應用程式小工具可進行設定。舉例來說,時鐘小工具可讓使用者設定要顯示哪個時區。
如果您想讓使用者自行設定小工具,請建立小工具設定 Activity
。這個活動會在小工具建立時或之後,由應用程式小工具主機自動啟動,具體取決於您指定的設定選項。
宣告設定活動
在 Android 資訊清單檔案中,將設定活動宣告為一般活動。應用程式小工具主機會使用 ACTION_APPWIDGET_CONFIGURE
動作啟動它,因此活動必須接受此意圖。例如:
<activity android:name=".ExampleAppWidgetConfigurationActivity">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_CONFIGURE"/>
</intent-filter>
</activity>
使用 android:configure
屬性,在 AppWidgetProviderInfo.xml
檔案中宣告活動。請參閱宣告此檔案的詳細資訊。以下是如何宣告設定活動的範例:
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
...
android:configure="com.example.android.ExampleAppWidgetConfigurationActivity"
... >
</appwidget-provider>
活動會使用完整的命名空間宣告,因為啟動器會從套件範圍外部參照該活動。
只要完成這些步驟,就能開始設定活動。接下來,您需要實作實際活動。
實作設定活動
實作活動時,請務必記住以下兩個重點:
- 應用程式小工具主機會呼叫設定活動,而設定活動必須一律傳回結果。結果必須包含由啟動活動的意圖傳遞的應用程式小工具 ID,並儲存在意圖額外項目中,做為
EXTRA_APPWIDGET_ID
。 - 系統不會在啟動設定活動時傳送
ACTION_APPWIDGET_UPDATE
廣播,也就是說,系統不會在建立小工具時呼叫onUpdate()
方法。設定活動負責在第一次建立小工具時,向AppWidgetManager
要求更新。不過,系統會在後續更新時呼叫onUpdate()
,只會略過第一次更新。
請參閱下節的程式碼片段,瞭解如何從設定傳回結果並更新小工具。
從設定活動更新小工具
當小工具使用設定活動時,活動會負責在設定完成後更新小工具。你可以直接透過 AppWidgetManager
要求更新。
以下是正確更新小工具及關閉設定活動的程序摘要:
從啟動活動的意圖取得 App Widget ID:
Kotlin
val appWidgetId = intent?.extras?.getInt( AppWidgetManager.EXTRA_APPWIDGET_ID, AppWidgetManager.INVALID_APPWIDGET_ID ) ?: AppWidgetManager.INVALID_APPWIDGET_ID
Java
Intent intent = getIntent(); Bundle extras = intent.getExtras(); int appWidgetId = AppWidgetManager.INVALID_APPWIDGET_ID; if (extras != null) { appWidgetId = extras.getInt( AppWidgetManager.EXTRA_APPWIDGET_ID, AppWidgetManager.INVALID_APPWIDGET_ID); }
將活動結果設為
RESULT_CANCELED
。這樣一來,如果使用者在活動結束前退出,系統會通知應用程式小工具主機,說明設定已取消,主機不會新增小工具:
Kotlin
val resultValue = Intent().putExtra(AppWidgetManager.EXTRA_APPWIDGET_ID, appWidgetId) setResult(Activity.RESULT_CANCELED, resultValue)
Java
int resultValue = new Intent().putExtra(AppWidgetManager.EXTRA_APPWIDGET_ID, appWidgetId); setResult(Activity.RESULT_CANCELED, resultValue);
根據使用者的偏好設定小工具。
設定完成後,請呼叫
getInstance(Context)
取得AppWidgetManager
的例項:Kotlin
val appWidgetManager = AppWidgetManager.getInstance(context)
Java
AppWidgetManager appWidgetManager = AppWidgetManager.getInstance(context);
請呼叫
updateAppWidget(int,RemoteViews)
,以RemoteViews
版面配置更新小工具:Kotlin
val views = RemoteViews(context.packageName, R.layout.example_appwidget) appWidgetManager.updateAppWidget(appWidgetId, views)
Java
RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.example_appwidget); appWidgetManager.updateAppWidget(appWidgetId, views);
建立返回意圖、使用活動結果設定該意圖,然後完成活動:
Kotlin
val resultValue = Intent().putExtra(AppWidgetManager.EXTRA_APPWIDGET_ID, appWidgetId) setResult(Activity.RESULT_OK, resultValue) finish()
Java
Intent resultValue = new Intent().putExtra(AppWidgetManager.EXTRA_APPWIDGET_ID, appWidgetId); setResult(RESULT_OK, resultValue); finish();
請參閱 GitHub 上的 ListWidgetConfigureActivity.kt
範例類別。
小工具設定選項
根據預設,應用程式小工具主機只會在使用者將小工具新增至主畫面後立即啟動設定活動一次。不過,您可以指定選項,讓使用者重新設定現有小工具,或提供預設的小工具設定來略過初始的小工具設定。
允許使用者重新設定放置的小工具
如要讓使用者重新設定現有的小工具,請在 appwidget-provider
的 widgetFeatures
屬性中指定 reconfigurable
標記。詳情請參閱宣告 AppWidgetProviderInfo.xml
檔案的指南。例如:
<appwidget-provider
android:configure="com.myapp.ExampleAppWidgetConfigurationActivity"
android:widgetFeatures="reconfigurable">
</appwidget-provider>
使用者只要輕觸並按住小工具,然後輕觸「重新設定」按鈕 (圖 1 中標示為 1),即可重新設定小工具。
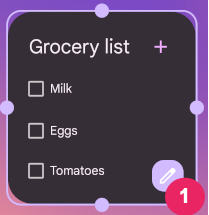
使用小工具的預設設定
您可以讓使用者略過初始設定步驟,提供更順暢的小工具體驗。為此,請在 widgetFeatures
欄位中同時指定 configuration_optional
和 reconfigurable
標記。這樣一來,系統就不會在使用者新增小工具後啟動設定活動。如先前所述,使用者日後仍可重新設定小工具。舉例來說,時鐘小工具可以略過初始設定,並預設顯示裝置時區。
以下範例說明如何將設定活動標示為可重新設定且為選用的活動:
<appwidget-provider
android:configure="com.myapp.ExampleAppWidgetConfigurationActivity"
android:widgetFeatures="reconfigurable|configuration_optional">
</appwidget-provider>