã đó trong tệp kê khai ứng dụng. - Sửa đổi cấu hình có thể tìm kiếm bằng thông tin về nhà cung cấp nội dung đã cung cấp cụm từ tìm kiếm được đề xuất.
- Lưu các truy vấn vào trình cung cấp nội dung của bạn mỗi khi thực hiện một lượt tìm kiếm.
Giống như khi hệ thống Android hiển thị hộp thoại tìm kiếm, hệ thống cũng sẽ hiện các cụm từ tìm kiếm được đề xuất dưới đây hộp thoại hoặc tiện ích tìm kiếm. Bạn cung cấp nguồn mà hệ thống truy xuất đề xuất.
Khi hệ thống xác định rằng hoạt động của bạn có thể tìm kiếm được và cung cấp đề xuất tìm kiếm, sau đây xảy ra khi người dùng nhập truy vấn:
- Hệ thống sẽ lấy văn bản cụm từ tìm kiếm (bất cứ thông tin nào người dùng bắt đầu nhập) và sẽ thực hiện truy vấn đến trình cung cấp nội dung có chứa đề xuất của bạn.
- Trình cung cấp nội dung của bạn trả về một
Cursor
trỏ đến tất cả các đề xuất phù hợp với văn bản cụm từ tìm kiếm. - Hệ thống sẽ hiện danh sách các đề xuất do
Cursor
cung cấp.
Sau khi các đề xuất truy vấn gần đây được hiển thị, những điều sau đây có thể xảy ra:
- Nếu người dùng nhập một khoá khác hoặc thay đổi truy vấn theo bất kỳ cách nào, thì các bước trước đó lặp lại và danh sách đề xuất được cập nhật.
- Nếu người dùng thực hiện tìm kiếm, đề xuất sẽ bị bỏ qua và tìm kiếm được chuyển tới
hoạt động có thể tìm kiếm bằng cách sử dụng ý định
ACTION_SEARCH
thông thường. - Nếu người dùng chọn một đề xuất, thì ý định
ACTION_SEARCH
sẽ được gửi đến hoạt động có thể tìm kiếm được bằng cách sử dụng văn bản gợi ý dưới dạng truy vấn.
Lớp SearchRecentSuggestionsProvider
mà bạn mở rộng cho trình cung cấp nội dung của mình
tự động thực hiện công việc trong các bước trước, nên có ít mã cần viết.
Tạo một trình cung cấp nội dung
Trình cung cấp nội dung bạn cần cho các đề xuất truy vấn gần đây là một cách triển khai
SearchRecentSuggestionsProvider
. Lớp học này sẽ làm mọi việc cho bạn. Bạn chỉ cần
viết một hàm khởi tạo lớp thực thi một dòng mã.
Ví dụ: dưới đây là triển khai hoàn chỉnh của trình cung cấp nội dung cho truy vấn gần đây đề xuất:
Kotlin
class MySuggestionProvider : SearchRecentSuggestionsProvider() { init { setupSuggestions(AUTHORITY, MODE) } companion object { const val AUTHORITY = "com.example.MySuggestionProvider" const val MODE: Int = SearchRecentSuggestionsProvider.DATABASE_MODE_QUERIES } }
Java
public class MySuggestionProvider extends SearchRecentSuggestionsProvider { public final static String AUTHORITY = "com.example.MySuggestionProvider"; public final static int MODE = DATABASE_MODE_QUERIES; public MySuggestionProvider() { setupSuggestions(AUTHORITY, MODE); } }
Cuộc gọi đến
setupSuggestions()
truyền tên của tổ chức phát hành tìm kiếm và chế độ cơ sở dữ liệu. Cơ quan tìm kiếm có thể là bất kỳ
nhưng cách tốt nhất là sử dụng tên đủ điều kiện cho nhà cung cấp nội dung của bạn, chẳng hạn như
tên gói theo sau là tên lớp của nhà cung cấp. Ví dụ: "com.example.MySuggestionProvider"
.
Chế độ cơ sở dữ liệu phải bao gồm
DATABASE_MODE_QUERIES
và có thể tuỳ ý bao gồm
DATABASE_MODE_2LINES
,
sẽ thêm một cột vào bảng đề xuất để bạn có thể cung cấp dòng văn bản thứ hai với mỗi
. Nếu bạn muốn cung cấp hai dòng trong mỗi đề xuất, hãy xem ví dụ sau:
Kotlin
const val MODE: Int = DATABASE_MODE_QUERIES or DATABASE_MODE_2LINES
Java
public final static int MODE = DATABASE_MODE_QUERIES | DATABASE_MODE_2LINES;
Khai báo trình cung cấp nội dung trong tệp kê khai ứng dụng với cùng một chuỗi uỷ quyền được dùng trong
lớp SearchRecentSuggestionsProvider
của bạn và trong cấu hình có thể tìm kiếm. Ví dụ:
<application> <provider android:name=".MySuggestionProvider" android:authorities="com.example.MySuggestionProvider" /> ... </application>
Sửa đổi cấu hình có thể tìm kiếm
Để định cấu hình hệ thống sử dụng nhà cung cấp đề xuất của bạn, hãy thêm thuộc tính
android:searchSuggestAuthority
và android:searchSuggestSelection
vào phần tử <searchable>
trong tệp cấu hình có thể tìm kiếm. Ví dụ:
<?xml version="1.0" encoding="utf-8"?> <searchable xmlns:android="http://schemas.android.com/apk/res/android" android:label="@string/app_label" android:hint="@string/search_hint" android:searchSuggestAuthority="com.example.MySuggestionProvider" android:searchSuggestSelection=" ?" > </searchable>
Giá trị cho android:searchSuggestAuthority
phải là tên đủ điều kiện cho
trình cung cấp nội dung khớp chính xác với uỷ quyền được sử dụng trong trình cung cấp nội dung, chẳng hạn như
"com.example.MySuggestionProvider"
trong các ví dụ trước.
Giá trị cho android:searchSuggestSelection
phải là một dấu chấm hỏi đứng trước
bởi một không gian: " ?"
. Đây là phần giữ chỗ cho đối số lựa chọn SQLite và
tự động được thay thế bằng văn bản truy vấn do người dùng nhập.
Lưu truy vấn
Để đưa các truy vấn gần đây vào bộ sưu tập của bạn, hãy thêm từng truy vấn nhận được từ các truy vấn có thể tìm kiếm
hoạt động vào SearchRecentSuggestionsProvider
của bạn. Để thực hiện việc này, hãy tạo một phiên bản của
SearchRecentSuggestions
và gọi
saveRecentQuery()
mỗi khi hoạt động có thể tìm kiếm của bạn nhận được một truy vấn. Ví dụ: dưới đây là cách bạn có thể lưu truy vấn
trong thời gian diễn ra hoạt động
onCreate()
phương thức:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.main) if (Intent.ACTION_SEARCH == intent.action) { intent.getStringExtra(SearchManager.QUERY)?.also { query -> SearchRecentSuggestions(this, MySuggestionProvider.AUTHORITY, MySuggestionProvider.MODE) .saveRecentQuery(query, null) } } }
Java
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); Intent intent = getIntent(); if (Intent.ACTION_SEARCH.equals(intent.getAction())) { String query = intent.getStringExtra(SearchManager.QUERY); SearchRecentSuggestions suggestions = new SearchRecentSuggestions(this, MySuggestionProvider.AUTHORITY, MySuggestionProvider.MODE); suggestions.saveRecentQuery(query, null); } }
Hàm khởi tạo SearchRecentSuggestionsProvider
yêu cầu
cùng một quyền và chế độ cơ sở dữ liệu do trình cung cấp nội dung của bạn khai báo.
Phương thức saveRecentQuery()
lấy chuỗi cụm từ tìm kiếm làm tham số đầu tiên
và không bắt buộc phải thêm chuỗi thứ hai làm dòng thứ hai của gợi ý hoặc chuỗi giá trị rỗng. Thứ hai
chỉ được sử dụng nếu bạn bật chế độ hai dòng cho các đề xuất tìm kiếm với
DATABASE_MODE_2LINES
. Nếu bạn bật chế độ hai dòng, thì văn bản truy vấn sẽ khớp với
dòng thứ hai khi hệ thống tìm các đề xuất phù hợp.
Xoá dữ liệu đề xuất
Để bảo vệ quyền riêng tư của người dùng, hãy luôn cung cấp cho người dùng một cách để xoá cụm từ tìm kiếm gần đây
nội dung đề xuất. Để xoá nhật ký truy vấn, hãy gọi
clearHistory()
.
Ví dụ:
Kotlin
SearchRecentSuggestions(this, HelloSuggestionsProvider.AUTHORITY, HelloSuggestionsProvider.MODE) .clearHistory()
Java
SearchRecentSuggestions suggestions = new SearchRecentSuggestions(this, HelloSuggestionProvider.AUTHORITY, HelloSuggestionProvider.MODE); suggestions.clearHistory();
Thực thi việc này theo lựa chọn "Xoá nhật ký tìm kiếm" của bạn mục trong trình đơn, mục lựa chọn ưu tiên hoặc nút. Cung cấp hộp thoại xác nhận để xác minh rằng người dùng muốn xoá nhật ký tìm kiếm của họ.