アプリは WindowInsetsCompat
を使用して、システムバーを操作する場合と同様に、画面キーボード(IME)をクエリして制御できます。アプリで WindowInsetsAnimationCompat
を使用して、ソフトウェア キーボードの開閉時にシームレスな遷移を作成することもできます。
前提条件
ソフトウェア キーボードの制御とアニメーションを設定する前に、端から端まで表示するようにアプリを構成します。これにより、システムバーや画面キーボードなどのシステム ウィンドウの切り欠きを処理できます。
キーボード ソフトウェアの公開設定を確認する
WindowInsets
を使用して、ソフトウェア キーボードの表示を確認します。
Kotlin
val insets = ViewCompat.getRootWindowInsets(view) ?: return val imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()) val imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom
Java
WindowInsetsCompat insets = ViewCompat.getRootWindowInsets(view); boolean imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()); int imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom;
または、ViewCompat.setOnApplyWindowInsetsListener
を使用して、ソフトウェア キーボードの可視性の変更をモニタリングすることもできます。
Kotlin
ViewCompat.setOnApplyWindowInsetsListener(view) { _, insets -> val imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()) val imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom insets }
Java
ViewCompat.setOnApplyWindowInsetsListener(view, (v, insets) -> { boolean imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()); int imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom; return insets; });
アニメーションをソフトウェア キーボードと同期する
ユーザーがテキスト入力フィールドをタップすると、次の例に示すように、キーボードが画面の下部からスライドして表示されます。
図 2 の「非同期」というラベルの付いた例は、Android 10(API レベル 29)のデフォルトの動作を示しています。この動作では、アプリのテキスト フィールドとコンテンツはキーボードのアニメーションと同期せずに、所定の位置にスナップされます。この動作は視覚的に不快に感じる場合があります。
Android 11(API レベル 30)以降では、
WindowInsetsAnimationCompat
を使用して、アプリの遷移と画面の下部からキーボードをスライドして上下させる操作を同期できます。図 2 の「同期済み」の例に示すように、これはよりスムーズに見えます。
キーボード アニメーションと同期されるビューを使用して WindowInsetsAnimationCompat.Callback
を構成します。
Kotlin
ViewCompat.setWindowInsetsAnimationCallback( view, object : WindowInsetsAnimationCompat.Callback(DISPATCH_MODE_STOP) { // Override methods. } )
Java
ViewCompat.setWindowInsetsAnimationCallback( view, new WindowInsetsAnimationCompat.Callback( WindowInsetsAnimationCompat.Callback.DISPATCH_MODE_STOP ) { // Override methods. });
WindowInsetsAnimationCompat.Callback
でオーバーライドできるメソッドはいくつかあります。onPrepare()
、onStart()
、onProgress()
、onEnd()
です。レイアウトを変更する前に、まず onPrepare()
を呼び出します。
onPrepare
は、インセット アニメーションの開始時と、アニメーションが原因でビューが再レイアウトされる前に呼び出されます。これを使用して、開始状態を保存できます。この場合は、ビューの下部座標です。
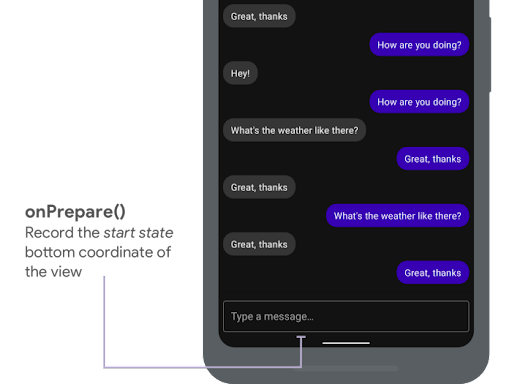
onPrepare()
を使用して開始状態を記録します。次のスニペットは、onPrepare
の呼び出しの例を示しています。
Kotlin
var startBottom = 0f override fun onPrepare( animation: WindowInsetsAnimationCompat ) { startBottom = view.bottom.toFloat() }
Java
float startBottom; @Override public void onPrepare( @NonNull WindowInsetsAnimationCompat animation ) { startBottom = view.getBottom(); }
onStart
は、インセット アニメーションの開始時に呼び出されます。これを使用して、すべてのビュー プロパティをレイアウト変更の最終状態に設定できます。いずれかのビューに OnApplyWindowInsetsListener
コールバックが設定されている場合は、この時点ですでに呼び出されています。ここで、ビュー プロパティの最終状態を保存することをおすすめします。
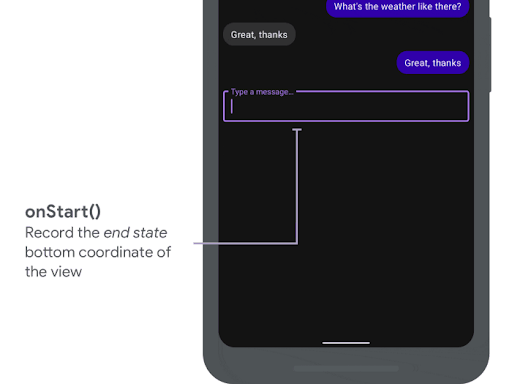
onStart()
を使用して終了状態を記録します。次のスニペットは、onStart
の呼び出しの例を示しています。
Kotlin
var endBottom = 0f override fun onStart( animation: WindowInsetsAnimationCompat, bounds: WindowInsetsAnimationCompat.BoundsCompat ): WindowInsetsAnimationCompat.BoundsCompat { // Record the position of the view after the IME transition. endBottom = view.bottom.toFloat() return bounds }
Java
float endBottom; @NonNull @Override public WindowInsetsAnimationCompat.BoundsCompat onStart( @NonNull WindowInsetsAnimationCompat animation, @NonNull WindowInsetsAnimationCompat.BoundsCompat bounds ) { endBottom = view.getBottom(); return bounds; }
onProgress
は、アニメーションの実行の一環としてインセットが変更されたときに呼び出されるため、オーバーライドして、キーボード アニメーションのすべてのフレームで通知を受け取ることができます。ビューのプロパティを更新して、ビューがキーボードと同期してアニメーション化されるようにします。
これで、レイアウトの変更はすべて完了です。たとえば、View.translationY
を使用してビューをシフトすると、このメソッドの呼び出しごとに値が徐々に減少し、最終的に 0
に達して元のレイアウト位置に戻ります。
onProgress()
を使用してアニメーションを同期する。次のスニペットは、onProgress
の呼び出しの例を示しています。
Kotlin
override fun onProgress( insets: WindowInsetsCompat, runningAnimations: MutableList<WindowInsetsAnimationCompat> ): WindowInsetsCompat { // Find an IME animation. val imeAnimation = runningAnimations.find { it.typeMask and WindowInsetsCompat.Type.ime() != 0 } ?: return insets // Offset the view based on the interpolated fraction of the IME animation. view.translationY = (startBottom - endBottom) * (1 - imeAnimation.interpolatedFraction) return insets }
Java
@NonNull @Override public WindowInsetsCompat onProgress( @NonNull WindowInsetsCompat insets, @NonNull List<WindowInsetsAnimationCompat> runningAnimations ) { // Find an IME animation. WindowInsetsAnimationCompat imeAnimation = null; for (WindowInsetsAnimationCompat animation : runningAnimations) { if ((animation.getTypeMask() & WindowInsetsCompat.Type.ime()) != 0) { imeAnimation = animation; break; } } if (imeAnimation != null) { // Offset the view based on the interpolated fraction of the IME animation. view.setTranslationY((startBottom - endBottom) * (1 - imeAnimation.getInterpolatedFraction())); } return insets; }
必要に応じて、onEnd
をオーバーライドできます。このメソッドは、アニメーションが終了した後に呼び出されます。このタイミングで、一時的な変更をクリーンアップすることをおすすめします。
参考情報
- GitHub の WindowInsetsAnimation