Hệ thống hoạt ảnh thuộc tính là một khung mạnh mẽ cho phép bạn để tạo ảnh động cho hầu hết nội dung. Bạn có thể xác định ảnh động để thay đổi bất kỳ thuộc tính đối tượng nào theo thời gian, bất kể nó có vẽ lên màn hình hay không. Ảnh động thuộc tính thay đổi thuộc tính (một trường trong một đối tượng) trong một khoảng thời gian nhất định. Để tạo hoạt ảnh cho nội dung nào đó, bạn chỉ định thuộc tính đối tượng mà bạn muốn tạo hiệu ứng động, chẳng hạn như vị trí của đối tượng trên màn hình, thời lượng bạn muốn tạo ảnh động và giá trị mà bạn muốn tạo ảnh động.
Hệ thống ảnh động thuộc tính cho phép bạn xác định các đặc điểm sau của một thuộc tính ảnh động:
- Thời lượng: Bạn có thể chỉ định thời lượng của ảnh động. Độ dài mặc định là 300 mili giây.
- Nội suy thời gian: Bạn có thể chỉ định cách tính giá trị cho thuộc tính dưới dạng hàm thời gian đã trôi qua hiện tại của ảnh động.
- Số lần và hành vi lặp lại: Bạn có thể chỉ định xem có lặp lại ảnh động khi ảnh động sẽ kết thúc thời lượng và số lần lặp lại ảnh động. Bạn cũng có thể chỉ định xem bạn có muốn ảnh động phát ngược lại hay không. Đặt chế độ phát ngược ảnh động tiến rồi lùi nhiều lần cho đến khi đạt đến số lần lặp lại.
- Tập hợp Trình tạo ảnh động: Bạn có thể nhóm ảnh động thành tập hợp logic để phát cùng nhau hoặc tuần tự hoặc sau độ trễ đã chỉ định.
- Độ trễ làm mới khung: Bạn có thể chỉ định tần suất làm mới khung hình của ảnh động. Chiến lược phát hành đĩa đơn được đặt mặc định là làm mới 10 mili giây một lần, nhưng tốc độ mà ứng dụng của bạn có thể làm mới khung hình là cuối cùng phụ thuộc vào mức độ bận nói chung của hệ thống cũng như tốc độ mà hệ thống có thể phục vụ bộ tính giờ cơ bản.
Để xem ví dụ đầy đủ về ảnh động thuộc tính, hãy xem
Lớp ChangeColor
trong CustomTransition
mẫu trên GitHub.
Cách hoạt động của ảnh động thuộc tính
Trước tiên, hãy tìm hiểu cách hoạt động của ảnh động qua một ví dụ đơn giản. Hình 1 mô tả
đối tượng giả định được tạo ảnh động với thuộc tính x
, đại diện cho
theo chiều ngang trên màn hình. Thời lượng của hoạt ảnh được đặt thành 40 mili giây và khoảng cách
là 40 pixel. Mỗi 10 mili giây, tốc độ làm mới khung hình mặc định, đối tượng sẽ di chuyển
theo chiều ngang 10 pixel. Kết thúc 40 mili giây, ảnh động dừng và đối tượng kết thúc lúc
vị trí ngang 40. Đây là ví dụ về ảnh động với nội suy tuyến tính, có nghĩa là
vật chuyển động với tốc độ không đổi.
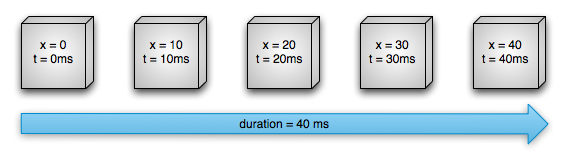
Hình 1. Ví dụ về ảnh động tuyến tính
Bạn cũng có thể chỉ định ảnh động để có nội suy phi tuyến tính. Hình 2 minh hoạ đối tượng giả định tăng tốc ở phần đầu ảnh động và giảm tốc ở ở cuối ảnh động. Đối tượng vẫn di chuyển 40 pixel trong 40 mili giây, nhưng phi tuyến tính. Trong đầu tiên, hoạt ảnh này tăng tốc đến điểm giữa rồi giảm tốc từ một nửa cho đến cuối ảnh động. Như Hình 2 cho thấy, quãng đường đã đi ở đầu và cuối của ảnh động nhỏ hơn ở giữa.
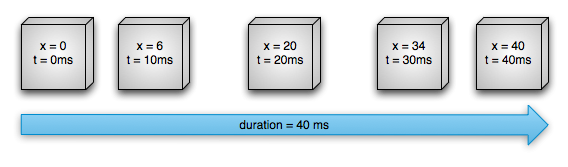
Hình 2. Ví dụ về ảnh động phi tuyến tính
Hãy cùng tìm hiểu chi tiết cách các thành phần quan trọng của hệ thống ảnh động thuộc tính sẽ tính toán các ảnh động như minh hoạ ở trên. Hình 3 mô tả cách các lớp chính làm việc với nhau.
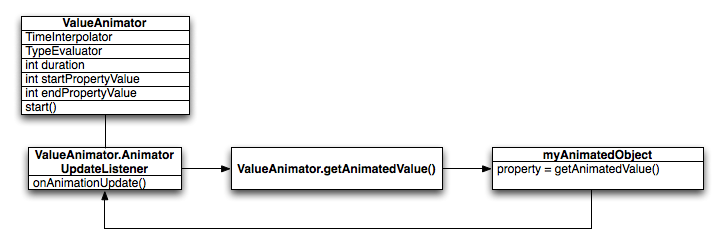
Hình 3. Cách tính ảnh động
Đối tượng ValueAnimator
theo dõi thời gian của ảnh động,
chẳng hạn như khoảng thời gian ảnh động đã chạy và giá trị hiện tại của thuộc tính
ảnh động.
ValueAnimator
đóng gói TimeInterpolator
, xác định nội suy ảnh động và TypeEvaluator
, xác định cách tính toán các giá trị cho thuộc tính đang
ảnh động. Ví dụ: trong Hình 2, TimeInterpolator
được sử dụng sẽ là
AccelerateDecelerateInterpolator
và TypeEvaluator
sẽ là IntEvaluator
.
Để bắt đầu tạo ảnh động, hãy tạo ValueAnimator
và cung cấp cho nó
các giá trị bắt đầu và kết thúc cho thuộc tính mà bạn muốn tạo ảnh động, cùng với thời lượng của
ảnh động. Khi bạn gọi start()
, ảnh động
đầu tiên. Trong toàn bộ ảnh động, ValueAnimator
sẽ tính toán một phân số đã trôi qua
từ 0 đến 1, dựa vào thời lượng của ảnh động và lượng thời gian đã trôi qua. Chiến lược phát hành đĩa đơn
phân số đã trôi qua biểu thị tỷ lệ phần trăm thời gian mà ảnh động đã hoàn tất, 0 nghĩa là 0%
và 1 nghĩa là 100%. Ví dụ: trong Hình 1, phân số đã trôi qua lúc t = 10 mili giây sẽ là 0, 25
vì tổng thời lượng là t = 40 mili giây.
Khi ValueAnimator
tính xong một phân số đã trôi qua,
gọi TimeInterpolator
hiện được đặt để tính toán
phân số nội suy. Phân số nội suy ánh xạ phân số đã trôi qua với một phân số mới
có tính đến nội suy thời gian được đặt. Ví dụ: trong Hình 2,
vì ảnh động tăng tốc từ từ, phần nội suy, khoảng 0,15, nhỏ hơn
phân số đã trôi qua, 0,25, với t = 10 ms. Trong Hình 1, phân số nội suy luôn giống với
phân số đã trôi qua.
Khi tính phân số nội suy, ValueAnimator
sẽ gọi
TypeEvaluator
thích hợp để tính giá trị của
mà bạn đang tạo ảnh động, dựa trên phân số nội suy, giá trị bắt đầu và
giá trị kết thúc của ảnh động. Ví dụ: trong Hình 2, phân số nội suy là .15 tại t =
10 mili giây, do đó, giá trị cho thuộc tính tại thời điểm đó sẽ là 0,15 × (40 - 0) hoặc 6.
Sự khác biệt giữa ảnh động thuộc tính và ảnh động chế độ xem
Hệ thống ảnh động dạng khung hiển thị cung cấp khả năng chỉ tạo ảnh động cho View
nên nếu muốn tạo ảnh động cho các đối tượng không phải View
, bạn phải triển khai
mã của riêng bạn để làm như vậy. Hệ thống ảnh động khung hiển thị cũng bị hạn chế ở chỗ nó chỉ
hiển thị một số khía cạnh của đối tượng View
để tạo ảnh động, chẳng hạn như việc điều chỉnh theo tỷ lệ và
chẳng hạn như xoay Chế độ xem nhưng không xoay màu nền.
Nhược điểm khác của hệ thống ảnh động khung hiển thị là hệ thống chỉ sửa đổi khi Khung hiển thị đã được vẽ chứ không phải là Khung hiển thị thực tế. Ví dụ: nếu bạn tạo ảnh động cho một nút di chuyển trên màn hình, nút này được vẽ chính xác, nhưng vị trí thực tế mà bạn có thể nhấp vào không thay đổi, vì vậy, bạn phải triển khai logic của riêng mình để xử lý vấn đề này.
Với hệ thống ảnh động thuộc tính, các hạn chế này sẽ được loại bỏ hoàn toàn và bạn có thể tạo ảnh động mọi thuộc tính của bất kỳ đối tượng nào (Khung hiển thị và không phải Khung hiển thị) và bản thân đối tượng đó thực sự được sửa đổi. Hệ thống ảnh động thuộc tính cũng mạnh mẽ hơn trong cách thực hiện ảnh động. Tại cấp cao, bạn chỉ định ảnh động cho các thuộc tính mà bạn muốn tạo ảnh động, chẳng hạn như màu sắc, vị trí hoặc kích thước và có thể xác định các khía cạnh của ảnh động, chẳng hạn như nội suy và đồng bộ hoá nhiều ảnh động.
Tuy nhiên, hệ thống ảnh động dạng khung hiển thị mất ít thời gian thiết lập hơn và cần ít mã hơn để viết. Nếu ảnh động dạng khung hiển thị hoàn thành mọi việc bạn cần làm, hoặc nếu mã hiện tại của bạn đã hoàn thành hoạt động theo cách bạn muốn, nên không cần phải sử dụng hệ thống ảnh động thuộc tính. Điều này cũng có thể nên sử dụng cả hai hệ thống ảnh động cho các tình huống khác nhau nếu phát sinh trường hợp sử dụng đó.
Tổng quan về API
Bạn có thể tìm thấy hầu hết các API của hệ thống ảnh động thuộc tính trong android.animation
. Vì hệ thống ảnh động khung hiển thị đã
xác định nhiều bộ nội suy trong android.view.animation
, bạn có thể sử dụng
các bộ nội suy đó trong hệ thống ảnh động thuộc tính. Các bảng sau đây mô tả các
các thành phần của hệ thống ảnh động thuộc tính.
Lớp Animator
cung cấp cấu trúc cơ bản để tạo
ảnh động. Bạn thường không sử dụng trực tiếp lớp này vì lớp này chỉ cung cấp rất ít
phải được mở rộng để hỗ trợ đầy đủ các giá trị tạo ảnh động. Nội dung sau đây
các lớp con mở rộng Animator
:
Bảng 1. Hoạ sĩ diễn hoạt
Lớp | Mô tả |
---|---|
ValueAnimator |
Công cụ thời gian chính cho ảnh động thuộc tính cũng tính toán các giá trị cho
thành ảnh động. Nó có tất cả chức năng cốt lõi để tính toán ảnh động
và chứa thông tin chi tiết về thời gian của từng ảnh động, thông tin về việc liệu một
lặp lại ảnh động, trình nghe nhận sự kiện cập nhật và khả năng thiết lập chế độ cài đặt tuỳ chỉnh
để đánh giá. Có hai phần để tạo ảnh động: tính toán
và đặt các giá trị đó trên đối tượng và thuộc tính đang được tạo ảnh động. ValueAnimator không thực hiện phần thứ hai, vì vậy bạn phải nghe
để biết nội dung cập nhật đối với các giá trị do ValueAnimator tính và
sửa đổi các đối tượng mà bạn muốn tạo ảnh động bằng logic của riêng mình. Xem phần giới thiệu về
Tạo ảnh động bằng ValueAnimator để biết thêm thông tin. |
ObjectAnimator |
Một lớp con của ValueAnimator cho phép bạn đặt mục tiêu
đối tượng và thuộc tính đối tượng để tạo ảnh động. Lớp này sẽ cập nhật thuộc tính cho phù hợp khi
hệ thống sẽ tính toán một giá trị mới cho ảnh động. Bạn muốn sử dụng
Hầu như lúc nào cũng ObjectAnimator ,
vì nó giúp quá trình tạo ảnh động cho các giá trị trên đối tượng mục tiêu dễ dàng hơn nhiều. Tuy nhiên,
đôi khi bạn muốn sử dụng ValueAnimator trực tiếp vì ObjectAnimator có một số hạn chế hơn, chẳng hạn như yêu cầu
các phương thức truy cập sẽ xuất hiện trên đối tượng mục tiêu. |
AnimatorSet |
Cung cấp cơ chế nhóm các ảnh động lại với nhau để có thể chạy trong với nhau. Bạn có thể đặt ảnh động để phát cùng nhau, theo tuần tự hoặc sau một độ trễ được chỉ định. Xem phần Biên đạo nhảy nhiều ảnh động bằng Bộ ảnh động để biết thêm thông tin. |
Trình đánh giá cho hệ thống ảnh động thuộc tính biết cách tính giá trị cho một giá trị nhất định
thuộc tính này. Các phương thức này lấy dữ liệu thời gian do Animator
cung cấp
lớp, giá trị bắt đầu và kết thúc của ảnh động, đồng thời tính toán các giá trị được tạo ảnh động của thuộc tính
dựa trên dữ liệu này. Hệ thống ảnh động thuộc tính cung cấp các trình đánh giá sau:
Bảng 2. Người đánh giá
Lớp/Giao diện | Mô tả |
---|---|
IntEvaluator |
Trình đánh giá mặc định để tính giá trị cho các thuộc tính int . |
FloatEvaluator |
Trình đánh giá mặc định để tính giá trị cho các thuộc tính float . |
ArgbEvaluator |
Trình đánh giá mặc định để tính toán giá trị cho các thuộc tính màu được biểu thị dưới dạng giá trị thập lục phân. |
TypeEvaluator |
Một giao diện giúp bạn tạo công cụ đánh giá của riêng mình. Nếu bạn đang tạo ảnh động cho một
thuộc tính đối tượng không phải là int , float hoặc màu sắc,
bạn phải triển khai giao diện TypeEvaluator để chỉ định cách
để tính toán các giá trị được tạo ảnh động của thuộc tính đối tượng. Bạn cũng có thể chỉ định TypeEvaluator tuỳ chỉnh cho int , float và màu sắc
giá trị khác nếu bạn muốn xử lý các loại đó khác với hành vi mặc định.
Xem phần Sử dụng TypeEvaluator để biết thêm
thông tin về cách viết người đánh giá tuỳ chỉnh. |
Bộ nội suy thời gian xác định cách tính toán các giá trị cụ thể trong ảnh động dưới dạng
hàm thời gian. Ví dụ: bạn có thể chỉ định ảnh động để chạy theo đường thẳng trên toàn bộ
ảnh động, tức là ảnh động di chuyển đồng đều trong toàn bộ thời gian, hoặc bạn có thể chỉ định ảnh động
để sử dụng thời gian phi tuyến tính, ví dụ: tăng tốc lúc bắt đầu và giảm tốc ở
ở cuối ảnh động. Bảng 3 mô tả các bộ nội suy có trong android.view.animation
. Nếu không có bộ nội suy nào được cung cấp phù hợp
nhu cầu của bạn, hãy triển khai giao diện TimeInterpolator
và tạo giao diện của riêng bạn. Hãy xem Sử dụng bộ nội suy để biết thêm thông tin về cách viết
bộ nội suy.
Bảng 3. Bộ nội suy
Lớp/Giao diện | Mô tả |
---|---|
AccelerateDecelerateInterpolator |
Bộ nội suy có tốc độ thay đổi bắt đầu và kết thúc chậm nhưng tăng tốc ở giữa. |
AccelerateInterpolator |
Bộ nội suy có tốc độ thay đổi bắt đầu từ từ, sau đó tăng tốc. |
AnticipateInterpolator |
Bộ nội suy có thay đổi bắt đầu lùi lại phía sau rồi hất lên phía trước. |
AnticipateOvershootInterpolator |
Bộ nội suy có thay đổi bắt đầu lùi lại phía sau, hất lên phía trước và vượt quá giá trị mục tiêu, sau đó trở về giá trị cuối cùng. |
BounceInterpolator |
Bộ nội suy có thay đổi bị trả lại ở cuối. |
CycleInterpolator |
Bộ nội suy có ảnh động lặp lại trong một số chu kỳ được chỉ định. |
DecelerateInterpolator |
Bộ nội suy có tốc độ thay đổi bắt đầu nhanh chóng sau đó giảm tốc. |
LinearInterpolator |
Bộ nội suy có tốc độ thay đổi không đổi. |
OvershootInterpolator |
Khi đó, bộ nội suy có thay đổi hất về phía trước và vượt quá giá trị cuối cùng quay lại. |
TimeInterpolator |
Giao diện cho phép bạn triển khai bộ nội suy của riêng mình. |
Tạo ảnh động bằng ValueAnimator
Lớp ValueAnimator
cho phép bạn tạo ảnh động cho các giá trị thuộc một số loại cho thuộc tính
thời lượng của ảnh động bằng cách chỉ định tập hợp int
, float
hoặc màu sắc
để tạo hiệu ứng ảnh động. Bạn nhận được ValueAnimator
bằng cách gọi một trong
phương thức gốc: ofInt()
, ofFloat()
hoặc ofObject()
. Ví dụ:
Kotlin
ValueAnimator.ofFloat(0f, 100f).apply { duration = 1000 start() }
Java
ValueAnimator animation = ValueAnimator.ofFloat(0f, 100f); animation.setDuration(1000); animation.start();
Trong mã này, ValueAnimator
bắt đầu tính các giá trị của
ảnh động, từ 0 đến 100, trong khoảng thời gian 1000 mili giây, khi phương thức start()
chạy.
Bạn cũng có thể chỉ định loại tuỳ chỉnh để tạo ảnh động bằng cách làm như sau:
Kotlin
ValueAnimator.ofObject(MyTypeEvaluator(), startPropertyValue, endPropertyValue).apply { duration = 1000 start() }
Java
ValueAnimator animation = ValueAnimator.ofObject(new MyTypeEvaluator(), startPropertyValue, endPropertyValue); animation.setDuration(1000); animation.start();
Trong mã này, ValueAnimator
bắt đầu tính các giá trị của
ảnh động, giữa startPropertyValue
và endPropertyValue
bằng cách sử dụng
logic do MyTypeEvaluator
cung cấp trong khoảng thời gian 1000 mili giây khi phương thức start()
chạy.
Bạn có thể sử dụng các giá trị của ảnh động bằng cách thêm
AnimatorUpdateListener
vào đối tượng ValueAnimator
, như minh hoạ trong
mã sau:
Kotlin
ValueAnimator.ofObject(...).apply { ... addUpdateListener { updatedAnimation -> // You can use the animated value in a property that uses the // same type as the animation. In this case, you can use the // float value in the translationX property. textView.translationX = updatedAnimation.animatedValue as Float } ... }
Java
animation.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() { @Override public void onAnimationUpdate(ValueAnimator updatedAnimation) { // You can use the animated value in a property that uses the // same type as the animation. In this case, you can use the // float value in the translationX property. float animatedValue = (float)updatedAnimation.getAnimatedValue(); textView.setTranslationX(animatedValue); } });
Trong onAnimationUpdate()
bạn có thể truy cập giá trị ảnh động đã cập nhật và sử dụng giá trị đó trong thuộc tính của
một trong các chế độ xem của bạn. Để biết thêm thông tin về trình nghe, hãy xem phần về
Trình nghe ảnh động.
Tạo ảnh động bằng ObjectAnimator
ObjectAnimator
là một lớp con của ValueAnimator
(được thảo luận trong phần trước) và kết hợp thời gian
công cụ và tính toán giá trị của ValueAnimator
với khả năng
tạo ảnh động cho thuộc tính được đặt tên của đối tượng mục tiêu. Điều này giúp việc tạo ảnh động cho mọi đối tượng trở nên dễ dàng hơn nhiều khi bạn
không cần triển khai ValueAnimator.AnimatorUpdateListener
nữa,
vì thuộc tính ảnh động sẽ tự động cập nhật.
Việc tạo thực thể cho ObjectAnimator
cũng tương tự như ValueAnimator
, nhưng bạn cũng chỉ định đối tượng và tên thuộc tính của đối tượng đó (như
Chuỗi) cùng với các giá trị để tạo hiệu ứng ảnh động giữa:
Kotlin
ObjectAnimator.ofFloat(textView, "translationX", 100f).apply { duration = 1000 start() }
Java
ObjectAnimator animation = ObjectAnimator.ofFloat(textView, "translationX", 100f); animation.setDuration(1000); animation.start();
Cách để ObjectAnimator
cập nhật các thuộc tính
thì bạn phải làm như sau:
- Thuộc tính đối tượng mà bạn đang tạo ảnh động phải có một hàm setter (kiểu viết lạc đà) ở dạng
set<PropertyName>()
. VìObjectAnimator
tự động cập nhật thuộc tính trong khi tạo ảnh động, thì thuộc tính đó phải có khả năng truy cập vào thuộc tính đó bằng phương thức setter này. Ví dụ: nếu tên thuộc tính làfoo
, bạn cần có phương thứcsetFoo()
. Nếu phương thức setter này không tồn tại, bạn sẽ có ba tùy chọn:- Thêm phương thức setter vào lớp nếu bạn có quyền làm như vậy.
- Sử dụng một lớp trình bao bọc mà bạn có quyền thay đổi và để trình bao bọc đó nhận được bằng một phương thức setter hợp lệ rồi chuyển tiếp phương thức đó đến đối tượng ban đầu.
- Thay vào đó, hãy sử dụng
ValueAnimator
.
- Nếu bạn chỉ chỉ định một giá trị cho tham số
values...
ở một trong các phương thức nhà máy củaObjectAnimator
, thì giá trị đó sẽ được coi là giá trị cuối cùng của ảnh động. Do đó, thuộc tính đối tượng mà bạn đang tạo ảnh động phải có hàm getter dùng để lấy giá trị bắt đầu của ảnh động. Hàm getter phải nằm trong biểu mẫuget<PropertyName>()
. Ví dụ: nếu tên thuộc tính làfoo
, bạn cần có một phương thứcgetFoo()
. - Phương thức getter (nếu cần) và setter của thuộc tính mà bạn đang tạo ảnh động phải
hoạt động trên cùng loại với giá trị bắt đầu và giá trị kết thúc mà bạn chỉ định cho
ObjectAnimator
. Ví dụ: bạn phải cótargetObject.setPropName(float)
vàtargetObject.getPropName()
nếu bạn tạoObjectAnimator
sau:ObjectAnimator.ofFloat(targetObject, "propName", 1f)
- Tuỳ thuộc vào thuộc tính hoặc đối tượng bạn đang tạo ảnh động, có thể bạn cần gọi phương thức
invalidate()
trên Khung hiển thị để buộc màn hình tự vẽ lại bằng đã cập nhật giá trị ảnh động. Bạn làm việc này trongonAnimationUpdate()
. Ví dụ: việc tạo ảnh động cho thuộc tính màu của một đối tượng có thể vẽ chỉ dẫn đến nội dung cập nhật cho thuộc tính khi đối tượng đó tự vẽ lại. Tất cả phương thức setter thuộc tính trên Khung hiển thị, chẳng hạn nhưsetAlpha()
vàsetTranslationX()
vô hiệu hoá Chế độ xem đúng cách, vì vậy, bạn không cần vô hiệu hoá Chế độ xem khi gọi với các giá trị mới. Để biết thêm thông tin về trình nghe, hãy xem phần về Trình nghe ảnh động.
Choreograph nhiều ảnh động bằng AnimatorSet
Trong nhiều trường hợp, bạn muốn phát một ảnh động phụ thuộc vào thời điểm một ảnh động khác bắt đầu hoặc
kết thúc. Hệ thống Android cho phép bạn nhóm các ảnh động lại với nhau vào một AnimatorSet
để bạn có thể chỉ định xem có bắt đầu ảnh động hay không
một lúc, tuần tự hay sau một khoảng thời gian trễ nhất định. Bạn cũng có thể lồng các đối tượng AnimatorSet
vào nhau.
Đoạn mã sau đây phát Animator
sau
đối tượng theo cách sau:
- Phát
bounceAnim
. - Phát
squashAnim1
,squashAnim2
,stretchAnim1
vàstretchAnim2
cùng lúc. - Phát
bounceBackAnim
. - Phát
fadeAnim
.
Kotlin
val bouncer = AnimatorSet().apply { play(bounceAnim).before(squashAnim1) play(squashAnim1).with(squashAnim2) play(squashAnim1).with(stretchAnim1) play(squashAnim1).with(stretchAnim2) play(bounceBackAnim).after(stretchAnim2) } val fadeAnim = ObjectAnimator.ofFloat(newBall, "alpha", 1f, 0f).apply { duration = 250 } AnimatorSet().apply { play(bouncer).before(fadeAnim) start() }
Java
AnimatorSet bouncer = new AnimatorSet(); bouncer.play(bounceAnim).before(squashAnim1); bouncer.play(squashAnim1).with(squashAnim2); bouncer.play(squashAnim1).with(stretchAnim1); bouncer.play(squashAnim1).with(stretchAnim2); bouncer.play(bounceBackAnim).after(stretchAnim2); ValueAnimator fadeAnim = ObjectAnimator.ofFloat(newBall, "alpha", 1f, 0f); fadeAnim.setDuration(250); AnimatorSet animatorSet = new AnimatorSet(); animatorSet.play(bouncer).before(fadeAnim); animatorSet.start();
Trình nghe ảnh động
Bạn có thể theo dõi các sự kiện quan trọng trong thời lượng của một ảnh động bằng các trình nghe được mô tả ở bên dưới.
Animator.AnimatorListener
onAnimationStart()
– Được gọi khi ảnh động bắt đầu.onAnimationEnd()
– Được gọi khi ảnh động kết thúc.onAnimationRepeat()
– Được gọi khi ảnh động lặp lại.onAnimationCancel()
– Được gọi khi ảnh động bị huỷ. Ảnh động đã bị huỷ cũng gọionAnimationEnd()
, bất kể kết thúc bằng cách nào.
ValueAnimator.AnimatorUpdateListener
-
onAnimationUpdate()
– được gọi trên mọi khung của ảnh động. Nghe sự kiện này để sử dụng các giá trị đã tính toán được tạo bởiValueAnimator
trong một ảnh động. Để sử dụng giá trị này, hãy truy vấn đối tượngValueAnimator
đã truyền vào sự kiện để nhận giá trị ảnh động hiện tại bằng phương thứcgetAnimatedValue()
. Triển khai việc này bạn bắt buộc phải sử dụng trình nghe nếu sử dụngValueAnimator
.Tuỳ thuộc vào thuộc tính hoặc đối tượng mà bạn đang tạo ảnh động, có thể bạn cần gọi lệnh
invalidate()
trên Chế độ xem để buộc vùng đó của màn hình để tự vẽ lại bằng các giá trị ảnh động mới. Ví dụ: tạo ảnh động cho thuộc tính màu sắc của một đối tượng Đối tượng có thể vẽ chỉ gây ra nội dung cập nhật cho màn hình khi đối tượng đó tự vẽ lại. Tất cả các phương thức setter thuộc tính trên Khung hiển thị, chẳng hạn nhưsetAlpha()
vàsetTranslationX()
vô hiệu hoá Chế độ xem đúng cách, do đó bạn không cần vô hiệu hoá Chế độ xem khi gọi các phương thức này bằng các giá trị mới.
-
Bạn có thể mở rộng lớp AnimatorListenerAdapter
thay vì
triển khai giao diện Animator.AnimatorListener
, nếu bạn không
muốn triển khai tất cả các phương thức của Animator.AnimatorListener
. Lớp AnimatorListenerAdapter
cung cấp giá trị trống
cách triển khai các phương thức mà bạn có thể chọn ghi đè.
Ví dụ: đoạn mã sau đây sẽ tạo một AnimatorListenerAdapter
chỉ cho onAnimationEnd()
gọi lại:
Kotlin
ObjectAnimator.ofFloat(newBall, "alpha", 1f, 0f).apply { duration = 250 addListener(object : AnimatorListenerAdapter() { override fun onAnimationEnd(animation: Animator) { balls.remove((animation as ObjectAnimator).target) } }) }
Java
ValueAnimator fadeAnim = ObjectAnimator.ofFloat(newBall, "alpha", 1f, 0f); fadeAnim.setDuration(250); fadeAnim.addListener(new AnimatorListenerAdapter() { public void onAnimationEnd(Animator animation) { balls.remove(((ObjectAnimator)animation).getTarget()); }
Tạo ảnh động cho các thay đổi về bố cục thành đối tượng ViewGroup
Hệ thống ảnh động thuộc tính cung cấp khả năng tạo ảnh động cho các thay đổi đối với đối tượng ViewGroup cũng như giúp bạn dễ dàng tạo ảnh động cho chính các đối tượng Thành phần hiển thị.
Bạn có thể tạo ảnh động cho các thay đổi về bố cục trong một ViewGroup bằng phương thức
Lớp LayoutTransition
. Các chế độ xem bên trong ViewGroup có thể
xem qua ảnh động xuất hiện và biến mất khi bạn thêm chúng vào hoặc
xoá các lớp đó khỏi ViewGroup hoặc khi bạn gọi
Phương thức setVisibility()
với
VISIBLE
, INVISIBLE
hoặc
GONE
. Các Chế độ xem còn lại trong ViewGroup cũng có thể
tạo hiệu ứng động vào vị trí mới khi bạn thêm hoặc xoá Chế độ xem. Bạn có thể xác định
các ảnh động sau đây trong đối tượng LayoutTransition
bằng cách gọi setAnimator()
và truyền vào đối tượng Animator
bằng một trong
hằng số LayoutTransition
sau đây:
APPEARING
– Một cờ cho biết ảnh động chạy trên các mục xuất hiện trong vùng chứa.CHANGE_APPEARING
– Một cờ cho biết ảnh động chạy trên các mục thay đổi do một mục mới xuất hiện trong vùng chứa.DISAPPEARING
– Một cờ cho biết ảnh động chạy trên các mục biến mất khỏi vùng chứa.CHANGE_DISAPPEARING
– Một cờ cho biết ảnh động chạy trên các mục đang thay đổi do một mục biến mất khỏi vùng chứa.
Bạn có thể xác định ảnh động tuỳ chỉnh của riêng mình cho 4 loại sự kiện này để tuỳ chỉnh giao diện của hiệu ứng chuyển đổi bố cục hoặc chỉ cần yêu cầu hệ thống ảnh động sử dụng ảnh động mặc định.
Để đặt thuộc tính android:animateLayoutchanges
thành true
cho giá trị
ViewGroup có những chức năng sau:
<LinearLayout android:orientation="vertical" android:layout_width="wrap_content" android:layout_height="match_parent" android:id="@+id/verticalContainer" android:animateLayoutChanges="true" />
Việc đặt thuộc tính này thành true sẽ tự động tạo ảnh động cho các Thành phần hiển thị được thêm vào hoặc bị xoá khỏi ViewGroup cũng như các Chế độ xem còn lại trong ViewGroup.
Tạo ảnh động cho các thay đổi về trạng thái của thành phần hiển thị bằng StateListAnimator
Lớp StateListAnimator
cho phép bạn xác định ảnh động sẽ chạy khi
trạng thái của khung hiển thị thay đổi. Đối tượng này hoạt động như một trình bao bọc cho
Đối tượng Animator
, gọi ảnh động đó bất cứ khi nào đối tượng được chỉ định
trạng thái khung hiển thị (chẳng hạn như "đã nhấn" hoặc "lấy làm tâm điểm") thay đổi.
Bạn có thể xác định StateListAnimator
trong tài nguyên XML có một gốc
Phần tử <selector>
và phần tử con <item>
mà mỗi phần tử chỉ định
một trạng thái thành phần hiển thị khác do lớp StateListAnimator
xác định. Một
<item>
chứa định nghĩa cho nhóm ảnh động thuộc tính.
Ví dụ: tệp sau đây tạo một ảnh động cho danh sách trạng thái thay đổi tỷ lệ x và y của chế độ xem khi được nhấn:
res/xml/animate_scale.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <!-- the pressed state; increase x and y size to 150% --> <item android:state_pressed="true"> <set> <objectAnimator android:propertyName="scaleX" android:duration="@android:integer/config_shortAnimTime" android:valueTo="1.5" android:valueType="floatType"/> <objectAnimator android:propertyName="scaleY" android:duration="@android:integer/config_shortAnimTime" android:valueTo="1.5" android:valueType="floatType"/> </set> </item> <!-- the default, non-pressed state; set x and y size to 100% --> <item android:state_pressed="false"> <set> <objectAnimator android:propertyName="scaleX" android:duration="@android:integer/config_shortAnimTime" android:valueTo="1" android:valueType="floatType"/> <objectAnimator android:propertyName="scaleY" android:duration="@android:integer/config_shortAnimTime" android:valueTo="1" android:valueType="floatType"/> </set> </item> </selector>
Để đính kèm trình tạo ảnh động danh sách trạng thái vào một khung hiển thị, hãy thêm
Thuộc tính android:stateListAnimator
như sau:
<Button android:stateListAnimator="@xml/animate_scale" ... />
Giờ đây, các ảnh động được xác định trong animate_scale.xml
sẽ được sử dụng khi nút này
các thay đổi về trạng thái.
Hoặc để gán một ảnh động cho danh sách trạng thái cho một khung hiển thị trong mã của bạn, hãy sử dụng
AnimatorInflater.loadStateListAnimator()
và chỉ định trình tạo ảnh động cho
khung hiển thị của mình bằng phương thức View.setStateListAnimator()
.
Hoặc thay vì tạo ảnh động cho các thuộc tính của khung hiển thị, bạn có thể phát một ảnh động có thể vẽ giữa
thay đổi trạng thái, sử dụng AnimatedStateListDrawable
.
Một số tiện ích hệ thống trong
Theo mặc định, Android 5.0 sử dụng các ảnh động này. Ví dụ sau đây minh hoạ cách
để xác định AnimatedStateListDrawable
làm tài nguyên XML:
<!-- res/drawable/myanimstatedrawable.xml --> <animated-selector xmlns:android="http://schemas.android.com/apk/res/android"> <!-- provide a different drawable for each state--> <item android:id="@+id/pressed" android:drawable="@drawable/drawableP" android:state_pressed="true"/> <item android:id="@+id/focused" android:drawable="@drawable/drawableF" android:state_focused="true"/> <item android:id="@id/default" android:drawable="@drawable/drawableD"/> <!-- specify a transition --> <transition android:fromId="@+id/default" android:toId="@+id/pressed"> <animation-list> <item android:duration="15" android:drawable="@drawable/dt1"/> <item android:duration="15" android:drawable="@drawable/dt2"/> ... </animation-list> </transition> ... </animated-selector>
Sử dụng TypeEvaluator
Nếu muốn tạo ảnh động cho một loại mà hệ thống Android không xác định, bạn có thể tự tạo ảnh động
bằng cách triển khai giao diện TypeEvaluator
. Các loại
mà hệ thống Android biết là int
, float
hoặc một màu.
được loại IntEvaluator
, FloatEvaluator
và ArgbEvaluator
hỗ trợ
người đánh giá.
Chỉ có một phương thức để triển khai trong TypeEvaluator
giao diện, phương thức evaluate()
. Điều này cho phép
trình tạo ảnh động mà bạn đang sử dụng để trả về một giá trị thích hợp cho thuộc tính ảnh động tại
điểm hiện tại của ảnh động. Lớp FloatEvaluator
minh hoạ
cách thực hiện việc này:
Kotlin
private class FloatEvaluator : TypeEvaluator<Any> { override fun evaluate(fraction: Float, startValue: Any, endValue: Any): Any { return (startValue as Number).toFloat().let { startFloat -> startFloat + fraction * ((endValue as Number).toFloat() - startFloat) } } }
Java
public class FloatEvaluator implements TypeEvaluator { public Object evaluate(float fraction, Object startValue, Object endValue) { float startFloat = ((Number) startValue).floatValue(); return startFloat + fraction * (((Number) endValue).floatValue() - startFloat); } }
Lưu ý: Khi ValueAnimator
(hoặc ObjectAnimator
) chạy, công cụ này sẽ tính một phân số hiện tại đã trôi qua của
ảnh động (một giá trị từ 0 đến 1) rồi tính toán phiên bản nội suy của giá trị đó tùy thuộc vào
vào bộ nội suy mà bạn đang sử dụng. Phân số nội suy là phân số mà TypeEvaluator
nhận được thông qua tham số fraction
. Do đó, bạn có thể làm như sau:
không phải tính đến bộ nội suy khi tính toán các giá trị được tạo ảnh động.
Sử dụng bộ nội suy
Bộ nội suy xác định cách tính các giá trị cụ thể trong ảnh động dưới dạng một hàm bất cứ lúc nào. Ví dụ: bạn có thể chỉ định ảnh động sẽ diễn ra theo đường thẳng trên toàn bộ ảnh động, tức là ảnh động sẽ di chuyển đồng đều trong toàn bộ thời gian hoặc bạn có thể chỉ định ảnh động để sử dụng phi tuyến tính, ví dụ: sử dụng tăng tốc hoặc giảm tốc ở đầu hoặc cuối ảnh động.
Bộ nội suy trong hệ thống ảnh động nhận được một phần từ Trình tạo ảnh động đại diện cho
thời gian đã trôi qua của hoạt ảnh. Bộ nội suy sửa đổi phân số này để trùng khớp với loại
ảnh động mà ứng dụng này hướng đến. Hệ thống Android cung cấp một tập hợp các bộ nội suy phổ biến trong
android.view.animation package
. Nếu không có cách nào trong số này phù hợp với
bạn có thể triển khai giao diện TimeInterpolator
và tạo
của bạn.
Ví dụ: cách bộ nội suy mặc định AccelerateDecelerateInterpolator
và LinearInterpolator
tính các phân số được nội suy được so sánh dưới đây.
LinearInterpolator
không ảnh hưởng đến phân số đã trôi qua. AccelerateDecelerateInterpolator
tăng tốc vào ảnh động và
sẽ giảm tốc. Các phương thức sau đây xác định logic cho các bộ nội suy này:
Tốc độ giảm tốc độ nội suy
Kotlin
override fun getInterpolation(input: Float): Float = (Math.cos((input + 1) * Math.PI) / 2.0f).toFloat() + 0.5f
Java
@Override public float getInterpolation(float input) { return (float)(Math.cos((input + 1) * Math.PI) / 2.0f) + 0.5f; }
LinearInterpolator (Bộ nội suy tuyến tính)
Kotlin
override fun getInterpolation(input: Float): Float = input
Java
@Override public float getInterpolation(float input) { return input; }
Bảng sau đây trình bày các giá trị gần đúng được tính theo bộ nội suy cho ảnh động kéo dài 1000 mili giây:
mili giây đã trôi qua | Phân số đã trôi qua/Phân số nội suy (Tuyến tính) | Phân số nội suy (Tăng tốc/Giảm tốc) |
---|---|---|
0 | 0 | 0 |
200 | 0,2 | 0,1 |
400 | 0,4 | ,345 |
600 | 0,6 | 0,8 |
800 | 0,8 | 0,9 |
1000 | 1 | 1 |
Như bảng hiển thị, LinearInterpolator
thay đổi các giá trị
ở cùng tốc độ thì 0,2 cho mỗi 200 mili giây. AccelerateDecelerateInterpolator
thay đổi các giá trị nhanh hơn LinearInterpolator
trong khoảng từ 200 mili giây đến 600 mili giây và chậm hơn trong khoảng từ 600 mili giây đến 600 mili giây
1000 mili giây.
Chỉ định khung hình chính
Đối tượng Keyframe
bao gồm một cặp thời gian/giá trị cho phép bạn xác định
trạng thái cụ thể tại một thời điểm cụ thể của ảnh động. Mỗi khung hình chính cũng có thể có
bộ nội suy để kiểm soát hành vi của ảnh động trong khoảng thời gian giữa
thời gian của khung hình chính và thời gian của khung hình chính này.
Để tạo thực thể cho đối tượng Keyframe
, bạn phải sử dụng một trong các hàm factory
phương thức ofInt()
, ofFloat()
hoặc ofObject()
để lấy loại Keyframe
thích hợp. Sau đó, bạn gọi
phương thức trạng thái ban đầu của ofKeyframe()
để
lấy đối tượng PropertyValuesHolder
. Sau khi có đối tượng, bạn có thể
lấy ảnh động bằng cách truyền vào đối tượng PropertyValuesHolder
và
đối tượng cần tạo hiệu ứng chuyển động. Đoạn mã sau đây minh hoạ cách thực hiện việc này:
Kotlin
val kf0 = Keyframe.ofFloat(0f, 0f) val kf1 = Keyframe.ofFloat(.5f, 360f) val kf2 = Keyframe.ofFloat(1f, 0f) val pvhRotation = PropertyValuesHolder.ofKeyframe("rotation", kf0, kf1, kf2) ObjectAnimator.ofPropertyValuesHolder(target, pvhRotation).apply { duration = 5000 }
Java
Keyframe kf0 = Keyframe.ofFloat(0f, 0f); Keyframe kf1 = Keyframe.ofFloat(.5f, 360f); Keyframe kf2 = Keyframe.ofFloat(1f, 0f); PropertyValuesHolder pvhRotation = PropertyValuesHolder.ofKeyframe("rotation", kf0, kf1, kf2); ObjectAnimator rotationAnim = ObjectAnimator.ofPropertyValuesHolder(target, pvhRotation); rotationAnim.setDuration(5000);
Tạo ảnh động cho khung hiển thị
Hệ thống ảnh động thuộc tính cho phép tinh giản ảnh động của các đối tượng và ưu đãi trong Thành phần hiển thị một vài ưu điểm so với hệ thống ảnh động khung hiển thị. Chế độ xem hệ thống ảnh động đã biến đổi các đối tượng Thành phần hiển thị bằng cách thay đổi cách vẽ các đối tượng đó. Đây là được xử lý trong vùng chứa của mỗi Khung hiển thị, vì bản thân Khung hiển thị đó không có thuộc tính nào để thao tác. Điều này dẫn đến việc Khung hiển thị được tạo ảnh động nhưng không gây ra thay đổi nào trong chính đối tượng Khung hiển thị. Chiến dịch này dẫn đến hành vi như một đối tượng vẫn tồn tại ở vị trí ban đầu, mặc dù đối tượng đó được vẽ ở một vị trí khác trên màn hình. Trong Android 3.0, các thuộc tính mới và các thuộc tính tương ứng Phương thức getter và setter đã được thêm vào để loại bỏ hạn chế này.
Hệ thống ảnh động thuộc tính
có thể tạo ảnh động cho Thành phần hiển thị trên màn hình bằng cách thay đổi thuộc tính thực tế trong các đối tượng Thành phần hiển thị đó. Trong
Ngoài ra, Chế độ xem cũng tự động gọi invalidate()
để làm mới màn hình mỗi khi thuộc tính của màn hình thay đổi. Các thuộc tính mới trong lớp View
hỗ trợ ảnh động thuộc tính là:
translationX
vàtranslationY
: Các thuộc tính này kiểm soát vị trí Chế độ xem được định vị dưới dạng delta từ toạ độ bên trái và trên cùng được thiết lập theo bố cục vùng chứa.rotation
,rotationX
vàrotationY
: Các thuộc tính này điều khiển chế độ xoay ở chế độ 2D (thuộc tínhrotation
) và 3D xung quanh điểm trung tâm.scaleX
vàscaleY
: Các thuộc tính này kiểm soát việc điều chỉnh tỷ lệ 2D của Xem xung quanh điểm trung tâm.pivotX
vàpivotY
: Các tài sản này kiểm soát vị trí của điểm trung tâm, là nơi xảy ra các phép biến đổi xoay và chia tỷ lệ. Theo mặc định, bảng tổng hợp điểm nằm ở tâm của vật thể.x
vày
: Đây là các thuộc tính tiện ích đơn giản để mô tả vị trí cuối cùng của Chế độ xem trong vùng chứa của nó, như là tổng của các giá trị bên trái và giá trị hàng đầu và Các giá trị TranslationX và TranslationY.alpha
: Biểu thị độ trong suốt alpha trên Chế độ xem. Giá trị này là 1 (không rõ ràng) theo mặc định, với giá trị 0 thể hiện độ trong suốt hoàn toàn (không hiển thị).
Để tạo ảnh động cho một thuộc tính của đối tượng Thành phần hiển thị (View), chẳng hạn như giá trị xoay hoặc màu sắc, bạn chỉ cần cần tạo một ảnh động thuộc tính và chỉ định thuộc tính Chế độ xem mà bạn muốn tạo ảnh động. Ví dụ:
Kotlin
ObjectAnimator.ofFloat(myView, "rotation", 0f, 360f)
Java
ObjectAnimator.ofFloat(myView, "rotation", 0f, 360f);
Để biết thêm thông tin về cách tạo ảnh động, hãy xem các phần về tạo ảnh động bằng ValueAnimator và ObjectAnimator.
Tạo ảnh động bằng ViewPropertyAnimator
ViewPropertyAnimator
cung cấp một cách đơn giản để tạo ảnh động cho nhiều
các thuộc tính của View
song song, sử dụng một Animator
cơ bản
. Lớp này hoạt động giống như ObjectAnimator
vì chương trình này sửa đổi
giá trị thực tế của các thuộc tính của thành phần hiển thị đó, nhưng hiệu quả hơn khi tạo ảnh động cho nhiều thuộc tính tại
một lần. Ngoài ra, mã để sử dụng ViewPropertyAnimator
rất nhiều
ngắn gọn và dễ đọc hơn. Các đoạn mã sau đây cho thấy sự khác biệt trong việc sử dụng nhiều
ObjectAnimator
đối tượng, một đối tượng duy nhất
ObjectAnimator
và ViewPropertyAnimator
khi
tạo ảnh động đồng thời cho thuộc tính x
và y
của khung hiển thị.
Nhiều đối tượng ObjectAnimator
Kotlin
val animX = ObjectAnimator.ofFloat(myView, "x", 50f) val animY = ObjectAnimator.ofFloat(myView, "y", 100f) AnimatorSet().apply { playTogether(animX, animY) start() }
Java
ObjectAnimator animX = ObjectAnimator.ofFloat(myView, "x", 50f); ObjectAnimator animY = ObjectAnimator.ofFloat(myView, "y", 100f); AnimatorSet animSetXY = new AnimatorSet(); animSetXY.playTogether(animX, animY); animSetXY.start();
Một ObjectAnimator
Kotlin
val pvhX = PropertyValuesHolder.ofFloat("x", 50f) val pvhY = PropertyValuesHolder.ofFloat("y", 100f) ObjectAnimator.ofPropertyValuesHolder(myView, pvhX, pvhY).start()
Java
PropertyValuesHolder pvhX = PropertyValuesHolder.ofFloat("x", 50f); PropertyValuesHolder pvhY = PropertyValuesHolder.ofFloat("y", 100f); ObjectAnimator.ofPropertyValuesHolder(myView, pvhX, pvhY).start();
ViewPropertyAnimator
Kotlin
myView.animate().x(50f).y(100f)
Java
myView.animate().x(50f).y(100f);
Để biết thêm thông tin chi tiết về ViewPropertyAnimator
, hãy xem trang Nhà phát triển Android tương ứng
blog
bài đăng.
Khai báo ảnh động trong XML
Hệ thống ảnh động thuộc tính cho phép bạn khai báo ảnh động thuộc tính bằng XML thay vì thực hiện theo phương thức lập trình. Bằng cách xác định ảnh động trong XML, bạn có thể dễ dàng sử dụng lại ảnh động trong nhiều hoạt động và dễ dàng chỉnh sửa chuỗi ảnh động.
Để phân biệt các tệp ảnh động sử dụng API ảnh động thuộc tính mới với các tệp sử dụng
khung ảnh động dạng khung hiển thị cũ,
kể từ Android 3.1, bạn nên lưu tệp XML cho ảnh động thuộc tính trong thư mục res/animator/
.
Các lớp ảnh động thuộc tính sau đây hỗ trợ khai báo XML với các thẻ XML sau:
ValueAnimator
–<animator>
ObjectAnimator
–<objectAnimator>
AnimatorSet
–<set>
Để tìm các thuộc tính mà bạn có thể dùng trong phần khai báo XML, hãy xem phần Ảnh động . Ví dụ sau đây phát 2 tập hợp ảnh động của đối tượng tuần tự, với tập hợp lồng nhau đầu tiên phát 2 ảnh động đối tượng cùng nhau:
<set android:ordering="sequentially"> <set> <objectAnimator android:propertyName="x" android:duration="500" android:valueTo="400" android:valueType="intType"/> <objectAnimator android:propertyName="y" android:duration="500" android:valueTo="300" android:valueType="intType"/> </set> <objectAnimator android:propertyName="alpha" android:duration="500" android:valueTo="1f"/> </set>
Để chạy ảnh động này, bạn phải tăng cường tài nguyên XML trong mã thành đối tượng AnimatorSet
, sau đó đặt đối tượng mục tiêu cho tất cả các ảnh động trước khi bắt đầu bộ ảnh động. Việc gọi setTarget()
sẽ đặt một đối tượng mục tiêu duy nhất cho tất cả thành phần con của AnimatorSet
để thuận tiện. Mã sau đây minh hoạ cách thực hiện việc này:
Kotlin
(AnimatorInflater.loadAnimator(myContext, R.animator.property_animator) as AnimatorSet).apply { setTarget(myObject) start() }
Java
AnimatorSet set = (AnimatorSet) AnimatorInflater.loadAnimator(myContext, R.animator.property_animator); set.setTarget(myObject); set.start();
Bạn cũng có thể khai báo ValueAnimator
trong XML, như
như trong ví dụ sau:
<animator xmlns:android="http://schemas.android.com/apk/res/android" android:duration="1000" android:valueType="floatType" android:valueFrom="0f" android:valueTo="-100f" />
Để sử dụng ValueAnimator
trước đó trong mã, bạn
phải tăng cường đối tượng, hãy thêm
AnimatorUpdateListener
,
lấy giá trị ảnh động đã cập nhật rồi sử dụng giá trị đó trong thuộc tính của một trong các chế độ xem của bạn
như minh hoạ trong đoạn mã sau:
Kotlin
(AnimatorInflater.loadAnimator(this, R.animator.animator) as ValueAnimator).apply { addUpdateListener { updatedAnimation -> textView.translationX = updatedAnimation.animatedValue as Float } start() }
Java
ValueAnimator xmlAnimator = (ValueAnimator) AnimatorInflater.loadAnimator(this, R.animator.animator); xmlAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() { @Override public void onAnimationUpdate(ValueAnimator updatedAnimation) { float animatedValue = (float)updatedAnimation.getAnimatedValue(); textView.setTranslationX(animatedValue); } }); xmlAnimator.start();
Để biết thông tin về cú pháp XML để xác định ảnh động thuộc tính, hãy xem phần Ảnh động .
Các tác động có thể xảy ra đối với hiệu suất của giao diện người dùng
Các ảnh động cập nhật giao diện người dùng gây ra thêm công việc kết xuất cho mỗi khung hình trong mà ảnh động chạy. Vì lý do này, việc sử dụng ảnh động cần nhiều tài nguyên có thể tác động tiêu cực đến hiệu suất của ứng dụng.
Công việc cần thiết để tạo ảnh động cho giao diện người dùng được thêm vào giai đoạn ảnh động của quy trình kết xuất. Bạn có thể tìm hiểu xem ảnh động của mình có ảnh hưởng đến hiệu suất của ứng dụng bằng cách bật tính năng Phân tích trực quan kết xuất GPU và theo dõi giai đoạn ảnh động. Để biết thêm thông tin, hãy xem phần Kết xuất GPU cấu hình hướng dẫn từng bước.