Ресурсы — это дополнительные файлы и статический контент, который использует ваш код, например растровые изображения, определения макета, строки пользовательского интерфейса, инструкции анимации и т. д.
Всегда экспортируйте ресурсы приложения, такие как изображения и строки, из вашего кода, чтобы вы могли поддерживать их независимо. Кроме того, предоставьте альтернативные ресурсы для конкретных конфигураций устройств, сгруппировав их в специально названных каталогах ресурсов. Во время выполнения Android использует соответствующий ресурс в зависимости от текущей конфигурации. Например, вы можете захотеть предоставить другой макет пользовательского интерфейса в зависимости от размера экрана или разные строки в зависимости от настроек языка.
После того как вы экспортируете ресурсы своего приложения, вы сможете получить к ним доступ, используя идентификаторы ресурсов, сгенерированные в классе R
вашего проекта. В этом документе показано, как группировать ресурсы в вашем проекте Android. Здесь также показано, как предоставить альтернативные ресурсы для конкретных конфигураций устройств, а затем получить к ним доступ из кода приложения или других XML-файлов.
Типы ресурсов группы
Поместите каждый тип ресурса в определенный подкаталог каталога res/
вашего проекта. Например, вот иерархия файлов для простого проекта:
MyProject/ src/ MyActivity.java res/ drawable/ graphic.png layout/ main.xml info.xml mipmap/ icon.png values/ strings.xml
Каталог res/
содержит все ресурсы в своих подкаталогах: ресурс изображения, два ресурса макета, каталог mipmap/
для значков запуска и файл строковых ресурсов. Имена каталогов ресурсов важны и описаны в таблице 1.
Примечание. Дополнительные сведения об использовании папок MIP-карт см. в разделе Размещение значков приложений в каталогах MIP-карт .
Таблица 1. Поддерживаемые каталоги ресурсов внутри каталога res/
проекта.
Каталог | Тип ресурса |
---|---|
animator/ | XML-файлы, определяющие анимацию свойств . |
anim/ | XML-файлы, определяющие анимацию Tween . Анимацию свойств также можно сохранить в этом каталоге, но для анимации свойств предпочтительнее использовать каталог animator/ , чтобы различать эти два типа. |
color/ | XML-файлы, определяющие список состояний цветов. Дополнительные сведения см. в разделе Ресурс списка состояний цвета . |
drawable/ | Файлы растровых изображений (PNG,
Дополнительные сведения см. в разделе Ресурсы, доступные для рисования . |
mipmap/ | Рисуемые файлы для разной плотности значков запуска. Дополнительную информацию об управлении значками запуска с помощью папок mipmap/ см. в разделе Размещение значков приложений в каталогах mipmap . |
layout/ | XML-файлы, определяющие макет пользовательского интерфейса. Дополнительные сведения см. в разделе Ресурс макета . |
menu/ | XML-файлы, определяющие меню приложения, например меню параметров, контекстное меню или подменю. Дополнительную информацию см. в разделе Ресурс меню . |
raw/ | Произвольные файлы для сохранения в необработанном виде. Чтобы открыть эти ресурсы с помощью необработанного Однако, если вам нужен доступ к исходным именам файлов и иерархии файлов, рассмотрите возможность сохранения ресурсов в каталоге |
values/ | XML-файлы, содержащие простые значения, такие как строки, целые числа и цвета. В то время как файлы ресурсов XML в других подкаталогах Поскольку каждый ресурс определен с помощью собственного XML-элемента, вы можете назвать файл как угодно и поместить разные типы ресурсов в один файл. Однако для ясности вы можете поместить уникальные типы ресурсов в разные файлы. Например, вот некоторые соглашения об именах файлов для ресурсов, которые вы можете создать в этом каталоге:
Дополнительные сведения см. в разделах Строковые ресурсы , Ресурс стиля и Дополнительные типы ресурсов . |
xml/ | Произвольные XML-файлы, которые можно прочитать во время выполнения, вызвав Resources.getXML() . Здесь необходимо сохранить различные файлы конфигурации XML, например конфигурацию поиска . |
font/ | Файлы шрифтов с такими расширениями, как TTF, OTF или TTC, или файлы XML, включающие элемент <font-family> . Дополнительные сведения о шрифтах как ресурсах см. в разделе Добавление шрифта как ресурса XML . |
Внимание: никогда не сохраняйте файлы ресурсов непосредственно в каталоге res/
. Это вызывает ошибку компилятора.
Дополнительную информацию об отдельных типах ресурсов см. в обзоре типов ресурсов .
Ресурсы, которые вы сохраняете в подкаталогах, определенных в таблице 1, являются вашими ресурсами по умолчанию. То есть эти ресурсы определяют дизайн и контент вашего приложения по умолчанию. Однако разные типы устройств на базе Android могут требовать разных типов ресурсов.
Например, вы можете предоставить различные ресурсы макета для устройств с экранами большего размера, чем обычно, чтобы использовать дополнительное пространство экрана. Вы также можете предоставить различные строковые ресурсы, которые переводят текст в пользовательском интерфейсе в зависимости от языковых настроек устройства. Чтобы предоставить эти разные ресурсы для разных конфигураций устройств, вам необходимо предоставить альтернативные ресурсы в дополнение к ресурсам по умолчанию.
Предоставьте альтернативные ресурсы
Большинство приложений предоставляют альтернативные ресурсы для поддержки определенных конфигураций устройств. Например, включите альтернативные ресурсы рисования для разных плотностей экрана и альтернативные строковые ресурсы для разных языков. Во время выполнения Android определяет текущую конфигурацию устройства и загружает соответствующие ресурсы для вашего приложения.
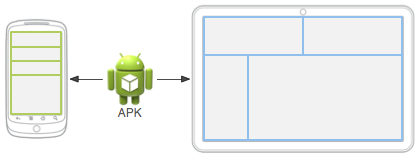
Рис. 1. Два устройства, использующие разные ресурсы макета в зависимости от размера экрана.
Чтобы указать альтернативы конкретной конфигурации для набора ресурсов, выполните следующие действия:
- Создайте новый каталог в
res/
с именем в форме<resources_name> - <qualifier>
.-
<resources_name>
— имя каталога соответствующих ресурсов по умолчанию (определено в таблице 1). -
<qualifier>
— это имя, указывающее индивидуальную конфигурацию, для которой должны использоваться эти ресурсы (определено в таблице 2).
Вы можете добавить более одного
<qualifier>
. Каждую отделяйте черточкой.Внимание: При добавлении нескольких квалификаторов их необходимо размещать в том же порядке, в котором они перечислены в таблице 2. Если квалификаторы расположены неправильно, ресурсы игнорируются.
-
- Сохраните соответствующие альтернативные ресурсы в этом новом каталоге. Файлы ресурсов должны называться точно так же, как файлы ресурсов по умолчанию.
Например, вот некоторые ресурсы по умолчанию и альтернативные ресурсы:
res/ drawable/ icon.png background.png drawable-hdpi/ icon.png background.png
Квалификатор hdpi
указывает, что ресурсы в этом каталоге предназначены для устройств с экраном высокой плотности. Изображения в этих доступных для рисования каталогах имеют размер для определенной плотности экрана, но имена файлов абсолютно одинаковы. Таким образом, идентификатор ресурса, который вы используете для ссылки на изображение icon.png
или background.png
, всегда один и тот же. Android выбирает версию каждого ресурса, которая лучше всего соответствует текущему устройству, сравнивая информацию о конфигурации устройства с квалификаторами в имени каталога ресурсов.
Внимание: При определении альтернативного ресурса убедитесь, что вы также определили ресурс в конфигурации по умолчанию. В противном случае ваше приложение может столкнуться с исключениями во время выполнения, когда устройство меняет конфигурацию. Например, если вы добавите строку только в values-en
, а не values
, ваше приложение может столкнуться с исключением Resource Not Found
, когда пользователь меняет системный язык по умолчанию.
В таблице 2 перечислены допустимые квалификаторы конфигурации в порядке приоритета. К одному имени каталога можно добавить несколько квалификаторов, разделяя каждый квалификатор дефисом. Если вы используете несколько квалификаторов для каталога ресурсов, вы должны добавить их к имени каталога в том порядке, в котором они перечислены в таблице.
Таблица 2. Имена квалификаторов конфигурации.
Конфигурация | Значения квалификаторов | Описание |
---|---|---|
МСС и МНК | Примеры:mcc310
mcc208-mnc00 | Мобильный код страны (MCC), за которым при необходимости следует код мобильной сети (MNC) с SIM-карты в устройстве. Например, Если устройство использует радиосвязь (то есть это телефон GSM), значения MCC и MNC берутся с SIM-карты. Вы также можете использовать только MCC, например, чтобы включить в свое приложение юридические ресурсы для конкретной страны. Если вам нужно указать только язык, используйте вместо этого квалификатор языка, алфавита (необязательно) и региона (необязательно) . Если вы используете квалификаторы MCC и MNC, делайте это осторожно и проверяйте, работает ли он должным образом. Также см. поля конфигурации |
Язык, алфавит (необязательно) и регион (необязательно). | Примеры:en fr en-rUS fr-rFR fr-rCA b+en b+en+US b+es+419 b+zh+Hant b+sr+Latn+RS | Язык определяется двухбуквенным кодом языка ISO 639-1 , за которым может следовать двухбуквенный код региона ISO 3166-1-alpha-2 (перед которым стоит строчная буква Коды не чувствительны к регистру. Префикс В Android 7.0 (уровень API 24) появилась поддержка языковых тегов BCP 47 , которые можно использовать для квалификации ресурсов, зависящих от языка и региона. Языковой тег состоит из последовательности одного или нескольких вложенных тегов, каждый из которых уточняет или сужает диапазон языка, идентифицируемый общим тегом. Дополнительную информацию о языковых тегах см. в разделе Теги для идентификации языков . Чтобы использовать языковой тег BCP 47, объедините Языковой тег может измениться в течение срока службы вашего приложения, если пользователи изменят свой язык в настройках системы. Сведения о том, как это может повлиять на ваше приложение во время выполнения, см. в разделе Обработка изменений конфигурации . Полное руководство по локализации вашего приложения для других языков см. в разделе Локализация вашего приложения . Также см. метод |
Направление макета | ldrtl ldltr | Направление макета вашего приложения. Это может применяться к любому ресурсу, например макетам, объектам рисования или значениям. Например, если вы хотите предоставить специальную раскладку для арабского языка и общую раскладку для любого другого языка с письмом справа налево, например персидского или иврита, вы используете следующие каталоги: Примечание. Чтобы включить функции макета справа налево для вашего приложения, необходимо установить Добавлено на уровне API 17. |
Наименьшая ширина | sw<N>dp Примеры: sw320dp sw600dp sw720dp и т. д. | Самый короткий размер области экрана, доступный приложению. В частности, Например, если ваш макет требует, чтобы наименьший размер области экрана всегда составлял не менее 600 dp, вы можете использовать этот квалификатор для создания ресурсов макета в каталоге Использование наименьшей ширины для определения общего размера экрана полезно, поскольку ширина часто является решающим фактором при разработке макета. Пользовательский интерфейс часто прокручивается по вертикали, но имеет довольно жесткие ограничения на минимальное пространство, необходимое для него по горизонтали. Доступная ширина также является ключевым фактором при определении того, использовать ли макет с одной панелью для мобильных телефонов или макет с несколькими панелями для планшетов. Таким образом, вас, вероятно, больше всего волнует, какая наименьшая возможная ширина будет на каждом устройстве. Наименьшая ширина устройства учитывает оформление экрана и системный интерфейс. Например, если на экране устройства есть постоянные элементы пользовательского интерфейса, которые занимают пространство вдоль оси наименьшей ширины, система объявляет, что наименьшая ширина меньше фактического размера экрана, поскольку это пиксели экрана, недоступные для вашего пользовательского интерфейса. . Некоторые значения, которые вы можете использовать здесь для распространенных размеров экрана:
Когда ваше приложение предоставляет несколько каталогов ресурсов с разными значениями квалификатора Добавлено на уровне API 13. Также см. атрибут Дополнительную информацию о проектировании для разных экранов с использованием этого квалификатора см. в разделе Адаптивный/адаптивный дизайн с представлениями . |
Доступная ширина и высота | w<N>dp h<N>dp Примеры: w720dp w1024dp h720dp h1024dp и т. д. | Указывает минимальную доступную ширину или высоту экрана (в единицах Доступная ширина и высота часто полезны для определения того, следует ли использовать многопанельный макет, поскольку даже на планшетном устройстве часто не требуется тот же многопанельный макет для книжной ориентации, что и для альбомной. Таким образом, вы можете использовать их для указания минимальной ширины и/или высоты, необходимой для макета, вместо того, чтобы использовать одновременно размер экрана и квалификаторы ориентации. Когда ваше приложение предоставляет несколько каталогов ресурсов с разными значениями для этих конфигураций, система использует тот, который ближе всего (не превышает) к текущей ширине экрана устройства. Ближайший к определяется путем добавления разницы между фактической шириной экрана и указанной шириной к разнице между фактической высотой экрана и указанной высотой, при этом неуказанные значения высоты и ширины имеют значение 0. Значения исключают область, занимаемую вставками Window , поэтому, если устройство имеет постоянные элементы пользовательского интерфейса по краям дисплея, значения ширины и высоты будут меньше реальных размеров экрана, даже если приложение отображается от края до края с использованием Некоторые незафиксированные вертикальные украшения экрана (например, строка состояния телефона, которая может быть скрыта в полноэкранном режиме) здесь не учитываются, а также украшения окон, такие как строка заголовка или панель действий, поэтому приложения должны быть готовы к работе с ними. несколько меньшее пространство, чем указано. Примечание. Система выбирает ресурс, соответствующий как по ширине, так и по высоте. Поэтому ресурс, в котором указаны оба параметра, предпочтительнее, чем ресурс, в котором указано только одно или другое. Например, если фактический экран имеет ширину 720 dp и высоту 1 280 dp, а один ресурс квалифицируется как w720dp, а другой — как w700dp-h1200dp, выбирается последний, даже если первый точно соответствует тому, что он указывает. Добавлено на уровне API 13. Также см. поля конфигурации Дополнительную информацию о проектировании для разных экранов с использованием этого квалификатора см. в разделе Адаптивный/адаптивный дизайн с представлениями . |
Размер экрана | small normal large xlarge |
Примечание. Использование квалификатора размера не означает, что ресурсы предназначены только для экранов такого размера. Если вы не предоставляете альтернативные ресурсы с квалификаторами, которые лучше соответствуют текущей конфигурации устройства, система может использовать те ресурсы, которые лучше всего подходят . Внимание: если все ваши ресурсы используют квалификатор размера, который больше текущего экрана, система не использует их, и ваше приложение аварийно завершает работу во время выполнения. Это происходит, например, если все ресурсы макета помечены квалификатором Добавлено в API уровня 4. Также см. поле конфигурации Дополнительную информацию см. в разделе Обзор совместимости экранов . |
Формат экрана | long notlong |
Добавлено в API уровня 4. Это основано исключительно на соотношении сторон экрана ( Также см. поле конфигурации |
Круглый экран | round notround |
Добавлено на уровне API 23. Также см. метод конфигурации |
Широкая цветовая гамма | widecg nowidecg |
Добавлено на уровне API 26. Также см. метод конфигурации |
Широкий динамический диапазон (HDR) | highdr lowdr |
Добавлено на уровне API 26. Также см. метод конфигурации |
Ориентация экрана | port land |
Это может измениться в течение срока службы вашего приложения, если пользователь поворачивает экран. Сведения о том, как это повлияет на ваше приложение во время выполнения, см. в разделе Обработка изменений конфигурации . Также см. поле конфигурации |
Режим пользовательского интерфейса | car desk television appliance watch vrheadset |
Добавлен уровень API 8; телевидение добавлено в API 13; часы добавлены в API 20. Информацию о том, как ваше приложение может реагировать, когда устройство вставляется в док-станцию или вынимается из нее, читайте в статье «Определение и мониторинг состояния стыковки» и введите . Это может измениться в течение срока службы вашего приложения, если пользователь поместит устройство в док-станцию. Вы можете включить или отключить некоторые из этих режимов с помощью |
Ночной режим | night notnight |
Добавлено на уровне API 8. Это может измениться в течение срока службы вашего приложения, если ночной режим оставить в автоматическом режиме (по умолчанию), и в этом случае режим меняется в зависимости от времени суток. Вы можете включить или отключить этот режим с помощью |
Плотность пикселей экрана (точек на дюйм) | ldpi mdpi hdpi xhdpi xxhdpi xxxhdpi nodpi tvdpi anydpi nnn dpi |
Между шестью основными плотностями существует соотношение масштабирования 3:4:6:8:12:16 (без учета плотности tvdpi). Таким образом, растровое изображение 9x9 в ldpi имеет размер 12x12 в mdpi, 18x18 в hdpi, 24x24 в xhdpi и так далее. Примечание. Использование квалификатора плотности не означает, что ресурсы предназначены только для экранов этой плотности. Если вы не предоставляете альтернативные ресурсы с квалификаторами, которые лучше соответствуют текущей конфигурации устройства, система использует те ресурсы, которые лучше всего подходят . Дополнительные сведения о том, как обрабатывать различные плотности экрана и как Android может масштабировать растровые изображения в соответствии с текущей плотностью, см. в разделе Обзор совместимости экранов . |
Тип сенсорного экрана | notouch finger |
Также см. поле конфигурации |
Наличие клавиатуры | keysexposed keyshidden keyssoft |
Если вы предоставляете ресурсы Это может измениться в течение срока службы вашего приложения, если пользователь откроет аппаратную клавиатуру. Сведения о том, как это повлияет на ваше приложение во время выполнения, см. в разделе Обработка изменений конфигурации . Также см. поля конфигурации |
Основной метод ввода текста | nokeys qwerty 12key |
Также см. поле конфигурации |
Наличие навигационной клавиши | navexposed navhidden |
Это может измениться в течение срока службы вашего приложения, если пользователь раскроет клавиши навигации. Сведения о том, как это повлияет на ваше приложение во время выполнения, см. в разделе Обработка изменений конфигурации . Также см. поле конфигурации |
Основной метод бессенсорной навигации | nonav dpad trackball wheel |
Также см. поле конфигурации |
Версия платформы (уровень API) | Примеры:v3 v4 v7 и т. д. | Уровень API, поддерживаемый устройством. Например, |
Примечание. Не все версии Android поддерживают все квалификаторы. Использование нового квалификатора неявно добавляет квалификатор версии платформы, чтобы старые устройства могли его игнорировать. Например, использование квалификатора w600dp
автоматически включает квалификатор v13
, поскольку квалификатор доступной ширины был новым на уровне API 13. Чтобы избежать каких-либо проблем, всегда включайте набор ресурсов по умолчанию (набор ресурсов без квалификаторов ). Дополнительную информацию см. в разделе Обеспечение наилучшей совместимости устройств с ресурсами .
Правила имени квалификатора
Вот несколько правил использования имен квалификаторов конфигурации:
- Для одного набора ресурсов можно указать несколько квалификаторов, разделенных дефисами. Например,
drawable-en-rUS-land
применяется к американско-английским устройствам в альбомной ориентации. - Квалификаторы должны быть расположены в порядке, указанном в таблице 2 .
- Неправильно:
drawable-hdpi-port/
- Правильно:
drawable-port-hdpi/
- Неправильно:
- Альтернативные каталоги ресурсов не могут быть вложенными. Например, вы не можете использовать
res/drawable/drawable-en/
. - Значения не чувствительны к регистру. Компилятор ресурсов преобразует имена каталогов в нижний регистр перед обработкой, чтобы избежать проблем в файловых системах, нечувствительных к регистру. Использование заглавных букв в именах предназначено только для удобства чтения.
- Поддерживается только одно значение для каждого типа квалификатора. Например, если вы хотите использовать одни и те же файлы для рисования для Испании и Франции, у вас не может быть каталога с именем
drawable-es-fr/
. Вместо этого вам нужны два каталога ресурсов, такие какdrawable-es/
иdrawable-fr/
, которые содержат соответствующие файлы. Однако вам не обязательно дублировать файлы в обоих местах. Вместо этого вы можете создать псевдоним для ресурса, как описано в разделе Создание ресурсов-псевдонимов .
После сохранения альтернативных ресурсов в каталогах, названных с помощью этих квалификаторов, Android автоматически применяет ресурсы в вашем приложении на основе текущей конфигурации устройства. Каждый раз, когда запрашивается ресурс, Android проверяет альтернативные каталоги ресурсов, содержащие запрошенный файл ресурса, а затем находит наиболее подходящий ресурс .
Если нет альтернативных ресурсов, соответствующих определенной конфигурации устройства, Android использует соответствующие ресурсы по умолчанию — набор ресурсов для определенного типа ресурсов, который не включает квалификатор конфигурации.
Создание ресурсов псевдонимов
Если у вас есть ресурс, который вы хотели бы использовать для нескольких конфигураций устройств, но не хотите предоставлять его в качестве ресурса по умолчанию, вам не нужно помещать один и тот же ресурс в несколько альтернативных каталогов ресурсов. Вместо этого вы можете создать альтернативный ресурс, который будет выступать в качестве псевдонима для ресурса, сохраненного в каталоге ресурсов по умолчанию.
Примечание. Не все ресурсы предлагают механизм, с помощью которого можно создать псевдоним для другого ресурса. В частности, анимация, меню, raw и другие неуказанные ресурсы в каталоге xml/
не поддерживают эту функцию.
Например, представьте, что у вас есть значок приложения icon.png
и вам нужна уникальная его версия для разных языков. Однако две локали, англо-канадская и франко-канадская, должны использовать одну и ту же версию. Вам не нужно копировать одно и то же изображение в каталог ресурсов как для англо-канадского, так и для франко-канадского языка. Вместо этого вы можете сохранить изображение, которое используется для обоих, используя любое имя, кроме icon.png
, например icon_ca.png
, и поместить его в каталог res/drawable/
по умолчанию. Затем создайте файл icon.xml
в res/drawable-en-rCA/
и res/drawable-fr-rCA/
, который ссылается на ресурс icon_ca.png
, используя элемент <bitmap>
. Это позволяет хранить только одну версию файла PNG и два небольших XML-файла, указывающих на него. Подробности смотрите в примерах в следующих разделах.
Рисуемый
Чтобы создать псевдоним для существующего объекта рисования, используйте элемент <drawable>
:
<?xml version="1.0" encoding="utf-8"?> <resources> <drawable name="icon">@drawable/icon_ca</drawable> </resources>
Если вы сохраните этот файл как icon.xml
в альтернативном каталоге ресурсов, например res/values-en-rCA/
, он скомпилируется в ресурс, на который вы можете ссылаться как R.drawable.icon
, но на самом деле это псевдоним для Ресурс R.drawable.icon_ca
, который сохраняется в res/drawable/
.
Макет
Чтобы создать псевдоним существующего макета, используйте элемент <include>
, заключенный в <merge>
:
<?xml version="1.0" encoding="utf-8"?> <merge> <include layout="@layout/main_ltr"/> </merge>
Если вы сохраните этот файл как main.xml
, он скомпилируется в ресурс, на который вы можете ссылаться как R.layout.main
, но на самом деле это псевдоним ресурса R.layout.main_ltr
.
Строки и другие простые значения
Чтобы создать псевдоним для существующей строки, используйте идентификатор ресурса нужной строки в качестве значения для новой строки:
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="hello">Hello</string> <string name="hi">@string/hello</string> </resources>
Ресурс R.string.hi
теперь является псевдонимом R.string.hello
.
Другие простые значения работают таким же образом, например цвета:
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="red">#f00</color> <color name="highlight">@color/red</color> </resources>
Доступ к ресурсам вашего приложения
После того как вы предоставите ресурс в своем приложении, вы сможете применить его, указав его идентификатор ресурса. Все идентификаторы ресурсов определяются в классе R
вашего проекта, который автоматически генерирует инструмент aapt
.
Когда ваше приложение скомпилировано, aapt
генерирует класс R
, который содержит идентификаторы всех ресурсов в вашем каталоге res/
. Для каждого типа ресурса существует подкласс R
, например R.drawable
для всех доступных для рисования ресурсов. И для каждого ресурса этого типа существует статическое целое число, например R.drawable.icon
. Это целое число представляет собой идентификатор ресурса, который вы можете использовать для получения ресурса.
Хотя в классе R
указываются идентификаторы ресурсов, вам не нужно заглядывать туда, чтобы найти идентификатор ресурса. Идентификатор ресурса всегда состоит из следующего:
- Тип ресурса : каждый ресурс сгруппирован по «типу», например
string
,drawable
иlayout
. Дополнительные сведения о различных типах см. в разделе Обзор типов ресурсов . - Имя ресурса , которое представляет собой либо имя файла без расширения, либо значение атрибута XML
android:name
, если ресурс представляет собой простое значение, например строку.
Доступ к ресурсу можно получить двумя способами:
- В коде: использование статического целого числа из подкласса вашего класса
R
, например:R.string.hello
string
— это тип ресурса, аhello
— имя ресурса. Существует множество API-интерфейсов Android, которые могут получить доступ к вашим ресурсам, если вы предоставите идентификатор ресурса в этом формате. Дополнительные сведения см. в разделе Доступ к ресурсам в коде . - В XML: использование специального синтаксиса XML, соответствующего идентификатору ресурса, определенному в вашем классе
R
, например:@string/hello
string
— это тип ресурса, аhello
— имя ресурса. Вы можете использовать этот синтаксис в ресурсе XML в любом месте, где ожидается значение, которое вы предоставляете в ресурсе. Дополнительные сведения см. в разделе Доступ к ресурсам из XML .
Доступ к ресурсам в коде
Вы можете использовать ресурс в коде, передав идентификатор ресурса в качестве параметра метода. Например, вы можете настроить ImageView
на использование ресурса res/drawable/myimage.png
с помощью setImageResource()
:
Котлин
val imageView = findViewById(R.id.myimageview) as ImageView imageView.setImageResource(R.drawable.myimage)
Ява
ImageView imageView = (ImageView) findViewById(R.id.myimageview); imageView.setImageResource(R.drawable.myimage);
Вы также можете получить отдельные ресурсы, используя методы в Resources
, экземпляр которых можно получить с помощью getResources()
.
Синтаксис
Вот синтаксис для ссылки на ресурс в коде:
[<package_name>.]R.<resource_type>.<resource_name>
-
<package_name>
— имя пакета, в котором находится ресурс (не требуется при ссылке на ресурсы из собственного пакета). -
<resource_type>
— это подклассR
для типа ресурса. -
<resource_name>
— это либо имя файла ресурса без расширения, либо значение атрибутаandroid:name
в элементе XML для простых значений.
Дополнительные сведения о каждом типе ресурсов и о том, как ссылаться на них, см. в разделе Обзор типов ресурсов .
Варианты использования
Существует множество методов, которые принимают параметр идентификатора ресурса, и вы можете получать ресурсы с помощью методов в Resources
. Вы можете получить экземпляр Resources
, используя Context.getResources()
.
Вот несколько примеров доступа к ресурсам в коде:
Котлин
// Load a background for the current screen from a drawable resource. window.setBackgroundDrawableResource(R.drawable.my_background_image) // Set the Activity title by getting a string from the Resources object, because // this method requires a CharSequence rather than a resource ID. window.setTitle(resources.getText(R.string.main_title)) // Load a custom layout for the current screen. setContentView(R.layout.main_screen) // Set a slide in animation by getting an Animation from the Resources object. flipper.setInAnimation(AnimationUtils.loadAnimation(this, R.anim.hyperspace_in)) // Set the text on a TextView object using a resource ID. val msgTextView = findViewById(R.id.msg) as TextView msgTextView.setText(R.string.hello_message)
Ява
// Load a background for the current screen from a drawable resource. getWindow().setBackgroundDrawableResource(R.drawable.my_background_image) ; // Set the Activity title by getting a string from the Resources object, because // this method requires a CharSequence rather than a resource ID. getWindow().setTitle(getResources().getText(R.string.main_title)); // Load a custom layout for the current screen. setContentView(R.layout.main_screen); // Set a slide in animation by getting an Animation from the Resources object. flipper.setInAnimation(AnimationUtils.loadAnimation(this, R.anim.hyperspace_in)); // Set the text on a TextView object using a resource ID. TextView msgTextView = (TextView) findViewById(R.id.msg); msgTextView.setText(R.string.hello_message);
Внимание: не изменяйте файл R.java
вручную. Он генерируется инструментом aapt
при компиляции вашего проекта. Любые изменения будут переопределены при следующей компиляции.
Доступ к ресурсам из XML
Вы можете определить значения для некоторых атрибутов и элементов XML, используя ссылку на существующий ресурс. Вы часто делаете это при создании файлов макета, чтобы предоставить строки и изображения для ваших виджетов.
Например, если вы добавляете Button
в свой макет, используйте строковый ресурс для текста кнопки:
<Button android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/submit" />
Синтаксис
Вот синтаксис для ссылки на ресурс в ресурсе XML:
@[<package_name>:]<resource_type>/<resource_name>
-
<package_name>
— имя пакета, в котором находится ресурс (не требуется при ссылке на ресурсы из того же пакета). -
<resource_type>
— это подклассR
для типа ресурса. -
<resource_name>
— это либо имя файла ресурса без расширения, либо значение атрибутаandroid:name
в элементе XML для простых значений.
Дополнительные сведения о каждом типе ресурсов и о том, как ссылаться на них, см. в разделе Обзор типов ресурсов .
Варианты использования
В некоторых случаях вам необходимо использовать ресурс для значения в XML, например, чтобы применить к виджету рисуемое изображение, но вы также можете использовать ресурс в XML в любом месте, где принимается простое значение. Например, если у вас есть следующий файл ресурсов, который включает ресурс цвета и строковый ресурс :
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="opaque_red">#f00</color> <string name="hello">Hello!</string> </resources>
Вы можете использовать эти ресурсы в следующем файле макета, чтобы установить цвет текста и текстовую строку:
<?xml version="1.0" encoding="utf-8"?> <EditText xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:textColor="@color/opaque_red" android:text="@string/hello" />
В этом случае вам не нужно указывать имя пакета в ссылке на ресурс, поскольку ресурсы взяты из вашего собственного пакета. Чтобы сослаться на системный ресурс, вам необходимо указать имя пакета, как показано в следующем примере:
<?xml version="1.0" encoding="utf-8"?> <EditText xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:textColor="@android:color/secondary_text_dark" android:text="@string/hello" />
Примечание. Всегда используйте строковые ресурсы, чтобы ваше приложение можно было локализовать для других языков. Сведения о создании альтернативных ресурсов (например, локализованных строк) см. в разделе Предоставление альтернативных ресурсов . Полное руководство по локализации вашего приложения для других языков см. в разделе Локализация вашего приложения .
Вы даже можете использовать ресурсы XML для создания псевдонимов. Например, вы можете создать рисуемый ресурс, который является псевдонимом другого рисуемого ресурса:
<?xml version="1.0" encoding="utf-8"?> <bitmap xmlns:android="http://schemas.android.com/apk/res/android" android:src="@drawable/other_drawable" />
Это звучит избыточно, но может быть очень полезно при использовании альтернативного ресурса. Дополнительную информацию см. в разделе о создании ресурсов псевдонимов .
Атрибуты ссылочного стиля
Ресурс атрибута стиля позволяет ссылаться на значение атрибута в текущей применяемой теме. Ссылка на атрибут стиля позволяет настроить внешний вид элементов пользовательского интерфейса, стилизуя их в соответствии со стандартными вариантами, предоставляемыми текущей темой, вместо предоставления жестко закодированного значения. Ссылка на атрибут стиля по существу говорит: «Используйте стиль, определенный этим атрибутом, в текущей теме».
Для ссылки на атрибут стиля синтаксис имени почти идентичен обычному формату ресурса, но вместо символа «at» ( @
) используется вопросительный знак ( ?
). Часть типа ресурса является необязательной. Итак, ссылочный синтаксис следующий:
?[<package_name>:][<resource_type>/]<resource_name>
Например, вот как вы можете ссылаться на атрибут, чтобы установить цвет текста, соответствующий вторичному цвету текста системной темы:
<EditText id="text" android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="?android:textColorSecondary" android:text="@string/hello_world" />
Здесь атрибут android:textColor
указывает имя атрибута стиля в текущей теме. Android теперь использует значение, примененное к атрибуту стиля android:textColorSecondary
в качестве значения для android:textColor
в этом виджете. Поскольку инструмент системных ресурсов знает, что в этом контексте ожидается ресурс атрибута, вам не нужно явно указывать тип ?android:attr/textColorSecondary
. Вы можете исключить тип attr
.
Доступ к оригинальным файлам
Хотя это и редкость, вам может потребоваться доступ к исходным файлам и каталогам. Если вы это сделаете, то сохранение файлов в res/
вам не поможет, потому что единственный способ прочитать ресурс из res/
— это использовать идентификатор ресурса. Вместо этого вы можете сохранить свои ресурсы в каталоге assets/
.
Файлам, сохраненным в каталоге assets/
не присваивается идентификатор ресурса, поэтому вы не можете ссылаться на них через класс R
или из ресурсов XML. Вместо этого вы можете запрашивать файлы в каталоге assets/
, как в обычной файловой системе, и читать необработанные данные с помощью AssetManager
.
Однако если все, что вам нужно, — это возможность читать необработанные данные (например, видео или аудиофайл), сохраните файл в каталоге res/raw/
и прочитайте поток байтов с помощью openRawResource()
.
Доступ к ресурсам платформы
Android содержит ряд стандартных ресурсов, таких как стили, темы и макеты. Чтобы получить доступ к этим ресурсам, укажите в ссылке на ресурс имя пакета android
. Например, Android предоставляет ресурс макета, который можно использовать для элементов списка в ListAdapter
:
Котлин
listAdapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, myarray)
Ява
setListAdapter(new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, myarray));
В этом примере simple_list_item_1
— это ресурс макета, определенный платформой для элементов в ListView
. Вы можете использовать это вместо создания собственного макета для элементов списка.
Обеспечить лучшую совместимость устройств с ресурсами
Чтобы ваше приложение поддерживало несколько конфигураций устройств, очень важно всегда предоставлять ресурсы по умолчанию для каждого типа ресурсов, которые использует ваше приложение.
Например, если ваше приложение поддерживает несколько языков, всегда добавляйте каталог values/
(в котором сохраняются ваши строки) без квалификатора языка и региона . Если вместо этого вы поместите все свои строковые файлы в каталоги, которые имеют квалификатор языка и региона, ваше приложение выйдет из строя при запуске на устройстве, на котором установлен язык, который ваши строки не поддерживают.
Пока вы предоставляете values/
ресурсы по умолчанию, ваше приложение работает правильно, даже если пользователь не понимает язык, который оно представляет. Это лучше, чем разбиться.
Аналогично, если вы предоставляете разные ресурсы макета в зависимости от ориентации экрана, выберите одну ориентацию по умолчанию. Например, вместо предоставления ресурсов макета в layout-land/
для альбомной ориентации и layout-port/
для портретной ориентации оставьте один из них по умолчанию, например layout/
для альбомной ориентации и layout-port/
для портретной ориентации.
Предоставление ресурсов по умолчанию важно не только потому, что ваше приложение может работать в конфигурации, которую вы не ожидали, но и потому, что в новые версии Android иногда добавляются квалификаторы конфигурации, которые не поддерживаются старыми версиями. Если вы используете новый квалификатор ресурса, но сохраняете совместимость кода со старыми версиями Android, то при запуске вашего приложения в более старой версии Android происходит сбой, если вы не предоставляете ресурсы по умолчанию, поскольку оно не может использовать ресурсы с именем новый квалификатор.
Например, если для вашего minSdkVersion
установлено значение 4, и вы квалифицируете все свои доступные для рисования ресурсы с использованием ночного режима ( night
или notnight
, которые были добавлены на уровне API 8), то устройство API уровня 4 не сможет получить доступ к вашим доступным для рисования ресурсам и падает. В этом случае вы, вероятно, захотите, чтобы notnight
был вашим ресурсом по умолчанию, поэтому исключите этот квалификатор и поместите доступные для рисования ресурсы либо в drawable/
либо drawable-night/
.
Короче говоря, чтобы обеспечить наилучшую совместимость устройств, всегда предоставляйте ресурсы по умолчанию для ресурсов, необходимых вашему приложению для правильной работы. Затем создайте альтернативные ресурсы для конкретных конфигураций устройств, используя квалификаторы конфигурации.
Из этого правила есть одно исключение: если minSdkVersion
вашего приложения имеет значение 4 или выше, вам не нужны доступные для рисования ресурсы по умолчанию, когда вы предоставляете альтернативные доступные для рисования ресурсы с квалификатором плотности экрана . Даже без доступных для рисования ресурсов по умолчанию Android может найти наилучшее соответствие среди альтернативных плотностей экрана и при необходимости масштабировать растровые изображения. Однако для наилучшего взаимодействия со всеми типами устройств предоставьте альтернативные варианты рисования для всех трех типов плотности.
Как Android находит наиболее подходящий ресурс
Когда вы запрашиваете ресурс, для которого вы предоставляете альтернативы, Android выбирает, какой альтернативный ресурс использовать во время выполнения, в зависимости от текущей конфигурации устройства. Чтобы продемонстрировать, как Android выбирает альтернативный ресурс, предположим, что следующие каталоги с возможностью рисования содержат разные версии одних и тех же изображений:
drawable/ drawable-en/ drawable-fr-rCA/ drawable-en-port/ drawable-en-notouch-12key/ drawable-port-ldpi/ drawable-port-notouch-12key/
Предположим, что следующая конфигурация устройства:
Локаль = en-GB
Ориентация экрана = port
Плотность пикселей экрана = hdpi
Тип сенсорного экрана = notouch
Основной метод ввода текста = 12key
Сравнивая конфигурацию устройства с доступными альтернативными ресурсами, Android выбирает drawables из drawable-en-port
.
Система принимает решение, какие ресурсы использовать, используя следующую логику:
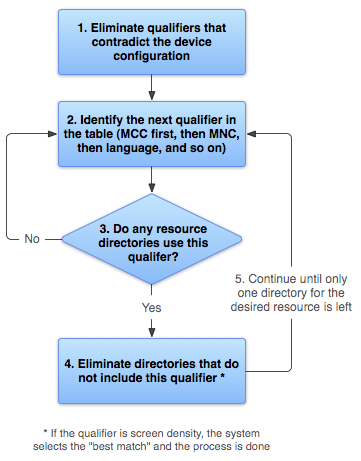
Рисунок 2. Блок-схема того, как Android находит наиболее подходящий ресурс.
- Удалите файлы ресурсов, которые противоречат конфигурации устройства.
Каталог
drawable-fr-rCA/
удален, поскольку он противоречит локалиen-GB
.drawable/ drawable-en/
drawable-fr-rCA/drawable-en-port/ drawable-en-notouch-12key/ drawable-port-ldpi/ drawable-port-notouch-12key/Исключение: плотность пикселей экрана — единственный параметр, который не исключен из-за противоречия. Несмотря на то, что плотность экрана устройства равна hdpi,
drawable-port-ldpi/
не исключается, поскольку на этом этапе каждая плотность экрана считается совпадающей. Дополнительную информацию см. в разделе Обзор совместимости экранов . - Найдите следующий по приоритету квалификатор в списке ( таблица 2 ). (Начните с MCC.)
- Включает ли какой-либо каталог ресурсов этот квалификатор?
- Если нет, вернитесь ко второму шагу и посмотрите следующий квалификатор. В этом примере ответ «нет», пока не будет достигнут квалификатор языка.
- Если да, перейдите к четвертому шагу.
- Удалите каталоги ресурсов, которые не включают этот квалификатор. В этом примере система затем удаляет все каталоги, которые не содержат квалификатор языка:
drawable/drawable-en/ drawable-en-port/ drawable-en-notouch-12key/drawable-port-ldpi/drawable-port-notouch-12key/Исключение: если рассматриваемым квалификатором является плотность пикселей экрана, Android выбирает вариант, который наиболее точно соответствует плотности экрана устройства. В общем, Android предпочитает уменьшать исходное изображение большего размера, а не увеличивать исходное изображение меньшего размера. Дополнительную информацию см. в разделе Обзор совместимости экранов .
- Повторяйте шаги второй, третий и четвертый, пока не останется только один каталог. В этом примере ориентация экрана — это следующий квалификатор, для которого есть совпадения. Таким образом, ресурсы, не указывающие ориентацию экрана, исключаются:
drawable-en/drawable-en-port/drawable-en-notouch-12key/Оставшийся каталог —
drawable-en-port
.
Хотя эта процедура выполняется для каждого запрошенного ресурса, система оптимизирует некоторые ее аспекты. Одна из таких оптимизаций заключается в том, что как только конфигурация устройства станет известна, она может исключить альтернативные ресурсы, которые никогда не могут совпадать. Например, если языком конфигурации является английский, то любой каталог ресурсов, для которого квалификатор языка установлен на значение, отличное от английского, никогда не включается в пул проверяемых ресурсов (хотя каталог ресурсов без квалификатора языка все равно включается).
При выборе ресурсов на основе квалификаторов размера экрана система использует ресурсы, предназначенные для экрана меньшего, чем текущий экран, если нет ресурсов, которые лучше соответствуют. Например, экран большого размера при необходимости использует ресурсы экрана нормального размера.
Однако если единственные доступные ресурсы больше текущего экрана, система не использует их, и ваше приложение аварийно завершает работу, если никакие другие ресурсы не соответствуют конфигурации устройства. Это происходит, например, если все ресурсы макета помечены квалификатором xlarge
, но устройство представляет собой экран обычного размера.
Примечание. Приоритет квалификатора (в таблице 2 ) более важен, чем количество квалификаторов, которые точно соответствуют устройству. В предыдущем примере на четвертом шаге последний вариант в списке включает три квалификатора, которые точно соответствуют устройству (ориентация, тип сенсорного экрана и метод ввода), тогда как drawable-en
имеет только один соответствующий параметр (язык). Однако язык имеет более высокий приоритет, чем эти другие квалификаторы, поэтому drawable-port-notouch-12key
исключается.