A basic notification usually includes a title, a line of text, and actions the user can perform in response. To provide more information, you can create large, expandable notifications by applying one of several notification templates as described in this document.
To start, build a notification with all the basic content as described in
Create a notification. Then,
call
setStyle()
with a style object and supply information corresponding to each template, as
shown in the following examples.
Add a large image
To add an image in your notification, pass an instance of
NotificationCompat.BigPictureStyle
to setStyle()
.
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setStyle(NotificationCompat.BigPictureStyle() .bigPicture(myBitmap)) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(myBitmap)) .build();
To make the image appear as a thumbnail only while the notification is
collapsed, as shown in the following figure, call
setLargeIcon()
and pass it the image. Then, call
BigPictureStyle.bigLargeIcon()
and pass it null
so the large icon goes away when the notification is
expanded:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setLargeIcon(myBitmap) .setStyle(NotificationCompat.BigPictureStyle() .bigPicture(myBitmap) .bigLargeIcon(null)) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setLargeIcon(myBitmap) .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(myBitmap) .bigLargeIcon(null)) .build();
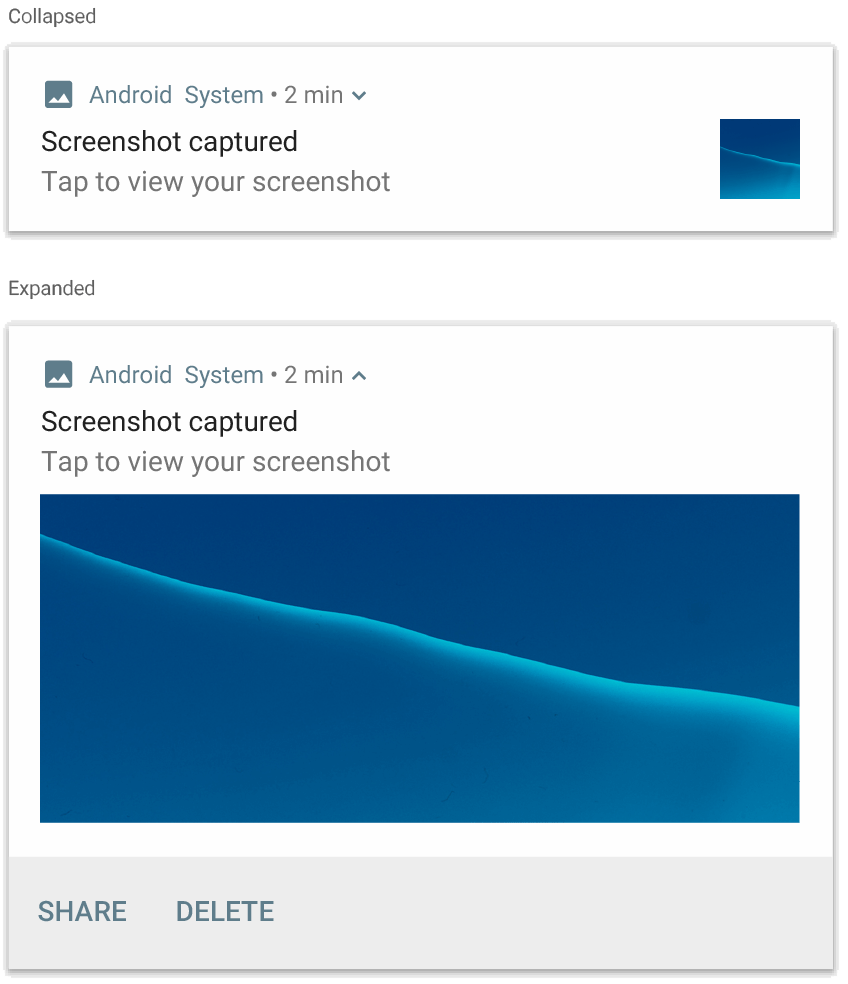
NotificationCompat.BigPictureStyle
.
Add a large block of text
Apply
NotificationCompat.BigTextStyle
to display text in the expanded content area of the notification:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_mail) .setContentTitle(emailObject.getSenderName()) .setContentText(emailObject.getSubject()) .setLargeIcon(emailObject.getSenderAvatar()) .setStyle(NotificationCompat.BigTextStyle() .bigText(emailObject.getSubjectAndSnippet())) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_mail) .setContentTitle(emailObject.getSenderName()) .setContentText(emailObject.getSubject()) .setLargeIcon(emailObject.getSenderAvatar()) .setStyle(new NotificationCompat.BigTextStyle() .bigText(emailObject.getSubjectAndSnippet())) .build();
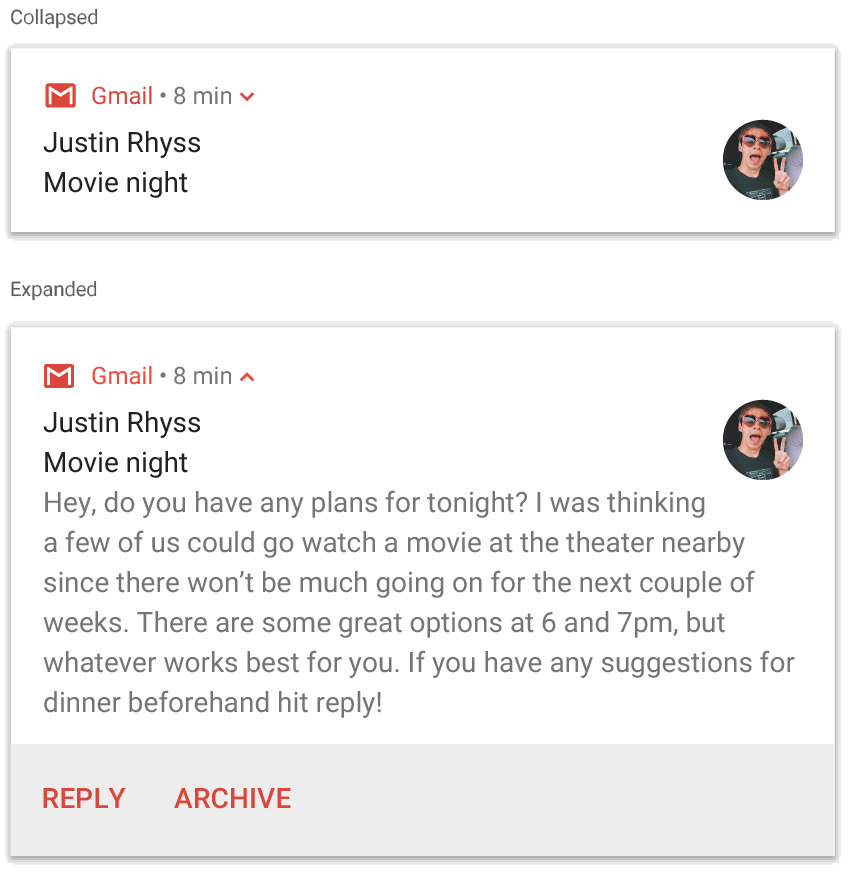
NotificationCompat.BigTextStyle
.
Create an inbox-style notification
Apply
NotificationCompat.InboxStyle
to a notification if you want to add multiple short summary lines, such as
snippets from incoming emails. This lets you add multiple pieces of content text
that are each truncated to one line, instead of the one continuous line of text
provided by NotificationCompat.BigTextStyle
.
To add a new line, call
addLine()
up to six times, as shown in the following example. If you add more than six
lines, only the first six are visible.
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_email_24) .setContentTitle("5 New mails from Frank") .setContentText("Check them out") .setLargeIcon(BitmapFactory.decodeResource(resources, R.drawable.logo)) .setStyle( NotificationCompat.InboxStyle() .addLine("Re: Planning") .addLine("Delivery on its way") .addLine("Follow-up") ) .build()
Java
Notification notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_email_24) .setContentTitle("5 New mails from Frank") .setContentText("Check them out") .setLargeIcon(BitmapFactory.decodeResource(resources, R.drawable.logo)) .setStyle( NotificationCompat.InboxStyle() .addLine("Re: Planning") .addLine("Delivery on its way") .addLine("Follow-up") ) .build();
The result looks like the following figure:
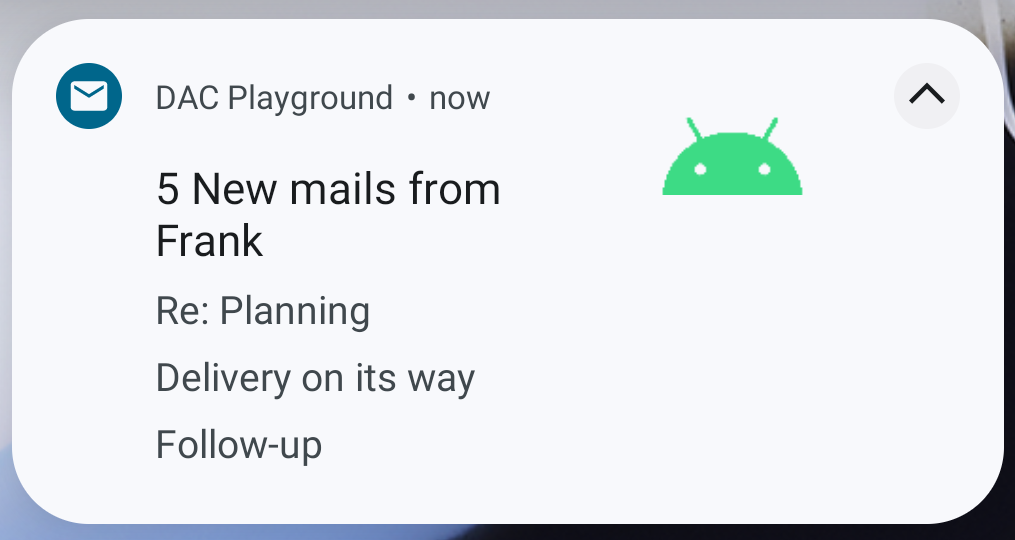
Show a conversation in a notification
Apply
NotificationCompat.MessagingStyle
to display sequential messages between any number of people. This is ideal for
messaging apps, because it provides a consistent layout for each message by
handling the sender name and message text separately, and each message can be
multiple lines long.
To add a new message, call
addMessage()
,
passing the message text, the time received, and the sender's name. You can also
pass this information as a
NotificationCompat.MessagingStyle.Message
object, as shown in the following example:
Kotlin
val message1 = NotificationCompat.MessagingStyle.Message( messages[0].getText(), messages[0].getTime(), messages[0].getSender()) val message2 = NotificationCompat.MessagingStyle.Message( messages[1].getText(), messages[1].getTime(), messages[1].getSender()) val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_message) .setStyle( NotificationCompat.MessagingStyle(resources.getString(R.string.reply_name)) .addMessage(message1) .addMessage(message2)) .build()
Java
NotificationCompat.MessagingStyle.Message message1 = new NotificationCompat.MessagingStyle.Message(messages[0].getText(), messages[0].getTime(), messages[0].getSender()); NotificationCompat.MessagingStyle.Message message2 = new NotificationCompat.MessagingStyle.Message(messages[1].getText(), messages[1].getTime(), messages[1].getSender()); Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_message) .setStyle(new NotificationCompat.MessagingStyle(resources.getString(R.string.reply_name)) .addMessage(message1) .addMessage(message2)) .build();
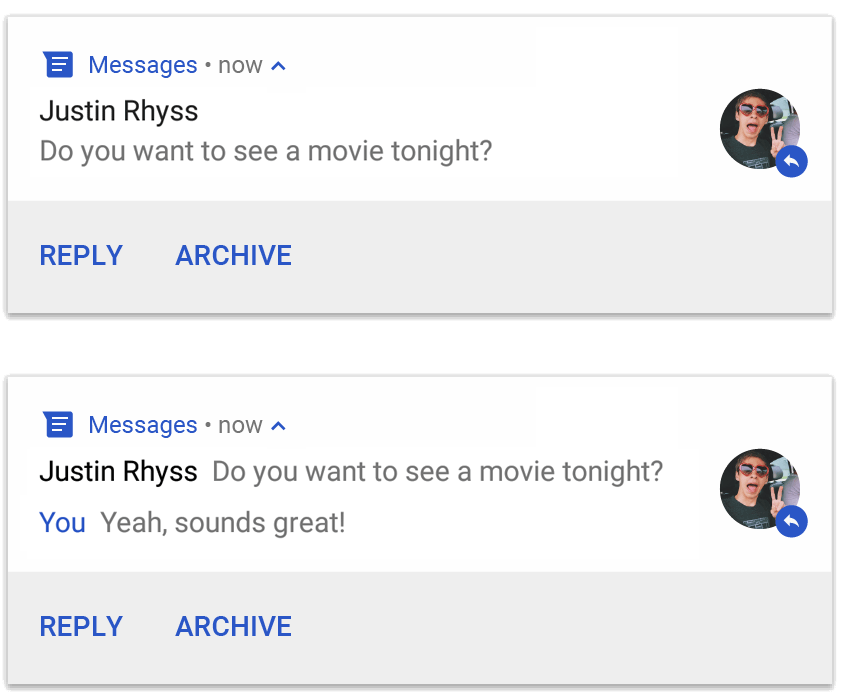
NotificationCompat.MessagingStyle
.
When using NotificationCompat.MessagingStyle
, any values given to
setContentTitle()
and
setContentText()
are ignored.
You can call
setConversationTitle()
to add a title that appears above the conversation. This might be the
user-created name of the group or, if it doesn't have a specific name, a list of
the participants in the conversation. Don't set a conversation title for
one-on-one chats, because the system uses the existence of this field as a hint
that the conversation is a group.
This style applies only on devices running Android 7.0 (API level 24) and later.
When using the compatibility library
(NotificationCompat
),
as demonstrated earlier, notifications with MessagingStyle
fall back
automatically to a supported expanded notification style.
When building a notification like this for a chat conversation, add a direct reply action.
Create a notification with media controls
Apply
MediaStyleNotificationHelper.MediaStyle
to display media playback controls and track information.
Specify your associated
MediaSession
in the
constructor. This allows Android to display the right information about your
media.
Call
addAction()
up to five times to display up to five icon buttons. Call setLargeIcon()
to
set the album artwork.
Unlike the other notification styles, MediaStyle
also lets you modify the
collapsed-size content view by specifying three action buttons that also appear
in the collapsed view. To do so, provide the action button indices to
setShowActionsInCompactView()
.
The following example shows how to create a notification with media controls:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) // Show controls on lock screen even when user hides sensitive content. .setVisibility(NotificationCompat.VISIBILITY_PUBLIC) .setSmallIcon(R.drawable.ic_stat_player) // Add media control buttons that invoke intents in your media service .addAction(R.drawable.ic_prev, "Previous", prevPendingIntent) // #0 .addAction(R.drawable.ic_pause, "Pause", pausePendingIntent) // #1 .addAction(R.drawable.ic_next, "Next", nextPendingIntent) // #2 // Apply the media style template. .setStyle(MediaStyleNotificationHelper.MediaStyle(mediaSession) .setShowActionsInCompactView(1 /* #1: pause button \*/)) .setContentTitle("Wonderful music") .setContentText("My Awesome Band") .setLargeIcon(albumArtBitmap) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) // Show controls on lock screen even when user hides sensitive content. .setVisibility(NotificationCompat.VISIBILITY_PUBLIC) .setSmallIcon(R.drawable.ic_stat_player) // Add media control buttons that invoke intents in your media service .addAction(R.drawable.ic_prev, "Previous", prevPendingIntent) // #0 .addAction(R.drawable.ic_pause, "Pause", pausePendingIntent) // #1 .addAction(R.drawable.ic_next, "Next", nextPendingIntent) // #2 // Apply the media style template. .setStyle(new MediaStyleNotificationHelper.MediaStyle(mediaSession) .setShowActionsInCompactView(1 /* #1: pause button */)) .setContentTitle("Wonderful music") .setContentText("My Awesome Band") .setLargeIcon(albumArtBitmap) .build();
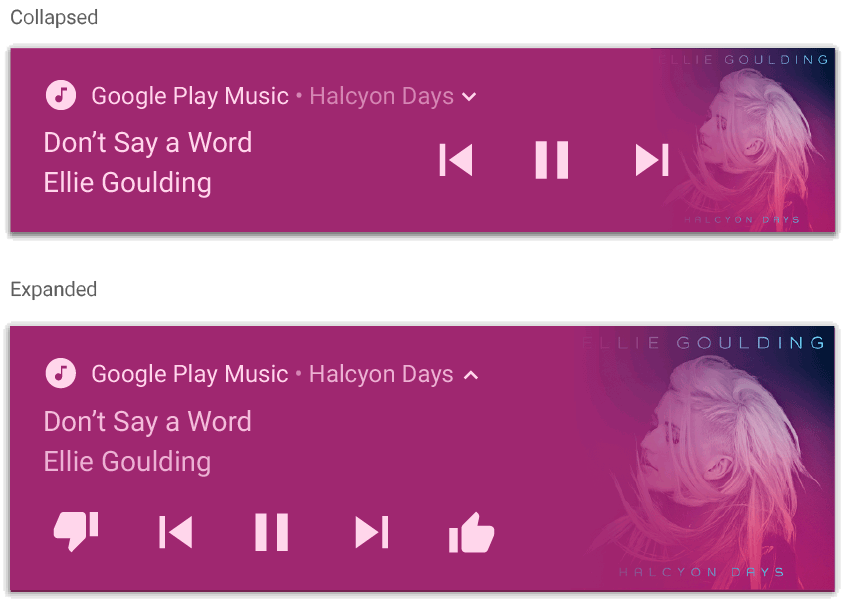
MediaStyleNotificationHelper.MediaStyle
.
Additional resources
See the following references for more information about MediaStyle
and
expandable notifications.