Organize your settings
Part of Android Jetpack.
Stay organized with collections
Save and categorize content based on your preferences.
Large and complex settings screens can make it difficult for a user to find a specific setting they want to change. The Preference library offers the following ways to better organize your settings screens.
Preference categories
If you have several related
Preference
objects on a single
screen, you can group them using a
PreferenceCategory
. A
PreferenceCategory
displays a category title and visually separates the
category.
To define a PreferenceCategory
in XML, wrap the Preference
tags with a
PreferenceCategory
, as follows:
<PreferenceScreen xmlns:app="http://schemas.android.com/apk/res-auto"> <PreferenceCategory app:key="notifications_category" app:title="Notifications"> <SwitchPreferenceCompat app:key="notifications" app:title="Enable message notifications"/> </PreferenceCategory> <PreferenceCategory app:key="help_category" app:title="Help"> <Preference app:key="feedback" app:summary="Report technical issues or suggest new features" app:title="Send feedback"/> </PreferenceCategory> </PreferenceScreen>
The result looks like the following:
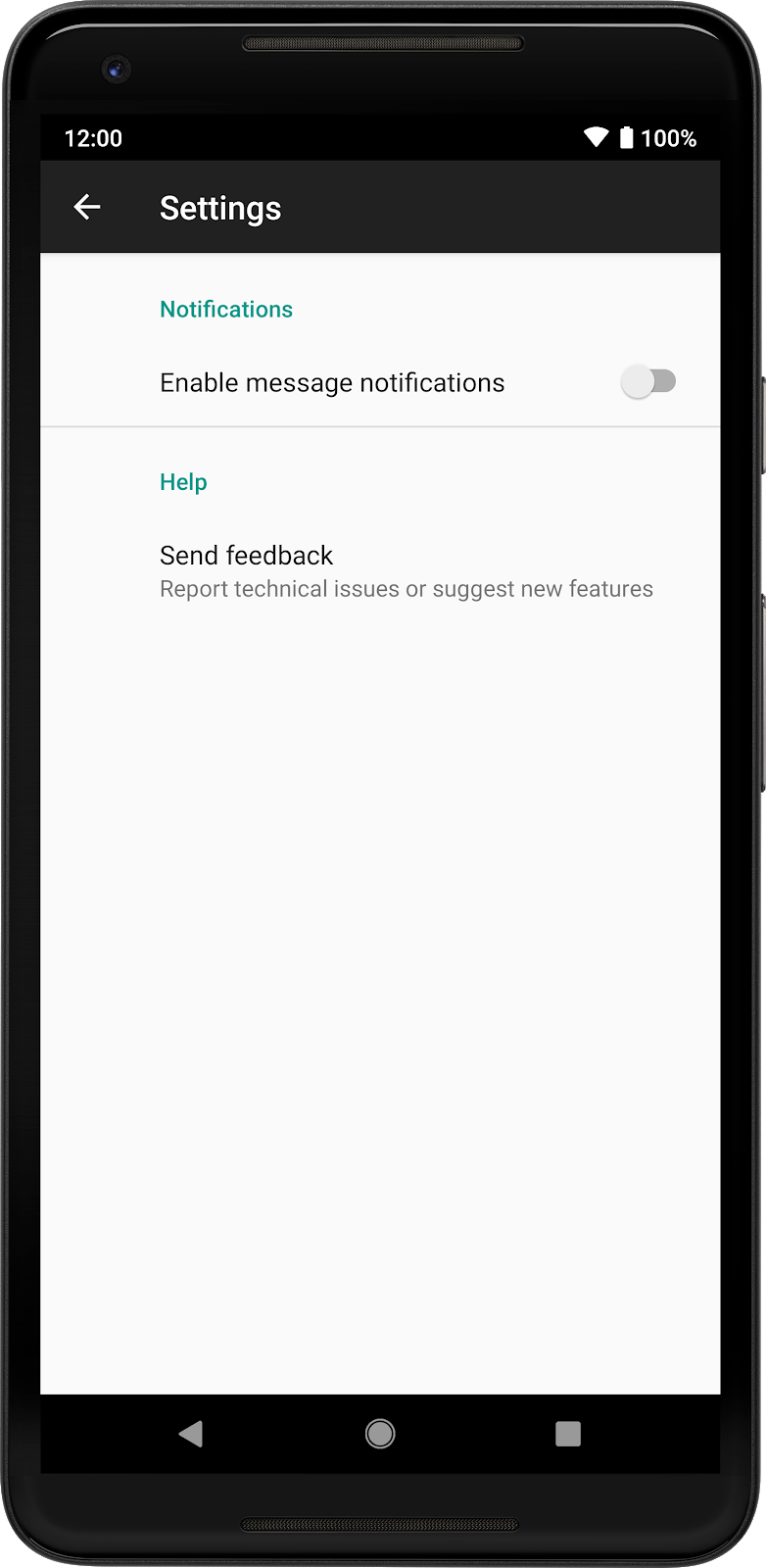
Split your hierarchy into multiple screens
If you have a large number of Preference
objects or distinct categories, you
can display them on separate screens. Each screen is a
PreferenceFragmentCompat
with its own separate hierarchy. Preference
objects
on your initial screen can then link to subscreens that contain related
preferences.
Figure 2 shows a simple hierarchy that contains two categories: Messages and Sync.
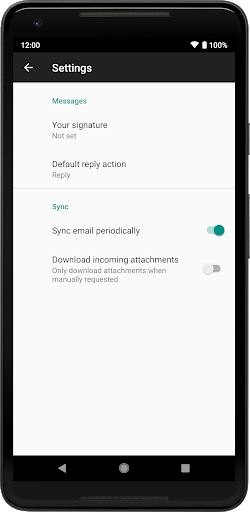
Figure 3 shows the same set of preferences split into multiple screens:
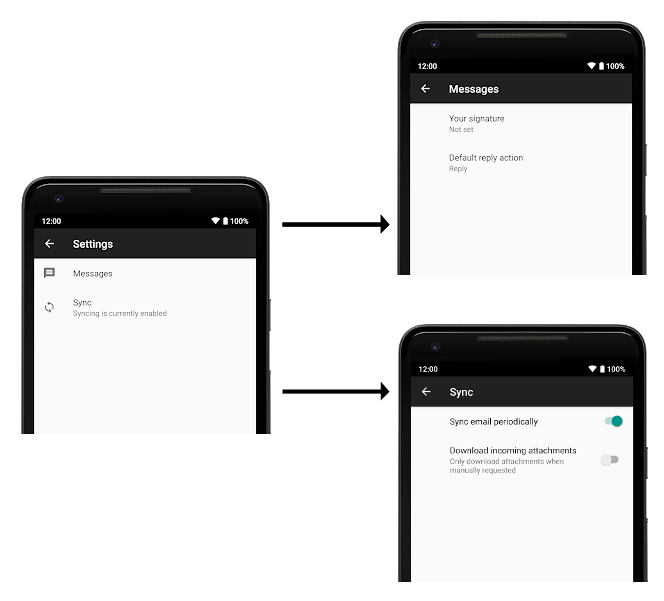
To link screens with a Preference
, you can declare an app:fragment
in XML or
you can use
Preference.setFragment()
.
Launch the full package name of the PreferenceFragmentCompat
when
the Preference
is tapped, as shown in the following example:
<Preference app:fragment="com.example.SyncFragment" .../>
When a user taps a Preference
with an associated Fragment
, the interface
method
PreferenceFragmentCompat.OnPreferenceStartFragmentCallback.onPreferenceStartFragment()
is called. This method is where you handle displaying the new screen and where
the screen is implemented in the surrounding Activity
.
A typical implementation looks similar to the following:
class MyActivity : AppCompatActivity(), PreferenceFragmentCompat.OnPreferenceStartFragmentCallback { ... override fun onPreferenceStartFragment(caller: PreferenceFragmentCompat, pref: Preference): Boolean { // Instantiate the new Fragment. val args = pref.extras val fragment = supportFragmentManager.fragmentFactory.instantiate( classLoader, pref.fragment) fragment.arguments = args fragment.setTargetFragment(caller, 0) // Replace the existing Fragment with the new Fragment. supportFragmentManager.beginTransaction() .replace(R.id.settings_container, fragment) .addToBackStack(null) .commit() return true } }
public class MyActivity extends AppCompatActivity implements PreferenceFragmentCompat.OnPreferenceStartFragmentCallback { ... @Override public boolean onPreferenceStartFragment(PreferenceFragmentCompat caller, Preference pref) { // Instantiate the new Fragment. final Bundle args = pref.getExtras(); final Fragment fragment = getSupportFragmentManager().getFragmentFactory().instantiate( getClassLoader(), pref.getFragment()); fragment.setArguments(args); fragment.setTargetFragment(caller, 0); // Replace the existing Fragment with the new Fragment. getSupportFragmentManager().beginTransaction() .replace(R.id.settings_container, fragment) .addToBackStack(null) .commit(); return true; } }
PreferenceScreens
Declaring nested hierarchies within the same XML resource using a nested
<PreferenceScreen>
is no longer supported. Use nested Fragment
objects
instead.
Use separate Activities
Alternatively, if you need to heavily customize each screen, or if you want full
Activity
transitions between screens, you can use a separate Activity
for
each PreferenceFragmentCompat
. By doing this, you can fully customize each
Activity
and its corresponding settings screen. For most apps, we don't
recommended this; instead, use Fragments
as previously described.
For more information about launching an Activity
from a Preference
, see
Preference actions.