在某些情況下,應用程式可能需要緊急引起使用者的注意,例如鬧鐘響起或來電。在指定搭載 Android 9 (API 級別 28) 以下版本的裝置的應用程式中,您可以透過在應用程式處於背景執行時啟動活動來處理此問題。本文件說明如何在搭載 Android 10 (API 級別 29) 至 Android 13 (API 級別 33) 的裝置上實現這項行為。
新增 POST_NOTIFICATIONS 權限
從 Android 13 開始,請在 AndroidManifest.xml
檔案中加入下列程式碼行:
<manifest ...> <uses-permission android:name="android.permission.POST_NOTIFICATIONS"/> <application ...> ... </application> </manifest>
取得這項資訊後,您就可以建立通知管道。
建立通知管道
建立通知管道,正確顯示通知,並讓使用者在應用程式設定中管理通知。如要進一步瞭解通知管道,請參閱「建立及管理通知管道」。
在 Application
類別的 onCreate
方法中建立通知管道:
Kotlin
class DACapp : Application() { override fun onCreate() { super.onCreate() val channel = NotificationChannel( CHANNEL_ID, "High priority notifications", NotificationManager.IMPORTANCE_HIGH ) val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager notificationManager.createNotificationChannel(channel) } }
使用者首次執行應用程式時,會在應用程式的「應用程式資訊」系統畫面中看到類似圖 1 的畫面:
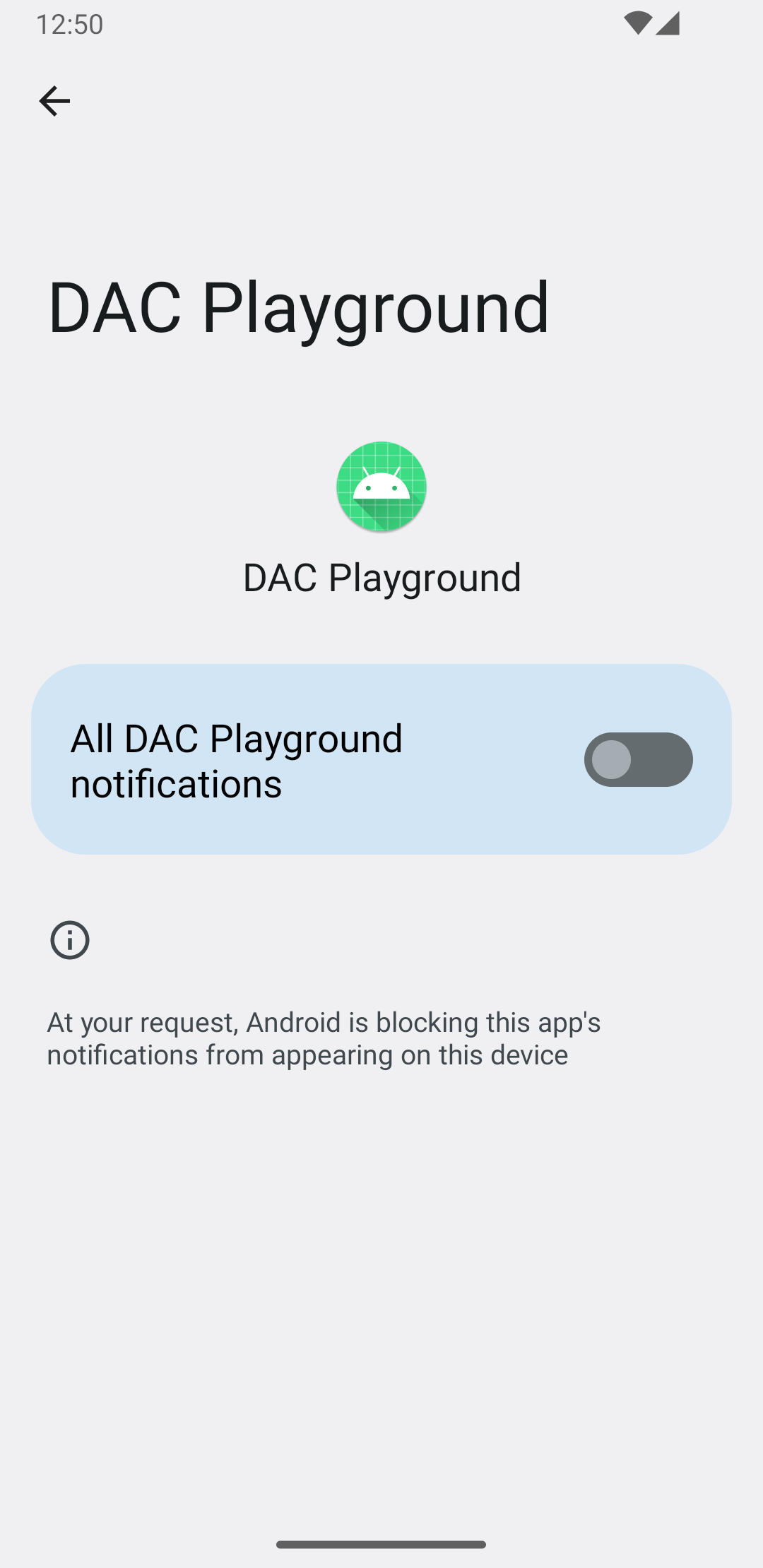
管理通知權限
從 Android 13 開始,您必須先向使用者要求通知權限,才能向他們顯示通知。
最少實作內容如下所示:
Kotlin
val permissionLauncher = rememberLauncherForActivityResult( contract = ActivityResultContracts.RequestPermission(), onResult = { hasNotificationPermission = it } ) ... Button( onClick = { if (!hasNotificationPermission) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU) { permissionLauncher.launch(Manifest.permission.POST_NOTIFICATIONS) } } }, ) { Text(text = "Request permission") }
如果裝置搭載 Android 13,輕觸 Request
permission
按鈕會觸發圖 2 所示的對話方塊:
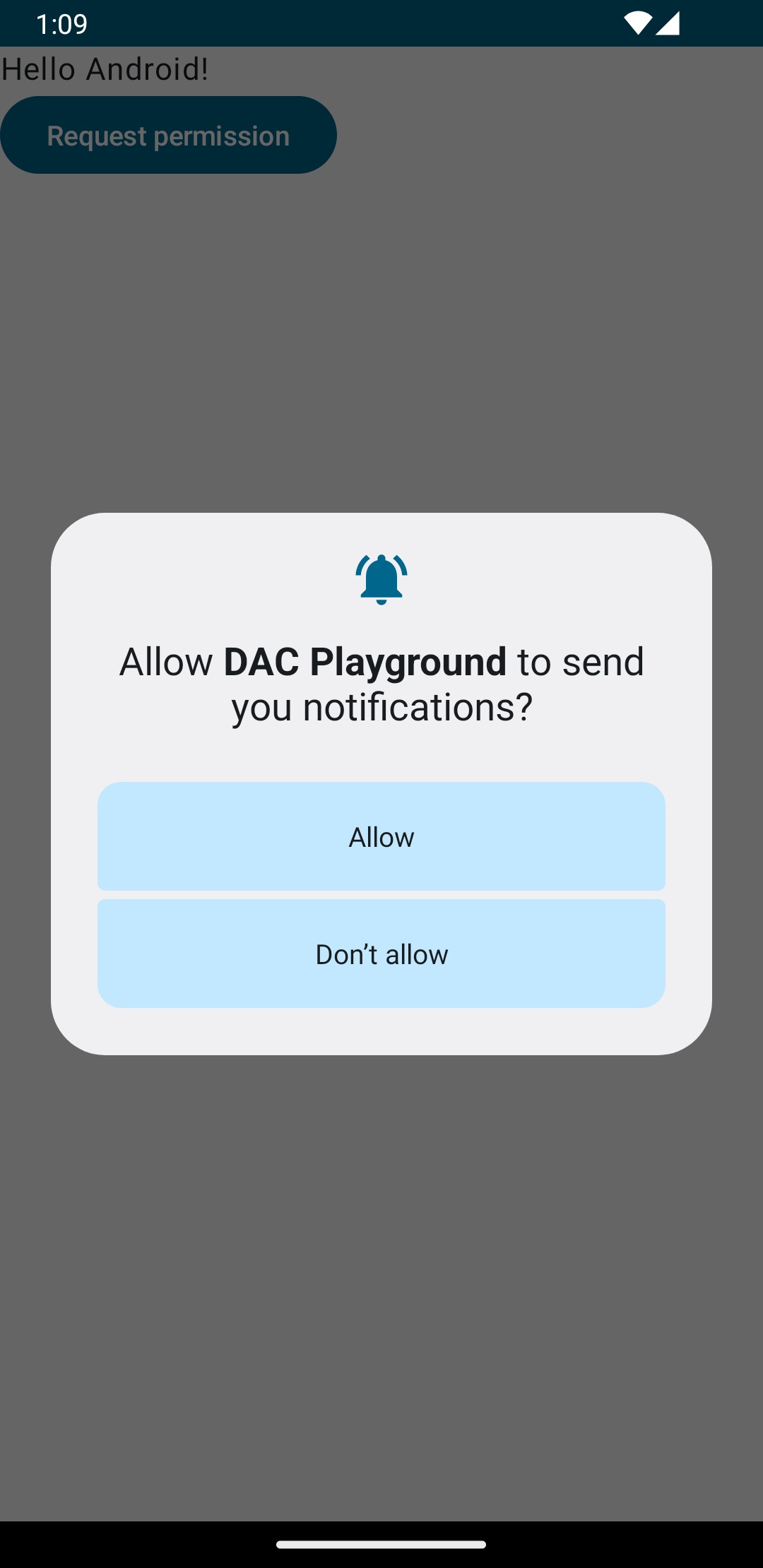
如果使用者接受權限要求,應用程式的「應用程式資訊」部分會如下圖 3 所示:
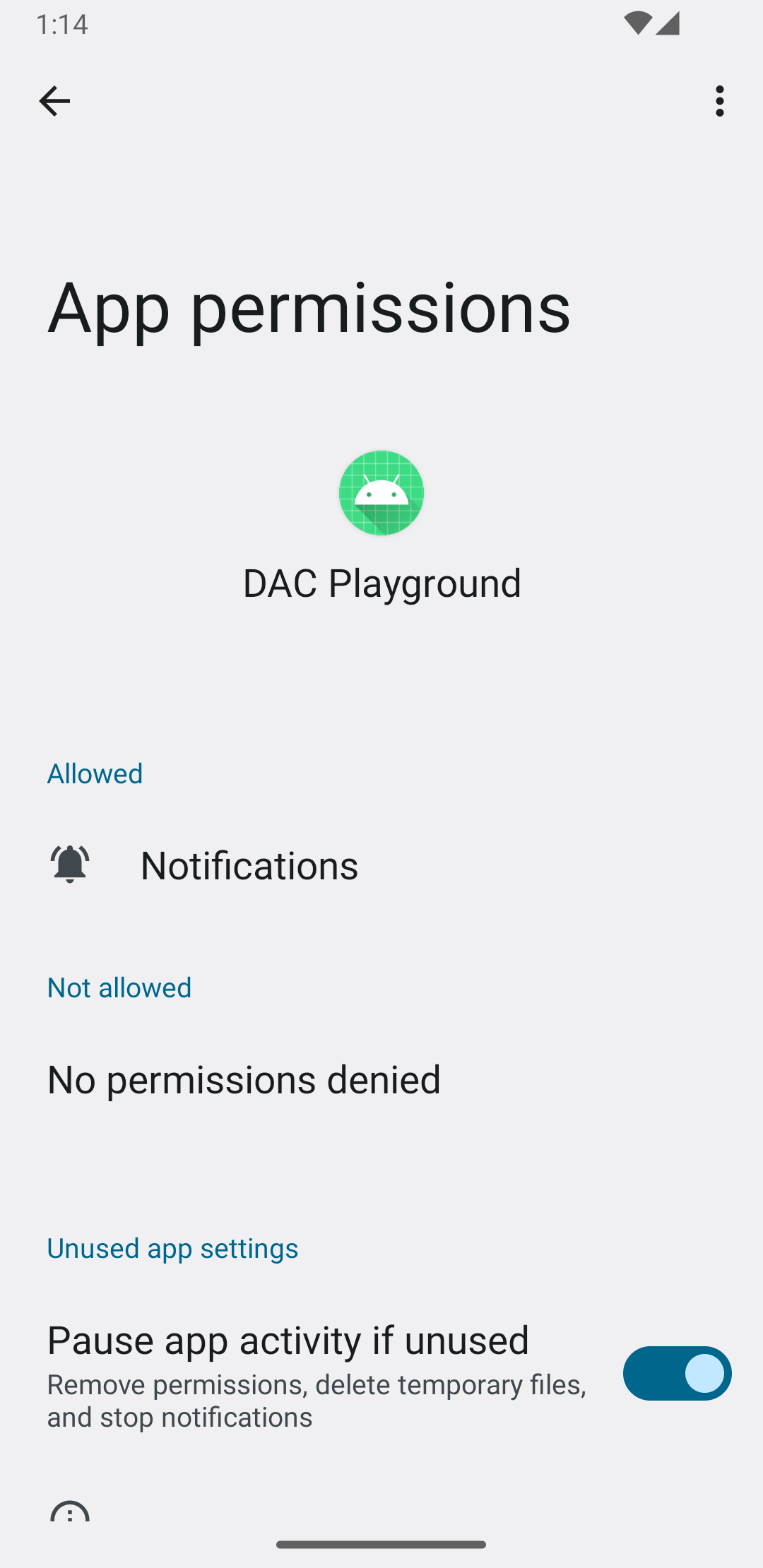
建立高優先順序通知
建立通知時,請附上說明性標題和訊息。
以下範例包含通知:
Kotlin
private fun showNotification() { val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager val notificationBuilder = NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_auto_awesome_24) .setContentTitle("HIGH PRIORITY") .setContentText("Check this dog puppy video NOW!") .setPriority(NotificationCompat.PRIORITY_HIGH) .setCategory(NotificationCompat.CATEGORY_RECOMMENDATION) notificationManager.notify(666, notificationBuilder.build()) }
向使用者顯示通知
呼叫 showNotification()
函式會觸發以下通知:
Kotlin
Button(onClick = { showNotification() }) { Text(text = "Show notification") }
本例中的通知如下圖 4 所示:
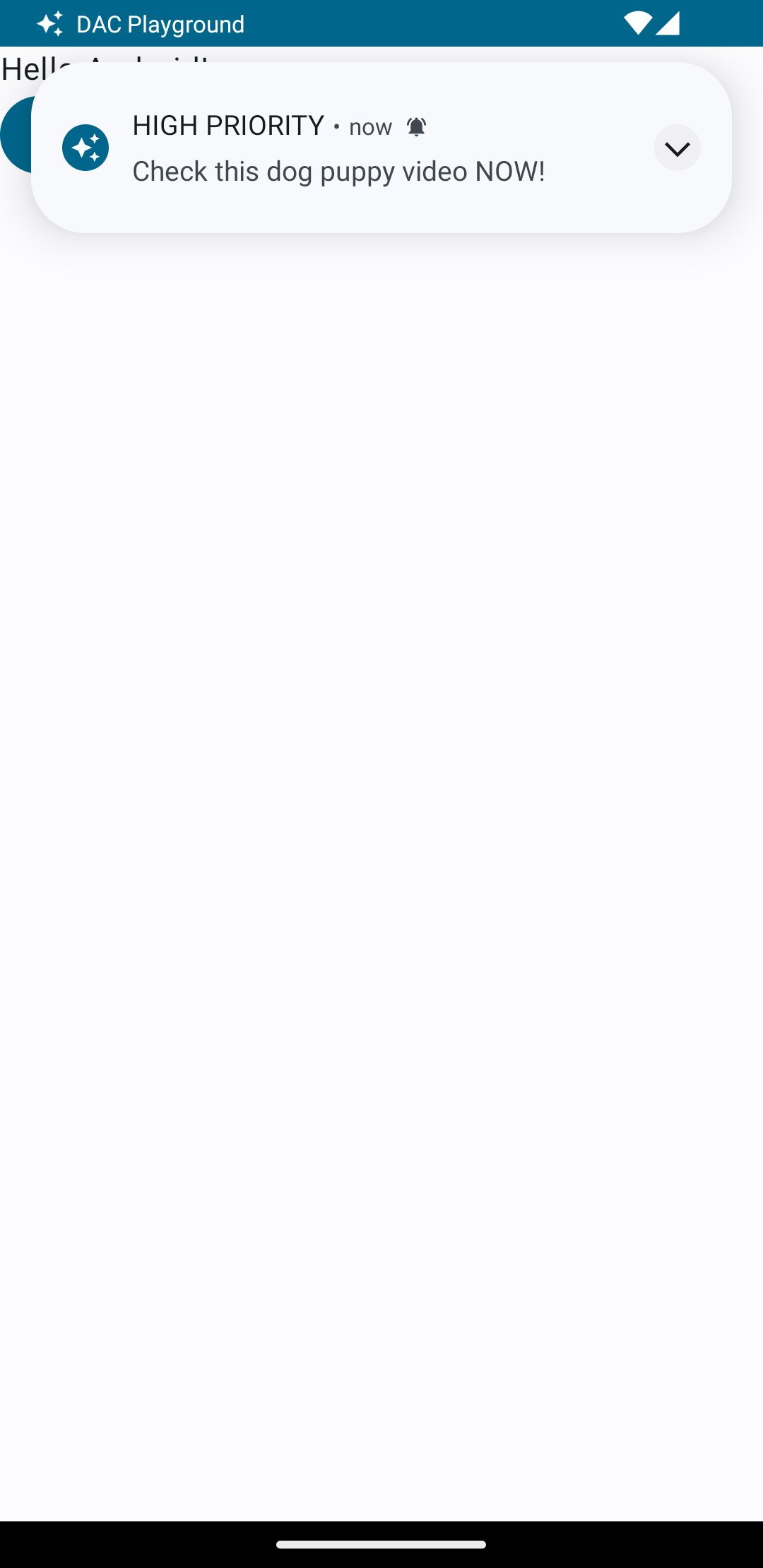
持續性通知
向使用者顯示通知時,他們可以確認或關閉應用程式的警示或提醒。舉例來說,使用者可以接聽或拒接來電。
如果通知是持續性通知 (例如來電),請將通知與前景服務建立關聯。以下程式碼片段說明如何顯示與前景服務相關聯的通知:
Kotlin
// Provide a unique integer for the "notificationId" of each notification. startForeground(notificationId, notification)
Java
// Provide a unique integer for the "notificationId" of each notification. startForeground(notificationId, notification);