基本通知通常包括标题、一行文本,以及用户可以执行的响应操作。如需提供更多信息,您可以应用本文档介绍的多个通知模板之一来创建大型展开式通知。
首先,使用创建通知中介绍的所有基本内容创建通知。然后,使用一个样式对象调用 setStyle()
,并提供与每个模板相对应的信息,如以下示例所示。
添加大图片
如需在通知中添加图片,请将 NotificationCompat.BigPictureStyle
的一个实例传递给 setStyle()
。
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setStyle(NotificationCompat.BigPictureStyle() .bigPicture(myBitmap)) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(myBitmap)) .build();
如需使该图片仅在通知收起时显示为缩略图(如下图所示),请调用 setLargeIcon()
并向其传递图片。然后,调用 BigPictureStyle.bigLargeIcon()
并向其传递 null
,以便大图标在通知展开时消失:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setLargeIcon(myBitmap) .setStyle(NotificationCompat.BigPictureStyle() .bigPicture(myBitmap) .bigLargeIcon(null)) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_post) .setContentTitle(imageTitle) .setContentText(imageDescription) .setLargeIcon(myBitmap) .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(myBitmap) .bigLargeIcon(null)) .build();
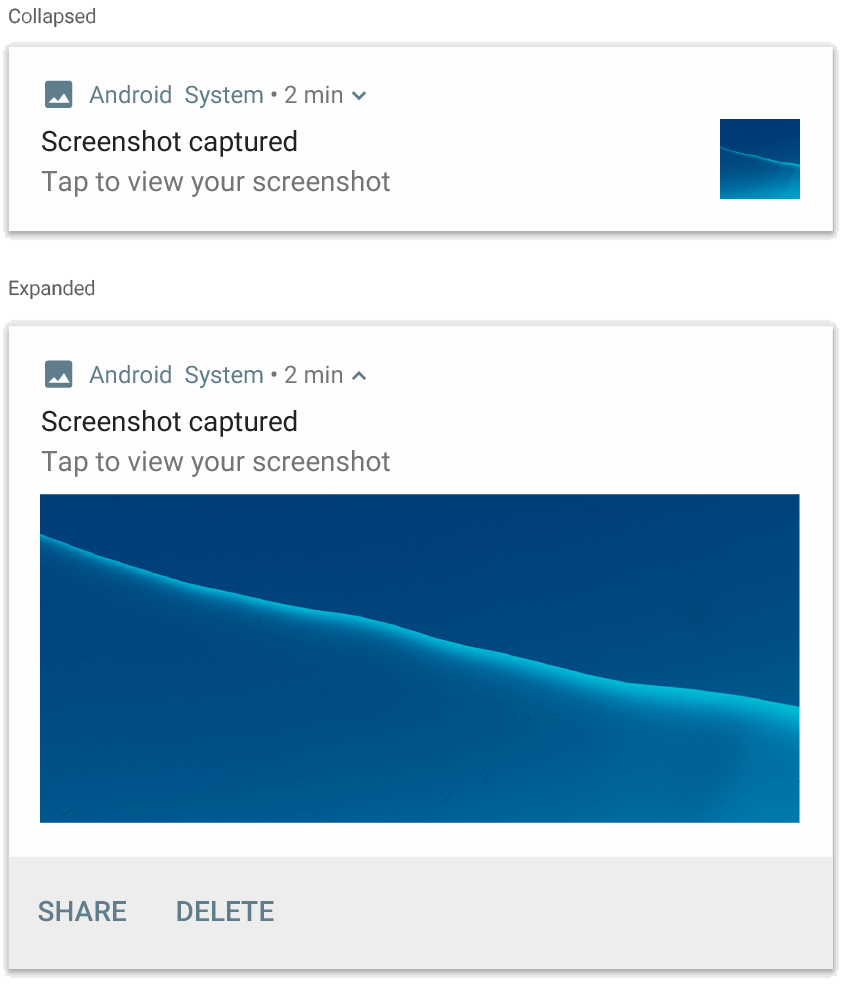
NotificationCompat.BigPictureStyle
的通知。
添加一大段文本
应用 NotificationCompat.BigTextStyle
,以在通知的展开内容区域显示文本:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_mail) .setContentTitle(emailObject.getSenderName()) .setContentText(emailObject.getSubject()) .setLargeIcon(emailObject.getSenderAvatar()) .setStyle(NotificationCompat.BigTextStyle() .bigText(emailObject.getSubjectAndSnippet())) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_mail) .setContentTitle(emailObject.getSenderName()) .setContentText(emailObject.getSubject()) .setLargeIcon(emailObject.getSenderAvatar()) .setStyle(new NotificationCompat.BigTextStyle() .bigText(emailObject.getSubjectAndSnippet())) .build();
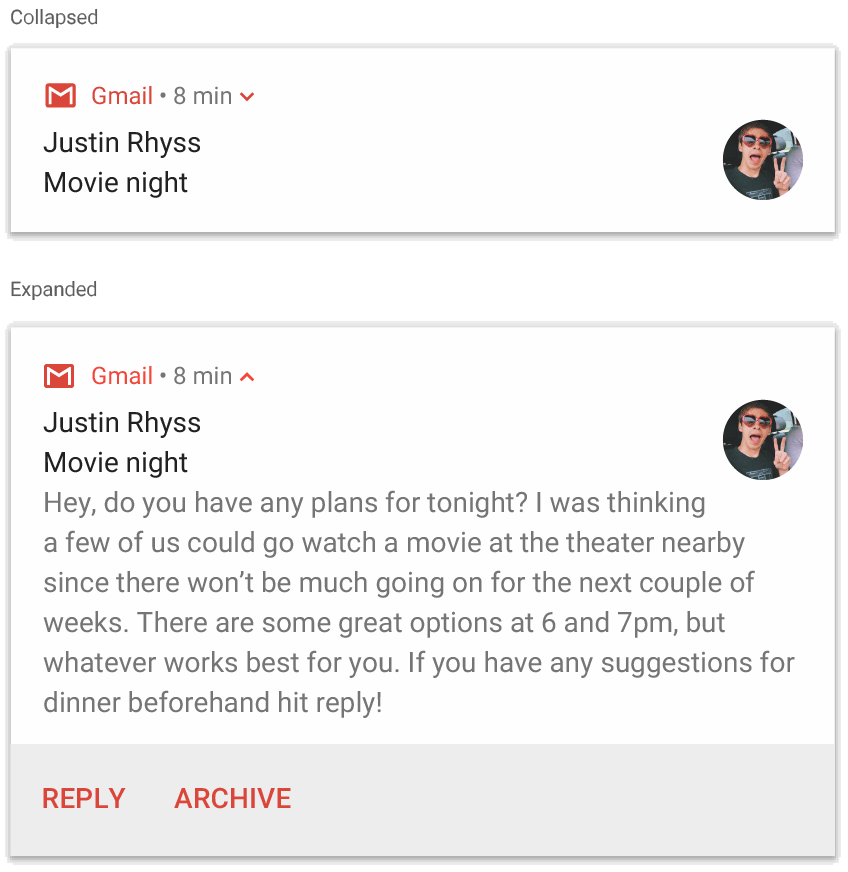
NotificationCompat.BigTextStyle
的通知。
创建收件箱样式的通知
如果您想添加多个简短摘要行(例如收到的电子邮件中的摘要),请对通知应用 NotificationCompat.InboxStyle
。这样,您就可以添加多条内容文本,并且每条文本均截断为一行,而不是显示为 NotificationCompat.BigTextStyle
提供的一个连续文本行。
如需添加新行,最多可调用 addLine()
6 次,如以下示例所示。如果添加的行超过 6 行,则系统只会显示前 6 行。
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_email_24) .setContentTitle("5 New mails from Frank") .setContentText("Check them out") .setLargeIcon(BitmapFactory.decodeResource(resources, R.drawable.logo)) .setStyle( NotificationCompat.InboxStyle() .addLine("Re: Planning") .addLine("Delivery on its way") .addLine("Follow-up") ) .build()
Java
Notification notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.baseline_email_24) .setContentTitle("5 New mails from Frank") .setContentText("Check them out") .setLargeIcon(BitmapFactory.decodeResource(resources, R.drawable.logo)) .setStyle( NotificationCompat.InboxStyle() .addLine("Re: Planning") .addLine("Delivery on its way") .addLine("Follow-up") ) .build();
结果如下图所示:
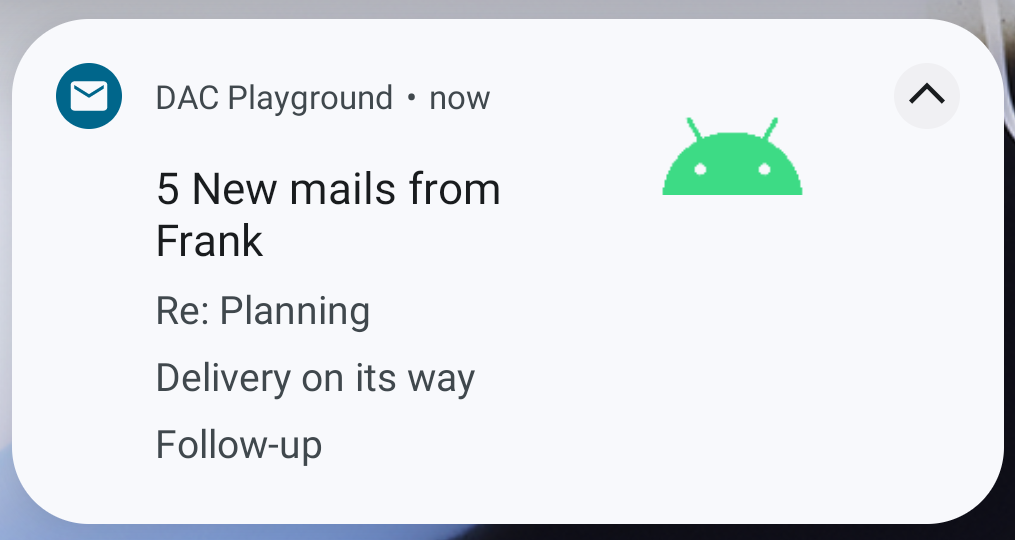
在通知中显示对话
应用 NotificationCompat.MessagingStyle
可显示任意人数之间依序发送的消息。这是即时通讯应用的理想之选,因为它通过单独处理发送人姓名和消息文本为每条消息提供一致的布局,而且每条消息可以在多行中显示。
如需添加新消息,请调用 addMessage()
,并传入消息文本、接收时间和发送人姓名。您也可以将这些信息作为 NotificationCompat.MessagingStyle.Message
对象传递,如以下示例所示:
Kotlin
val message1 = NotificationCompat.MessagingStyle.Message( messages[0].getText(), messages[0].getTime(), messages[0].getSender()) val message2 = NotificationCompat.MessagingStyle.Message( messages[1].getText(), messages[1].getTime(), messages[1].getSender()) val notification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_message) .setStyle( NotificationCompat.MessagingStyle(resources.getString(R.string.reply_name)) .addMessage(message1) .addMessage(message2)) .build()
Java
NotificationCompat.MessagingStyle.Message message1 = new NotificationCompat.MessagingStyle.Message(messages[0].getText(), messages[0].getTime(), messages[0].getSender()); NotificationCompat.MessagingStyle.Message message2 = new NotificationCompat.MessagingStyle.Message(messages[1].getText(), messages[1].getTime(), messages[1].getSender()); Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.new_message) .setStyle(new NotificationCompat.MessagingStyle(resources.getString(R.string.reply_name)) .addMessage(message1) .addMessage(message2)) .build();
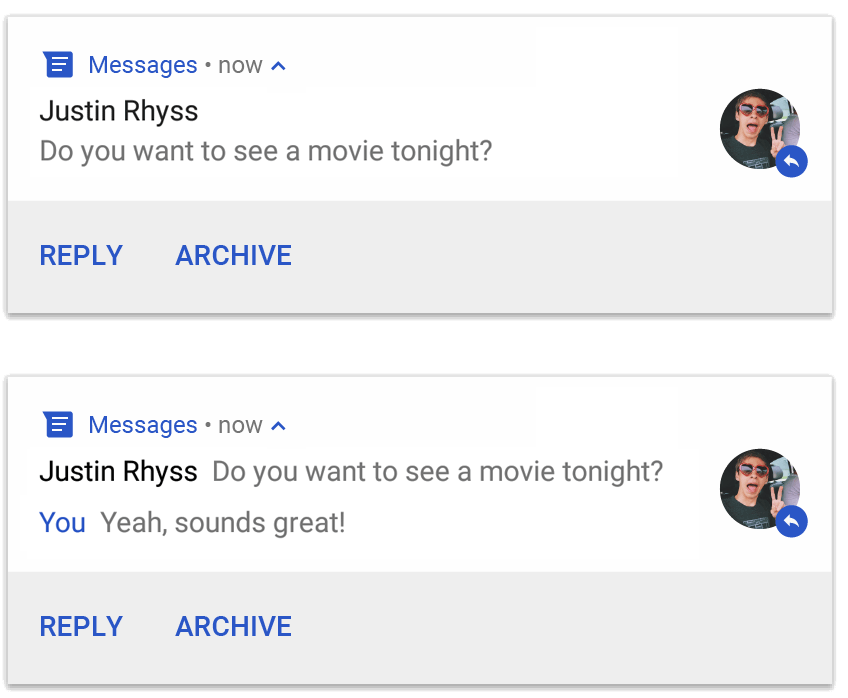
NotificationCompat.MessagingStyle
的通知。
使用 NotificationCompat.MessagingStyle
时,为 setContentTitle()
和 setContentText()
赋予的任何值均会被忽略。
您可以调用 setConversationTitle()
来添加显示在会话上方的标题。这可能是用户创建的群组名称,也可能是会话参与者的列表(如果没有具体名称)。请勿为一对一聊天设置会话标题,因为如果有此字段,系统会将其当做表明会话是群组的提示。
此样式仅适用于搭载 Android 7.0(API 级别 24)及更高版本的设备。如前所示,使用兼容性库 (NotificationCompat
) 时,采用 MessagingStyle
的通知会自动回退到支持的展开式通知样式。
在为聊天会话创建此类通知时,请添加直接回复操作。
使用媒体控件创建通知
应用 MediaStyleNotificationHelper.MediaStyle
可显示媒体播放控件和曲目信息。
在构造函数中指定关联的 MediaSession
。这样,Android 就可以显示有关您的媒体的正确信息。
您最多可调用 addAction()
5 次,以显示最多 5 个图标按钮。调用 setLargeIcon()
可设置专辑封面。
与其他通知样式不同,MediaStyle
还允许您通过指定三个操作按钮(这些操作按钮也应在收起的视图中显示)修改收起尺寸的内容视图。为此,请向 setShowActionsInCompactView()
提供操作按钮索引。
以下示例展示了如何创建包含媒体控件的通知:
Kotlin
val notification = NotificationCompat.Builder(context, CHANNEL_ID) // Show controls on lock screen even when user hides sensitive content. .setVisibility(NotificationCompat.VISIBILITY_PUBLIC) .setSmallIcon(R.drawable.ic_stat_player) // Add media control buttons that invoke intents in your media service .addAction(R.drawable.ic_prev, "Previous", prevPendingIntent) // #0 .addAction(R.drawable.ic_pause, "Pause", pausePendingIntent) // #1 .addAction(R.drawable.ic_next, "Next", nextPendingIntent) // #2 // Apply the media style template. .setStyle(MediaStyleNotificationHelper.MediaStyle(mediaSession) .setShowActionsInCompactView(1 /* #1: pause button \*/)) .setContentTitle("Wonderful music") .setContentText("My Awesome Band") .setLargeIcon(albumArtBitmap) .build()
Java
Notification notification = new NotificationCompat.Builder(context, CHANNEL_ID) // Show controls on lock screen even when user hides sensitive content. .setVisibility(NotificationCompat.VISIBILITY_PUBLIC) .setSmallIcon(R.drawable.ic_stat_player) // Add media control buttons that invoke intents in your media service .addAction(R.drawable.ic_prev, "Previous", prevPendingIntent) // #0 .addAction(R.drawable.ic_pause, "Pause", pausePendingIntent) // #1 .addAction(R.drawable.ic_next, "Next", nextPendingIntent) // #2 // Apply the media style template. .setStyle(new MediaStyleNotificationHelper.MediaStyle(mediaSession) .setShowActionsInCompactView(1 /* #1: pause button */)) .setContentTitle("Wonderful music") .setContentText("My Awesome Band") .setLargeIcon(albumArtBitmap) .build();
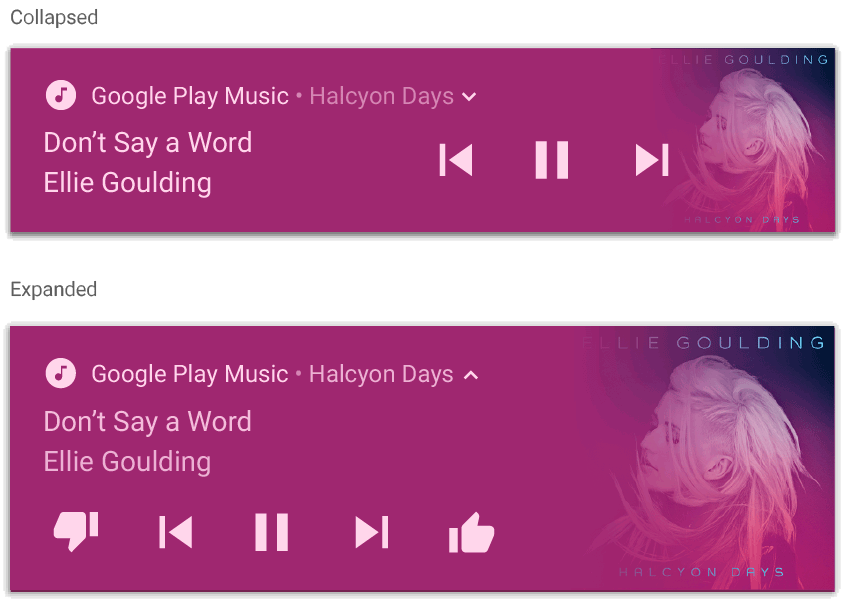
MediaStyleNotificationHelper.MediaStyle
的通知。
其他资源
如需详细了解 MediaStyle
和可展开通知,请参阅以下参考文档。