Em determinadas situações, seu app pode precisar chamar a atenção do usuário com urgência, por exemplo, para um alarme ativado ou uma chamada recebida. Em apps destinados a dispositivos que executam o Android 9 (nível 28 da API) ou versões anteriores, é possível iniciar uma atividade enquanto o app está em segundo plano. Este documento mostra como alcançar esse comportamento em dispositivos com o Android 10 (nível 29 da API) ao Android 13 (nível 33 da API).
Adicionar a permissão POST_NOTES
A partir do Android 13, adicione a linha abaixo ao
Arquivo AndroidManifest.xml
:
<manifest ...> <uses-permission android:name="android.permission.POST_NOTIFICATIONS"/> <application ...> ... </application> </manifest>
Depois de fazer isso, você pode criar um canal de notificação.
Criar um canal de notificação
Crie um canal de notificação para mostrar suas notificações corretamente e permitir que o usuário gerencie as notificações nas configurações do app. Para saber mais sobre canais de notificação, consulte Criar e gerenciar canais de notificação.
Crie seus canais de notificação na classe Application
onCreate
:
Kotlin
class DACapp : Application() { override fun onCreate() { super.onCreate() val channel = NotificationChannel( CHANNEL_ID, "High priority notifications", NotificationManager.IMPORTANCE_HIGH ) val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager notificationManager.createNotificationChannel(channel) } }
Quando o usuário executa o app pela primeira vez, ele vê algo como a Figura 1 na tela do sistema Informações do app:
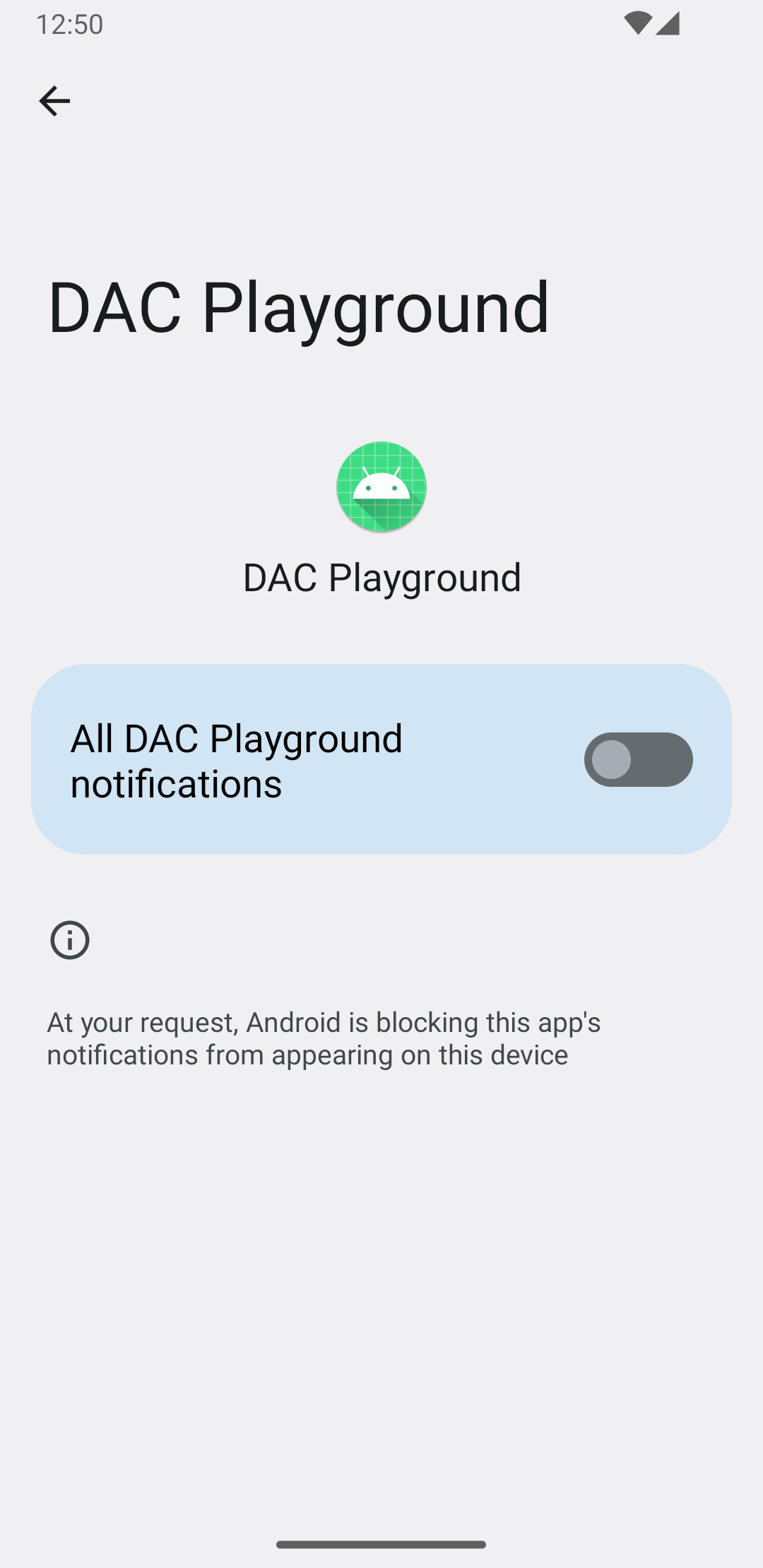
Gerenciar permissões de notificações
A partir do Android 13, solicite permissões de notificação antes de mostrar notificações aos usuários.
A implementação mínima fica assim:
Kotlin
val permissionLauncher = rememberLauncherForActivityResult( contract = ActivityResultContracts.RequestPermission(), onResult = { hasNotificationPermission = it } ) ... Button( onClick = { if (!hasNotificationPermission) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU) { permissionLauncher.launch(Manifest.permission.POST_NOTIFICATIONS) } } }, ) { Text(text = "Request permission") }
Se o dispositivo estiver executando o Android 13, tocar no botão Request
permission
vai acionar a caixa de diálogo mostrada na Figura 2:
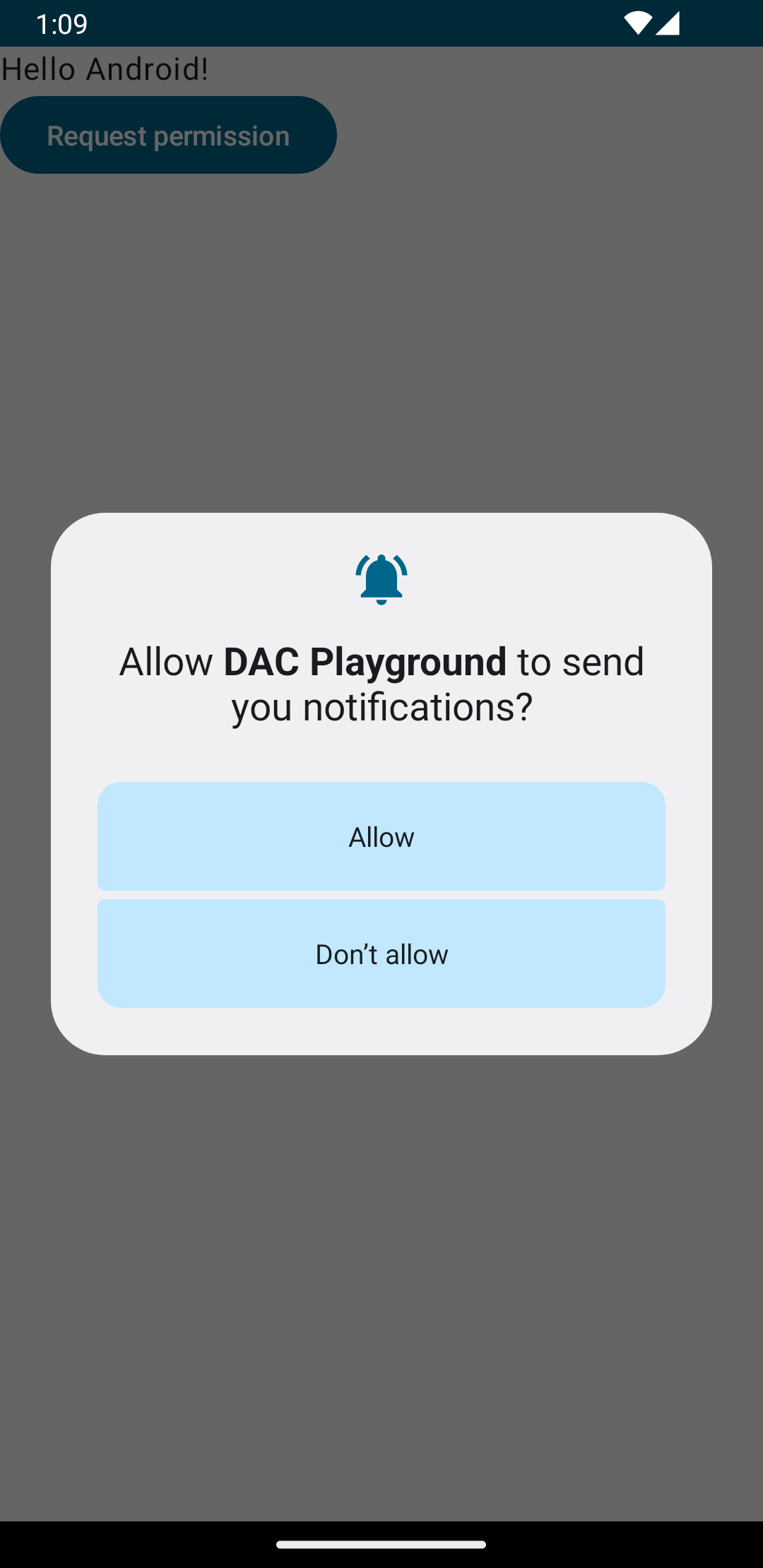
Se o usuário aceitar a solicitação de permissão, a seção Informações do app vai ficar como na Figura 3:
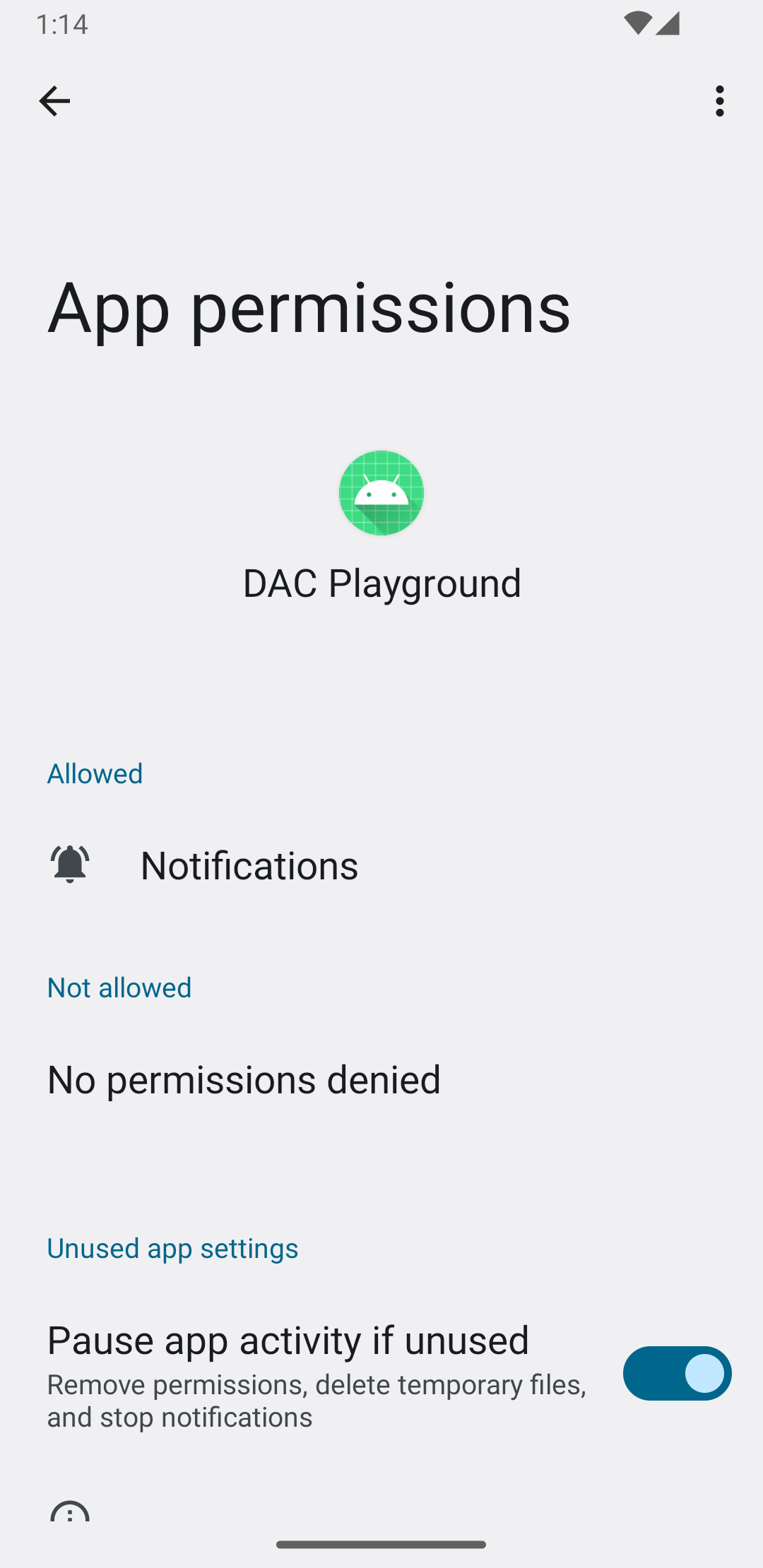
Criar uma notificação de prioridade alta
Ao criar a notificação, inclua um título e uma mensagem descritivos.
O exemplo a seguir contém uma notificação:
Kotlin
private fun showNotification() { val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager val notificationBuilder = NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_auto_awesome_24) .setContentTitle("HIGH PRIORITY") .setContentText("Check this dog puppy video NOW!") .setPriority(NotificationCompat.PRIORITY_HIGH) .setCategory(NotificationCompat.CATEGORY_RECOMMENDATION) notificationManager.notify(666, notificationBuilder.build()) }
Exibir a notificação para o usuário
Chamar a função showNotification()
aciona a notificação da seguinte maneira:
Kotlin
Button(onClick = { showNotification() }) { Text(text = "Show notification") }
A notificação neste exemplo é semelhante à Figura 4:
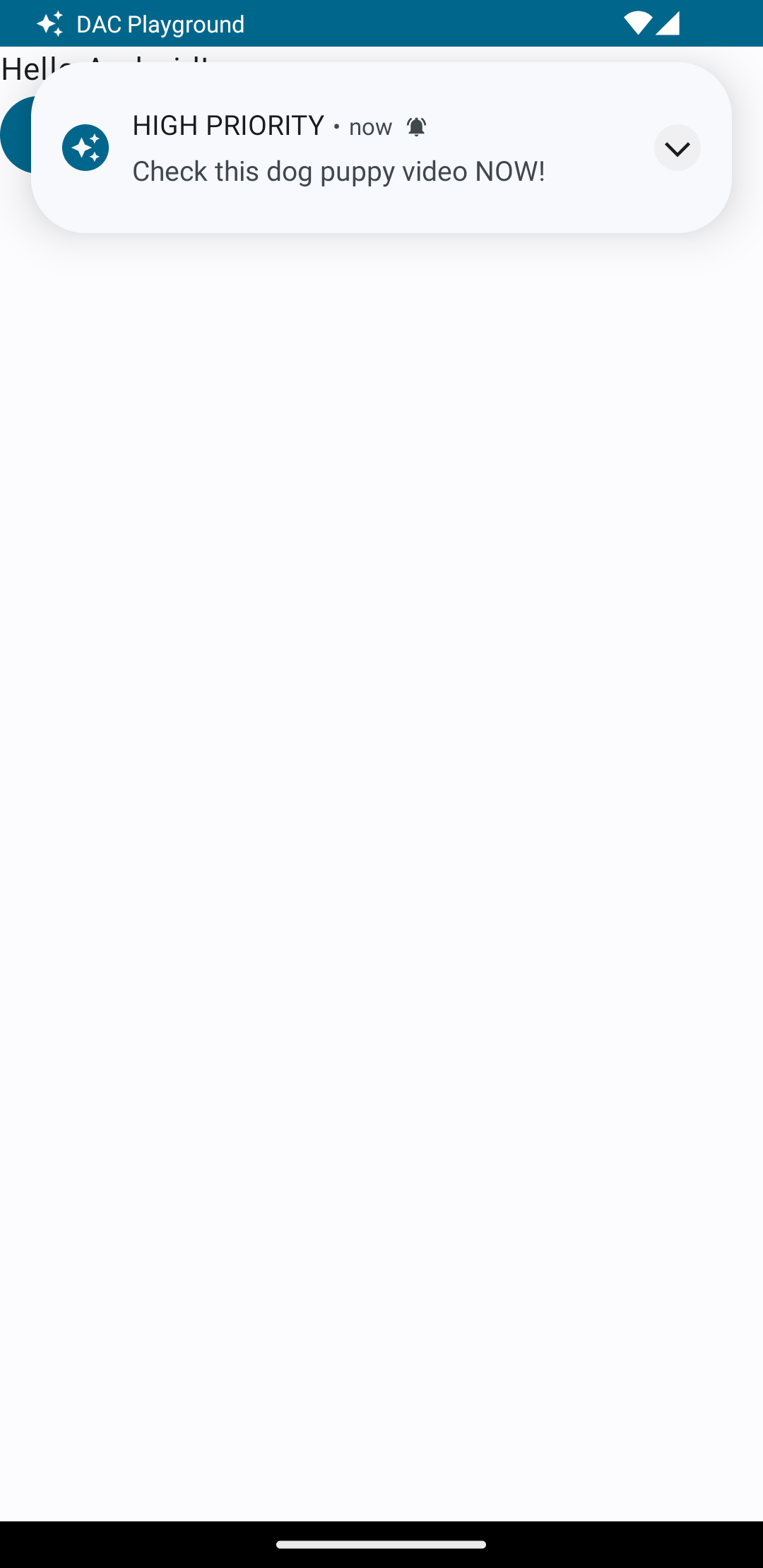
Notificação em andamento
Quando você mostra a notificação para o usuário, ele pode confirmar ou dispensar o alerta ou lembrete do app. Por exemplo, o usuário pode aceitar ou rejeitar um uma chamada telefônica recebida.
Se a notificação for contínua, como uma chamada telefônica recebida, associe a notificação a um serviço em primeiro plano. O snippet de código abaixo mostra como exibir uma notificação associada a um serviço em primeiro plano:
Kotlin
// Provide a unique integer for the "notificationId" of each notification. startForeground(notificationId, notification)
Java
// Provide a unique integer for the "notificationId" of each notification. startForeground(notificationId, notification);