In Figma, variants are used to group different variations of the same component together, such as different states or sizes. Relay preserves a component’s variants when it is translated to code. In this section, we’ll see how Relay handles variants in designs.
Starting point
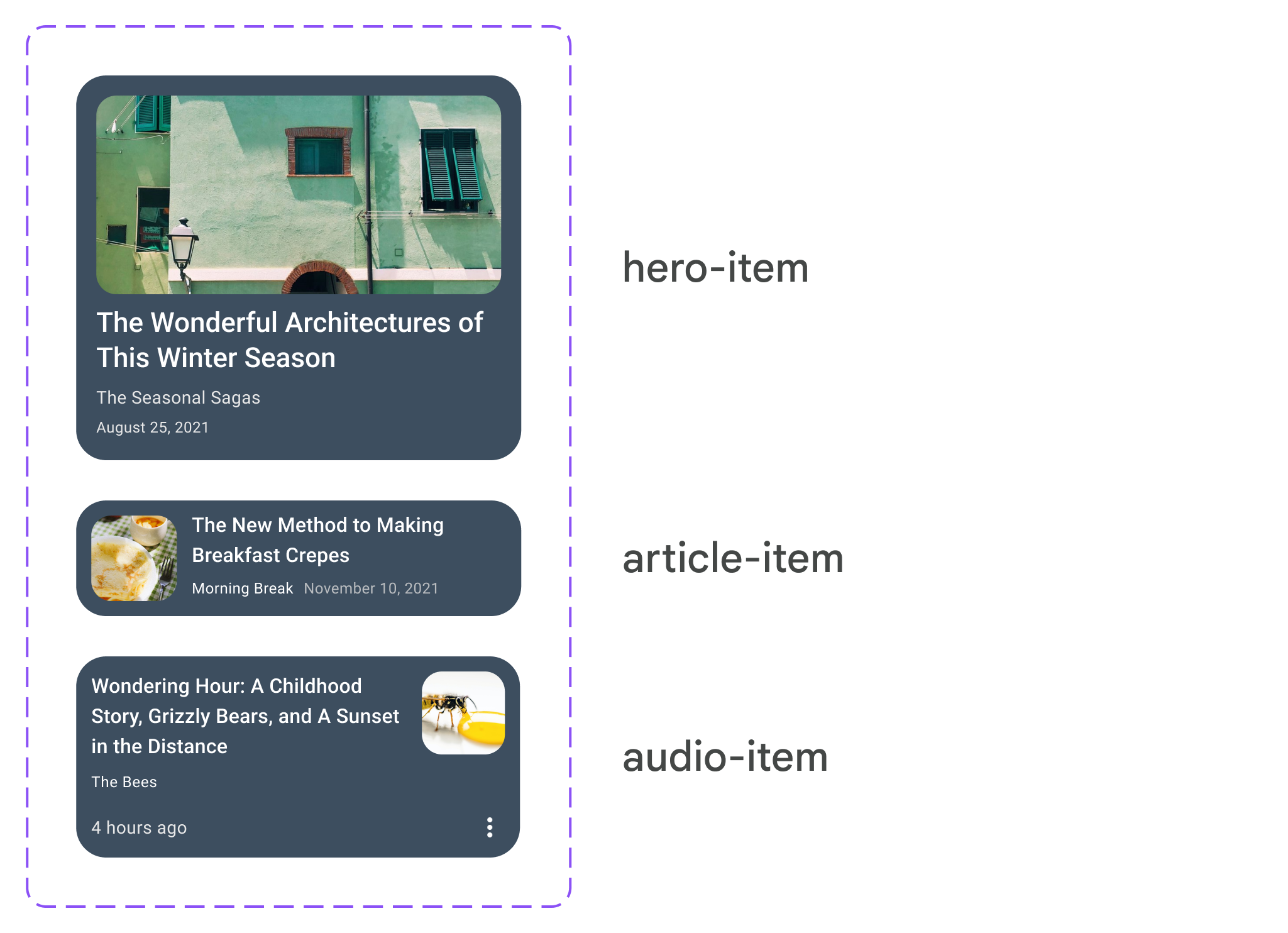
We'll start with a Figma file that contains a News Card component with three variants:
- hero-item is for the most important news article
- article-item is for a typical article
- audio-item is for an article that you listen to, instead of read
Copy Figma example
We’ll be using an existing Figma file as an example for this tutorial. Make sure you’re logged into your Figma account.
- Download HelloNews.fig to your computer.
In Figma, go to the file browser. Along the left-hand side, hover over Drafts. A + icon will appear — click the + icon, then Import. Select the HelloNews.fig file that you just downloaded. This can take anywhere from 10 seconds to a minute.
Open the imported file in Figma.
Create a UI package
The Relay for Figma plugin adds extra information to our component, so we can work with our developers who will use our component in their code.
- Open the Relay for Figma plugin in your file (in menu bar: Plugins > Relay for Figma). Click Get Started.
Select the component and click Create UI Package.
With the UI Package selected, add a description to the summary box. For example: “News card component intended to display news items for a list”.
Save named version
Now that you have created a UI package, prepare your component to share it with the development team.
- Open the Figma Relay plugin, if not already open.
- Click Share with developer.
- From the Share with developer screen, you can enter a new version name and description in the Save version history section.
Click Save.
Example Title: Initial New Card
Example Description: First iteration of the news article items
Download Android Studio project
For the Android Studio part of this tutorial, we will use a pre-configured Android Studio project. This project contains an app that displays news articles in a plain text format.
- Download the sample HelloNews.zip file.
- Double-click the file to unzip it, which will create a folder called HelloNews. Move it to your home folder.
- Go back to Android Studio. Go to File > Open, navigate to your home folder, select HelloNews, and click Open.
- When you open the project, Android Studio will ask you if you trust the project. Click Trust Project.
Import into Android Studio
Let’s import our Figma component into the project.
Back in Figma, copy the URL of the News Card Tutorial Figma file. We’ll use this URL to import our components. In the upper right-hand corner of Figma, click on the Share button. In the dialog box that opens, click Copy Link.
Switch to the HelloNews project in Android Studio. Go to File > New > Import UI Packages… from the Android Studio menu bar.
Paste the URL of your Figma file and click Next.
- Once the file is done fetching (which may take some time), click Finish.
Click
to build your project. This may take a minute or so.
Preview app & component
In Android view, open
app/java/com/example/hellonews/ui/home/HomeScreen.kt
, you’ll see a preview of the app, which displays several news articles in a plain text format, with print stories above audio stories.Open up
app/java (generated)/com/example/hellonews/newscard/NewsCard.kt
. This is the generated Jetpack Compose code for our Figma component. From the preview, we can see that three variants of the NewsCard component have been translated from Figma to code. Let’s take a closer look at the code.The
View
enum allows us to choose which variant to use for this component. The name of the enum and its values were derived from the variants of our Figma component. This is used in theview
parameter in our NewsCard composable.The NewsCard composable was generated from the UI package. It includes a parameter of type
View
, which sets the variant of the news card to instantiate.package com.example.myapplication.newscard import ... // Design to select for NewsCard enum class View { HeroItem, ArticleItem, AudioItem } /** * News card component intended to display news items for a list. * * This composable was generated from the UI Package 'news_card'. * Generated code; do not edit directly */ @Composable fun NewsCard( modifier: Modifier = Modifier, view: View = View.HeroItem ) {...}
Next up
We are not quite ready to use NewsCard yet. The component doesn’t know how to display different news stories, only the same hardcoded one in Figma. So, if we were to add the component now, all we would get is the same news story repeated. We need a way to specify which parts of NewsCard should be filled with dynamic data.
In this section, we'll add content parameters to the NewsCard component.
Recommended for you
- Note: link text is displayed when JavaScript is off
- Content parameters
- Scroll
- Using Compose in Views