ऐप्लिकेशन को अक्सर समान स्टाइल वाले कंटेनर में डेटा दिखाना पड़ता है, जैसे
कंटेनर, जिनमें सूची में मौजूद आइटम के बारे में जानकारी होती है. यह सिस्टम,
CardView
एपीआई का इस्तेमाल करके
उस जानकारी को कार्ड में दिखाएं जो पूरे प्लैटफ़ॉर्म पर एक जैसी दिखती है. इसके लिए
उदाहरण के लिए, कार्ड में उनके मौजूदा व्यू ग्रुप के ऊपर डिफ़ॉल्ट ऊंचाई होती है, इसलिए
तो सिस्टम उनके नीचे परछाई बना लेता है. कार्ड एक तरीका प्रदान करते हैं, जिसमें
व्यू, कंटेनर के लिए एक ही स्टाइल में दिखते हैं.
डिपेंडेंसी जोड़ें
CardView
विजेट, AndroidX का हिस्सा है. इसका इस्तेमाल इसमें करने के लिए
अपने ऐप्लिकेशन मॉड्यूल के build.gradle
में नीचे दी गई डिपेंडेंसी जोड़ें
फ़ाइल:
Groovy
dependencies { implementation "androidx.cardview:cardview:1.0.0" }
Kotlin
dependencies { implementation("androidx.cardview:cardview:1.0.0") }
कार्ड बनाएं
CardView
का इस्तेमाल करने के लिए, इसे अपनी लेआउट फ़ाइल में जोड़ें. इसे व्यू ग्रुप के तौर पर इस्तेमाल करके,
अन्य दृश्य शामिल होते हैं. यहां दिए गए उदाहरण में, CardView
में
उपयोगकर्ता को कुछ जानकारी दिखाने के लिए ImageView
और कुछ TextViews
:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:padding="16dp"
android:background="#E0F7FA"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.cardview.widget.CardView
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<androidx.constraintlayout.widget.ConstraintLayout
android:padding="4dp"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/header_image"
android:layout_width="match_parent"
android:layout_height="200dp"
android:src="@drawable/logo"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/title"
style="@style/TextAppearance.MaterialComponents.Headline3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="I'm a title"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/header_image" />
<TextView
android:id="@+id/subhead"
style="@style/TextAppearance.MaterialComponents.Subtitle2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="I'm a subhead"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/title" />
<TextView
android:id="@+id/body"
style="@style/TextAppearance.MaterialComponents.Body1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="I'm a supporting text. Very Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum."
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/subhead" />
</androidx.constraintlayout.widget.ConstraintLayout>
</androidx.cardview.widget.CardView>
</androidx.constraintlayout.widget.ConstraintLayout>
पिछला कोड स्निपेट यह मानते हुए नीचे दिया गया कुछ मिलता-जुलता बनाता है Android के लोगो वाली इमेज का इस्तेमाल किया जा रहा है:
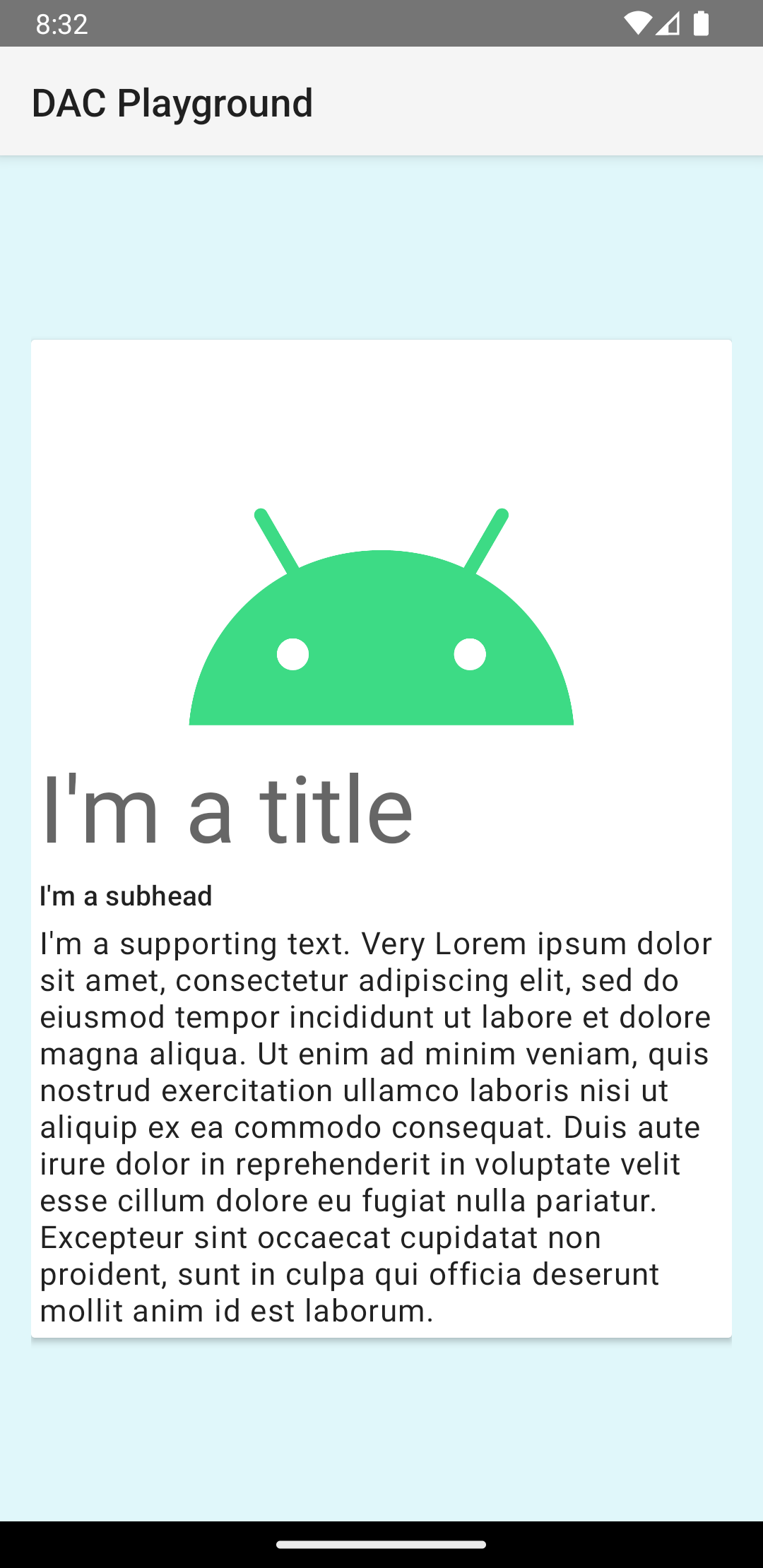
इस उदाहरण में दिया गया कार्ड, डिफ़ॉल्ट ऊंचाई के साथ स्क्रीन पर ड्रॉ किया गया है, जो
इससे सिस्टम इसके नीचे परछाई बना देता है. आप कस्टम ऊंचाई प्रदान कर सकते हैं
card_view:cardElevation
एट्रिब्यूट वाले कार्ड के लिए. इससे ज़्यादा ऊपर दिखने वाला कार्ड
ऊंचाई पर परछाई ज़्यादा साफ़ होती है और कम ऊंचाई पर बने कार्ड में
हल्का शैडो. CardView
, Android पर असल ऊंचाई और डाइनैमिक शैडो का इस्तेमाल करता है
5.0 (एपीआई लेवल 21) और उसके बाद के वर्शन.
CardView
विजेट के लुक को पसंद के मुताबिक बनाने के लिए, इन प्रॉपर्टी का इस्तेमाल करें:
- अपने लेआउट में कोने का दायरा सेट करने के लिए,
card_view:cardCornerRadius
का इस्तेमाल करें एट्रिब्यूट की वैल्यू सबमिट करें. - अपने कोड में कोने का दायरा सेट करने के लिए,
CardView.setRadius
तरीके का इस्तेमाल करें. - किसी कार्ड के बैकग्राउंड का रंग सेट करने के लिए,
card_view:cardBackgroundColor
का इस्तेमाल करें एट्रिब्यूट की वैल्यू सबमिट करें.