Mit Verknüpfungen können Sie Ihren Nutzern bestimmte Inhalte schnell auf Teile deiner App zugreifen.
<ph type="x-smartling-placeholder">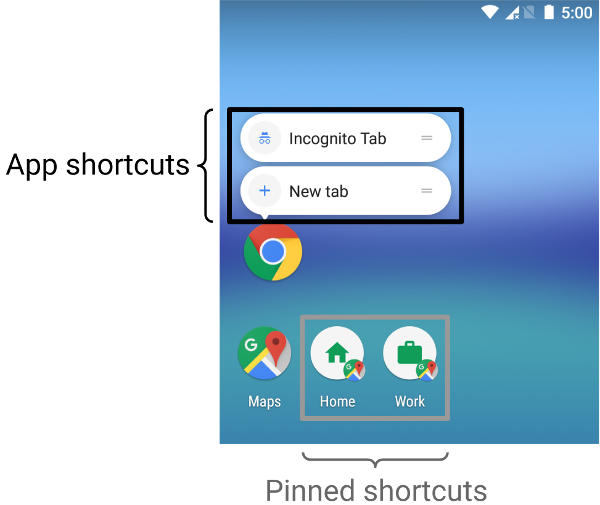
Wie Sie Inhalte mithilfe von Verknüpfungen bereitstellen, hängt vom Anwendungsfall und davon ab, ob ist der Kontext der Verknüpfung app- oder nutzergesteuert. Obwohl eine statische Der Kontext der Verknüpfung ändert sich nicht und der Kontext der dynamischen Verknüpfung ändert sich ständig. ist Ihre App in beiden Fällen maßgeblich für den Kontext. In Fällen, in denen Nutzende festlegen, wie Ihre App Inhalte an Nutzer bereitstellt, z. B. über eine angepinnte Verknüpfung, der Kontext von den Nutzenden definiert wird. In den folgenden Szenarien werden einige Anwendungsbereiche beschrieben: Fälle für die einzelnen Verknüpfungstypen ein:
- Statisch Verknüpfungen eignen sich am besten für Apps, die über eine einheitliche während der gesamten Lebensdauer der Interaktion eines Nutzers mit dem Da die meisten Launcher nur Display vier gleichzeitig verwenden, sind statische Tastenkombinationen hilfreich, um einen Ablauf auszuführen. auf einheitliche Weise zu erledigen, z. B. ob Nutzende ihren Kalender oder auf bestimmte Weise kommunizieren .
- Dynamisch Verknüpfungen werden für Aktionen in Apps verwendet, kontextsensibel sind. Kontextabhängige Tastenkombinationen sind auf die Aktionen, die Nutzende in einer App durchführen. Wenn Sie z. B. ein Spiel entwickeln, wenn die Nutzenden bei der Einführung mit ihrem aktuellen Level beginnen, müssen Sie die häufig verwendete Tastenkombinationen. Mit einer dynamischen Verknüpfung kannst du die Verknüpfung aktualisieren wenn der Nutzer ein Level beendet.
- Angepinnt Tastenkombinationen werden für bestimmte nutzergesteuerte Aktionen verwendet. Beispiel: kann ein Nutzer eine bestimmte Website an den Launcher anpinnen. Dies ist da Nutzende so eine benutzerdefinierte Aktion ausführen können, z. B. können wir die Website in einem Schritt schneller aufrufen als -Instanz eines Browsers.
Statische Verknüpfungen erstellen
Statische Verknüpfungen stellen Links zu allgemeinen Aktionen in Ihrer App bereit. Aktionen müssen für die gesamte Lebensdauer der aktuellen Version Ihrer App gleich bleiben. Gute Optionen für statische Tastenkombinationen sind das Anzeigen von gesendeten Nachrichten, das Festlegen einer Wecker und Anzeige der Trainingsaktivität des Nutzers für den Tag.
So erstellen Sie eine statische Verknüpfung:
-
Suchen Sie in der Datei
AndroidManifest.xml
Ihrer App die Aktivität, deren Intent-Filter sind auf dieandroid.intent.action.MAIN
Aktion und dieandroid.intent.category.LAUNCHER
Kategorie. -
Fügen Sie ein
<meta-data>
-Element zu dieser Aktivität hinzufügen, das auf die Ressourcendatei verweist, in der die Tastenkombinationen sind definiert: <ph type="x-smartling-placeholder"><manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.myapplication"> <application ... > <activity android:name="Main"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> <meta-data android:name="android.app.shortcuts" android:resource="@xml/shortcuts" /> </activity> </application> </manifest>
-
Erstellen Sie eine neue Ressourcendatei mit dem Namen
res/xml/shortcuts.xml
. -
Fügen Sie in der neuen Ressourcendatei ein
<shortcuts>
-Stammelement hinzu. die eine Liste mit<shortcut>
-Elementen enthält. In jeder<shortcut>
-Element, einschließlich Informationen zu einem statischen Element Verknüpfung, einschließlich Symbol, Beschreibungslabels und der gestarteten Intents in der App:<shortcuts xmlns:android="http://schemas.android.com/apk/res/android"> <shortcut android:shortcutId="compose" android:enabled="true" android:icon="@drawable/compose_icon" android:shortcutShortLabel="@string/compose_shortcut_short_label1" android:shortcutLongLabel="@string/compose_shortcut_long_label1" android:shortcutDisabledMessage="@string/compose_disabled_message1"> <intent android:action="android.intent.action.VIEW" android:targetPackage="com.example.myapplication" android:targetClass="com.example.myapplication.ComposeActivity" /> <!-- If your shortcut is associated with multiple intents, include them here. The last intent in the list determines what the user sees when they launch this shortcut. --> <categories android:name="android.shortcut.conversation" /> <capability-binding android:key="actions.intent.CREATE_MESSAGE" /> </shortcut> <!-- Specify more shortcuts here. --> </shortcuts>
Attributwerte anpassen
Die folgende Liste enthält Beschreibungen für die verschiedenen Attribute in
ein statisches Kürzel. Geben Sie einen Wert für android:shortcutId
und
android:shortcutShortLabel
. Alle anderen Werte sind optional.
-
android:shortcutId
-
Ein String-Literal, das das Kürzel darstellt, wenn ein
<ph type="x-smartling-placeholder">ShortcutManager
-Objekt Vorgänge für dieses Objekt ausführt. -
android:shortcutShortLabel
-
Eine kurze Wortgruppe, die den Zweck der Verknüpfung beschreibt. Wenn möglich, und beschränken Sie die Kurzbeschreibung auf 10 Zeichen.
Weitere Informationen finden Sie unter
<ph type="x-smartling-placeholder">setShortLabel()
-
android:shortcutLongLabel
-
Eine erweiterte Wortgruppe, die den Zweck der Verknüpfung beschreibt. Wenn es genug wird dieser Wert in der Übersicht angezeigt
android:shortcutShortLabel
Beschränken Sie nach Möglichkeit diesen langen Zeitraum Beschreibung auf 25 Zeichen.Weitere Informationen finden Sie unter
<ph type="x-smartling-placeholder">setLongLabel()
-
android:shortcutDisabledMessage
-
Die Nachricht, die in einem unterstützten Launcher angezeigt wird, wenn der Nutzer versucht, eine deaktivierte Tastenkombination zu starten. In der Nachricht muss dem Nutzer erklärt werden, warum Verknüpfung ist deaktiviert. Der Wert dieses Attributs hat keine Auswirkungen, wenn
<ph type="x-smartling-placeholder">android:enabled
isttrue
. -
android:enabled
-
Bestimmt, ob der Nutzer mit der Tastenkombination über eine unterstützte Launcher. Der Standardwert von
android:enabled
isttrue
. Wenn Sie es auffalse
setzen, legen Sie einandroid:shortcutDisabledMessage
erklären, warum Sie dem Deaktivieren der Tastenkombination. Falls Sie der Meinung sind, dass Sie eine solche Nachricht nicht benötigen, vollständig aus der XML-Datei entfernen. -
android:icon
-
Die Bitmap oder adaptiv , das im Launcher verwendet wird, wenn dem Nutzer die Verknüpfung angezeigt wird. Dieses kann der Pfad zu einem Bild oder die Ressourcendatei sein, die den Bild. Verwenden Sie nach Möglichkeit adaptive Symbole, um die Leistung und Einheitlichkeit.
<ph type="x-smartling-placeholder">
Innere Elemente konfigurieren
Die XML-Datei, in der die statischen Tastenkombinationen einer Anwendung aufgeführt sind, unterstützt Folgendes:
-Elemente innerhalb der einzelnen <shortcut>
-Elemente. Ich
muss für jedes ein inneres intent
-Element enthalten sein.
statische Tastenkombination, die Sie definieren.
-
intent
-
Die Aktion, die das System startet, wenn der Nutzer die Tastenkombination auswählt. Dieser Intent muss einen Wert für
<ph type="x-smartling-placeholder">android:action
bereitstellen .Sie können mehrere Intents für eine einzelne Verknüpfung angeben. Weitere Informationen finden Sie unter Verwalten mehreren Intents und Aktivitäten, Festlegen einen Intent und die
TaskStackBuilder
-Klassenreferenz. -
categories
-
Bietet eine Gruppierung der Aktionstypen, die die Verknüpfungen Ihrer App verwenden z. B. neue Chatnachrichten erstellen.
Eine Liste der unterstützten Kategorien für Tastenkombinationen findest du in der
ShortcutInfo
Klassenreferenz. -
capability-binding
-
Deklariert die Funktion mit der Verknüpfung verknüpft ist.
Im vorherigen Beispiel ist das Kürzel mit einer deklarierten Funktion verknüpft. für
CREATE_MESSAGE
, eine App Actions Intent ein. Mit dieser Funktionsbindung können Nutzer gesprochene Befehle mit Google Assistant, um eine Verknüpfung aufzurufen.
Dynamische Verknüpfungen erstellen
Dynamische Verknüpfungen bieten Links zu spezifischen, kontextabhängigen Aktionen in für Ihre App. Diese Aktionen können sich ändern, je nachdem, ob du deine App oder währenddessen nutzt ausgeführt wird. Dynamische Tastenkombinationen eignen sich beispielsweise für Anrufe an eine bestimmte Person, zu einem bestimmten Ort navigieren und ein Spiel nach dem letzten Speichervorgang des Nutzers laden. Punkt. Sie können Unterhaltungen auch mit dynamischen Tastenkombinationen öffnen.
Die
ShortcutManagerCompat
Die Jetpack-Bibliothek ist ein nützliches Hilfsmittel für
ShortcutManager
API, mit der du dynamische Verknüpfungen in deiner App verwalten kannst. Mit der
Die ShortcutManagerCompat
-Bibliothek reduziert Boilerplate-Code und hilft
damit deine Verknüpfungen unter allen Android-Versionen einheitlich funktionieren. Dieses
ist auch erforderlich, um dynamische Verknüpfungen zu senden, damit sie
auf Google-Oberflächen wie Assistant angezeigt werden, mit dem
Google Shortcuts Integration Library.
Mit der ShortcutManagerCompat
API kann Ihre Anwendung Folgendes ausführen:
Operationen mit dynamischen Kürzeln:
-
Push und Update: Verwenden Sie
pushDynamicShortcut()
um dynamische Verknüpfungen zu veröffentlichen und zu aktualisieren. Wenn bereits dynamische oder angepinnten Tastenkombinationen mit derselben ID, werden alle änderbaren Tastenkombinationen aktualisiert. -
Entfernen:Zum Entfernen einer Reihe dynamischer Tastenkombinationen verwenden Sie
removeDynamicShortcuts()
Alle dynamischen Tastenkombinationen entfernen mitremoveAllDynamicShortcuts()
Weitere Informationen zum Ausführen von Vorgängen für Tastenkombinationen finden Sie unter
Verknüpfungen verwalten
und die
ShortcutManagerCompat
Referenz.
Hier siehst du ein Beispiel, wie du eine dynamische Verknüpfung erstellst und mit deinem App:
Kotlin
val shortcut = ShortcutInfoCompat.Builder(context, "id1") .setShortLabel("Website") .setLongLabel("Open the website") .setIcon(IconCompat.createWithResource(context, R.drawable.icon_website)) .setIntent(Intent(Intent.ACTION_VIEW, Uri.parse("https://www.mysite.example.com/"))) .build() ShortcutManagerCompat.pushDynamicShortcut(context, shortcut)
Java
ShortcutInfoCompat shortcut = new ShortcutInfoCompat.Builder(context, "id1") .setShortLabel("Website") .setLongLabel("Open the website") .setIcon(IconCompat.createWithResource(context, R.drawable.icon_website)) .setIntent(new Intent(Intent.ACTION_VIEW, Uri.parse("https://www.mysite.example.com/"))) .build(); ShortcutManagerCompat.pushDynamicShortcut(context, shortcut);
Google Shortcuts Integration Library hinzufügen
Die Google Shortcuts Integration Library ist eine optionale Jetpack-Bibliothek. Es können Sie dynamische Verknüpfungen erstellen, die auf Android-Oberflächen wie wie der Launcher und Google-Oberflächen wie Assistant. Diese Bibliothek verwenden können Nutzer Verknüpfungen finden, mit denen sie schnell auf bestimmte Inhalte zugreifen oder Inhalte noch einmal abspielen können Aktionen in Ihrer App ausführen.
Eine Messaging-App kann z. B. eine dynamische Verknüpfung für einen Kontakt namens „Alex“ nachdem ein Nutzer dieser Person eine Nachricht gesendet hat. Nachdem die dynamische Verknüpfung wenn der Nutzer Assistant fragt: „Hey Google, sende eine Nachricht an Alex über ExampleApp" erhalten, kann Assistant ExampleApp starten und automatisch konfigurieren um eine Nachricht an Alex zu senden.
Dynamische Tastenkombinationen, die mit dieser Bibliothek übertragen werden, unterliegen nicht den Limits für Tastenkombinationen pro Gerät durchgesetzt werden. So kann Ihre App jedes Mal eine Verknüpfung senden, wenn ein der Nutzer eine verknüpfte Aktion in Ihrer App ausführt. Häufige Tastenkombinationen senden ermöglicht Google, die Nutzungsmuster des Nutzers zu verstehen und kontextbezogene Vorschläge zu machen relevante Tastenkombinationen zu finden.
Beispielsweise kann Assistant durch Verknüpfungen lernen, die von deinem Fitness-Tracking-App, die Nutzende in der Regel jeden Morgen und proaktiv ausführen. „Starte ein Lauftraining“ vorschlagen wenn der Nutzer sein Smartphone in die Hand nimmt. morgens.
Die Google Shortcuts Integration Library
bietet keine adressierbaren
Funktionalität selbst. Wenn du diese Mediathek zu deiner App hinzufügst, können Google-Plattformen
in den Verknüpfungen, die deine App mit ShortcutManagerCompat
sendet.
So verwenden Sie diese Bibliothek in Ihrer App:
-
Aktualisieren Sie Ihre
gradle.properties
-Datei, um sie zu unterstützen AndroidX-Bibliotheken:android.useAndroidX=true # Automatically convert third-party libraries to use AndroidX android.enableJetifier=true
-
Fügen Sie in
app/build.gradle
Abhängigkeiten für die Shortcuts Integration Library undShortcutManagerCompat
:dependencies { implementation "androidx.core:core:1.6.0" implementation 'androidx.core:core-google-shortcuts:1.0.0' ... }
Wenn die Bibliotheksabhängigkeiten zu Ihrem Android-Projekt hinzugefügt wurden, kann Ihre App
Methode pushDynamicShortcut()
von
ShortcutManagerCompat
, um geeignete dynamische Verknüpfungen zu senden
zur Anzeige im Launcher und auf teilnehmenden Google-Oberflächen.
Angepinnte Verknüpfungen erstellen
Unter Android 8.0 (API-Level 26) und höher können Sie angepinnte Verknüpfungen erstellen. Im Gegensatz zu statischen und dynamischen Tastenkombinationen werden angepinnte Tastenkombinationen in unterstützten als separate Symbole. In Abbildung 1 sehen Sie den Unterschied zwischen diesen beiden Optionen. Arten von Tastenkombinationen.
<ph type="x-smartling-placeholder">Wenn du mithilfe deiner App eine Verknüpfung zu einem unterstützten Launcher anpinnen möchtest, führe die folgenden Schritten:
-
Verwenden Sie
isRequestPinShortcutSupported()
überprüfen, ob der Standard-Launcher des Geräts In-App-Anpinnen unterstützt, Tastenkombinationen. -
Sie haben zwei Möglichkeiten, ein
ShortcutInfo
-Objekt zu erstellen, je nachdem, ob die Verknüpfung vorhanden ist:-
Wenn die Verknüpfung vorhanden ist, erstellen Sie ein
ShortcutInfo
-Objekt, das enthält nur die ID der vorhandenen Verknüpfung. Das System findet und pinnen alle automatisch weitere Informationen zu der Verknüpfung abrufen. -
Wenn du eine neue Verknüpfung anpinnst, erstelle eine
ShortcutInfo
Objekt, das eine ID, einen Intent und ein kurzes Label für das neue .
-
Wenn die Verknüpfung vorhanden ist, erstellen Sie ein
-
Pinne die Verknüpfung durch folgenden Aufruf an den Launcher des Geräts an:
<ph type="x-smartling-placeholder">requestPinShortcut()
Während dieses Vorgangs können Sie einePendingIntent
an, mit dem Ihre App nur benachrichtigt wird, wenn die Verknüpfung angepinnt wird. erfolgreich war.Nachdem eine Verknüpfung angepinnt ist, kann Ihre App deren Inhalt mithilfe der
updateShortcuts()
. Weitere Informationen finden Sie unter Aktualisieren Tastenkombinationen.
Im folgenden Code-Snippet sehen Sie, wie eine angepinnte Verknüpfung erstellt wird.
<ph type="x-smartling-placeholder">Kotlin
val shortcutManager = getSystemService(ShortcutManager::class.java) if (shortcutManager!!.isRequestPinShortcutSupported) { // Enable the existing shortcut with the ID "my-shortcut". val pinShortcutInfo = ShortcutInfo.Builder(context, "my-shortcut").build() // Create the PendingIntent object only if your app needs to be notified // that the user let the shortcut be pinned. If the pinning operation fails, // your app isn't notified. Assume here that the app implements a method // called createShortcutResultIntent() that returns a broadcast intent. val pinnedShortcutCallbackIntent = shortcutManager.createShortcutResultIntent(pinShortcutInfo) // Configure the intent so that your app's broadcast receiver gets the // callback successfully. For details, see PendingIntent.getBroadcast(). val successCallback = PendingIntent.getBroadcast(context, /* request code */ 0, pinnedShortcutCallbackIntent, /* flags */ 0) shortcutManager.requestPinShortcut(pinShortcutInfo, successCallback.intentSender) }
Java
ShortcutManager shortcutManager = context.getSystemService(ShortcutManager.class); if (shortcutManager.isRequestPinShortcutSupported()) { // Enable the existing shortcut with the ID "my-shortcut". ShortcutInfo pinShortcutInfo = new ShortcutInfo.Builder(context, "my-shortcut").build(); // Create the PendingIntent object only if your app needs to be notified // that the user let the shortcut be pinned. If the pinning operation fails, // your app isn't notified. Assume here that the app implements a method // called createShortcutResultIntent() that returns a broadcast intent. Intent pinnedShortcutCallbackIntent = shortcutManager.createShortcutResultIntent(pinShortcutInfo); // Configure the intent so that your app's broadcast receiver gets the // callback successfully. For details, see PendingIntent.getBroadcast(). PendingIntent successCallback = PendingIntent.getBroadcast(context, /* request code */ 0, pinnedShortcutCallbackIntent, /* flags */ 0); shortcutManager.requestPinShortcut(pinShortcutInfo, successCallback.getIntentSender()); }
Aktivität für benutzerdefinierte Verknüpfungen erstellen
<ph type="x-smartling-placeholder">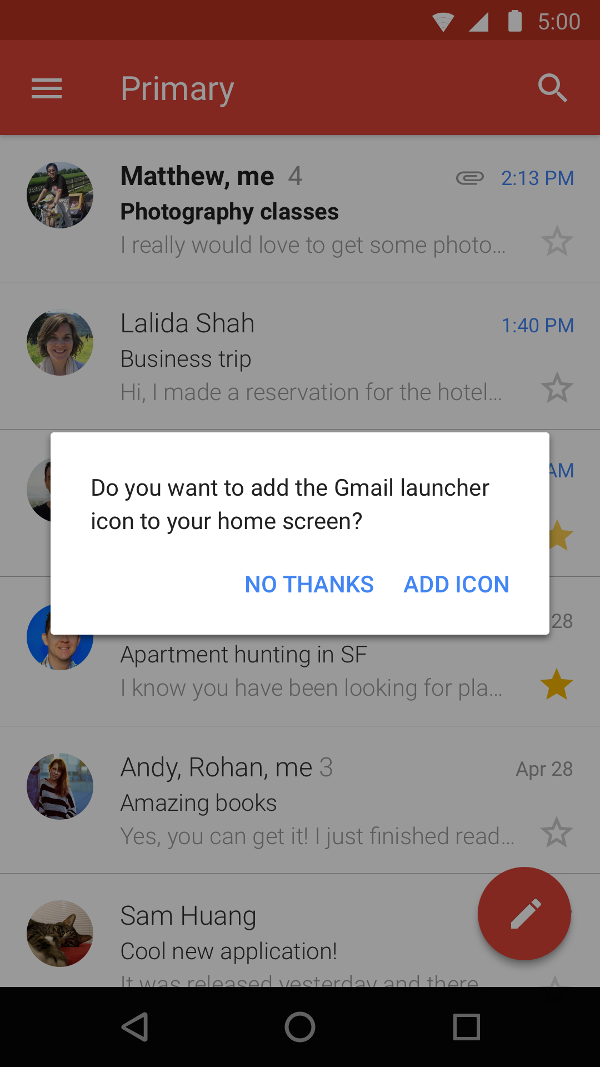
Sie können auch eine spezielle Aktivität erstellen, mit benutzerdefinierten Optionen und einer Bestätigungsschaltfläche. Abbildung 2 zeigt eine ein Beispiel für diese Art von Aktivität in der Gmail App.
Fügen Sie in der Manifestdatei Ihrer App
ACTION_CREATE_SHORTCUT
auf die
<intent-filter>
-Elements. Mit dieser Deklaration wird das folgende Verhalten eingerichtet, wenn der Nutzer versucht,
um eine Verknüpfung zu erstellen:
- Das System startet die spezielle Aktivität Ihrer App.
- Der Nutzer legt Optionen für die Verknüpfung fest.
- Der Nutzer wählt die Bestätigungsschaltfläche aus.
-
Deine App erstellt die Verknüpfung mithilfe der
createShortcutResultIntent()
. Diese Methode gibt einIntent
, die Ihre App an die zuvor ausgeführte Aktivität zurücksendet, indem siesetResult()
. -
Deine App ruft an
finish()
zur Aktivität, mit der die benutzerdefinierte Verknüpfung erstellt wurde.
Gleichermaßen kann Ihre App Nutzer auffordern, angepinnte Verknüpfungen zum Zuhause hinzuzufügen nach der Installation oder beim ersten Start der App angezeigt. Diese Methode ist da Nutzer so leichter eine Verknüpfung als Teil ihrer normalen Workflow.
Tastenkombinationen testen
Wenn du die Verknüpfungen deiner App testen möchtest, installiere die App auf einem Gerät mit einem Launcher die Tastenkombinationen unterstützt. Führen Sie dann die folgenden Aktionen aus:
- Berühren und Halte das Launcher-Symbol deiner App gedrückt, um die von dir definierten Verknüpfungen zu sehen für Ihre App.
- Ziehe eine Verknüpfung, um sie im Launcher des Geräts anzupinnen.