לעתים קרובות, אפליקציות צריכות להציג נתונים במאגרים באותו סגנון, כמו מאגרים שמכילים מידע על הפריטים ברשימה. המערכת מספקת את ה-API CardView
כדי שתוכלו להציג מידע בכרטיסים עם מראה עקבי בכל הפלטפורמה. לדוגמה, לכרטיסים יש גובה ברירת מחדל מעל קבוצת התצוגה שמכילה אותם, ולכן המערכת מצייר צללים מתחת לכרטיסים. הכרטיסים מאפשרים להכיל קבוצה של תצוגות מפורטות תוך שמירה על סגנון עקבי של הקונטיינר.
מוסיפים את יחסי התלות
הווידג'ט CardView
הוא חלק מ-AndroidX. כדי להשתמש בו בפרויקט, מוסיפים את התלות הבאה לקובץ build.gradle
של מודול האפליקציה:
מגניב
dependencies { implementation "androidx.cardview:cardview:1.0.0" }
Kotlin
dependencies { implementation("androidx.cardview:cardview:1.0.0") }
יצירת כרטיסים
כדי להשתמש בקובץ CardView
, צריך להוסיף אותו לקובץ הפריסה. אפשר להשתמש בו כקבוצת תצוגות כדי להכיל תצוגות אחרות. בדוגמה הבאה, ה-CardView
מכיל ImageView
וכמה TextViews
כדי להציג מידע מסוים למשתמש:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:padding="16dp"
android:background="#E0F7FA"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.cardview.widget.CardView
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<androidx.constraintlayout.widget.ConstraintLayout
android:padding="4dp"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/header_image"
android:layout_width="match_parent"
android:layout_height="200dp"
android:src="@drawable/logo"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/title"
style="@style/TextAppearance.MaterialComponents.Headline3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="I'm a title"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/header_image" />
<TextView
android:id="@+id/subhead"
style="@style/TextAppearance.MaterialComponents.Subtitle2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="I'm a subhead"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/title" />
<TextView
android:id="@+id/body"
style="@style/TextAppearance.MaterialComponents.Body1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="I'm a supporting text. Very Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum."
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/subhead" />
</androidx.constraintlayout.widget.ConstraintLayout>
</androidx.cardview.widget.CardView>
</androidx.constraintlayout.widget.ConstraintLayout>
קטע הקוד הקודם יוצר משהו שדומה לזה, בהנחה שמשתמשים באותה תמונה של הלוגו של Android:
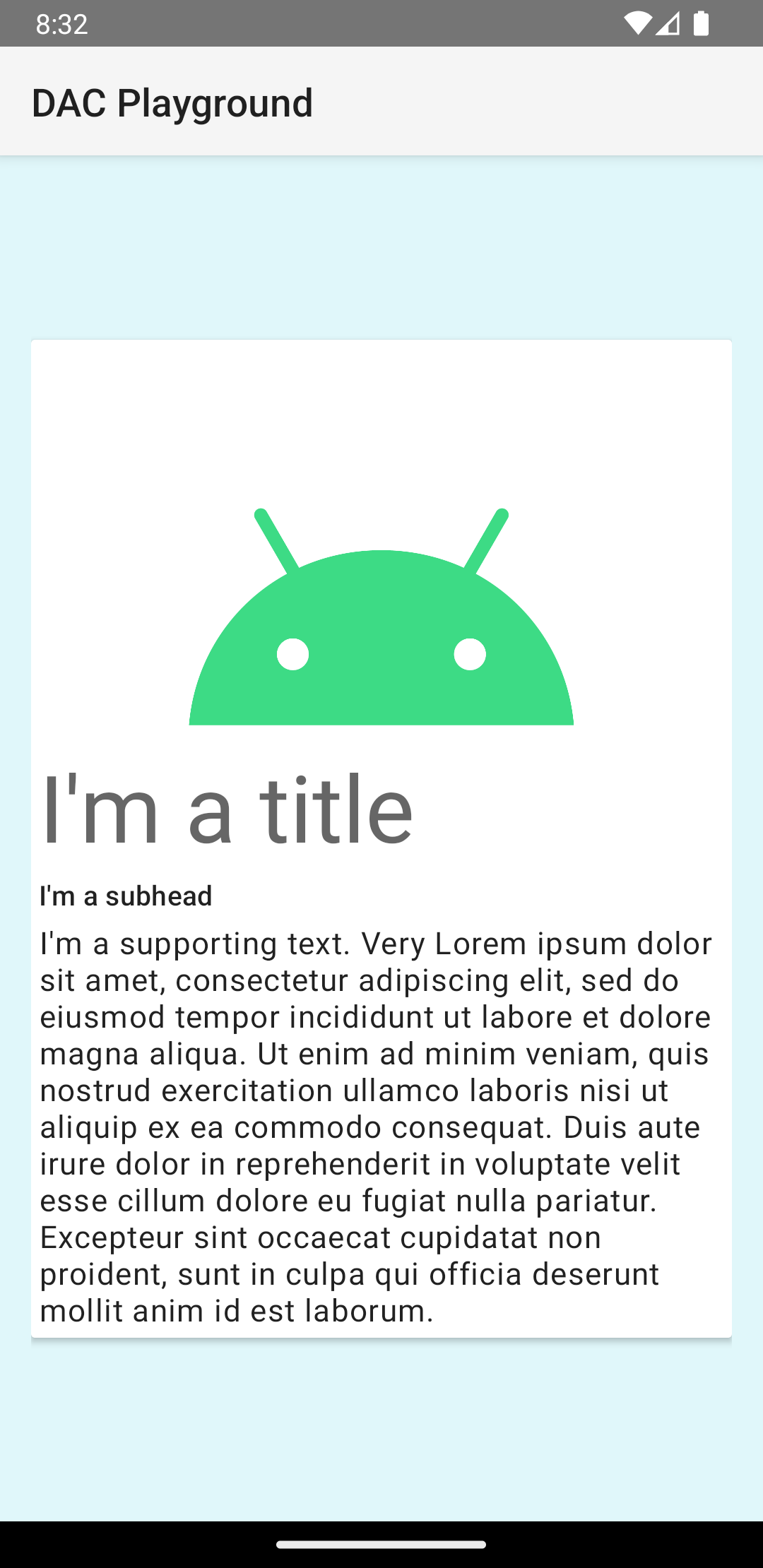
הכרטיס בדוגמה הזו מוצג במסך עם גובה ברירת מחדל, וכתוצאה מכך המערכת מציירת צל מתחתיו. אפשר לספק גובה מותאם אישית לכרטיס באמצעות המאפיין card_view:cardElevation
. כרטיס שנמצא בגובה גבוה יותר יקבל צללית בולטת יותר, וכרטיס שנמצא בגובה נמוך יותר יקבל צללית בהירה יותר. CardView
משתמש בגובה אמיתי ובצללים דינמיים ב-Android 5.0 (רמת API 21) ואילך.
המאפיינים הבאים מאפשרים לכם להתאים אישית את המראה של הווידג'ט CardView
:
- כדי להגדיר את רדיוס הפינות בפריסות, משתמשים במאפיין
card_view:cardCornerRadius
. - כדי להגדיר את רדיוס הפינה בקוד, משתמשים בשיטה
CardView.setRadius
. - כדי להגדיר את צבע הרקע של כרטיס, משתמשים במאפיין
card_view:cardBackgroundColor
.