Es posible que tu app necesite llamar la atención del usuario con urgencia en ciertas situaciones, como cuando suena una alarma o entra una llamada. En las apps orientadas a dispositivos que ejecutan Android 9 (nivel de API 28) o versiones anteriores, puedes controlar esto iniciando una actividad mientras la app está en segundo plano. En este documento, se muestra cómo lograr este comportamiento en dispositivos que ejecutan Android 10 (nivel de API 29) a Android 13 (nivel de API 33).
Agrega el permiso POST_NOTIFICATIONS
A partir de Android 13, agrega la siguiente línea a tu archivo AndroidManifest.xml
:
<manifest ...> <uses-permission android:name="android.permission.POST_NOTIFICATIONS"/> <application ...> ... </application> </manifest>
Una vez que tengas esto, podrás crear un canal de notificaciones.
Cómo crear un canal de notificaciones
Crea un canal de notificaciones para mostrarlas correctamente y permitir que el usuario las administre en la configuración de la app. Para obtener más información sobre los canales de notificaciones, consulta Cómo crear y administrar canales de notificaciones.
Crea tus canales de notificaciones en el método onCreate
de la clase Application
:
Kotlin
class DACapp : Application() { override fun onCreate() { super.onCreate() val channel = NotificationChannel( CHANNEL_ID, "High priority notifications", NotificationManager.IMPORTANCE_HIGH ) val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager notificationManager.createNotificationChannel(channel) } }
Cuando el usuario ejecuta tu app por primera vez, ve algo como la Figura 1 en la pantalla del sistema Información de la app:
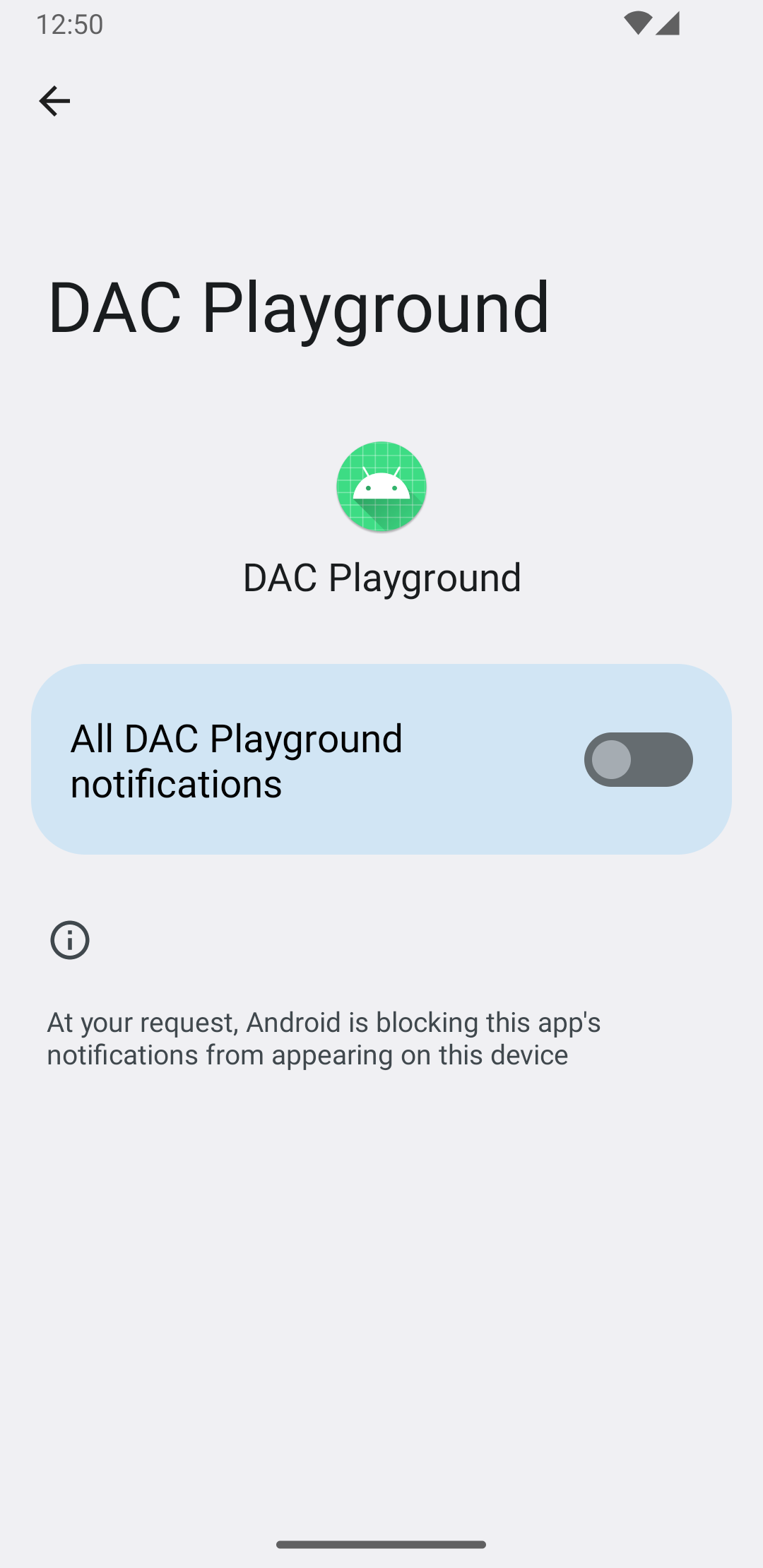
Cómo administrar los permisos de notificaciones
A partir de Android 13, solicita permisos de notificación antes de mostrarles notificaciones a los usuarios.
La implementación mínima se ve de la siguiente manera:
Kotlin
val permissionLauncher = rememberLauncherForActivityResult( contract = ActivityResultContracts.RequestPermission(), onResult = { hasNotificationPermission = it } ) ... Button( onClick = { if (!hasNotificationPermission) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU) { permissionLauncher.launch(Manifest.permission.POST_NOTIFICATIONS) } } }, ) { Text(text = "Request permission") }
Si tu dispositivo ejecuta Android 13, presionar el botón Request
permission
activa el diálogo que se muestra en la Figura 2:
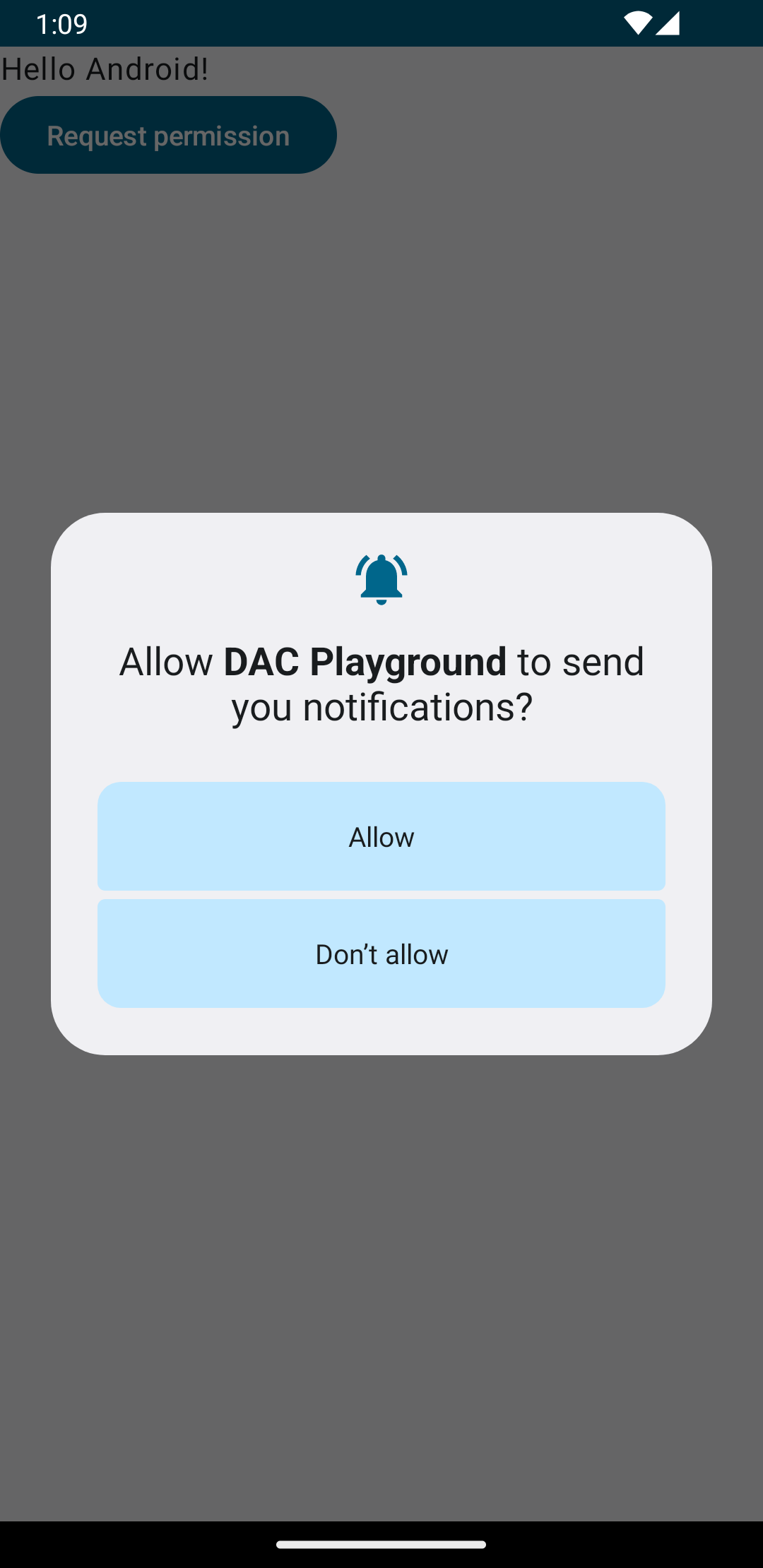
Si el usuario acepta la solicitud de permiso, la sección Información de la app de la app se verá como en la figura 3:
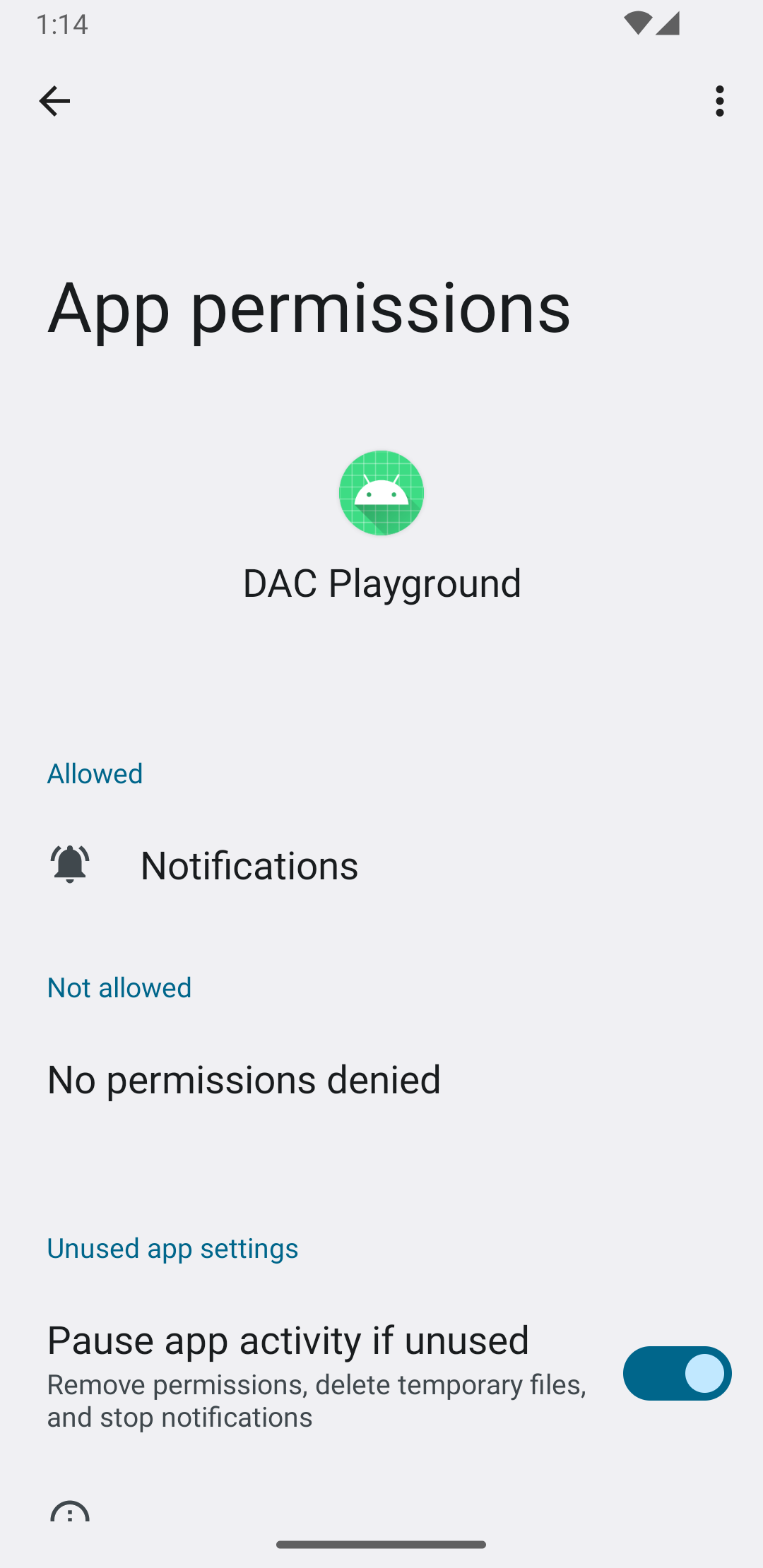
Cómo crear una notificación de prioridad alta
Cuando crees la notificación, incluye un título y un mensaje descriptivos.
El siguiente ejemplo contiene una notificación:
Kotlin
private fun showNotification() { val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager val notificationBuilder = NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_auto_awesome_24) .setContentTitle("HIGH PRIORITY") .setContentText("Check this dog puppy video NOW!") .setPriority(NotificationCompat.PRIORITY_HIGH) .setCategory(NotificationCompat.CATEGORY_RECOMMENDATION) notificationManager.notify(666, notificationBuilder.build()) }
Cómo mostrar la notificación al usuario
Llamar a la función showNotification()
activa la notificación de la siguiente manera:
Kotlin
Button(onClick = { showNotification() }) { Text(text = "Show notification") }
La notificación de este ejemplo se ve como la figura 4:
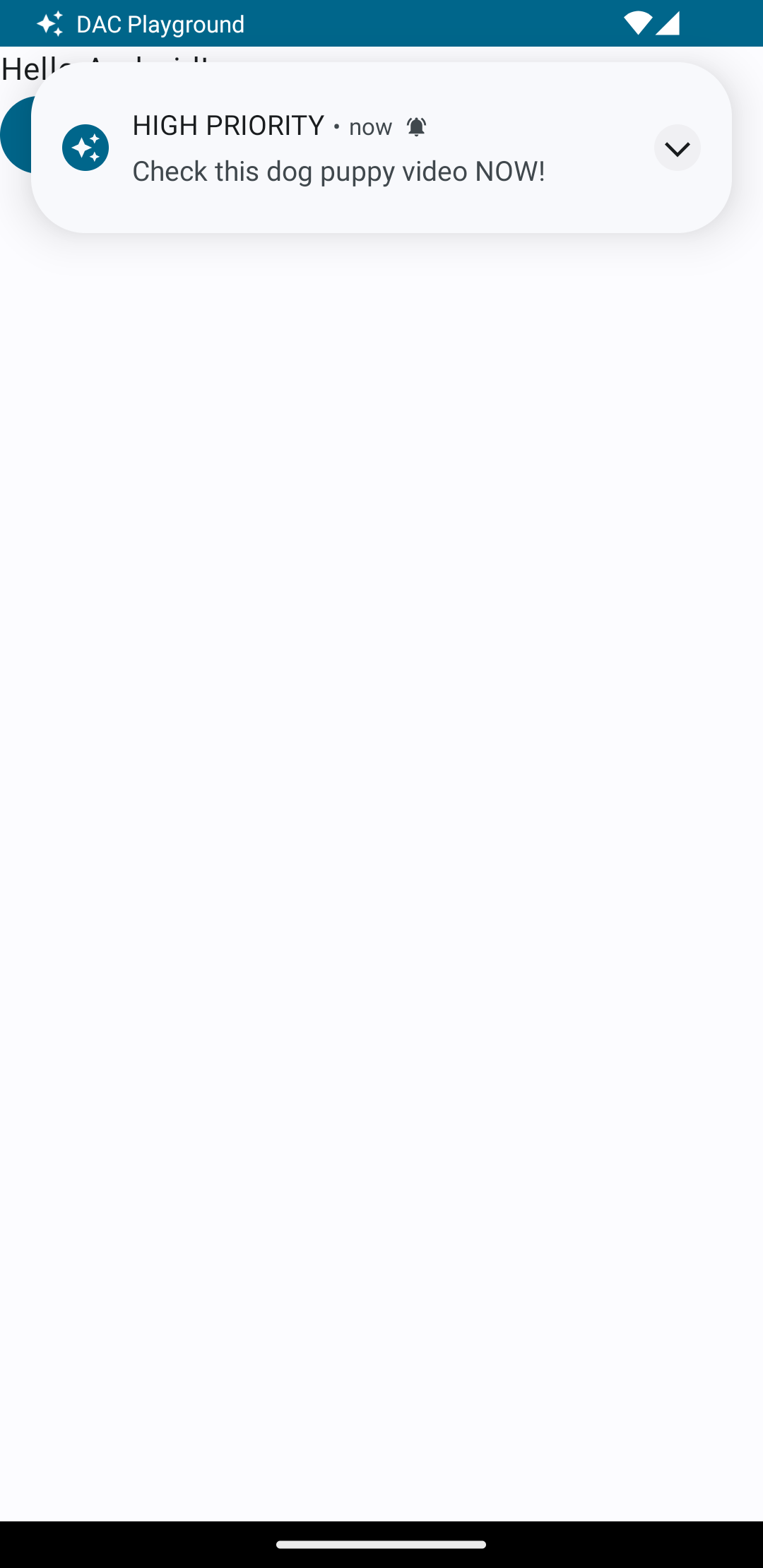
Notificación en curso
Cuando se muestre la notificación al usuario, este puede aceptar o descartar la alerta o el recordatorio de tu app. Por ejemplo, puede aceptar o rechazar una llamada entrante.
Si tu notificación es constante, como una llamada entrante, asóciala con un servicio en primer plano. En el siguiente fragmento de código, se muestra cómo mostrar una notificación asociada con un servicio en primer plano:
Kotlin
// Provide a unique integer for the "notificationId" of each notification. startForeground(notificationId, notification)
Java
// Provide a unique integer for the "notificationId" of each notification. startForeground(notificationId, notification);