레이아웃에 거의 사용되지 않는 복잡한 뷰가 필요한 경우가 있습니다. 여부 실행취소, 실행취소 등 중요함이 있으므로 야기할 수 있는 경우에만 뷰를 로드하여 렌더링 속도를 확인할 수 있습니다
앱에 복잡한 뷰가 있는 경우 리소스 로드를 지연할 수 있음
향후 니즈에 맞는
ViewStub
:
복잡하고 거의 사용되지 않는 뷰
ViewStub 정의
ViewStub
는 다음과 같은 크기가 없는 간단한 뷰입니다.
무언가를 그리거나 레이아웃에 참여할 수 없습니다. 따라서 필요한 리소스가
확장하거나 뷰 계층 구조에 남겨두는 것입니다. 각 ViewStub
에 포함되는 사항
android:layout
속성을 사용하여 확장할 레이아웃을 지정합니다.
나중에 사용자 여정에서 로드하려는 레이아웃이 있다고 가정해 보겠습니다. 앱:
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:src="@drawable/logo" android:layout_width="match_parent" android:layout_height="match_parent"/> </FrameLayout>
다음 ViewStub
를 사용하여 로드를 연기할 수 있습니다. 만들기
무언가를 표시하거나 로드하는 경우 참조된 레이아웃을 표시하도록 해야 합니다.
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/root" android:layout_width="match_parent" android:layout_height="match_parent"> <ViewStub android:id="@+id/stub_import" android:inflatedId="@+id/panel_import" android:layout="@layout/heavy_layout_we_want_to_postpone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="bottom" /> </FrameLayout>
ViewStub 레이아웃 로드
이전 섹션의 코드 스니펫은 다음 그림과 같은 것을 생성합니다. 1:
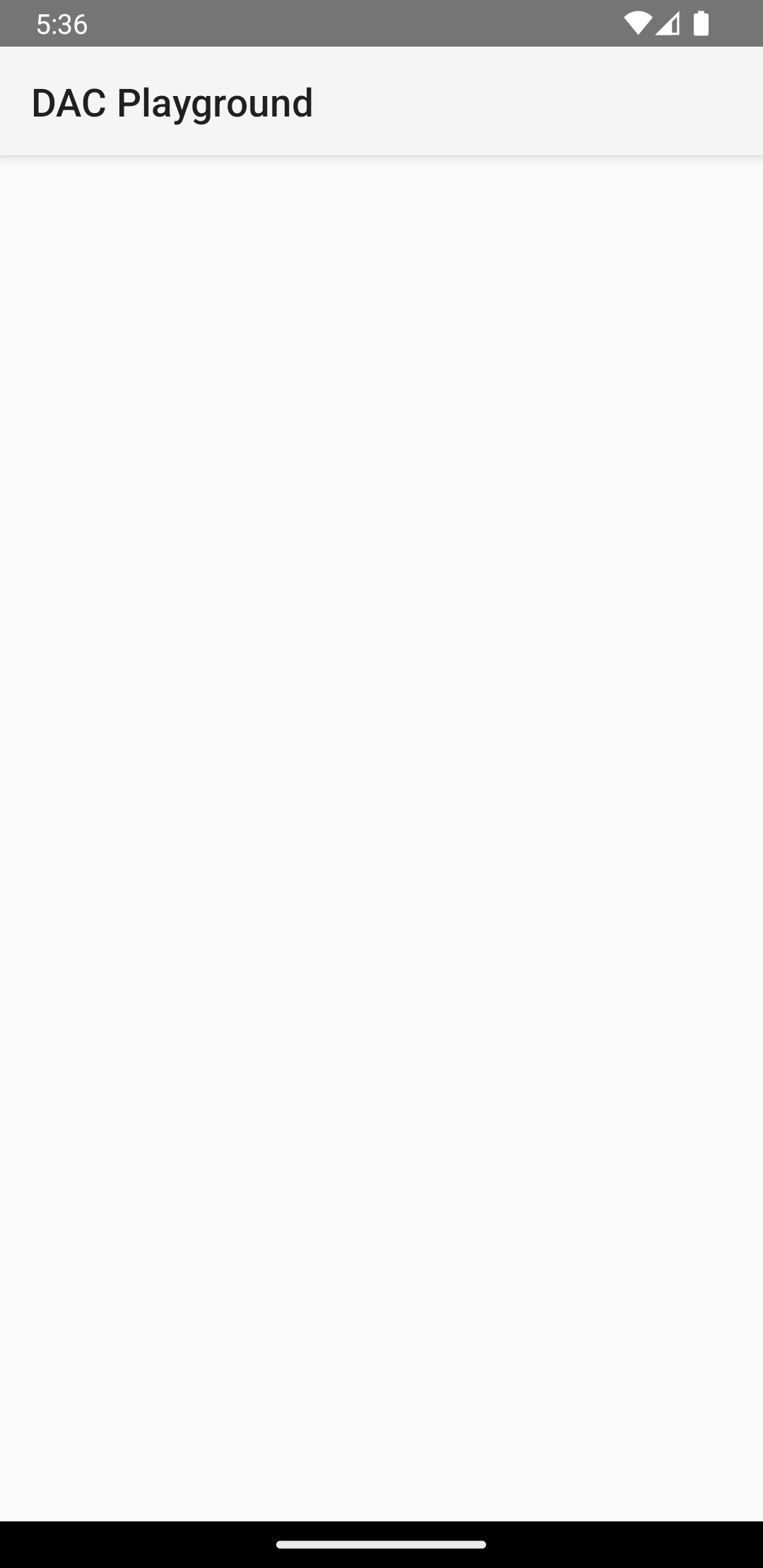
ViewStub
무거운 레이아웃을 숨기는 것입니다ViewStub
에서 지정한 레이아웃을 로드하려면 다음 안내를 따르세요.
또는
setVisibility(View.VISIBLE)
또는 전화
inflate()
입니다.
다음 코드 스니펫은 지연된 로드를 시뮬레이션합니다. 화면이 다음과 같이 로드됩니다.
Activity
및 onCreate()
에서 평소와 같은 경우
heavy_layout_we_want_to_postpone
레이아웃:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_old_xml) Handler(Looper.getMainLooper()) .postDelayed({ findViewById<View>(R.id.stub_import).visibility = View.VISIBLE // Or val importPanel: View = findViewById<ViewStub>(R.id.stub_import).inflate() }, 2000) }
자바
@Override void onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_old_xml); Handler(Looper.getMainLooper()) .postDelayed({ findViewById<View>(R.id.stub_import).visibility = View.VISIBLE // Or val importPanel: View = findViewById<ViewStub>(R.id.stub_import).inflate() }, 2000); }
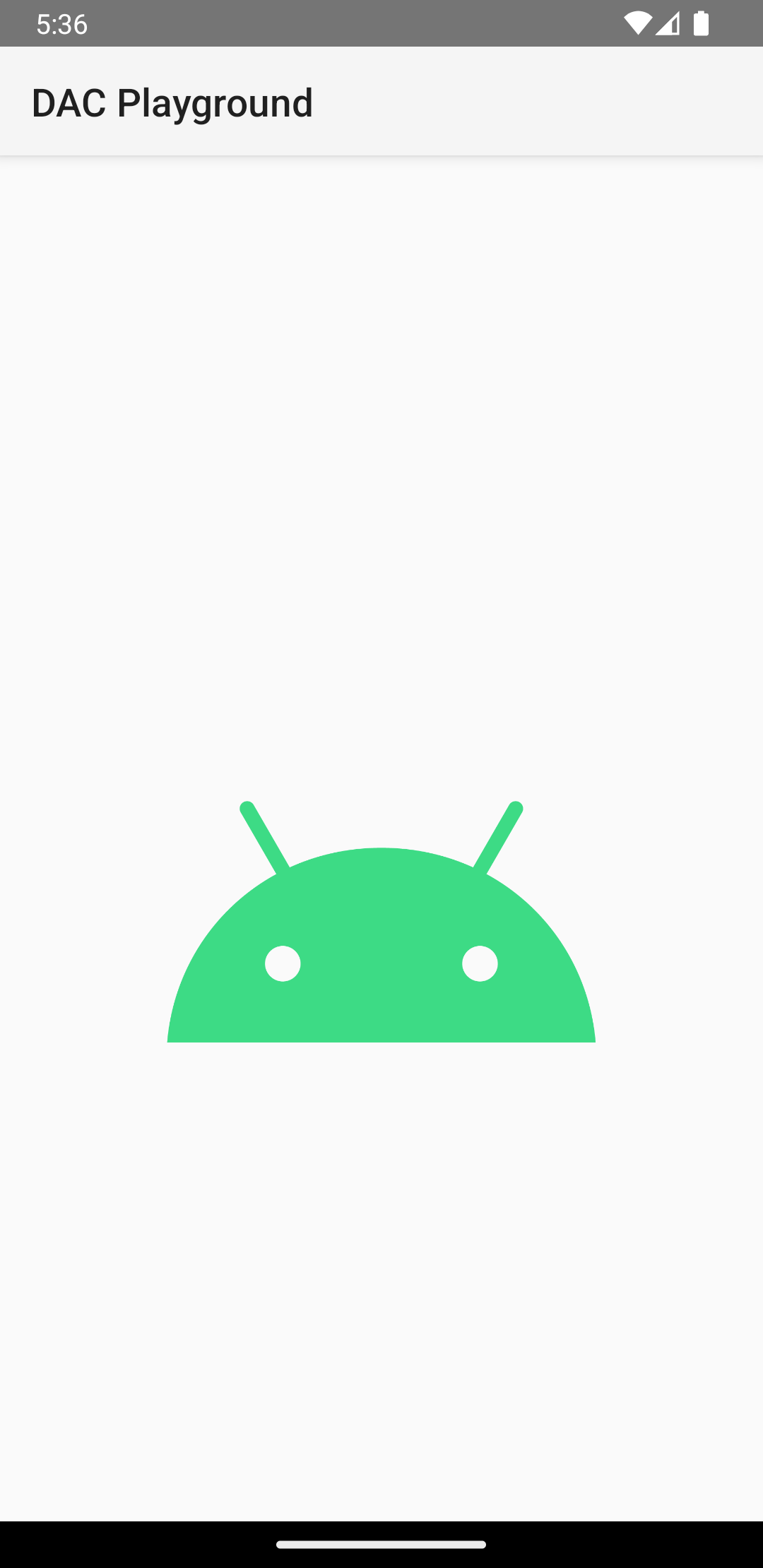
ViewStub
요소는 표시되거나 확장되면 더 이상
뷰 계층 구조입니다. 확장된 레이아웃과
이 레이아웃의 루트 뷰는 android:inflatedId
에서 지정합니다.
ViewStub
의 속성입니다. 지정된 ID android:id
는 ViewStub
ViewStub
확장될 수 있습니다.
이 주제에 관한 자세한 내용은 블로그 게시물을 참고하세요. 최적화 스텁 포함