Bazı içerikler, durum çubuğunda veya gezinme çubuğunda herhangi bir gösterge olmadan tam ekranda en iyi şekilde deneyimlenebilir. Videolar, oyunlar, resim galerileri, kitaplar ve sunum slaytları bunlara örnek olarak verilebilir. Bu, yoğun içerik modu olarak adlandırılır. Bu sayfada, kullanıcılarla tam ekran içeriklerle nasıl daha derin bir etkileşim kurabileceğinizi görebilirsiniz.
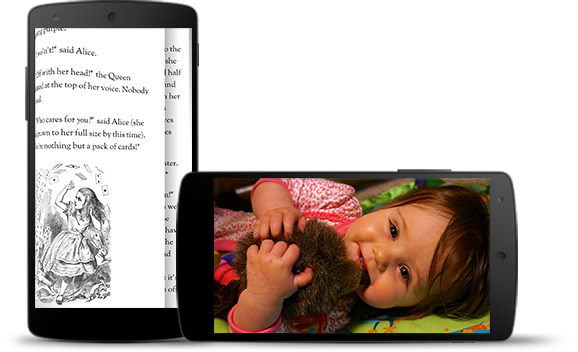
Tam ekran modu, kullanıcıların oyun sırasında yanlışlıkla çıkış yapmalarını önler ve resim, video ve kitaplardan keyif almaları için sürükleyici bir deneyim sunar. Ancak kullanıcıların bildirimleri kontrol etmek, anlık aramalar yapmak veya başka işlemler yapmak için uygulamalar arasında ne sıklıkta geçiş yaptığını göz önünde bulundurun. Tam sayfa modu, kullanıcıların sistem gezinme menüsüne kolayca erişmesini engellediği için bu modu yalnızca kullanıcı deneyimine sağladığı avantaj, ekstra ekran alanı kullanmanın ötesine geçtiğinde kullanın.
Sistem çubuklarını gizlemek için WindowInsetsControllerCompat.hide()
, göstermek için WindowInsetsControllerCompat.show()
simgesini kullanın.
Aşağıdaki snippet'te, sistem çubuklarını gizlemek ve göstermek için bir düğmeyi yapılandırma örneği gösterilmektedir.
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { ... val windowInsetsController = WindowCompat.getInsetsController(window, window.decorView) // Configure the behavior of the hidden system bars. windowInsetsController.systemBarsBehavior = WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener(window.decorView) { view, windowInsets -> // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()) } } else { binding.toggleFullscreenButton.setOnClickListener { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()) } } ViewCompat.onApplyWindowInsets(view, windowInsets) } }
Java
@Override protected void onCreate(Bundle savedInstanceState) { ... WindowInsetsControllerCompat windowInsetsController = WindowCompat.getInsetsController(getWindow(), getWindow().getDecorView()); // Configure the behavior of the hidden system bars. windowInsetsController.setSystemBarsBehavior( WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE ); // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener( getWindow().getDecorView(), (view, windowInsets) -> { // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener(v -> { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()); }); } else { binding.toggleFullscreenButton.setOnClickListener(v -> { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()); }); } return ViewCompat.onApplyWindowInsets(view, windowInsets); }); }
İsteğe bağlı olarak, bir kullanıcı etkileşimde bulunduğunda gizlenmesini ve davranışını belirlemek üzere sistem çubuklarının türünü belirtebilirsiniz.
Gizlenecek sistem çubuklarını belirtme
Gizlenecek sistem çubuklarının türünü belirtmek için WindowInsetsControllerCompat.hide()
parametresine aşağıdakilerden birini iletin.
Her iki sistem çubuğunu da gizlemek için
WindowInsetsCompat.Type.systemBars()
simgesini kullanın.Yalnızca durum çubuğunu gizlemek için
WindowInsetsCompat.Type.statusBars()
simgesini kullanın.Yalnızca gezinme çubuğunu gizlemek için
WindowInsetsCompat.Type.navigationBars()
simgesini kullanın.
Gizli sistem çubuklarının davranışını belirtme
Kullanıcı gizli sistem çubuklarıyla etkileşimde bulunduğunda bu çubukların nasıl davranacağını belirtmek için WindowInsetsControllerCompat.setSystemBarsBehavior()
öğesini kullanın.
İlgili ekranda tüm kullanıcı etkileşimlerinde gizli sistem çubuklarını göstermek için
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_TOUCH
simgesini kullanın.Sistem hareketlerinde gizli sistem çubuklarını göstermek için
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_SWIPE
simgesini kullanın (ör. çubuğun gizlendiği ekran kenarından kaydırın).Sistem hareketleriyle gizli sistem çubuklarını geçici olarak göstermek için
WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE
simgesini kullanın (ör. çubuğun gizlendiği ekran kenarından kaydırın). Bu geçici sistem çubukları uygulamanızın içeriğinin üzerini kaplar, belirli bir düzeyde şeffaflık içerebilir ve kısa bir zaman aşımının ardından otomatik olarak gizlenir.