The app bar lets you add buttons for user actions. This feature lets you put the most important actions for the current context at the top of the app. For example, a photo browsing app might show share and create album buttons at the top when the user is looking at their photo roll. When the user looks at an individual photo, the app might show crop and filter buttons.
Space in the app bar is limited. If an app declares more actions than can fit in the app bar, the app bar sends the excess actions to an overflow menu. The app can also specify that an action always shows in the overflow menu, instead of displaying on the app bar.
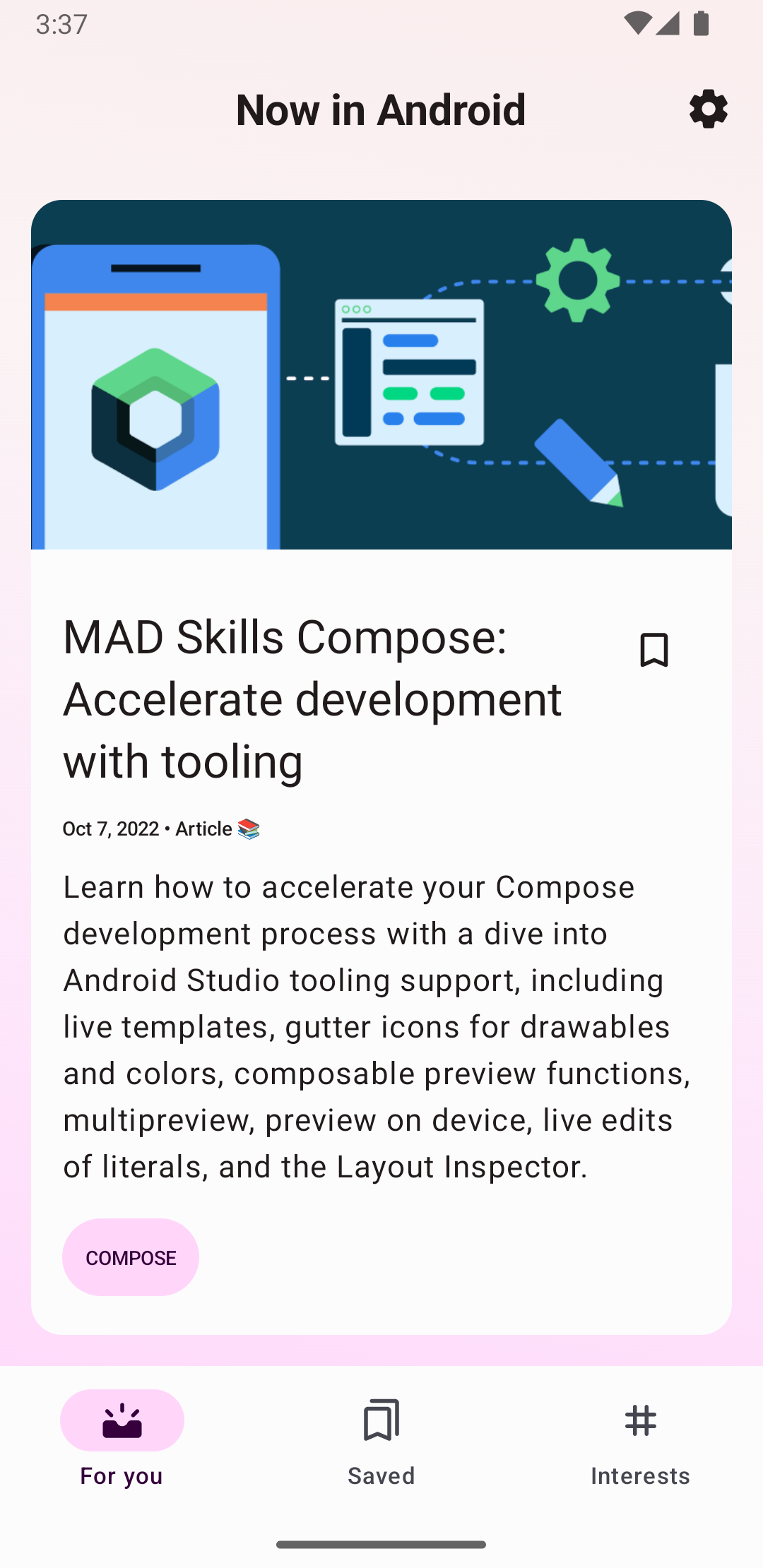
Add action buttons
All action buttons and other items available in the action overflow are
defined in an XML
menu resource. To add
actions to the action bar, create a new XML file in your project's
res/menu/
directory.
Add an
<item>
element for each item you want to include in the action bar, as shown in the
following sample menu XML file:
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto"> <!-- "Mark Favorite", must appear as action button if possible. --> <item android:id="@+id/action_favorite" android:icon="@drawable/ic_favorite_black_48dp" android:title="@string/action_favorite" app:showAsAction="ifRoom"/> <!-- Settings, must always be in the overflow. --> <item android:id="@+id/action_settings" android:title="@string/action_settings" app:showAsAction="never"/> </menu>
The app:showAsAction
attribute specifies whether the action is
shown as a button on the app bar. If you set
app:showAsAction="ifRoom"
—as in the example code's
favorite action—the action displays as a button if there is room in
the app bar for it. If there isn't enough room, excess actions are sent to the
overflow menu. If you set app:showAsAction="never"
—as in the
example code's settings action—the action is always listed in the
overflow menu and not displayed in the app bar.
The system uses the action's icon as the action button if the action displays in the app bar. You can find many useful icons in Material Icons.
Respond to actions
When the user selects one of the app bar items, the system calls your
activity's
onOptionsItemSelected()
callback method and passes a
MenuItem
object
to indicate which item was tapped. In your implementation of
onOptionsItemSelected()
, call the
MenuItem.getItemId()
method to determine which item was tapped. The ID returned matches the value you
declare in the corresponding <item>
element's
android:id
attribute.
For example, the following code snippet checks which action the user selects. If the method doesn't recognize the user's action, it invokes the superclass method:
Kotlin
override fun onOptionsItemSelected(item: MenuItem) = when (item.itemId) { R.id.action_settings -> { // User chooses the "Settings" item. Show the app settings UI. true } R.id.action_favorite -> { // User chooses the "Favorite" action. Mark the current item as a // favorite. true } else -> { // The user's action isn't recognized. // Invoke the superclass to handle it. super.onOptionsItemSelected(item) } }
Java
@Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case R.id.action_settings: // User chooses the "Settings" item. Show the app settings UI. return true; case R.id.action_favorite: // User chooses the "Favorite" action. Mark the current item as a // favorite. return true; default: // The user's action isn't recognized. // Invoke the superclass to handle it. return super.onOptionsItemSelected(item); } }