Android 16 introduces great new features and APIs for developers. The following sections summarize these features to help you get started with the related APIs.
For a detailed list of new, modified, and removed APIs, read the API diff report. For details on new APIs visit the Android API reference — new APIs are highlighted for visibility.You should also review areas where platform changes might affect your apps. For more information, see the following pages:
- Behavior changes that affect apps when they target Android 16
- Behavior changes that affect all apps regardless of
targetSdkVersion
.
Core functionality
Android includes new APIs that expand core capabilities of the Android system.
Two Android API releases in 2025
- This preview is for the next major release of Android with a planned launch in Q2 of 2025. This release is similar to all of our API releases in the past, where we can have planned behavior changes that are often tied to a targetSdkVersion.
- We're planning the major release a quarter earlier (Q2 rather than Q3 in prior years) to better align with the schedule of device launches across our ecosystem, so more devices can get the major release of Android sooner. With the major release coming in Q2, you'll need to do your annual compatibility testing a few months earlier than in previous years to make sure your apps are ready.
- We plan to have another release in Q4 of 2025 which also will include new developer APIs. The Q2 major release will be the only release in 2025 to include planned behavior changes that could affect apps.
In addition to new developer APIs, the Q4 minor release will pick up feature updates, optimizations, and bug fixes; it will not include any app-impacting behavior changes.
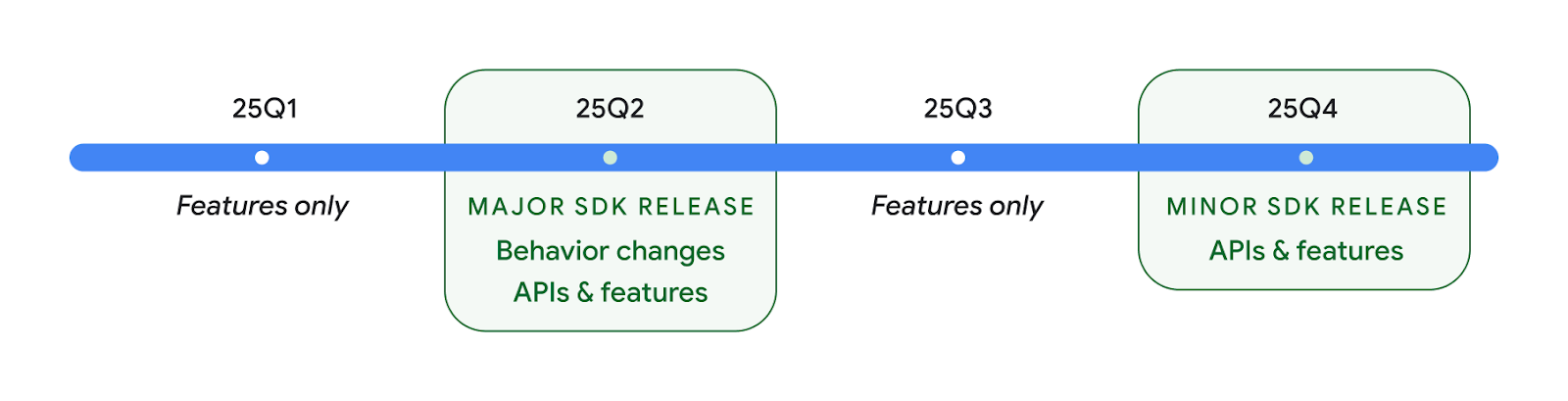
We'll continue to have quarterly Android releases. The Q1 and Q3 updates in-between the API releases will provide incremental updates to help ensure continuous quality. We're actively working with our device partners to bring the Q2 release to as many devices as possible.
Using new APIs with major and minor releases
Guarding a code block with a check for API level is done today using
the SDK_INT
constant with
VERSION_CODES
. This will continue
to be supported for major Android releases.
if (SDK_INT >= VERSION_CODES.BAKLAVA) {
// Use APIs introduced in Android 16
}
The new SDK_INT_FULL
constant can be used for API checks against both major and minor versions with
the new VERSION_CODES_FULL
enumeration.
if (SDK_INT_FULL >= VERSION_CODES_FULL.[MAJOR or MINOR RELEASE]) {
// Use APIs introduced in a major or minor release
}
You can also use the
Build.getMinorSdkVersion()
method to get just the minor SDK version.
val minorSdkVersion = Build.getMinorSdkVersion(VERSION_CODES_FULL.BAKLAVA)
These APIs have not yet been finalized and are subject to change, so please send us feedback if you have any concerns.
User experience and system UI
Android 16 gives app developers and users more control and flexibility for configuring their device to fit their needs.
Progress-centric notifications
Android 16 introduces progress-centric notifications to help users seamlessly track user-initiated, start-to-end journeys.
Notification.ProgressStyle
is a new notification
style that lets you create progress-centric notifications. Key use cases include
rideshare, delivery, and navigation. Within the Notification.ProgressStyle
class, you can denote states and milestones in a user journey using
points and segments.
To learn more, see the Progress-centric notifications documentation page.
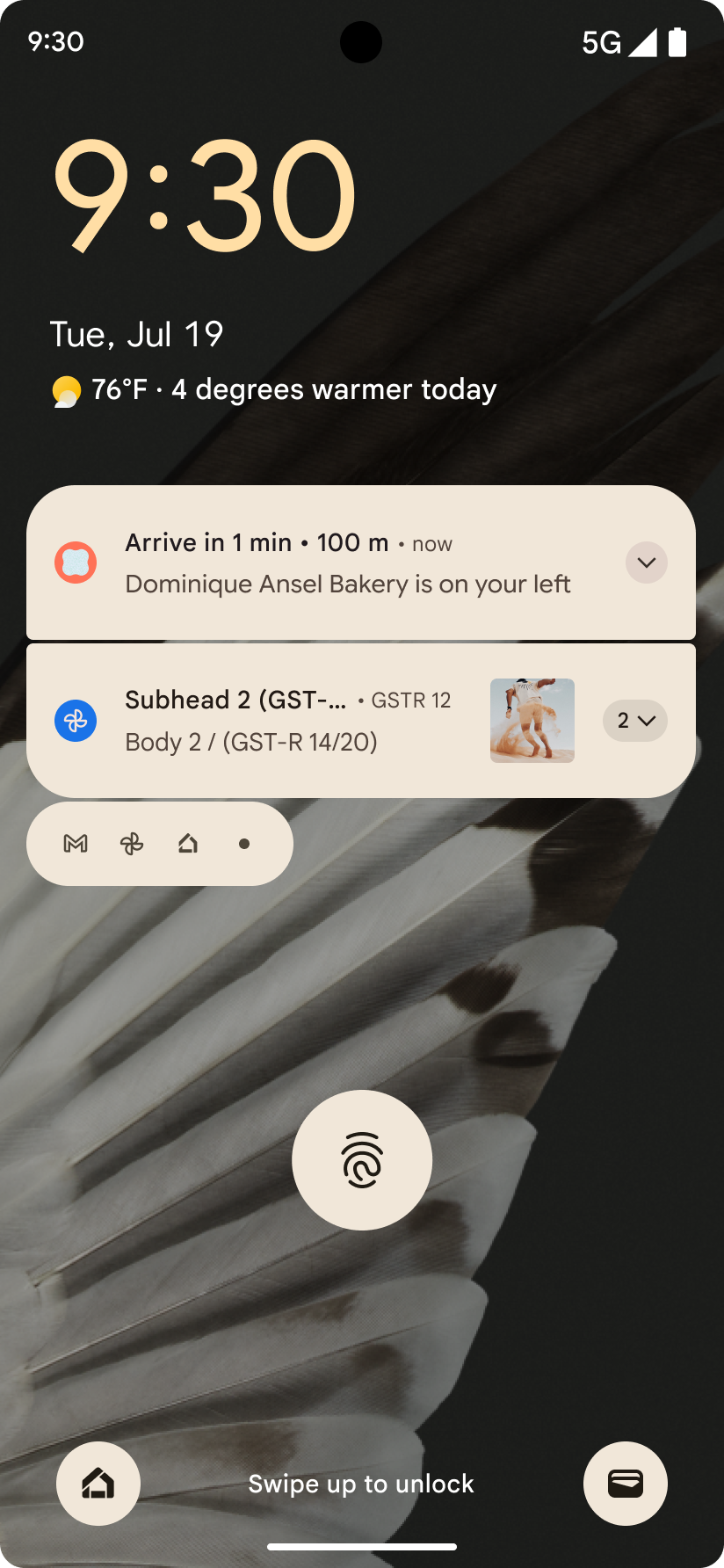
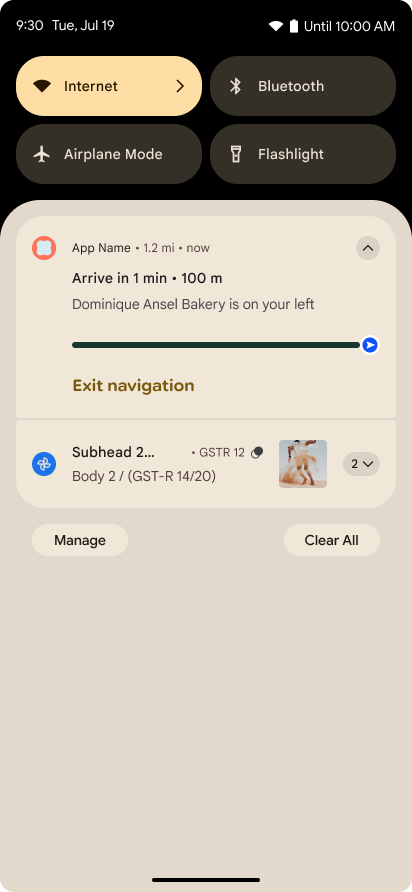
Predictive back updates
Android 16 adds new APIs to help you enable predictive back system animations in
gesture navigation such as the back-to-home animation. Registering the
onBackInvokedCallback
with the new
PRIORITY_SYSTEM_NAVIGATION_OBSERVER
allows your app to
receive the regular onBackInvoked
call whenever the
system handles a back navigation without impacting the normal back navigation
flow.
Android 16 additionally adds the
finishAndRemoveTaskCallback()
and
moveTaskToBackCallback
. By registering these callbacks
with the OnBackInvokedDispatcher
, the system can trigger
specific behaviors and play corresponding ahead-of-time animations when the back
gesture is invoked.
Richer haptics
Android has exposed control over the haptic actuator ever since its inception.
Android 11 added support for more complex haptic effects that more advanced
actuators could support through
VibrationEffect.Compositions
of device-defined semantic
primitives.
Android 16 adds haptic APIs that let apps define the amplitude and frequency curves of a haptic effect while abstracting away differences between device capabilities.
Developer productivity and tools
While most of our work to improve your productivity centers around tools like Android Studio, Jetpack Compose, and the Android Jetpack libraries, we always look for ways in the platform to help you realize your vision.
Content handling for live wallpapers
In Android 16, the live wallpaper framework is gaining a new content API to
address the challenges of dynamic, user-driven wallpapers. Currently, live
wallpapers incorporating user-provided content require complex, service-specific
implementations. Android 16 introduces
WallpaperDescription
and
WallpaperInstance
. WallpaperDescription lets you
identify distinct instances of a live wallpaper from the same service. For
example, a wallpaper that has instances on both the home screen and on the lock
screen may have unique content in both places. The wallpaper picker and
WallpaperManager
use this metadata to better present
wallpapers to users, streamlining the process for you to create diverse and
personalized live wallpaper experiences.
Performance and battery
Android 16 introduces APIs that help gather insights about your apps.
System-triggered profiling
ProfilingManager
was
added in Android 15, giving apps the ability to
request profiling data collection using Perfetto on public devices in the field.
However, since this profiling must be started from the app, critical flows such
as startups or ANRs would be difficult or impossible for apps to capture.
To help with this, Android 16 introduces system-triggered profiling to
ProfilingManager
. Apps can register interest in receiving traces for certain
triggers such as cold start reportFullyDrawn
or ANRs, and then the system starts and stops a trace on the app's behalf. After
the trace completes, the results are delivered to the app's data directory.
Start component in ApplicationStartInfo
ApplicationStartInfo
was added in Android
15, allowing an app to see reasons
for process start, start type, start times, throttling, and other useful
diagnostic data. Android 16 adds
getStartComponent()
to distinguish what component type triggered the start, which can be helpful for
optimizing the startup flow of your app.
Better job introspection
The JobScheduler#getPendingJobReason()
API returns a reason why a job
might be pending. However, a job might be pending for multiple reasons.
In Android 16, we are introducing a new API
JobScheduler#getPendingJobReasons(int jobId)
, which returns multiple
reasons why a job is pending, due to both explicit constraints set by the
developer and implicit constraints set by the system.
We're also introducing
JobScheduler#getPendingJobReasonsHistory(int jobId)
, which returns a list
of the most recent constraint changes.
We recommend using the API to help you debug why your jobs may not be executing, especially if you're seeing reduced success rates of certain tasks or have bugs around latency of certain job completion. For example, updating widgets in the background failed to occur or prefetch job failed to be called prior to app start.
This can also better help you understand if certain jobs are not completing due to system defined constraints versus explicitly set constraints.
Adaptive refresh rate
Adaptive refresh rate (ARR), introduced in Android 15, enables the display refresh rate on supported hardware to adapt to the content frame rate using discrete VSync steps. This reduces power consumption while eliminating the need for potentially jank-inducing mode-switching.
Android 16 introduces hasArrSupport()
and
getSuggestedFrameRate(int)
while restoring
getSupportedRefreshRates()
to make it easier for your apps to take
advantage of ARR. RecyclerView
1.4 internally supports ARR when it is settling from a fling or
smooth scroll, and we're continuing our work to add ARR
support into more Jetpack libraries. This frame rate article covers
many of the APIs you can use to set the frame rate so that your app can directly
use ARR.
Headroom APIs in ADPF
The SystemHealthManager
introduces the
getCpuHeadroom
and
getGpuHeadroom
APIs, designed to provide games and
resource-intensive apps with estimates of available CPU and GPU resources. These
methods offer a way for you to gauge how your app or game can best improve
system health, particularly when used in conjunction with other Android Dynamic
Performance Framework (ADPF) APIs that detect thermal
throttling.
By using CpuHeadroomParams
and
GpuHeadroomParams
on supported devices, you can
customize the time window used to compute the headroom and select between
average or minimum resource availability. This can help you reduce your CPU or
GPU resource usage accordingly, leading to better user experiences and improved
battery life.
Accessibility
Android 16 adds new accessibility APIs and features that can help you bring your app to every user.
Improved accessibility APIs
Android 16 adds additional APIs to enhance UI semantics that help improve consistency for users that rely on accessibility services, such as TalkBack.
Outline text for maximum text contrast
Users with low vision often have reduced contrast sensitivity, making it challenging to distinguish objects from their backgrounds. To help these users, Android 16 introduces outline text, replacing high contrast text, which draws a larger contrasting area around text to greatly improve legibility.
Android 16 contains new AccessibilityManager
APIs to let
your apps check or register a listener to
see if this mode is enabled. This is primarily for UI Toolkits like Compose to
offer a similar visual experience. If you maintain a UI Toolkit library or your
app performs custom text rendering that bypasses the
android.text.Layout
class then you can use this to know
when outline text is enabled.
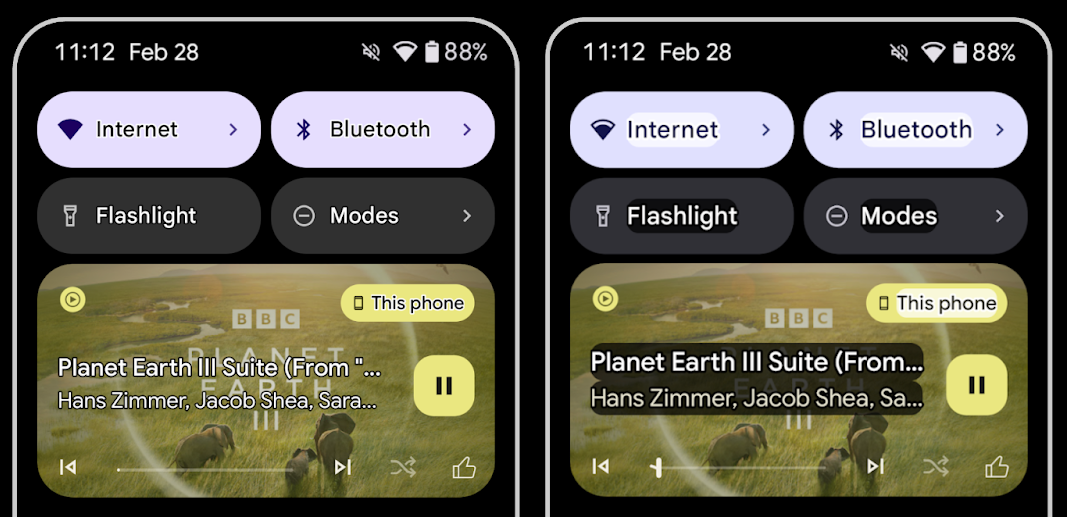
Duration added to TtsSpan
Android 16 extends TtsSpan
with a TYPE_DURATION
,
consisting of ARG_HOURS
, ARG_MINUTES
,
and ARG_SECONDS
. This lets you directly annotate time
duration, ensuring accurate and consistent text-to-speech output with services
like TalkBack.
Support elements with multiple labels
Android currently allows UI elements to derive their accessibility label from
another, and now offers the ability for multiple labels to be associated, a
common scenario in web content. By introducing a list-based API within
AccessibilityNodeInfo
, Android can directly support these
multi-label relationships. As part of this change, we've deprecated
AccessibilityNodeInfo#setLabeledBy
and
#getLabeledBy
in favor of
#addLabeledBy
, #removeLabeledBy
, and
#getLabeledByList
.
Improved support for expandable elements
Android 16 adds accessibility APIs that allow you to convey the expanded or
collapsed state of interactive elements, such as menus and expandable lists. By
setting the expanded state using setExpandedState
and
dispatching TYPE_WINDOW_CONTENT_CHANGED AccessibilityEvents
with a CONTENT_CHANGE_TYPE_EXPANDED
content change type,
you can ensure that screen readers like TalkBack announce
state changes, providing a more intuitive and inclusive user experience.
Indeterminate ProgressBars
Android 16 adds RANGE_TYPE_INDETERMINATE
, giving a way for
you to expose RangeInfo
for both determinate and
indeterminate ProgressBar
widgets, allowing services like
TalkBack to more consistently provide feedback for progress
indicators.
Tri-state CheckBox
The new AccessibilityNodeInfo
getChecked
and setChecked(int)
methods in Android 16 now support a "partially checked" state in addition to
"checked" and "unchecked." This replaces the deprecated boolean
isChecked
and setChecked(boolean)
.
Supplemental descriptions
When an accessibility service describes a ViewGroup
, it
combines content labels from its child views. If you provide a
contentDescription
for the ViewGroup
, accessibility services assume you are
also overriding the description of non-focusable child views. This can be
problematic if you want to label things like a drop-down (for example, "Font
Family") while preserving the current selection for accessibility (for example,
"Roboto"). Android 16 adds setSupplementalDescription
so
you can provide text that provides information about a ViewGroup
without
overriding information from its children.
Required form fields
Android 16 adds setFieldRequired
to
AccessibilityNodeInfo
so apps can tell an accessibility
service that input to a form field is required. This is an important scenario
for users filling out many types of forms, even things as simple as a required
terms and conditions checkbox, helping users to consistently identify and
quickly navigate between required fields.
Phone as microphone input for voice calls with LEA hearing aids
Android 16 adds the capability for users of LE Audio hearing aids to switch between the built-in microphones on the hearing aids and the microphone on their phone for voice calls. This can be helpful in noisy environments or other situations where the hearing aid's microphones might not perform well.
Ambient volume controls for LEA hearing aids
Android 16 adds the capability for users of LE Audio hearing aids to adjust the volume of ambient sound that is picked up by the hearing aid's microphones. This can be helpful in situations where background noise is too loud or too quiet.
Camera
Android 16 enhances support for professional camera users, allowing for hybrid
auto exposure along with precise color temperature and tint adjustments. A new
night mode indicator helps your app know when to switch to and from a night mode
camera session. New Intent
actions make it easier to capture motion photos,
and we're continuing to improve UltraHDR images with support for HEIC encoding
and new parameters from the ISO 21496-1 draft standard.
Hybrid auto-exposure
Android 16 adds new hybrid auto-exposure modes to Camera2, allowing you to manually control specific aspects of exposure while letting the auto-exposure (AE) algorithm handle the rest. You can control ISO + AE, and exposure time + AE, providing greater flexibility compared to the current approach where you either have full manual control or rely entirely on auto-exposure.
fun setISOPriority() {
// ... (Your existing code before the snippet) ...
val availablePriorityModes = mStaticInfo.characteristics.get(
CameraCharacteristics.CONTROL_AE_AVAILABLE_PRIORITY_MODES
)
// ... (Your existing code between the snippets) ...
// Turn on AE mode to set priority mode
reqBuilder.set(
CaptureRequest.CONTROL_AE_MODE,
CameraMetadata.CONTROL_AE_MODE_ON
)
reqBuilder.set(
CaptureRequest.CONTROL_AE_PRIORITY_MODE,
CameraMetadata.CONTROL_AE_PRIORITY_MODE_SENSOR_SENSITIVITY_PRIORITY
)
reqBuilder.set(
CaptureRequest.SENSOR_SENSITIVITY,
TEST_SENSITIVITY_VALUE
)
val request: CaptureRequest = reqBuilder.build()
// ... (Your existing code after the snippet) ...
}
Precise color temperature and tint adjustments
Android 16 adds camera support for fine color temperature and tint adjustments
to better support professional video recording applications. In previous Android
versions, you could control white balance settings through
CONTROL_AWB_MODE
, which contains options limited to a
preset list, such as Incandescent,
Cloudy, and Twilight. The
COLOR_CORRECTION_MODE_CCT
enables the use of
COLOR_CORRECTION_COLOR_TEMPERATURE
and
COLOR_CORRECTION_COLOR_TINT
for precise adjustments of
white balance based on the correlated color temperature.
fun setCCT() {
// ... (Your existing code before this point) ...
val colorTemperatureRange: Range<Int> =
mStaticInfo.characteristics[CameraCharacteristics.COLOR_CORRECTION_COLOR_TEMPERATURE_RANGE]
// Set to manual mode to enable CCT mode
reqBuilder[CaptureRequest.CONTROL_AWB_MODE] = CameraMetadata.CONTROL_AWB_MODE_OFF
reqBuilder[CaptureRequest.COLOR_CORRECTION_MODE] = CameraMetadata.COLOR_CORRECTION_MODE_CCT
reqBuilder[CaptureRequest.COLOR_CORRECTION_COLOR_TEMPERATURE] = 5000
reqBuilder[CaptureRequest.COLOR_CORRECTION_COLOR_TINT] = 30
val request: CaptureRequest = reqBuilder.build()
// ... (Your existing code after this point) ...
}
The following examples show how a photo would look after applying different color temperature and tint adjustments:
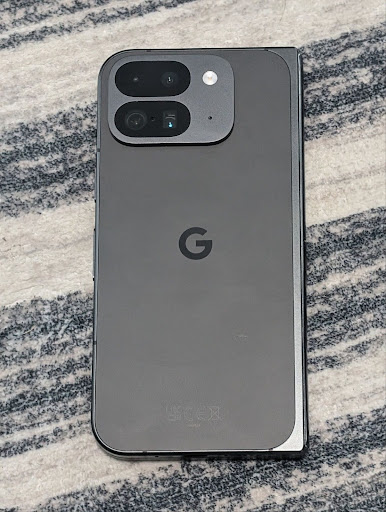
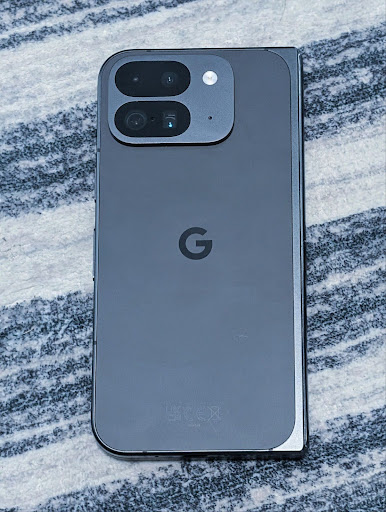
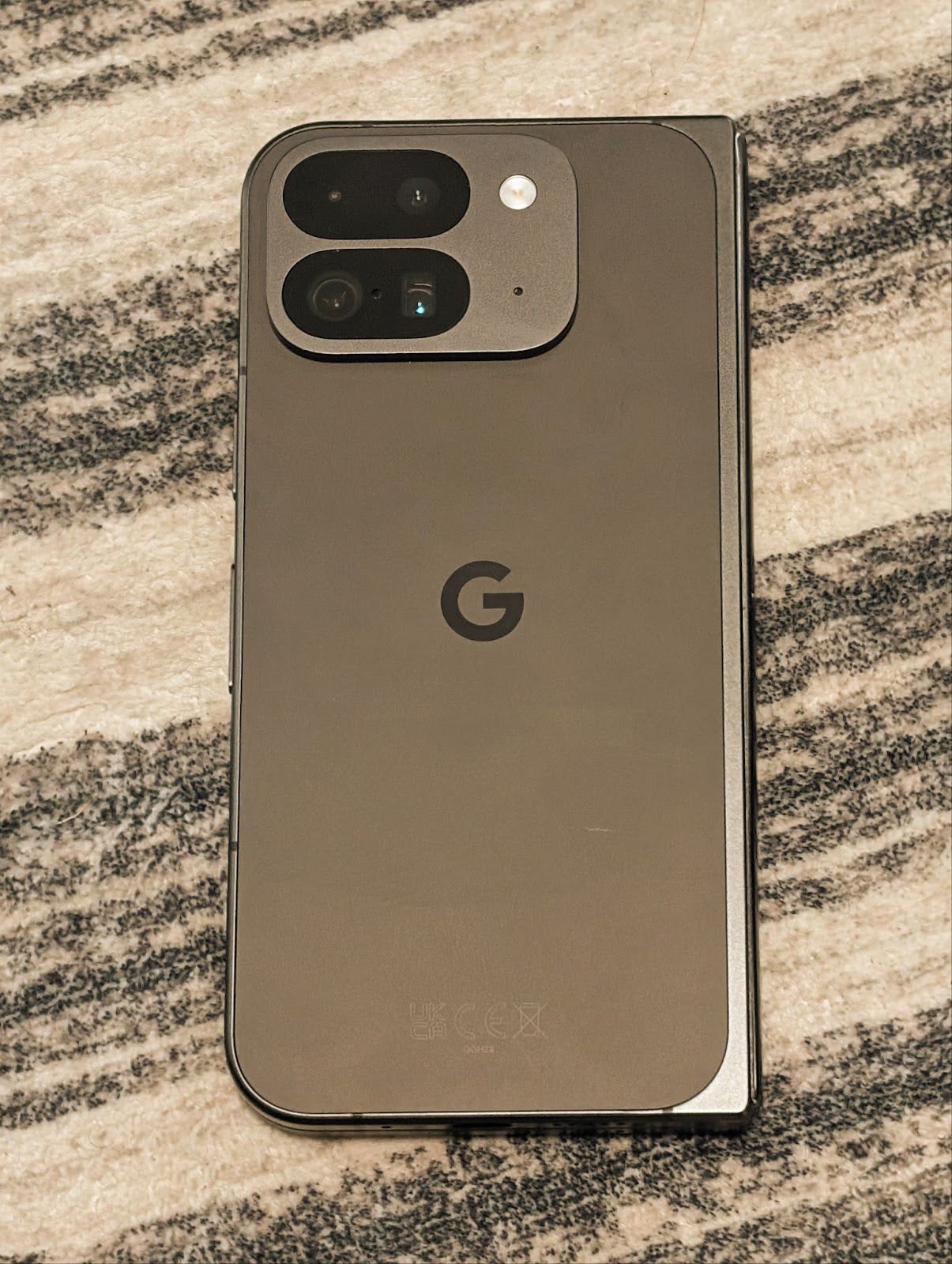
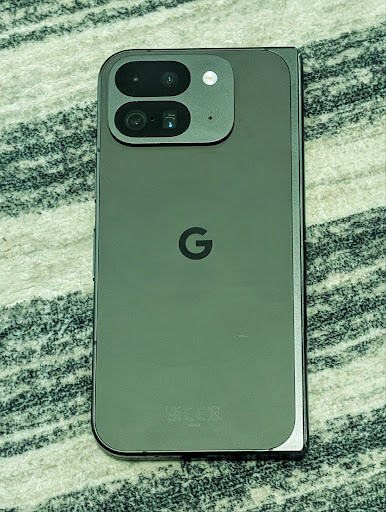
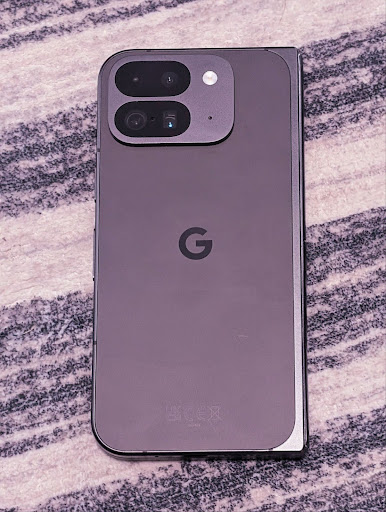
Camera night mode scene detection
To help your app know when to switch to and from a night mode camera session,
Android 16 adds EXTENSION_NIGHT_MODE_INDICATOR
. If
supported, it's available in the CaptureResult
within
Camera2.
This is the API we briefly mentioned as coming soon in the How Instagram enabled users to take stunning low light photos blog post. That post is a practical guide on how to implement night mode together with a case study that links higher-quality in-app night mode photos with an increase in the number of photos shared from the in-app camera.
Motion photo capture intent actions
Android 16 adds standard Intent actions —
ACTION_MOTION_PHOTO_CAPTURE
, and
ACTION_MOTION_PHOTO_CAPTURE_SECURE
— which request that
the camera application capture a motion photo and return
it.
You must either pass an extra EXTRA_OUTPUT
to control
where the image will be written, or a Uri
through
Intent.setClipData(ClipData)
. If you don't set a
ClipData
, it will be copied there for you when calling
Context.startActivity(Intent)
.
UltraHDR image enhancements
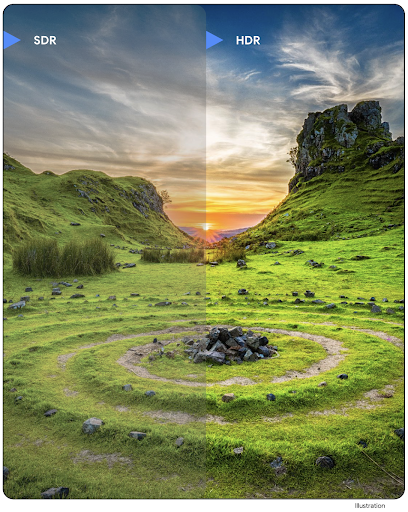
Android 16 continues our work to deliver dazzling image quality with UltraHDR
images. It adds support for UltraHDR images in the HEIC file
format. These images will get ImageFormat
type
HEIC_ULTRAHDR
and will contain an embedded gainmap similar
to the existing UltraHDR JPEG format. We're working on AVIF support for UltraHDR
as well, so stay tuned.
In addition, Android 16 implements additional parameters in UltraHDR from the ISO 21496-1 draft standard, including the ability to get and set the colorspace that gainmap math should be applied in, as well as support for HDR encoded base images with SDR gainmaps.
Graphics
Android 16 includes the latest graphics improvements, such as custom graphic effects with AGSL.
Custom graphical effects with AGSL
Android 16 adds RuntimeColorFilter
and
RuntimeXfermode
, allowing you to author complex effects like
Threshold, Sepia, and Hue Saturation and apply them to draw calls. Since Android
13, you've been able to use AGSL to create custom
RuntimeShaders that extend Shader
. The new API
mirrors this, adding an AGSL-powered RuntimeColorFilter
that
extends ColorFilter
, and a Xfermode
effect that
lets you implement AGSL-based custom compositing and blending between source and
destination pixels.
private val thresholdEffectString = """
uniform half threshold;
half4 main(half4 c) {
half luminosity = dot(c.rgb, half3(0.2126, 0.7152, 0.0722));
half bw = step(threshold, luminosity);
return bw.xxx1 * c.a;
}"""
fun setCustomColorFilter(paint: Paint) {
val filter = RuntimeColorFilter(thresholdEffectString)
filter.setFloatUniform(0.5);
paint.colorFilter = filter
}
Connectivity
Android 16 updates the platform to give your app access to the latest advances in communication and wireless technologies.
Ranging with enhanced security
Android 16 adds support for robust security features in Wi-Fi location on supported devices with Wi-Fi 6's 802.11az, allowing apps to combine the higher accuracy, greater scalability, and dynamic scheduling of the protocol with security enhancements including AES-256-based encryption and protection against MITM attacks. This allows it to be used more safely in proximity use cases, such as unlocking a laptop or a vehicle door. 802.11az is integrated with the Wi-Fi 6 standard, leveraging its infrastructure and capabilities for wider adoption and easier deployment.
Generic ranging APIs
Android 16 includes the new RangingManager
, which provides
ways to determine the distance and angle on supported hardware between the local
device and a remote device. RangingManager
supports the usage of a variety of
ranging technologies such as BLE channel sounding, BLE RSSI-based ranging, Ultra
Wideband, and Wi-Fi round trip time.
Media
Android 16 includes a variety of features that improve the media experience.
Photo picker improvements
The photo picker provides a safe, built-in way for users to grant your app access to selected images and videos from both local and cloud storage, instead of their entire media library. Using a combination of Modular System Components through Google System Updates and Google Play services, it's supported back to Android 4.4 (API level 19). Integration requires just a few lines of code with the associated Android Jetpack library.
Android 16 includes the following improvements to the photo picker:
- Embedded photo picker: New APIs that enable apps to embed the photo picker into their view hierarchy. This allows it to feel like a more integrated part of the app while still leveraging the process isolation that allows users to select media without the app needing overly broad permissions. To maximize compatibility across platform versions and simplify your integration, you'll want to use the forthcoming Android Jetpack library if you want to integrate the embedded photo picker.
- Cloud search in photo picker: New APIs that enable searching from the cloud media provider for the Android photo picker. Search functionality in the photo picker is coming soon.
Advanced Professional Video
Android 16 introduces support for the Advanced Professional Video (APV) codec which is designed to be used for professional level high quality video recording and post production.
The APV codec standard has the following features:
- Perceptually lossless video quality (close to raw video quality)
- Low complexity and high throughput intra-frame-only coding (without pixel domain prediction) to better support editing workflows
- Support for high bit-rate range up to a few Gbps for 2K, 4K and 8K resolution content, enabled by a lightweight entropy coding scheme
- Frame tiling for immersive content and for enabling parallel encoding and decoding
- Support for various chroma sampling formats and bit-depths
- Support for multiple decoding and re-encoding without severe visual quality degradation
- Support multi-view video and auxiliary video like depth, alpha, and preview
- Support for HDR10/10+ and user-defined metadata
A reference implementation of APV is provided through the OpenAPV project. Android 16 will implement support for the APV 422-10 Profile that provides YUV 422 color sampling along with 10-bit encoding and for target bitrates of up to 2Gbps.
Privacy
Android 16 includes a variety of features that help app developers protect user privacy.
Health Connect updates
Health Connect in the developer preview adds ACTIVITY_INTENSITY
, a new
data type defined according to World Health Organization guidelines around
moderate and vigorous activity. Each record requires the start time, the end
time and whether the activity intensity is moderate or vigorous.
Health Connect also contains updated APIs supporting health records. This allows apps to read and write medical records in FHIR format with explicit user consent. This API is in an early access program. If you'd like to participate, sign up to be part of our early access program.
Privacy Sandbox on Android
Android 16 incorporates the latest version of the Privacy Sandbox on Android, part of our ongoing work to develop technologies where users know their privacy is protected. Our website has more about the Privacy Sandbox on Android developer beta program to help you get started. Check out the SDK Runtime which allows SDKs to run in a dedicated runtime environment separate from the app they are serving, providing stronger safeguards around user data collection and sharing.
Security
Android 16 includes features that help you enhance your app's security and protect your app's data.
Key sharing API
Android 16 adds APIs that support sharing access to
Android Keystore keys with other apps. The new
KeyStoreManager
class supports
granting and revoking access to keys
by app uid, and includes an API for apps to access shared
keys.
Device form factors
Android 16 gives your apps the support to get the most out of Android's form factors.
Standardized picture and audio quality framework for TVs
The new MediaQuality
package in Android 16 exposes
a set of standardized APIs for access to audio and picture profiles and
hardware-related settings. This allows streaming apps to query profiles and
apply them to media dynamically:
- Movies mastered with a wider dynamic range require greater color accuracy to see subtle details in shadows and adjust to ambient light, so a profile that prefers color accuracy over brightness may be appropriate.
- Live sporting events are often mastered with a narrow dynamic range, but are often watched in daylight, so a profile that preferences brightness over color accuracy can give better results.
- Fully interactive content wants minimal processing to reduce latency, and wants higher frame rates, which is why many TV's ship with a game profile.
The API allows apps to switch between profiles and users to enjoy tuning supported TVs to best suit their content.
Internationalization
Android 16 adds features and capabilities that complement the user experience when a device is used in different languages.
Vertical text
Android 16 adds low-level support for rendering and measuring text vertically to
provide foundational vertical writing support for library developers. This is
particularly useful for languages like Japanese that commonly use vertical
writing systems. A new flag,
VERTICAL_TEXT_FLAG
,
has been added to the Paint
class. When
this flag is set using
Paint.setFlags
, Paint's
text measurement APIs will report vertical advances instead of horizontal
advances, and Canvas
will draw text
vertically.
val text = "「春は、曙。」"
Box(
Modifier.padding(innerPadding).background(Color.White).fillMaxSize().drawWithContent {
drawIntoCanvas { canvas ->
val paint = Paint().apply { textSize = 64.sp.toPx() }
// Draw text vertically
paint.flags = paint.flags or VERTICAL_TEXT_FLAG
val height = paint.measureText(text)
canvas.nativeCanvas.drawText(
text,
0,
text.length,
size.width / 2,
(size.height - height) / 2,
paint
)
}
}
) {}
Measurement system customization
Users can now customize their measurement system in regional preferences within
Settings. The user preference is included as part of the locale code, so you can
register a BroadcastReceiver
on
ACTION_LOCALE_CHANGED
to handle locale configuration changes when
regional preferences change.
Using formatters can help match the local experience. For example, "0.5 in" in English (United States), is "12,7 mm" for a user who has set their phone to English (Denmark) or who uses their phone in English (United States) with the metric system as the measurement system preference.
To find these settings, open the Settings app and navigate to System > Languages & region.