Bei ziehbasierten Animationen wird eine Reibungskraft verwendet, die proportional zu einer die Geschwindigkeit des Objekts. Sie können damit eine Eigenschaft eines Objekts animieren und , um die Animation allmählich zu beenden. Sie hat einen anfänglichen Schwung, der hauptsächlich von der Geschwindigkeit der Geste abhängt, und verlangsamt sich allmählich. Die endet, wenn die Geschwindigkeit der Animation niedrig genug ist. dass keine Änderung auf dem Gerätebildschirm sichtbar ist.
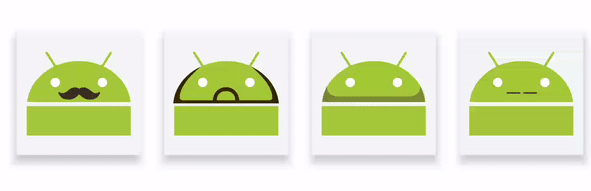
Weitere Informationen zu verwandten Themen finden Sie in den folgenden Leitfäden:
AndroidX-Bibliothek hinzufügen
Wenn Sie die physikbasierten Animationen verwenden möchten, müssen Sie Ihrem Projekt die AndroidX-Bibliothek hinzufügen wie folgt:
- Öffnen Sie die
build.gradle
-Datei für Ihr App-Modul. - Füge dem Abschnitt
dependencies
die AndroidX-Bibliothek hinzu.Cool
dependencies { implementation 'androidx.dynamicanimation:dynamicanimation:1.0.0' }
Kotlin
dependencies { implementation("androidx.dynamicanimation:dynamicanimation:1.0.0") }
Fling-Animation erstellen
Mit der Klasse FlingAnimation
können Sie eine Wischanimation für ein Objekt erstellen. Um eine Fling-Animation zu erstellen, erstellen Sie einen
Instanz der FlingAnimation
-Klasse und
ein Objekt und die zugehörige Eigenschaft des Objekts angeben, das animiert werden soll.
Kotlin
val fling = FlingAnimation(view, DynamicAnimation.SCROLL_X)
Java
FlingAnimation fling = new FlingAnimation(view, DynamicAnimation.SCROLL_X);
Geschwindigkeit einstellen
Die Startgeschwindigkeit definiert die Geschwindigkeit, mit der sich eine Animationseigenschaft zu Beginn der Animation ändert. Die Standardstartgeschwindigkeit ist auf null Pixel pro Sekunde festgelegt. Daher müssen Sie eine Startgeschwindigkeit damit die Animation nicht sofort endet.
Sie können einen festen Wert als Anfangsgeschwindigkeit verwenden oder sie anhand der Geschwindigkeit einer Touch-Geste festlegen. Wenn Sie einen festen Wert angeben, definieren Sie den Wert in dp pro Sekunde und konvertieren ihn in Pixel. pro Sekunde. Wenn der Wert in dp pro Sekunde definiert wird, kann die Geschwindigkeit unabhängig von der Dichte und den Formfaktoren eines Geräts. Weitere Informationen zum Umrechnen der Startgeschwindigkeit in Pixel pro Sekunde finden Sie im Abschnitt dp/s in Pixel/s umrechnen im Artikel Federanimation.
Rufen Sie die Methode setStartVelocity()
auf, um die Geschwindigkeit in Pixeln pro Sekunde festzulegen. Die Methode gibt das Fling-Objekt zurück, für das die Geschwindigkeit festgelegt ist.
Hinweis: Verwenden Sie die Klassen GestureDetector.OnGestureListener
und VelocityTracker
, um die Geschwindigkeit von Touch-Gesten abzurufen und zu berechnen.
Wertebereich für Animation festlegen
Sie können den Mindest- und den Höchstwert für die Animation festlegen, wenn Sie den Property-Wert auf einen bestimmten Bereich beschränken möchten. Diese Bereichssteuerung ist besonders nützlich, wenn Sie Eigenschaften animieren, deren zum Beispiel Alpha (von 0 bis 1).
Hinweis: Wenn der Wert einer Wischanimation den Minimal- oder Maximalwert erreicht, endet die Animation.
Rufen Sie zum Festlegen der Mindest- und Höchstwerte die setMinValue()
auf.
bzw. setMaxValue()
.
Beide Methoden geben das Animationsobjekt zurück, für das Sie den Wert festgelegt haben.
Reibung festlegen
Mit der Methode setFriction()
können Sie
und reibungslose Abläufe bieten. Sie definiert, wie schnell die Geschwindigkeit in einer Animation abnimmt.
Hinweis: Wenn Sie die Reibung nicht zu Beginn der Animation festlegen, wird der Standardwert „1“ verwendet.
Die Methode gibt das Objekt zurück, dessen Animation den von Ihnen angegebenen Reibungswert verwendet.
Beispielcode
Das folgende Beispiel zeigt einen horizontalen Wisch. Die Geschwindigkeit, die von der
lautet der Geschwindigkeits-Tracker velocityX
und die Scroll-Grenzen sind
auf 0 festgelegt und
maxScroll. Die Hürde ist auf 1,1 festgelegt.
Kotlin
FlingAnimation(view, DynamicAnimation.SCROLL_X).apply { setStartVelocity(-velocityX) setMinValue(0f) setMaxValue(maxScroll) friction = 1.1f start() }
Java
FlingAnimation fling = new FlingAnimation(view, DynamicAnimation.SCROLL_X); fling.setStartVelocity(-velocityX) .setMinValue(0) .setMaxValue(maxScroll) .setFriction(1.1f) .start();
Mindestsichtbare Änderung festlegen
Wenn Sie eine benutzerdefinierte Property animieren, die nicht in Pixeln definiert ist, sollten Sie die minimale Änderung des Animationswerts festlegen, die für Nutzer sichtbar ist. Sie bestimmt einen angemessenen Grenzwert für das Ende der Animation.
Diese Methode muss nicht aufgerufen werden, wenn DynamicAnimation.ViewProperty
animiert wird, da die minimale sichtbare Änderung aus der Property abgeleitet wird. Beispiel:
- Der Standardwert für die Mindeständerung, die sichtbar ist, beträgt 1 Pixel für Ansichtseigenschaften wie
TRANSLATION_X
,TRANSLATION_Y
,TRANSLATION_Z
,SCROLL_X
undSCROLL_Y
. - Bei Animationen mit Drehung, z. B.
ROTATION
,ROTATION_X
undROTATION_Y
, beträgt die minimale sichtbare ÄnderungMIN_VISIBLE_CHANGE_ROTATION_DEGREES
, also 1/10 Pixel. - Bei Animationen mit Deckkraft ist die minimale Sichtbarkeitsänderung
MIN_VISIBLE_CHANGE_ALPHA
bzw. 1/256.
Wenn Sie die minimale sichtbare Änderung für eine Animation festlegen möchten, rufen Sie die Methode setMinimumVisibleChange()
auf und übergeben Sie entweder eine der Konstanten für die minimale sichtbare Änderung oder einen Wert, den Sie für eine benutzerdefinierte Property berechnen müssen. Weitere Informationen zur Berechnung dieses Werts finden Sie im Abschnitt Minimalen sichtbaren Änderungswert berechnen.
Kotlin
anim.minimumVisibleChange = DynamicAnimation.MIN_VISIBLE_CHANGE_SCALE
Java
anim.setMinimumVisibleChange(DynamicAnimation.MIN_VISIBLE_CHANGE_SCALE);
Hinweis: Sie müssen nur dann einen Wert übergeben, wenn Sie ein benutzerdefinierte Eigenschaft, die nicht in Pixeln definiert ist.
Mindestwert für sichtbare Änderung berechnen
Verwenden Sie die folgende Formel, um den minimalen sichtbaren Änderungswert für eine benutzerdefinierte Property zu berechnen:
Minimal sichtbare Änderung = Bereich des benutzerdefinierten Attributwerts ÷ Bereich der Animation in Pixeln
Beispielsweise geht die Eigenschaft, die Sie animieren möchten, von 0 bis 100. Das entspricht einer Veränderung um 200 Pixel. Gemäß der Formel beträgt die minimale sichtbare Änderung 100 ÷ 200 = 0,5 Pixel.